This tutorial will teach you how to access the Coinbase Pro API using Python. We’ll use the coinbase-pro library and the requests library to make API calls. We’ll also show you how to authenticate and access private API endpoints. This tutorial is for anyone who wants to learn how to use the Coinbase Pro API in Python, whether you are a beginner trader or an experienced developer.
What Is Coinbase Pro?
Coinbase Pro is an online cryptocurrency exchange platform offering a simple and secure way to instantly buy, sell, and trade digital assets across various trading pairs. Coinbase Pro also lets you get cryptocurrency information, such as the current price, volume, ticker information, and trades for a cryptocurrency.
Brian Armstrong and Fred Ehrsam founded Coinbase in May 2012. Coinbase headquarters are located in San Francisco, USA. Coinbase Pro is available in more than 100 countries.
Coinbase and Coinbase Pro are subsidiaries of the same parent company. Coinbase is geared towards beginner traders interested in buying, selling, exchanging, and receiving funds through a virtual wallet. Coinbase Pro, as the name suggests, targets advanced users who want to buy, sell, store, withdraw, and trade digital assets via an online cryptocurrency exchange.
What Is the Coinbase Pro API?
Coinbase Pro API is a REST API allowing users to access various Coinbase Pro endpoints in code. The Coinbase Pro API allows you to develop customized financial software and trading bots using Coinbase Pro features.
Coinbase Pro Pros and Cons
Pros:
- Simple and Intuitive interface.
- Fees are lower for high-volume trading.
- Coinbase offers a rich API.
- Fully regulated by the US.
- High liquidity.
Cons:
- High fees compared to other online exchanges.
- Slow customer support.
- Not beginner-friendly.
Coinbase Pro Pricing Plans
You can create a free account with Coinbase Pro, which allows you to use the Coinbase Pro API without any fee. However, you must pay trading fees for all your trading activities.
Coinbase Pro follows a tiered price plan depending on your 30-day USD trading volume.
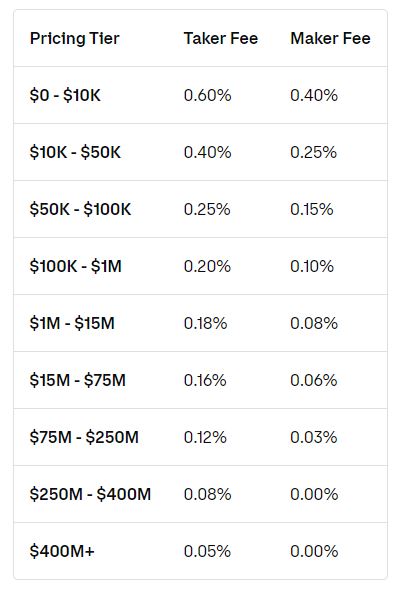
Coinbase Pro Price Plans – Image Source: Coinbase
Get Started with Coinbase Pro API
You must sign-up with Coinbase Pro before using the Coinbase Pro API endpoints.
If you only intend to test the API features, you can create a Coinbase Pro sandbox account.
The sign-up process is precisely the same.
If you already have a Coinbase account, you can use the same credentials to sign in to Coinbase Pro. Your accounts will be automatically integrated.
The Coinbase Pro sign-up page looks like this. Click the “Get started” button from the top right corner of the page and fill out the sign-up form.
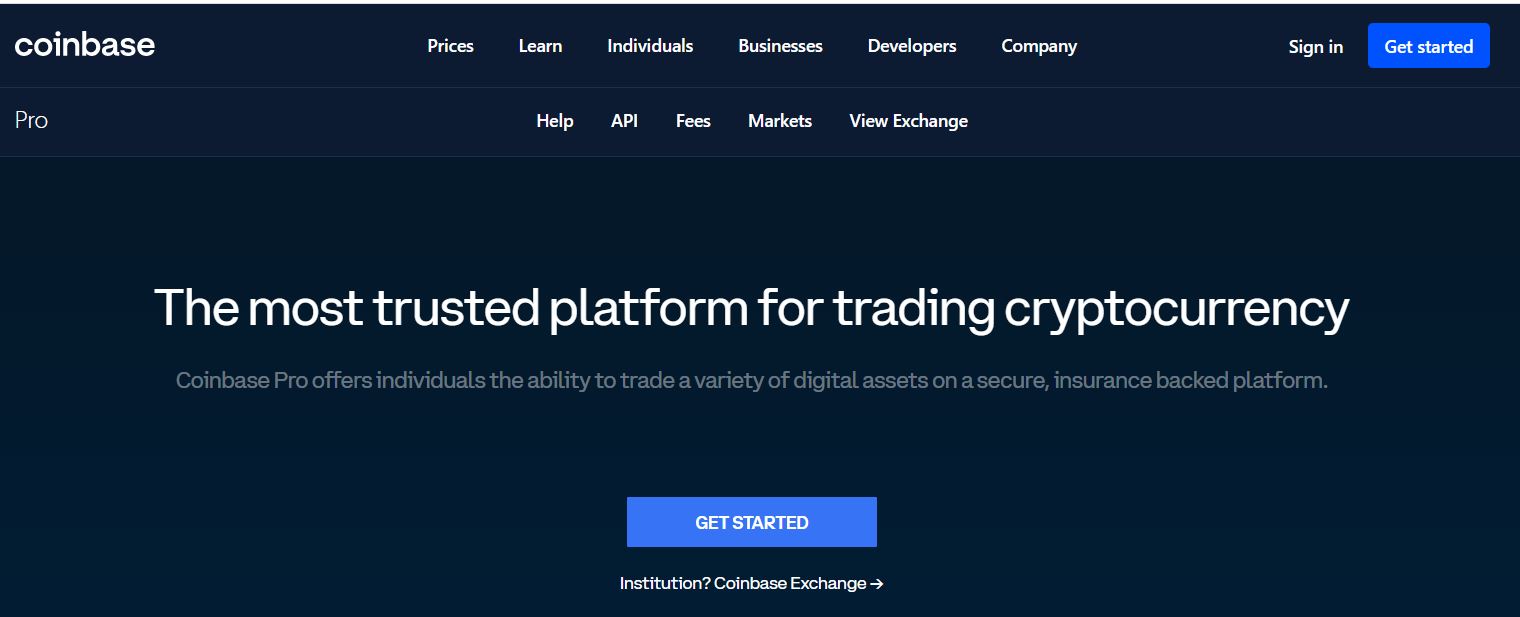
You need an API key, API secret, and passphrase to access the Coinbase Pro API endpoints.
To generate these credentials, sign in to your Coinbase Pro account and click on your profile avatar from the top-right corner of the dashboard. Click the “API” option from the dropdown list.
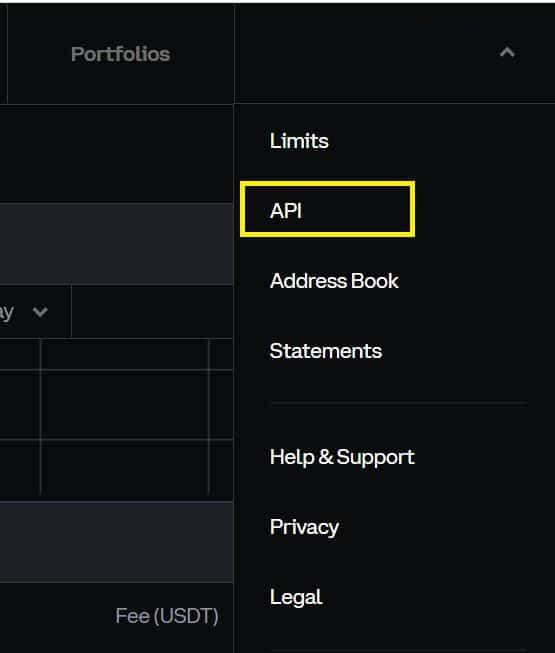
Click the “New API Key” button on the following screen.
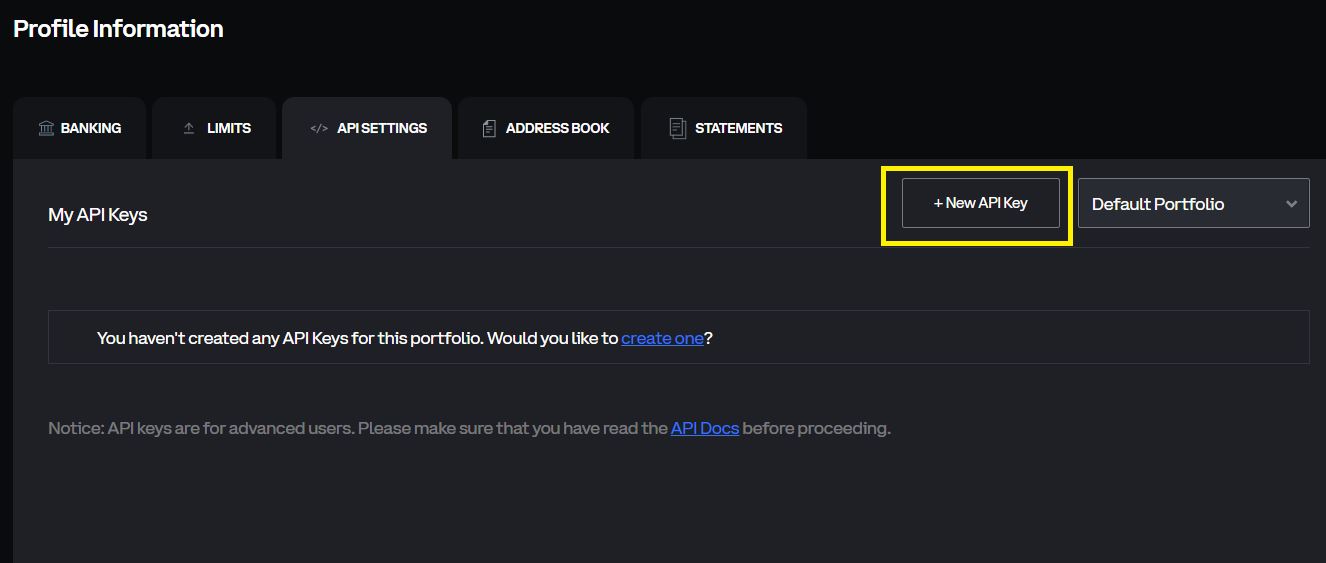
You will see the following window. Select all three trading permissions (View, Trade, Transfer) to access all of the API endpoints. You will see your passphrase on this screen. Save it at a secure place.
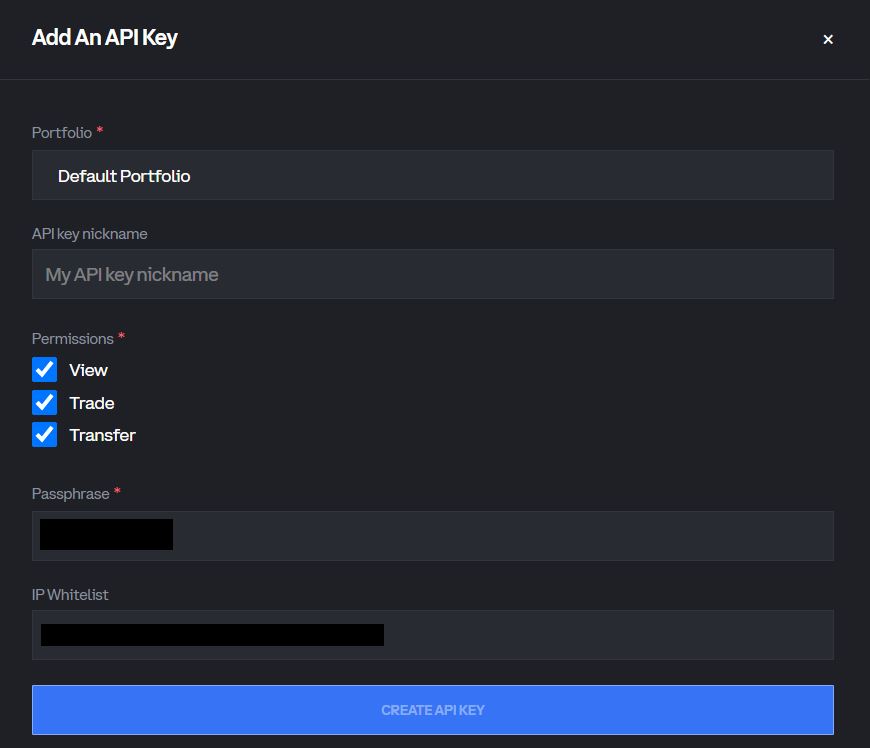
Click the “Create API Key” button. You will receive a verification code in your email, which you have to enter to generate an API key. Doing so displays the following window.
You will have to enable two-factor authentication before seeing the following window that displays your API secret. Save it in a safe place.
Click the “Done” button.
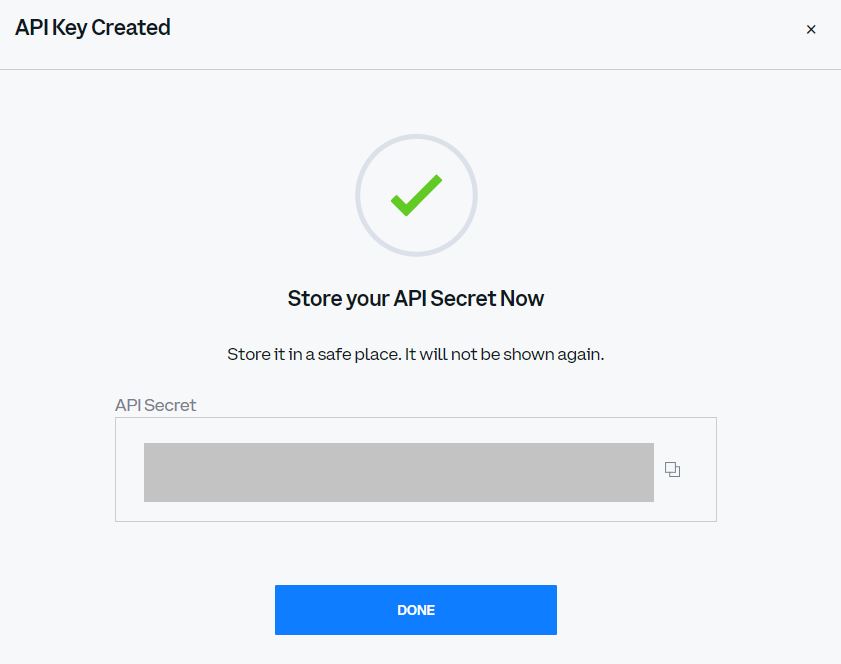
You will see the following screen where you can find all your existing and new API keys. Strangely, the API key, API secret, and passphrase are displayed at different places during the API key generation process.
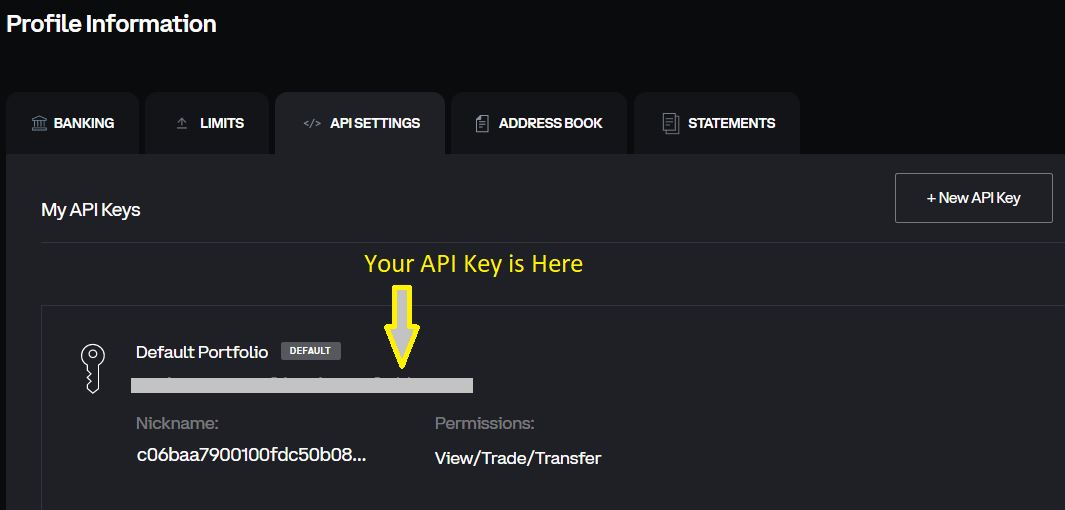
Fetching Data Using Coinbase Pro API in Python
There are a couple of ways you can access data using Coinbase Pro API in Python:
- Using the Python coinbase-pro client.
- Using the Python requests library.
Coinbase Pro API Coinbasepro-python Library Example
Run the following pip command to install the coinbase-pro library.
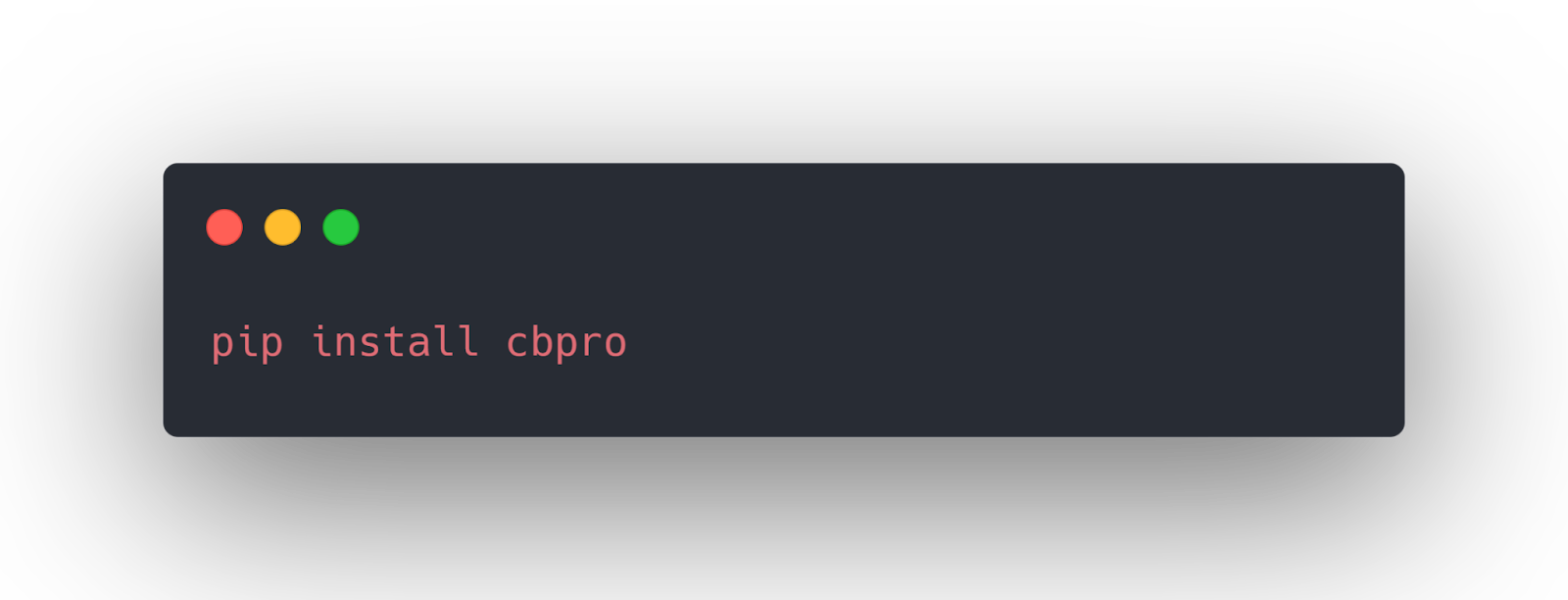
Coinbase-pro Library Public Client Example
The Coinbase API offers public and private endpoints. You can access the public endpoints without authenticating yourself via the API Key, API secret, and passphrases.
The coinbase-pro library implements the PublicClient() class that allows you to access the public endpoints of the Coinbase Pro library.
The following example shows that you can use the get_currencies() of the PublicClient() class to get the list of currencies that Coinbase Pro supports.
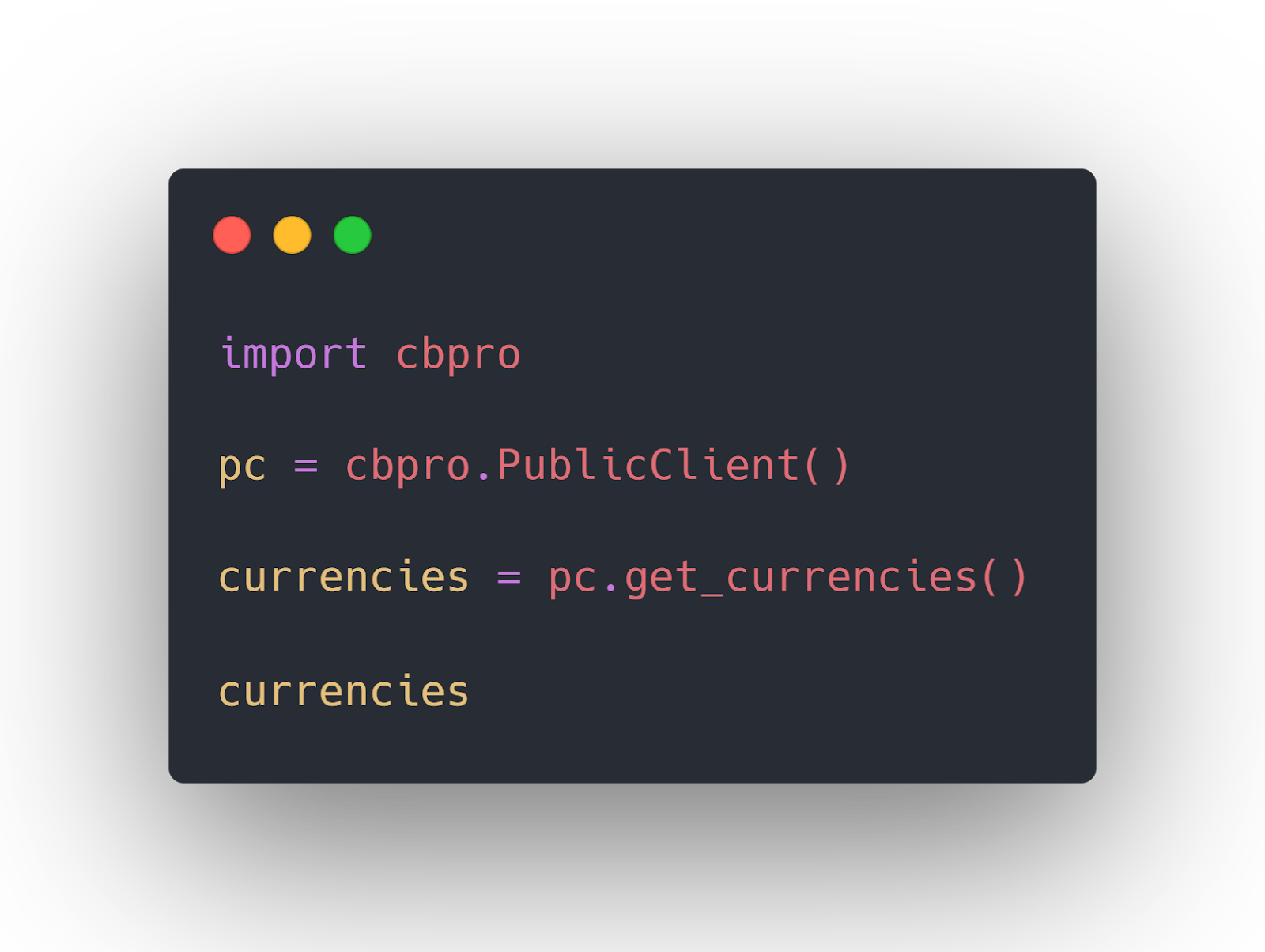
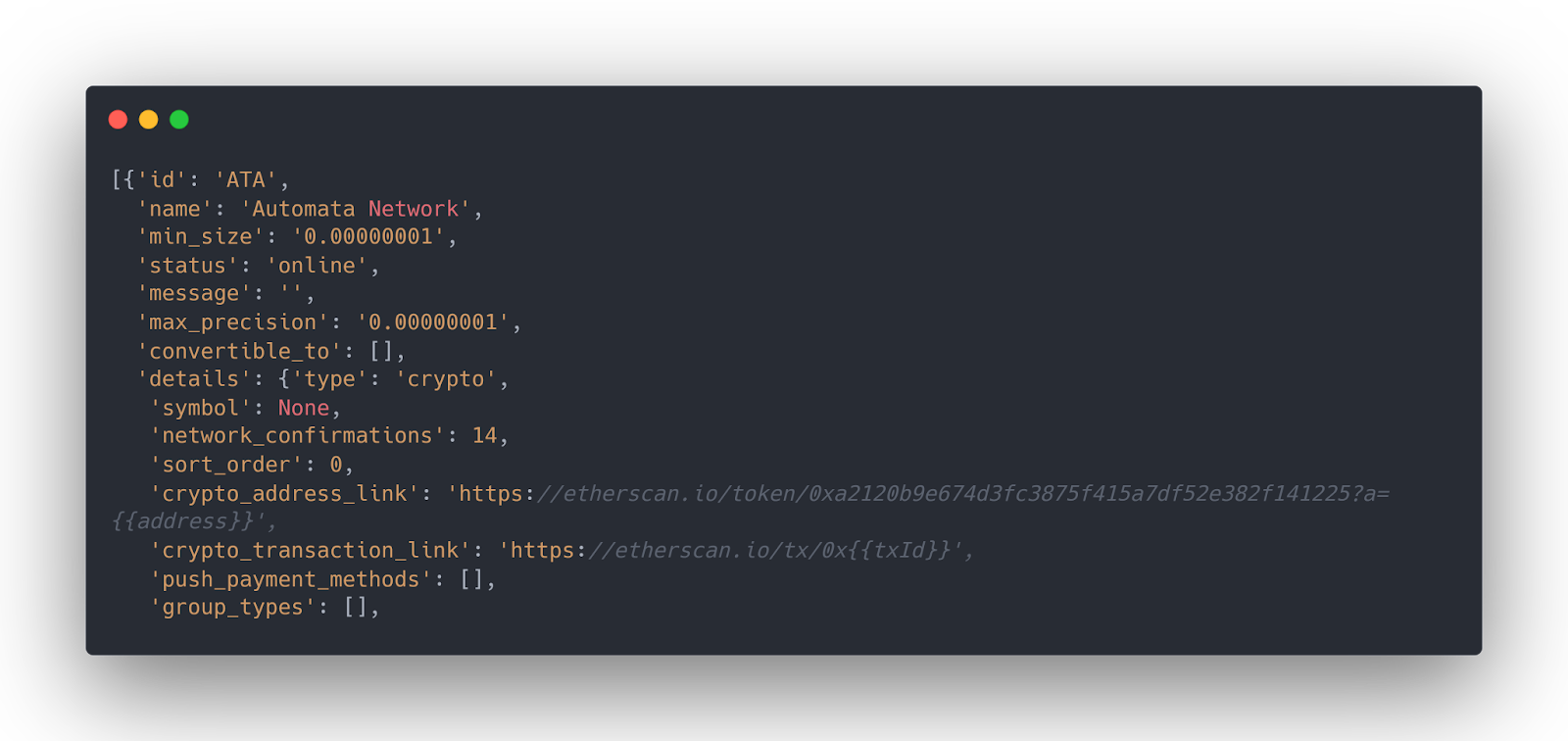
Whether you use a real Coinbase Pro account or a Sandbox account you can access Coinbase Pro API public endpoints without authentication.
In the following sections, I will explain how to call the Coinbase Pro private endpoints via real and sandbox accounts.
Coinbase-pro Authenticated Real Account Client
To access private endpoints of the Coinbase Pro library, you can use the AuthenticatedClient class methods. You must pass your API Key, secret key, and passphrase to the class. I stored these credentials in environment variables, which you can access in your Python code via the Python os module.
For example, the following script shows how to get your account information using the get_accounts() method.
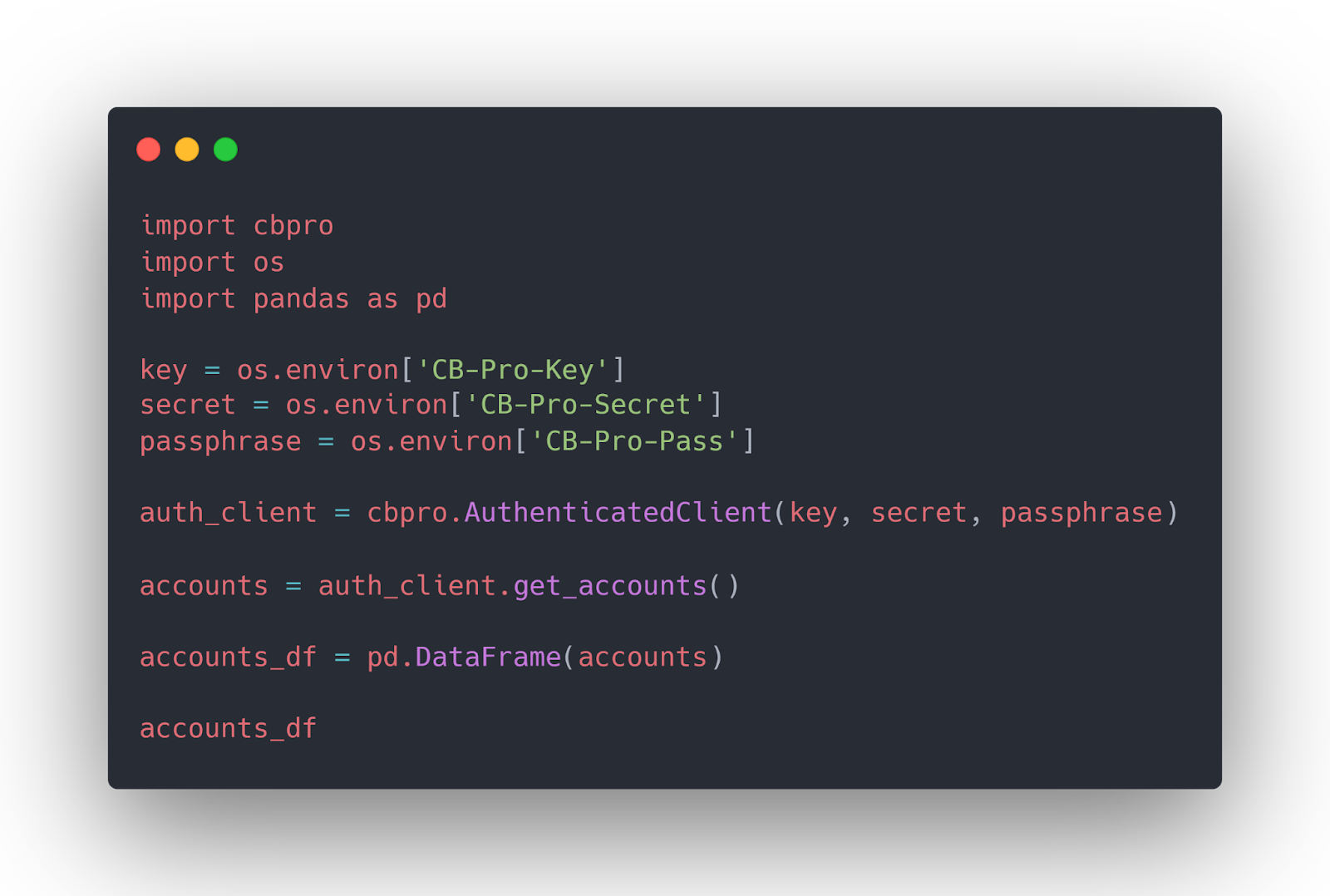
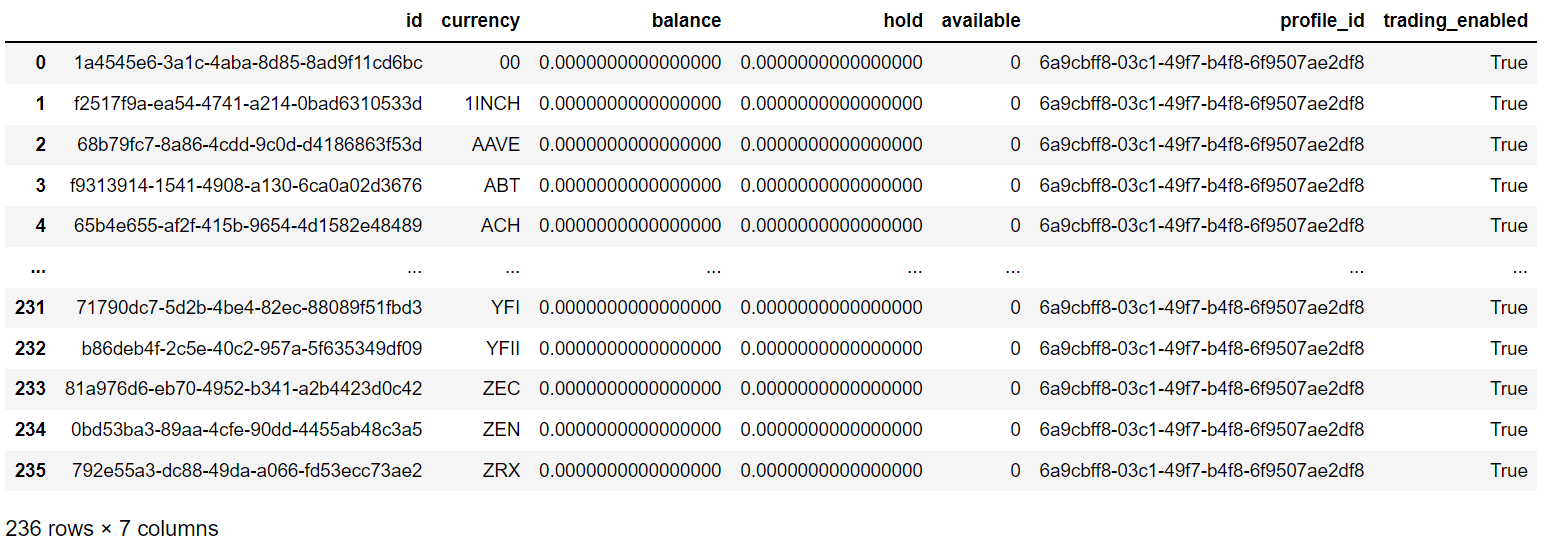
Coinbase-pro Authenticated Sandbox Client
To access the Coinbase pro sandbox account, you must pass the Sandbox pro API URL (https://api-public.sandbox.exchange.coinbase.com) to the api_url attribute of the AuthenticatedClient class constructor. The rest of the code remains the same.
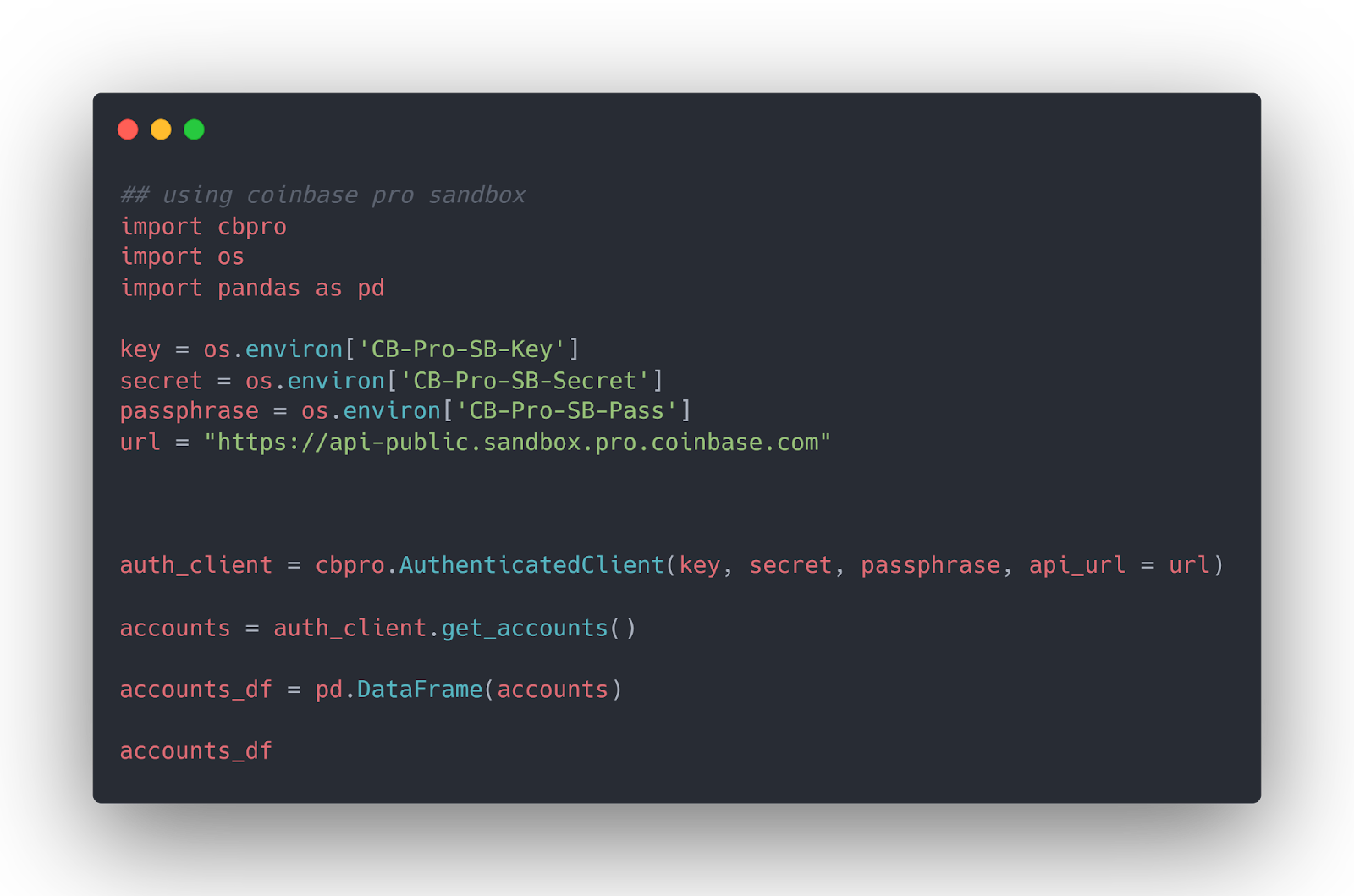
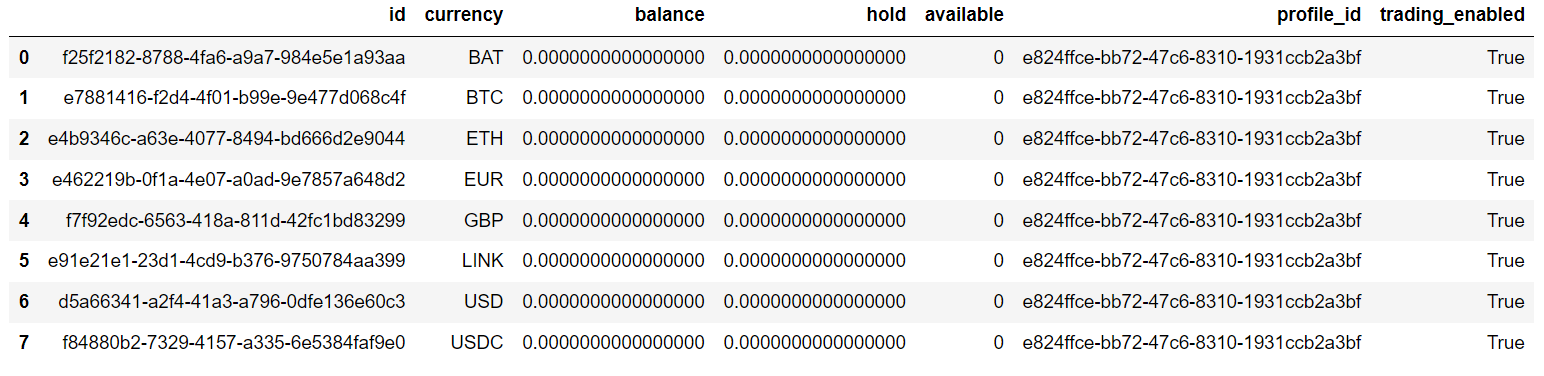
Coinbase Pro Python Request Library Example
The Python coinbase-pro library doesn’t implement all endpoints of the Coinbase Pro API. In such cases, you can use the Python requests library.
REST API Public Client Example
As with the coinbase-pro library, you can access the Coinbase Pro API public endpoints without passing authentication information to the requests library functions.
For example, the following script returns all Coinbase Pro currencies via the requests library’s get() function.
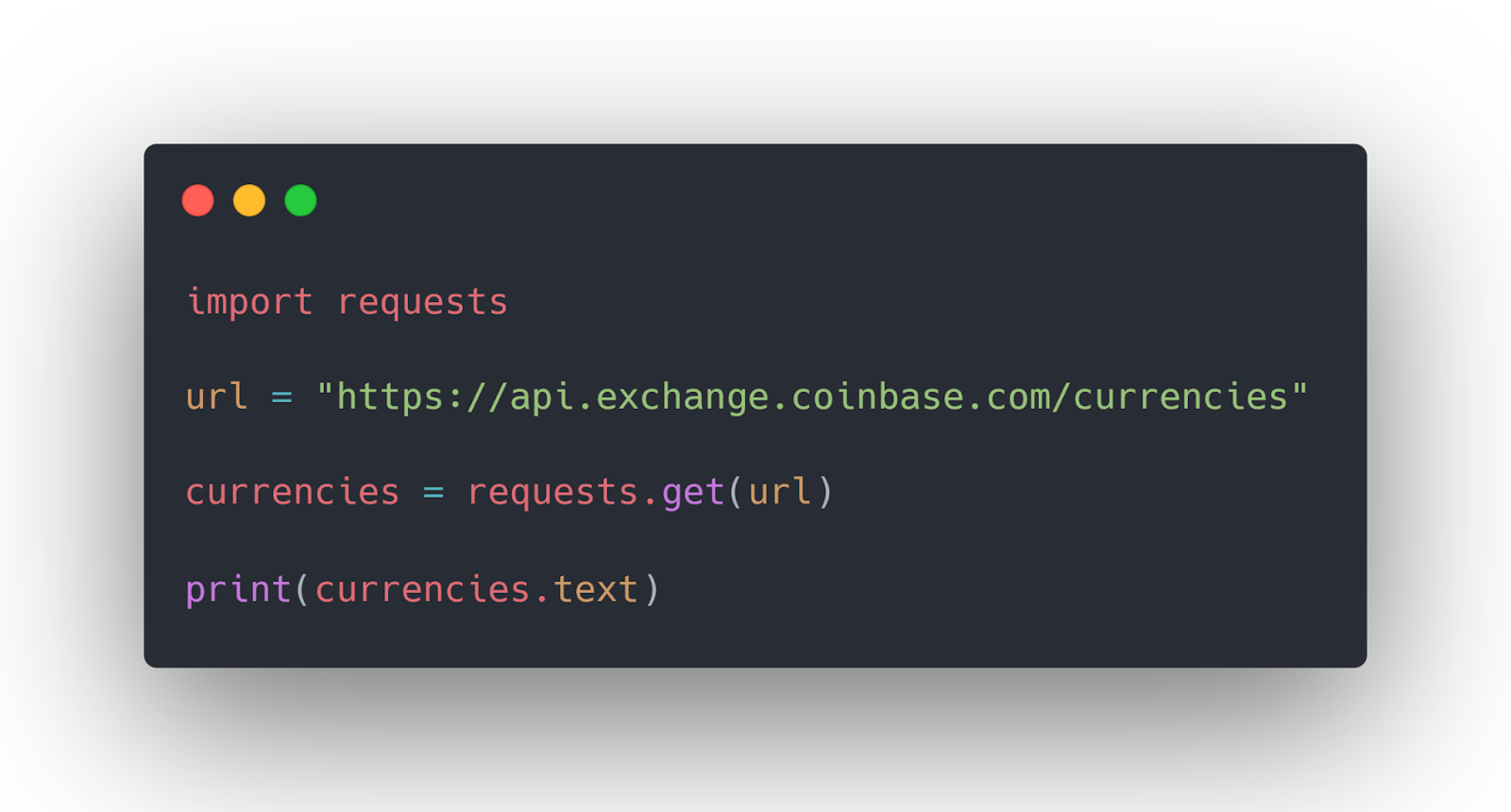
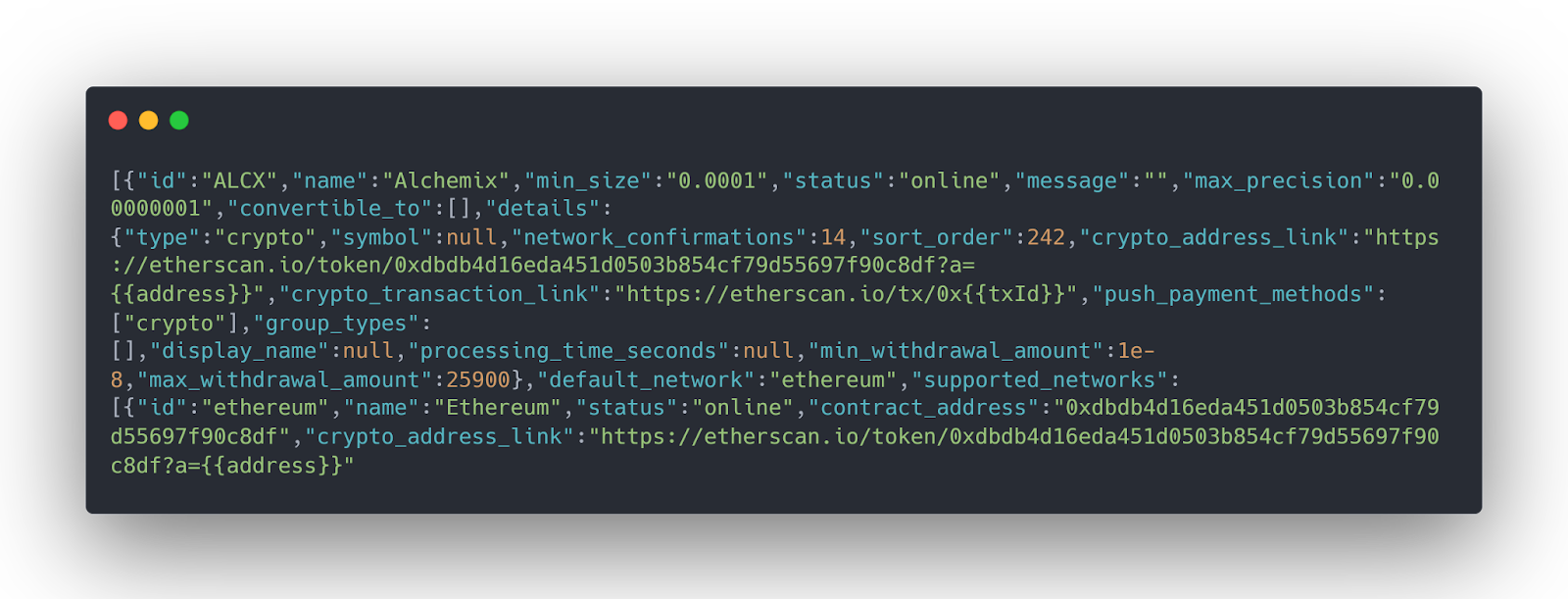
REST API Authenticated Real Account Client
Accessing Coinbase Pro API endpoints via the requests function is a bit tricky. The Coinbase Pro documentation explains the authentication process. However, I found an easy way to authenticate requests library functions using the coinbase-pro library’s CBProAuth class.
You need to instantiate CBProAuth class and pass it to the auth attribute of your requests library functions.
For example, the following Python code shows how to get your account information via the Python requests library’s get() function that makes a REST API call to the Coinbase Pro API.
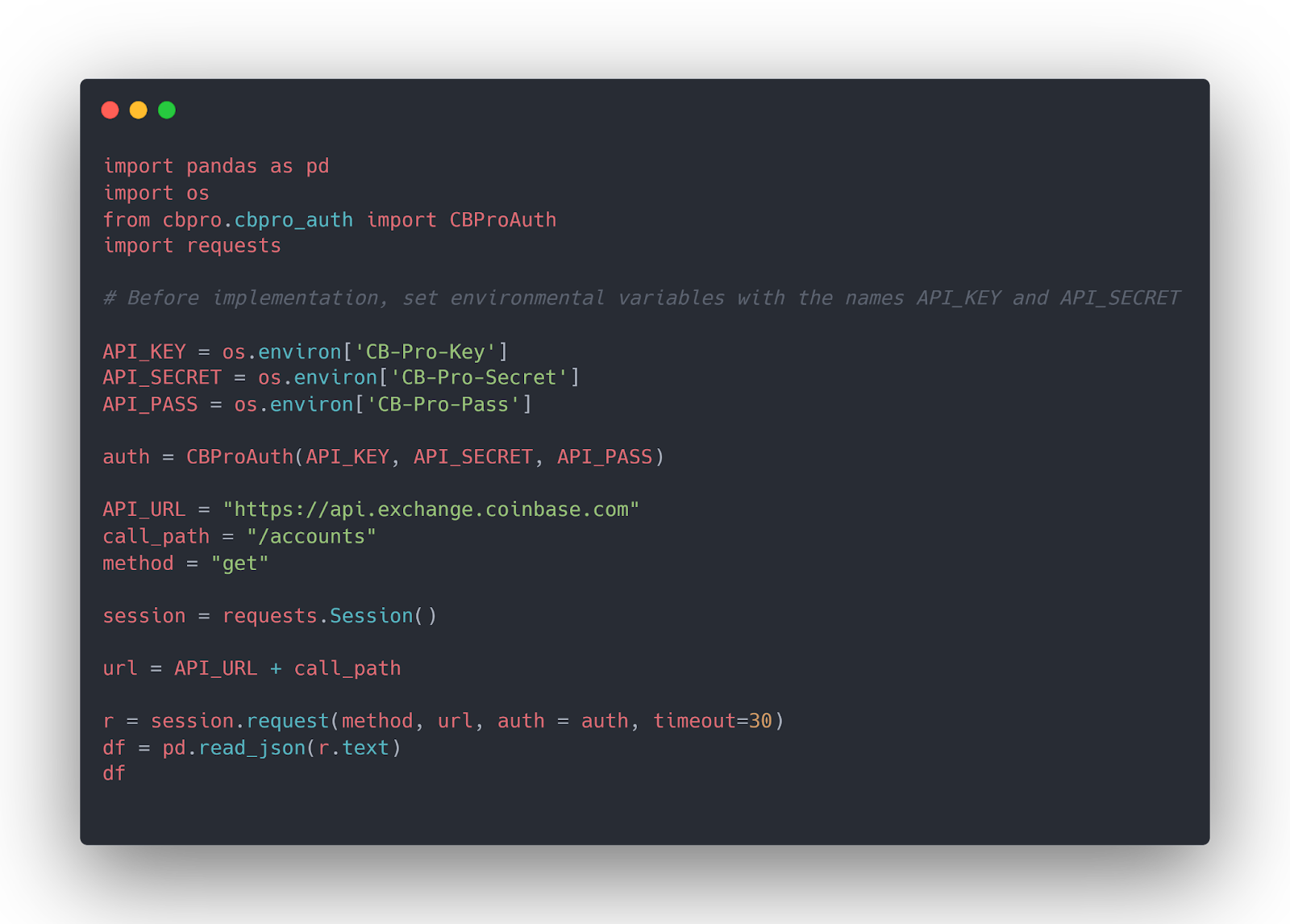
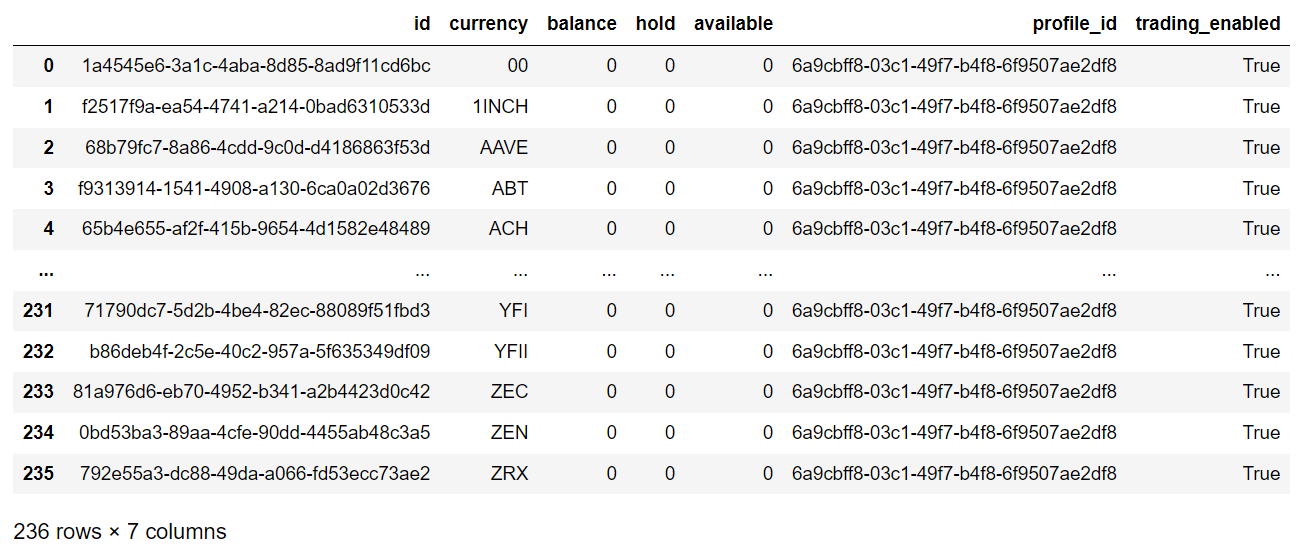
REST API Authenticated Sandbox Client
To make an authenticated REST API call to the Sandbox account via the requests library function, use the https://api-public.sandbox.exchange.coinbase.com URL.
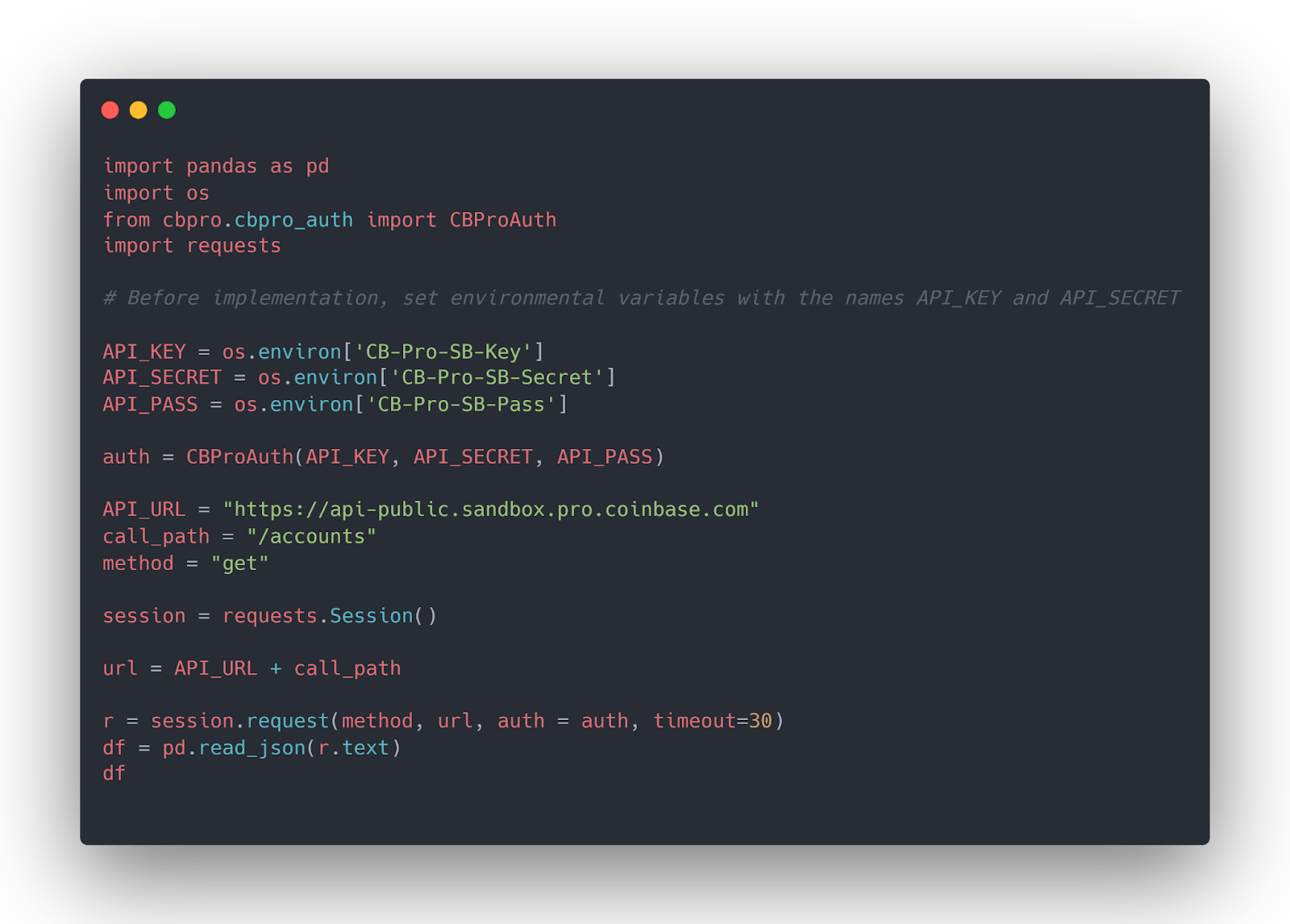
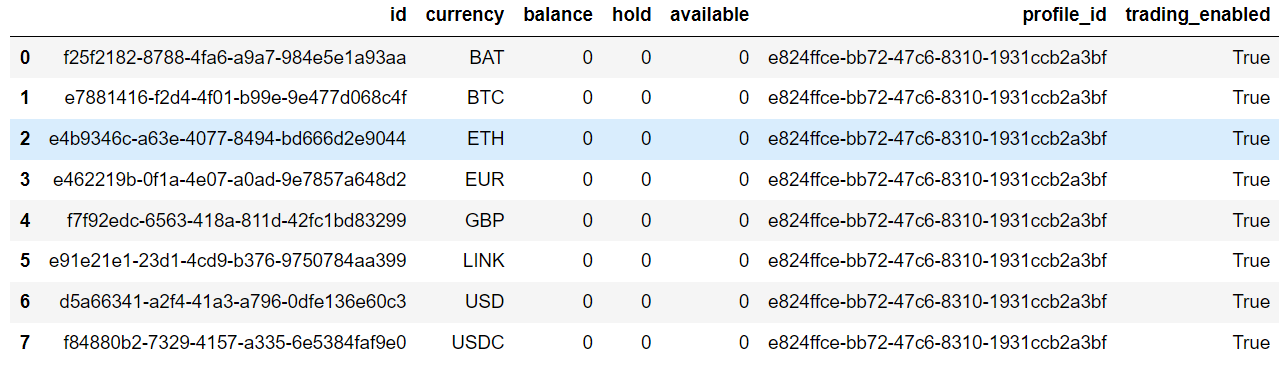
Coinbase Pro Websockets API Example
Websockets allow you to access real-time cryptocurrency information, e.g., ticker information, OHLCV information, etc.
Websockets with Real Account
To access Coinbase Pro API endpoints via Websockets, you must create a class inheriting the WebsocketClient class of the coinbase-pro library. The URL for the Websocket feed of a real account is wss://ws-feed.pro.coinbase.com/.
The on_open() method is called once before the socket opens. You can initialize the information you want to retrieve from the Coinbase Pro API.
The on_message() method is called every time a new response is received from the websocket feed of the Coinbase Pro API. You can print the retrieved information in this message.
The on_close() method is called once the Websocket has been closed.
The following script prints the latest ticker prices of ETH-USD after every second until 50 responses are received from the Websocket.
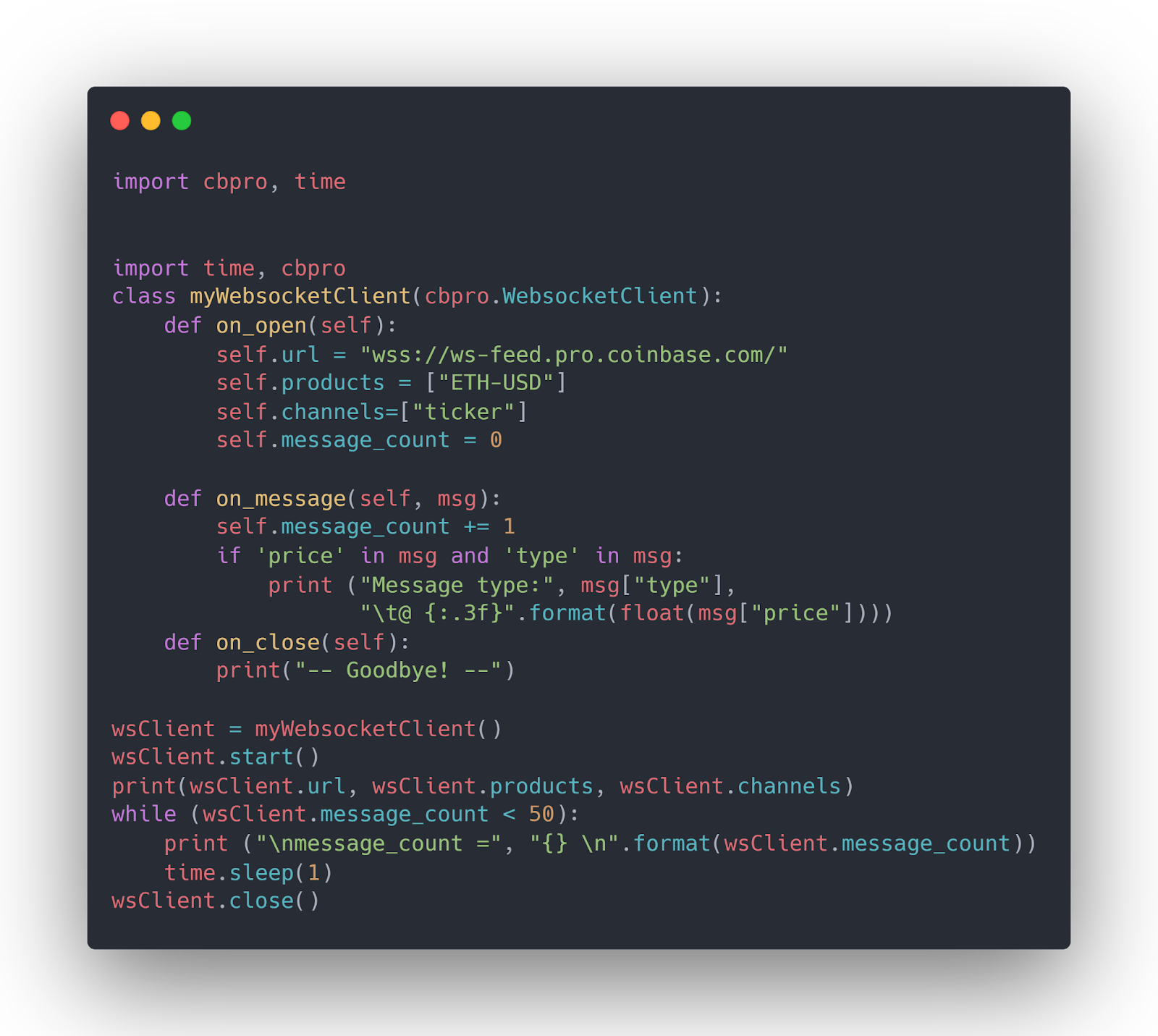
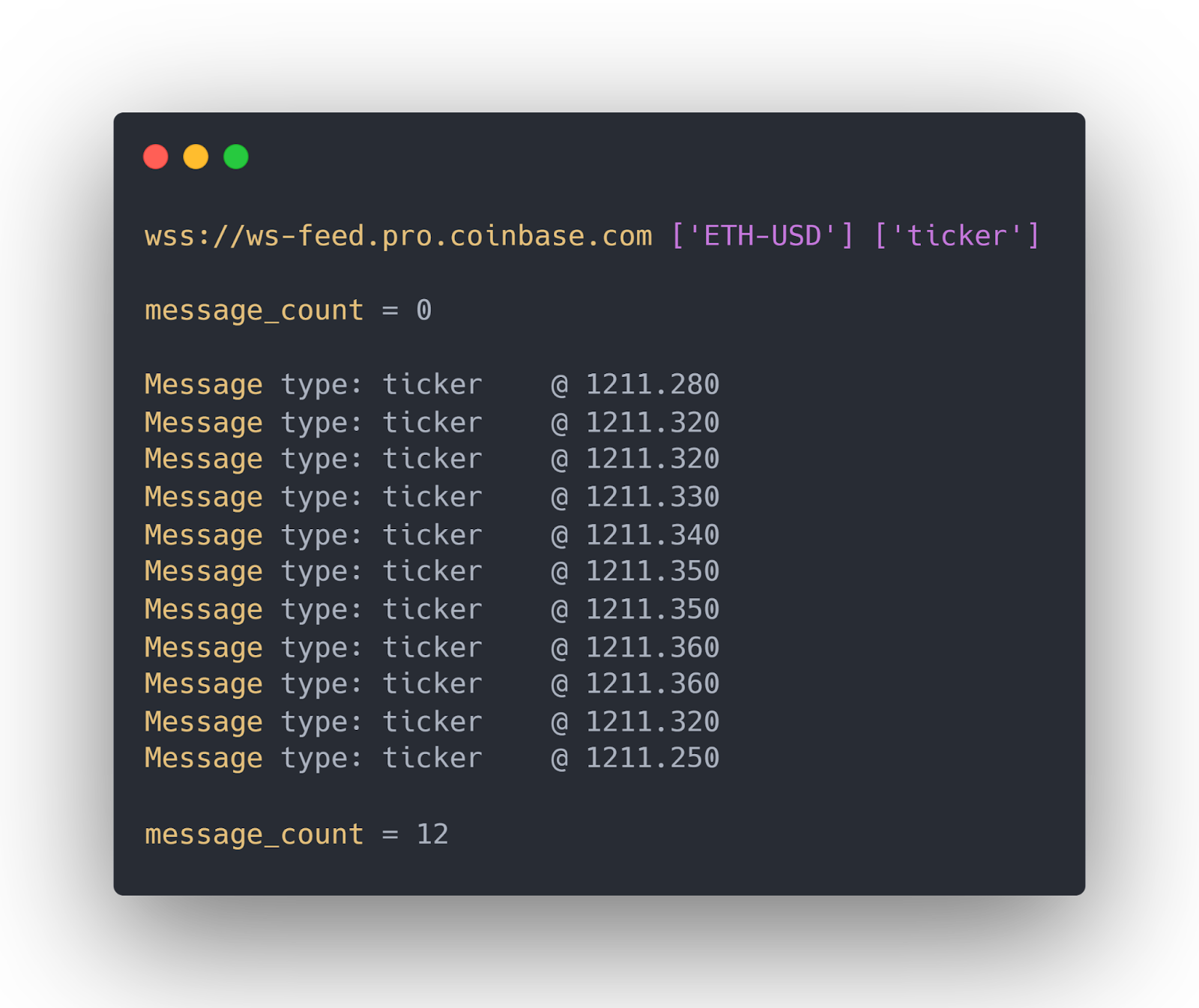
Websockets with Sandbox Account
Everything remains the same to access the sandbox account websocket feed as you see in the previous section, except that the Websocket feed URL, in this case, is: wss://ws-feed-public.sandbox.exchange.coinbase.com/
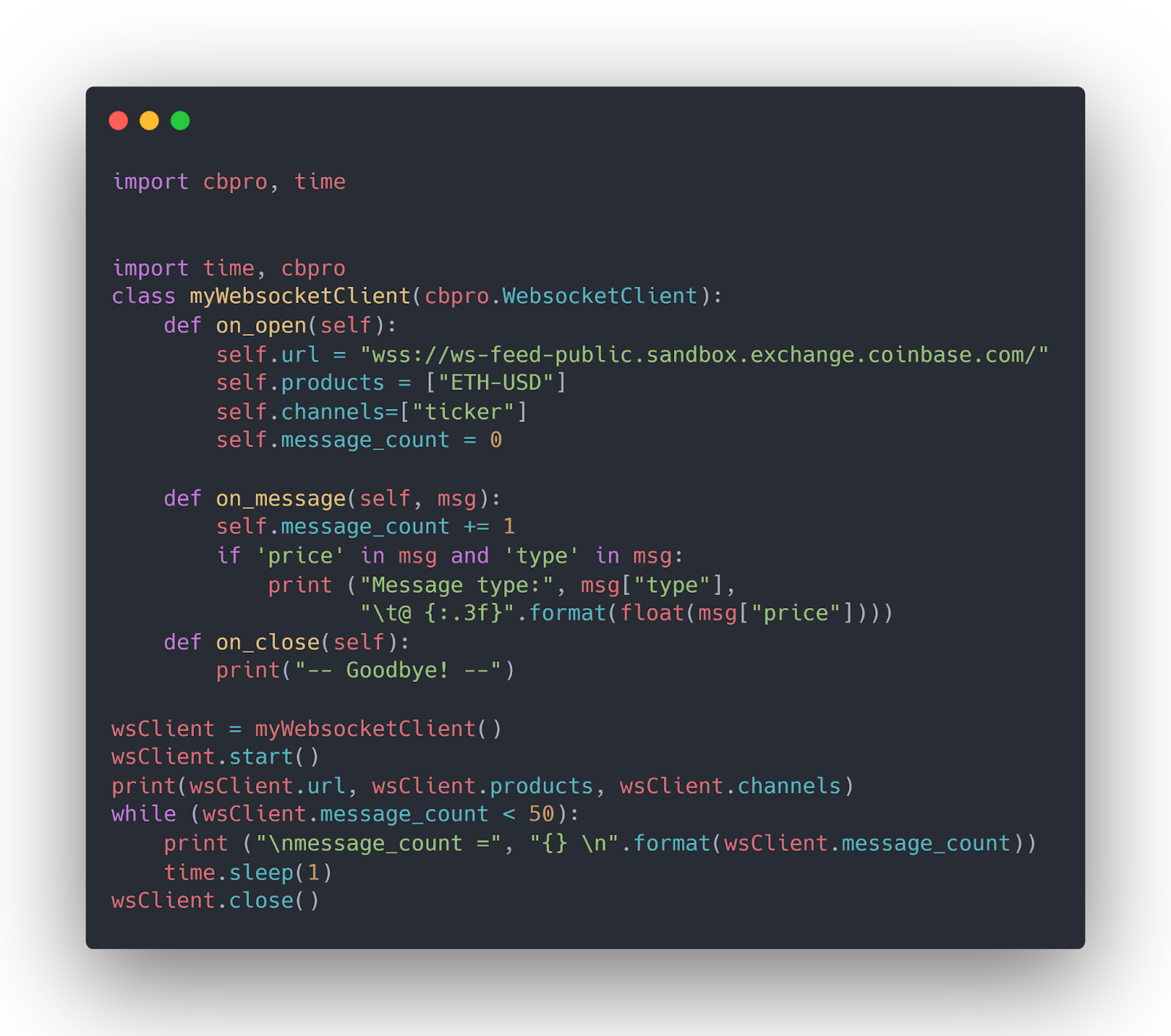
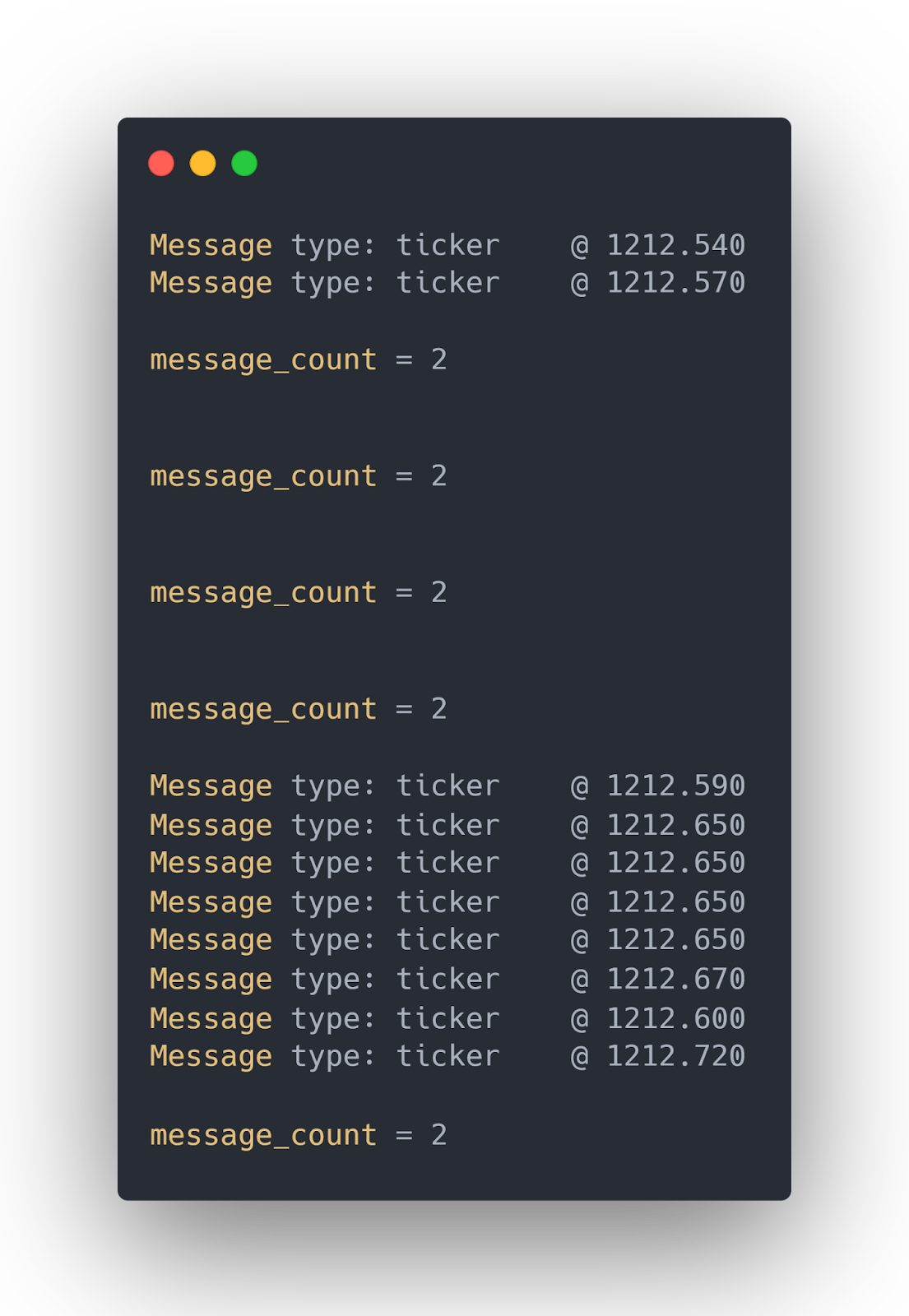
Coinbase Pro API Endpoints
The Coinbase Pro API divides its API functions into the following endpoints:
- Currencies
- Products
- Wrapped assets
- Accounts
- Address book
- Coinbase accounts
- Fees
- Coinbase Price Oracle
- Users
- Reports
- Conversions
- Transfers
- Orders
- Profiles
The endpoints of currencies, products, and wrapped assets contain public functions you can access without authentication.
See the Coinbase Pro API reference to get information about all the REST API calls for various endpoints.
Currencies Endpoint
You can get a list of all coinbase currencies and information about a single currency using the currencies endpoint functions.
Get All Coinbase Currencies
The get_currencies() method returns a list of all coinbase currencies.
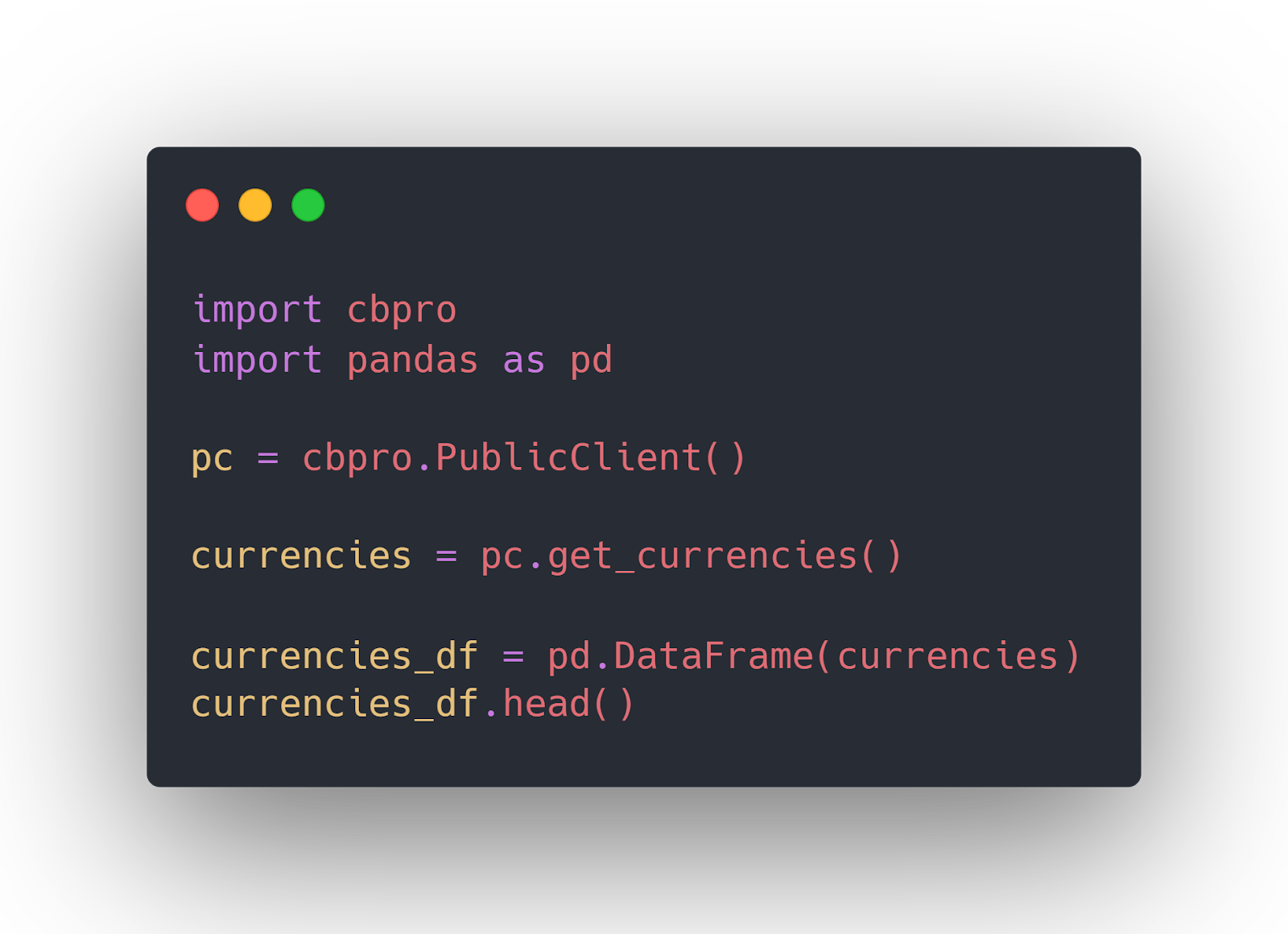
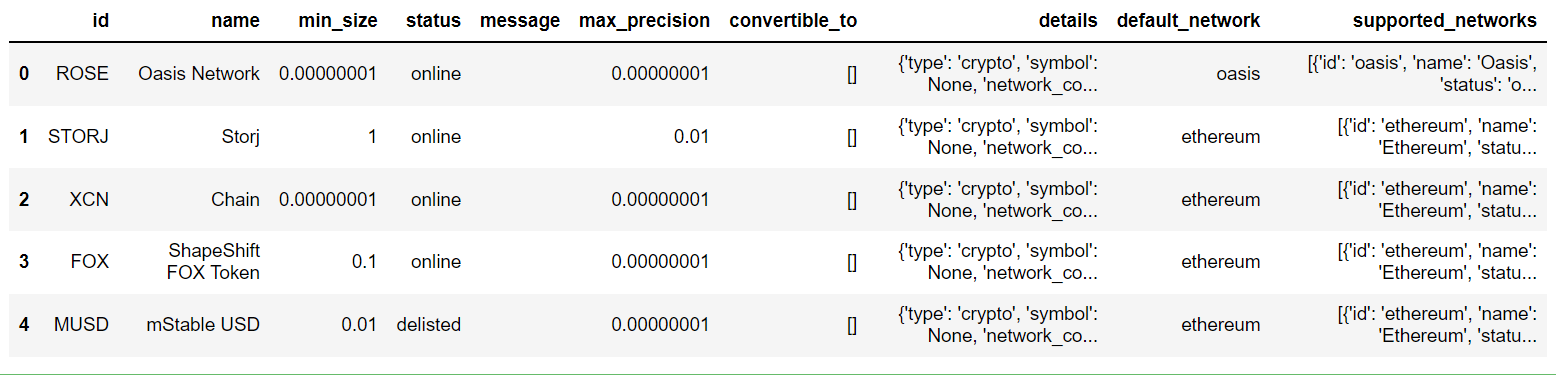
Get Single Currency Information with Coinbase
The coinbase-pro library doesn’t implement a function to retrieve details of a single currency. You can use the requests library in this case. For example, the following script returns details of Bitcoin.
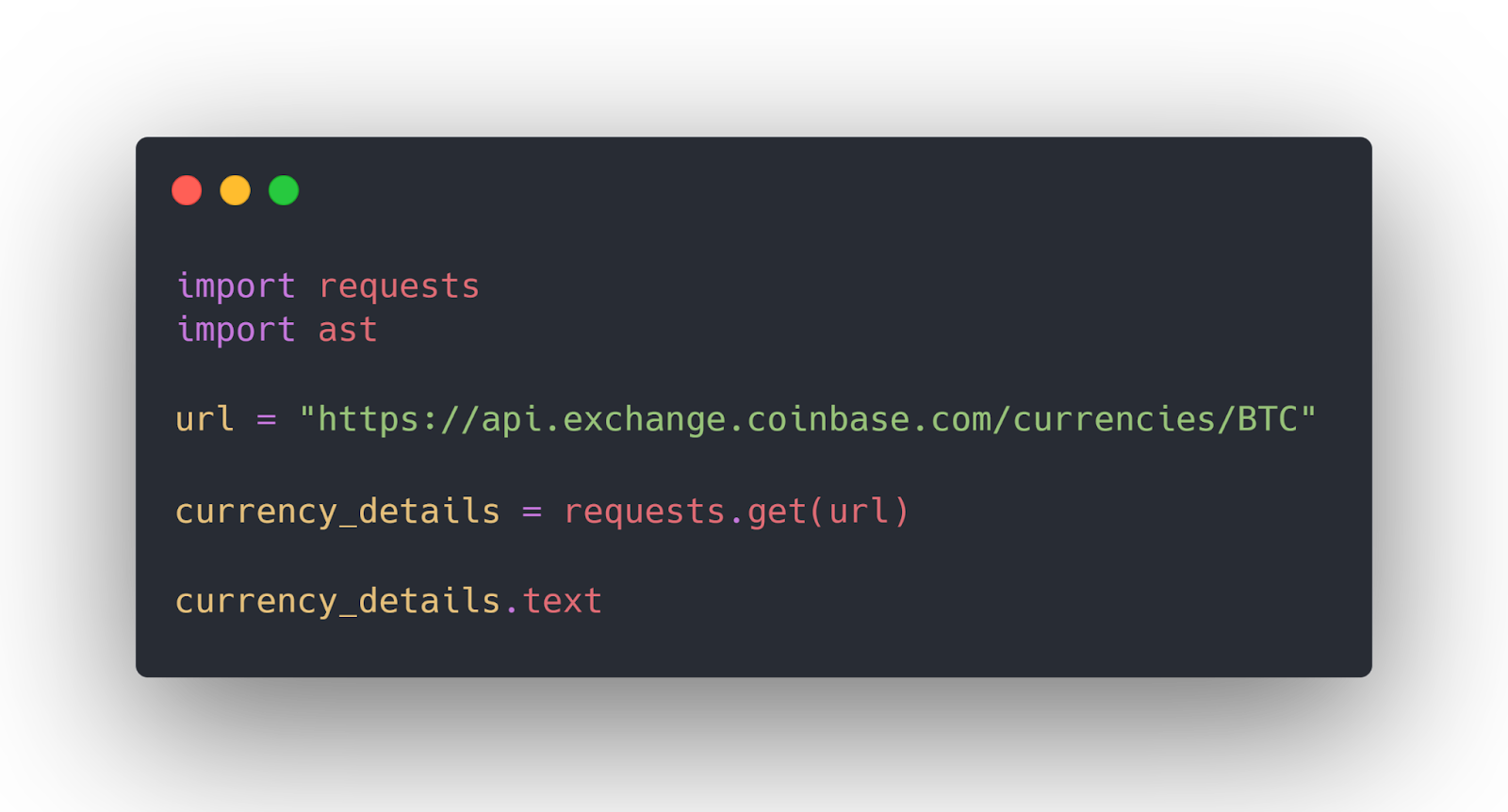
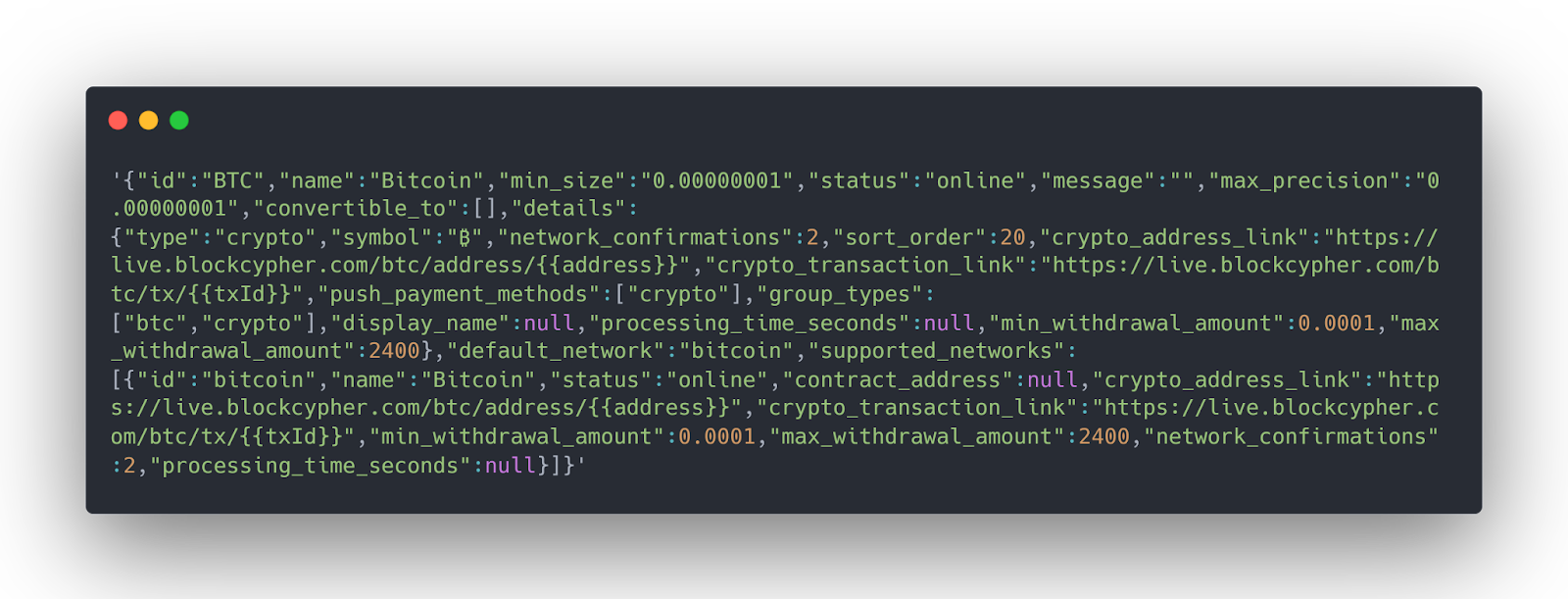
Products Endpoint
Product endpoint functions allow you to retrieve ticker prices, live product trades, and historical product data.
Get All Coinbase Trading Pairs
You can get a list of all coinbase trading pairs using the get_products() method from the PublicClient class.
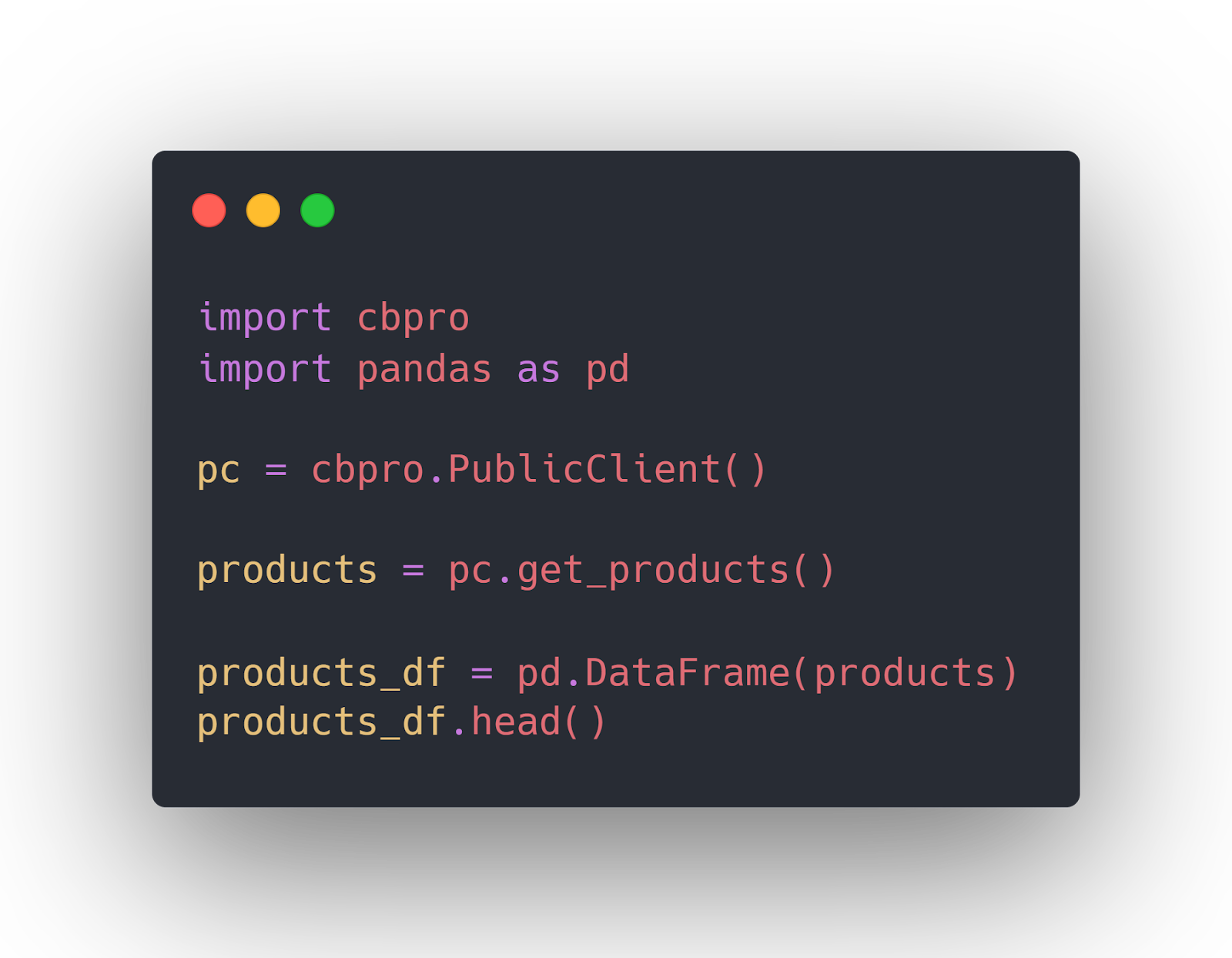
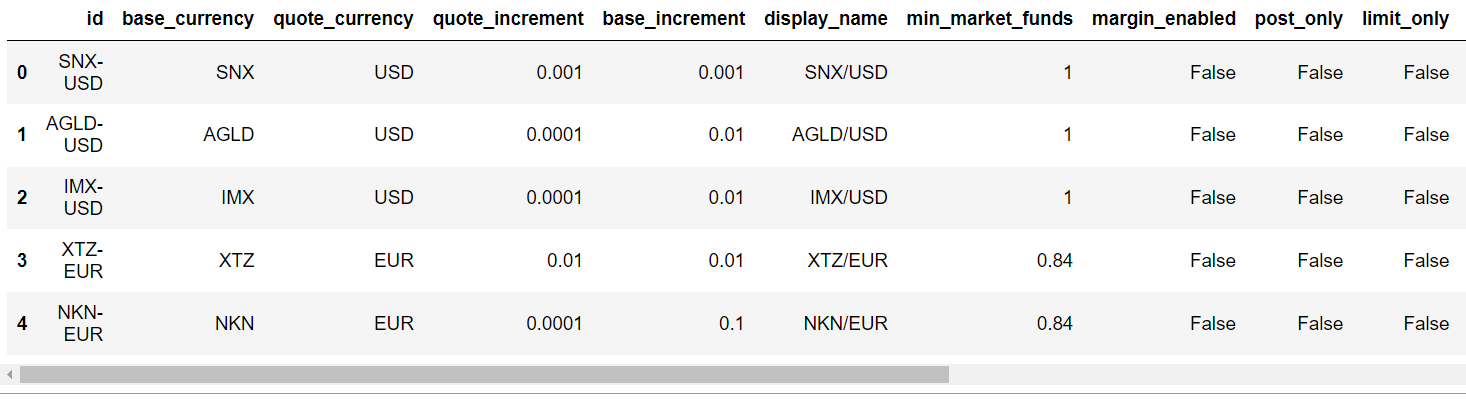
Get a Single Product
You can use the requests library to get information about a single product or a trading pair.
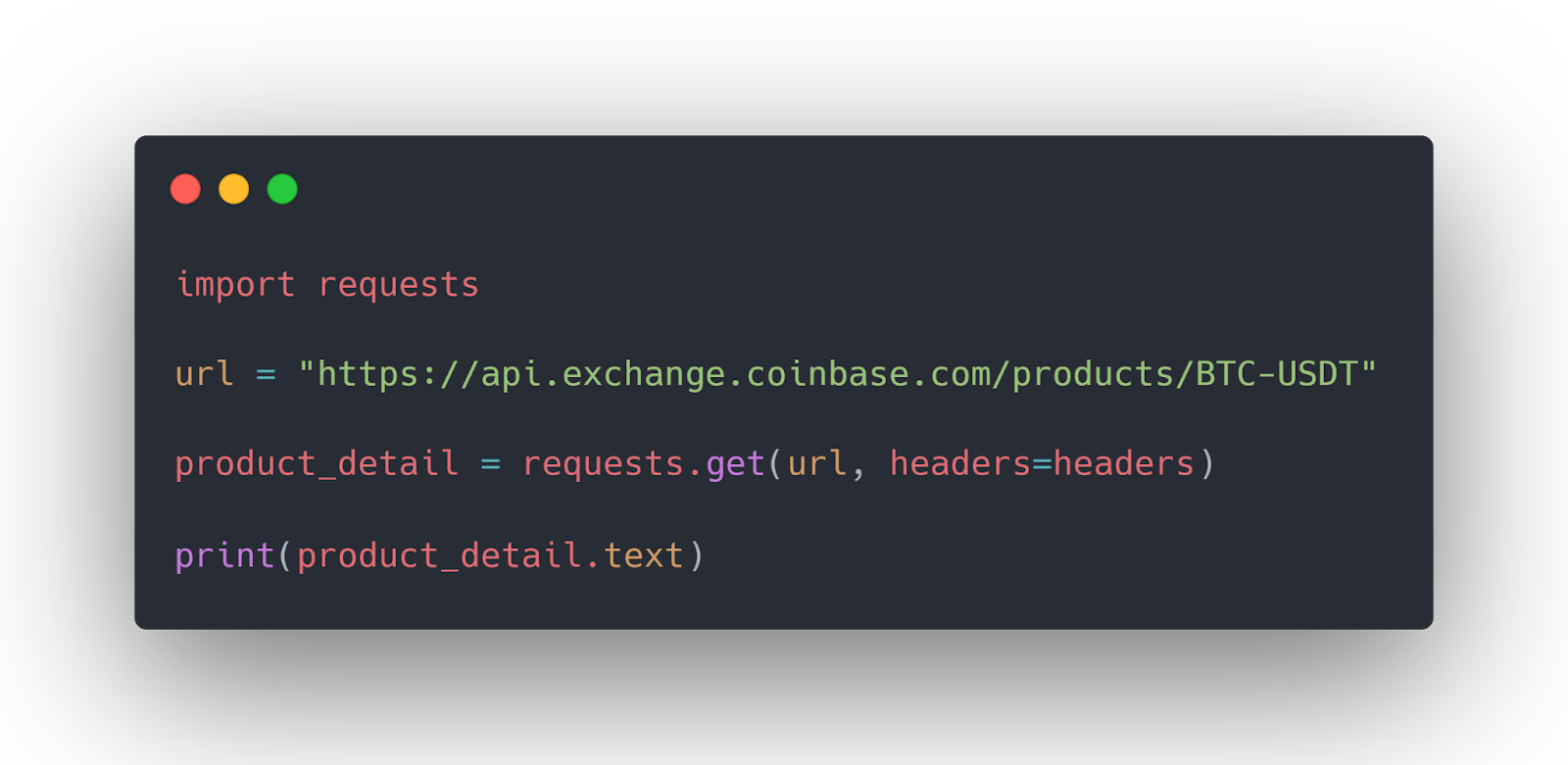
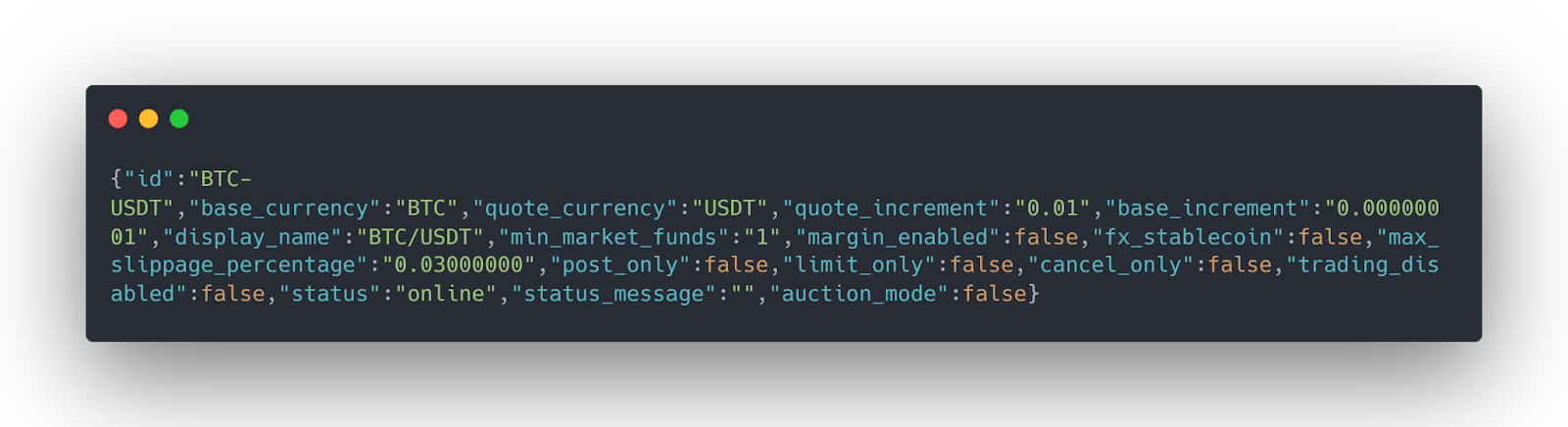
Get Coinbase Product Tickers
To get the product ticker for a trading pair, call the get_product_ticker() method of the PublicClient class.
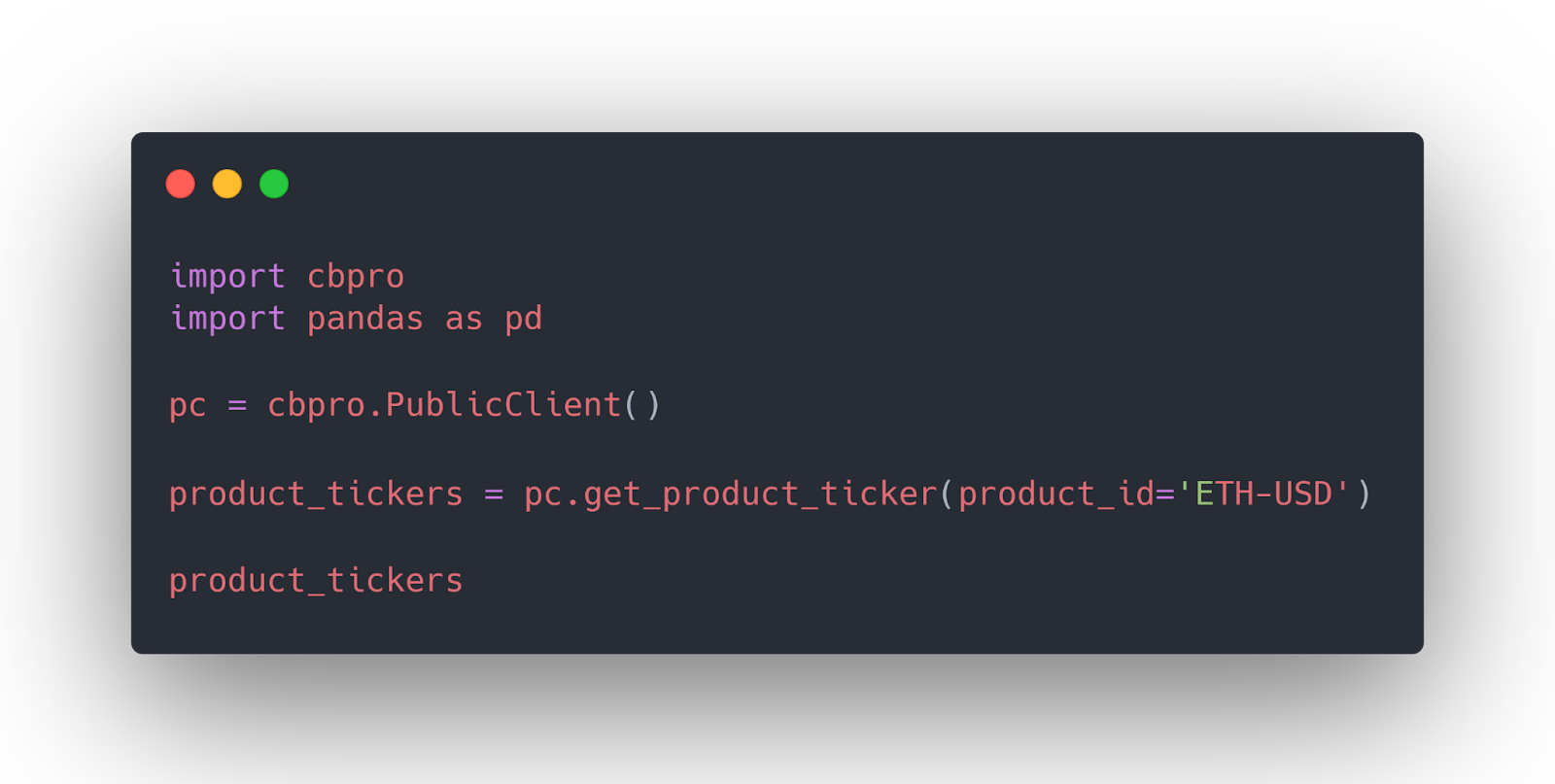
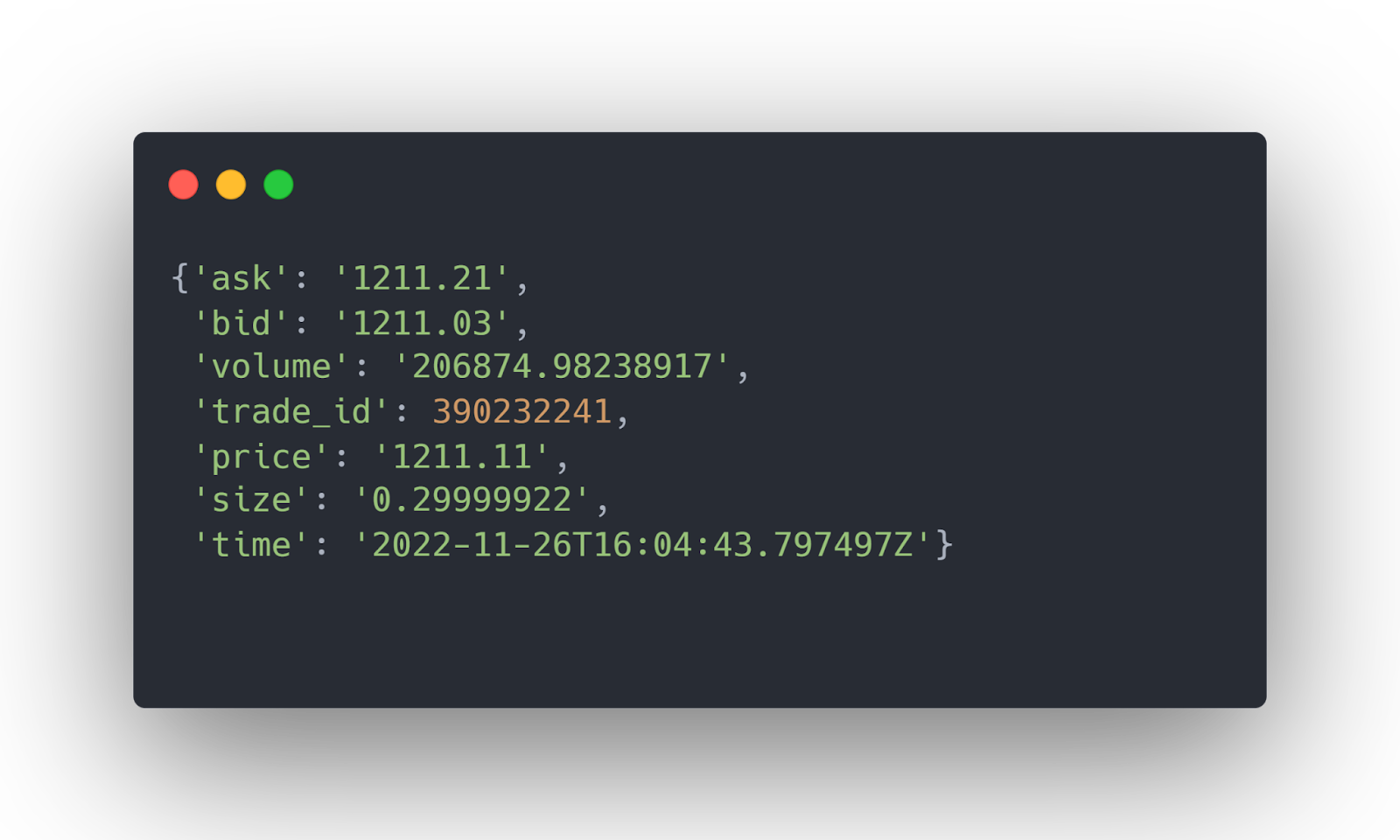
Get Coinbase Product Trades
To get a list of the latest trades for a product, you can use the get_product_trades() method, which returns a Python generator. You can iterate the generator to see the latest trade values.
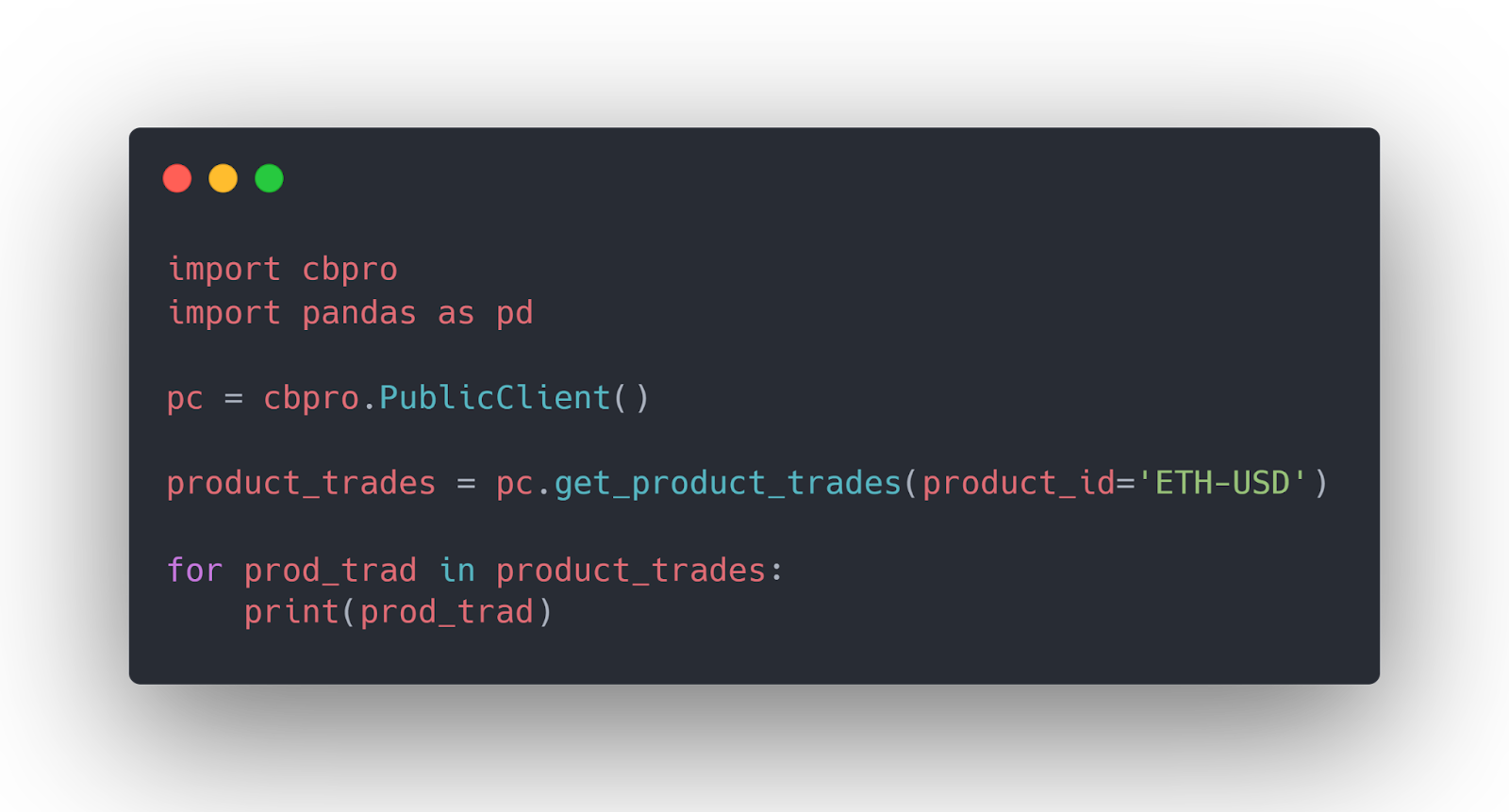
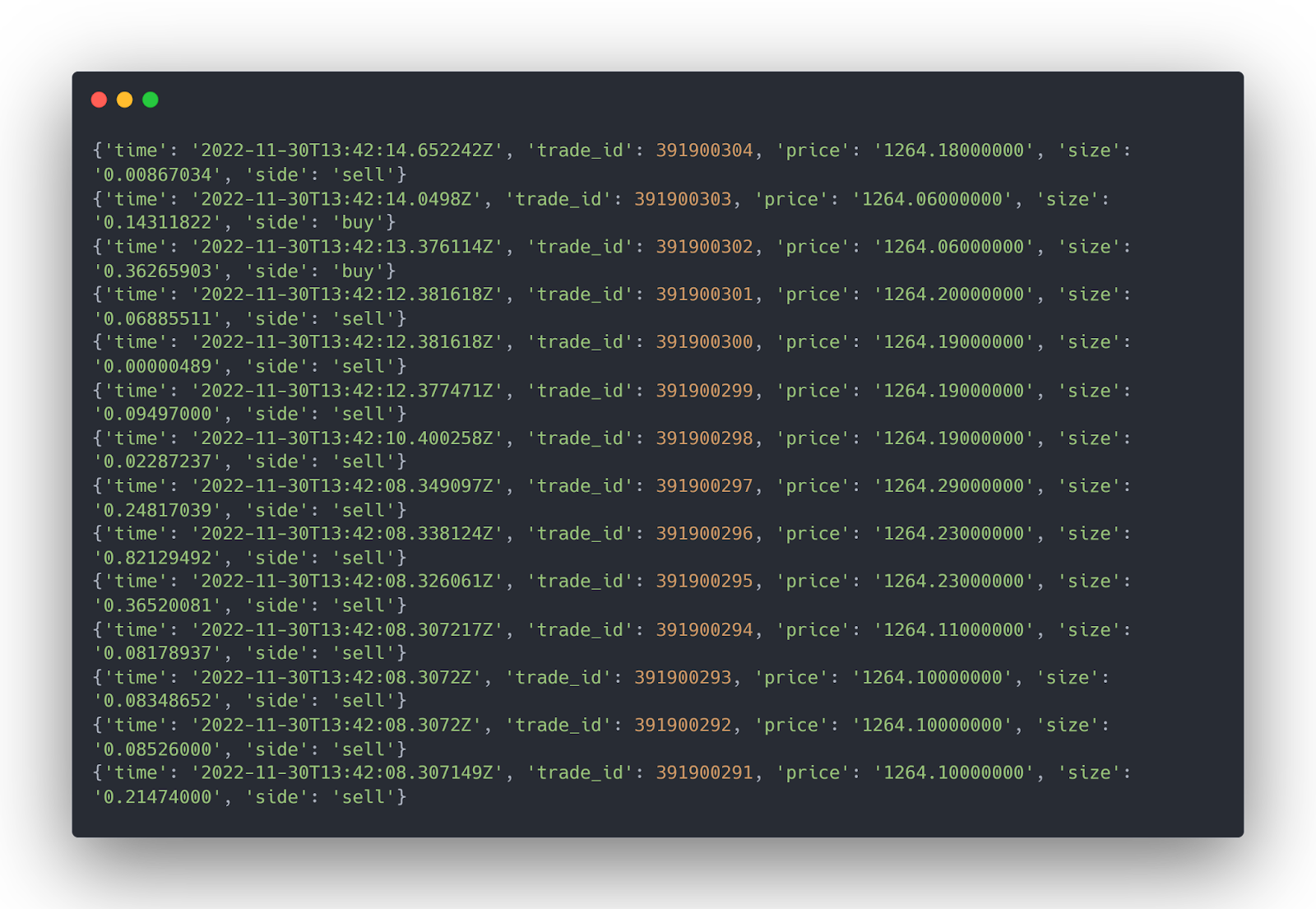
Get Historical Data for Trading Pairs
You can get historical market data for a trading pair using the get_product_historic_rates() method. You must pass the product symbol, start and end dates for retrieving data, and the granularity level (in seconds) to the get_product_historic_rates() method. The granularity levels must be passed in seconds, and the accepted values are 60, 300, 900, 3600, 21600, and 86400.
The following script returns historical low, high, open, close, and volume data for ETH-USD trading pairs for 20 days with a granularity of 24 hours (86400 seconds = 24 hours).
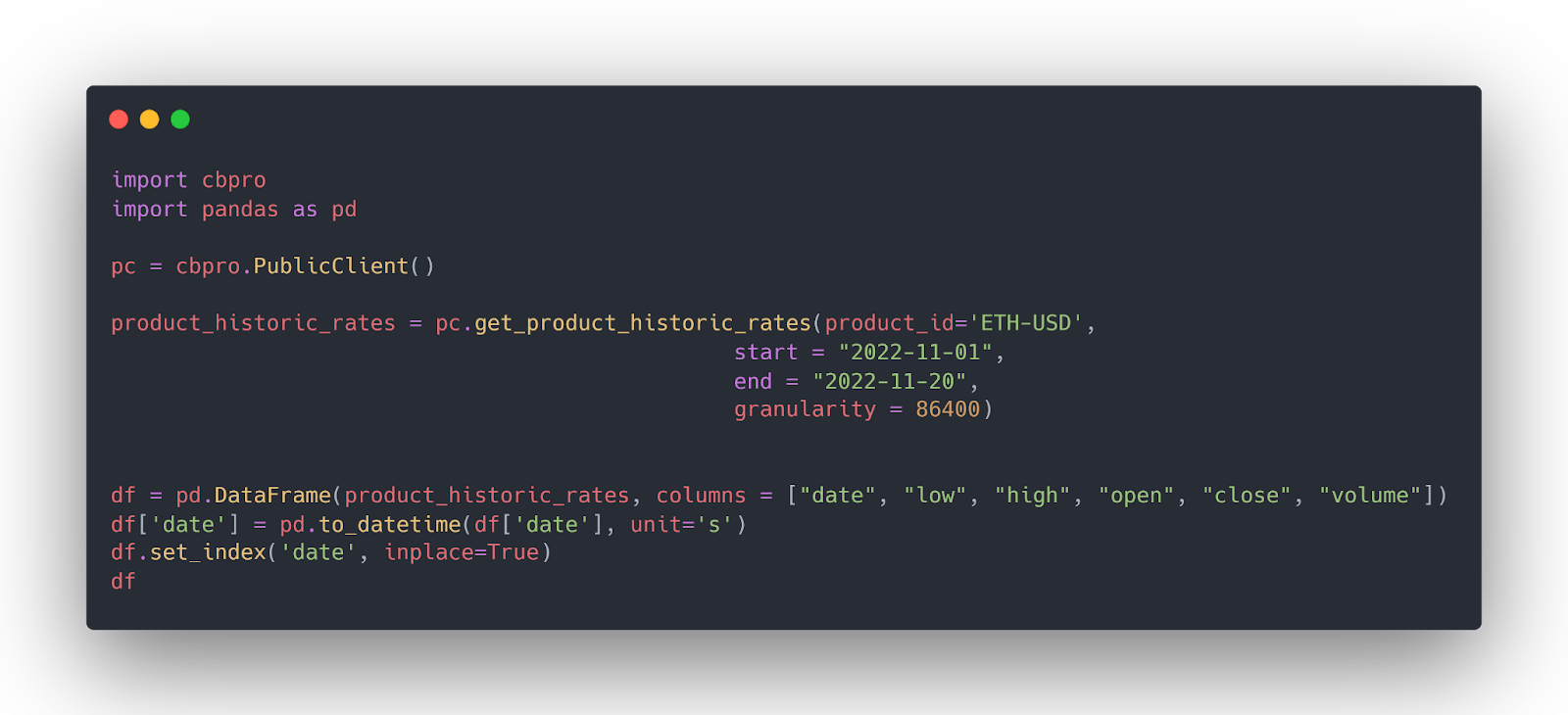
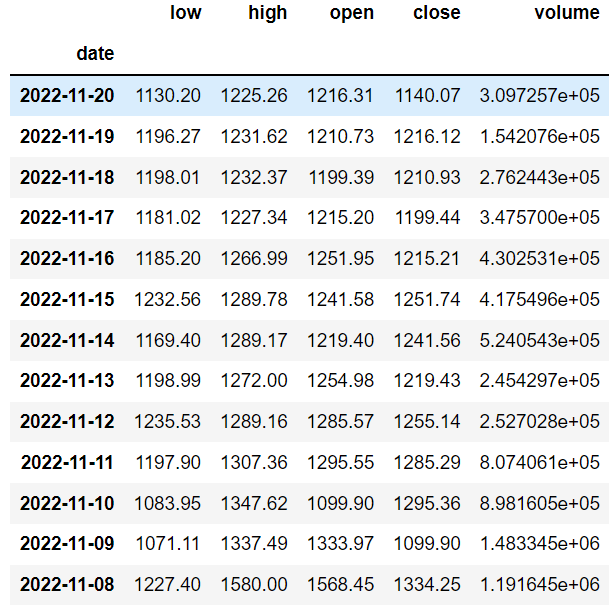
You can draw a line plot or Candlestick chart to plot the historical values. The following script shows an example of a line plot.
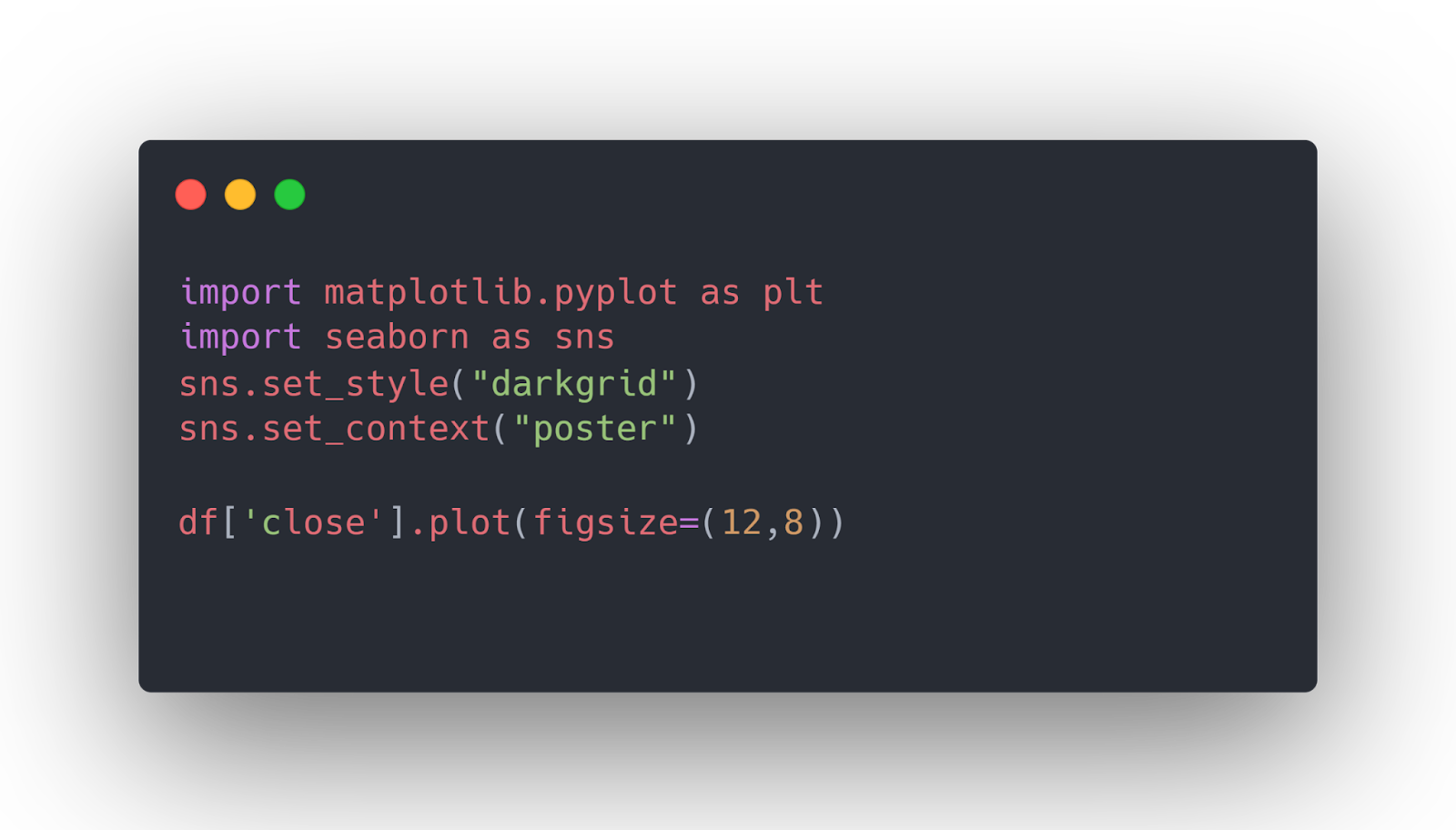
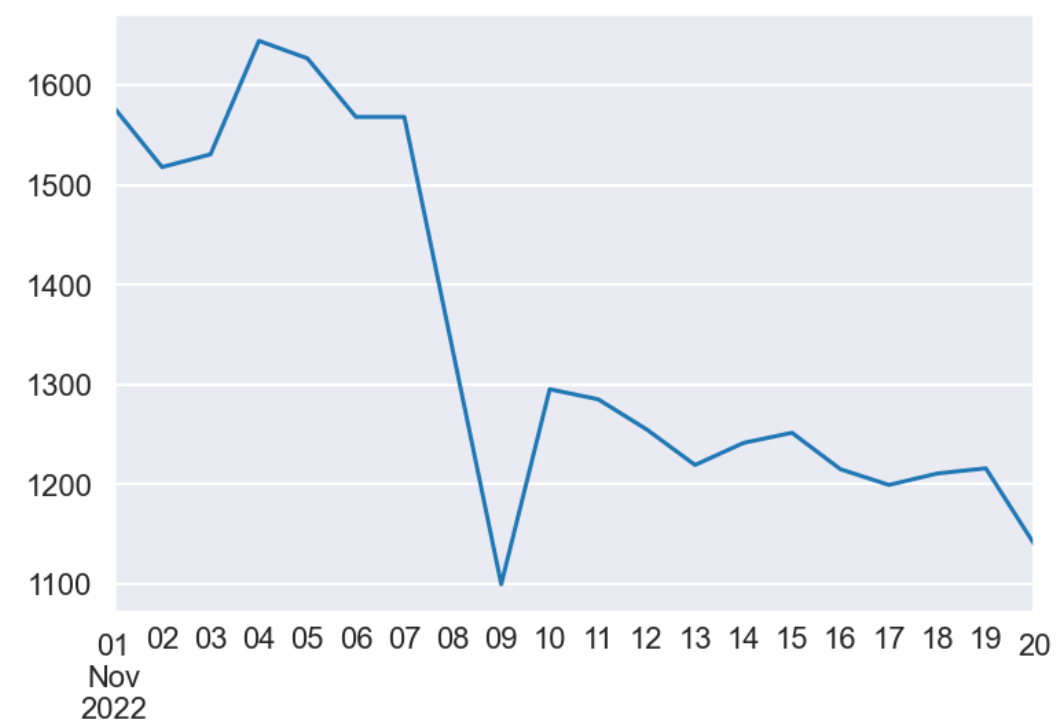
Wrapped Assets Endpoint
A wrapped asset is a digital asset pegged to the value of another original digital asset. The coinbase-pro library doesn’t implement functions for this endpoint.
Get All Coinbase Wrapped Assets
You can use the Python requests library to get all Coinbase wrapped assets.
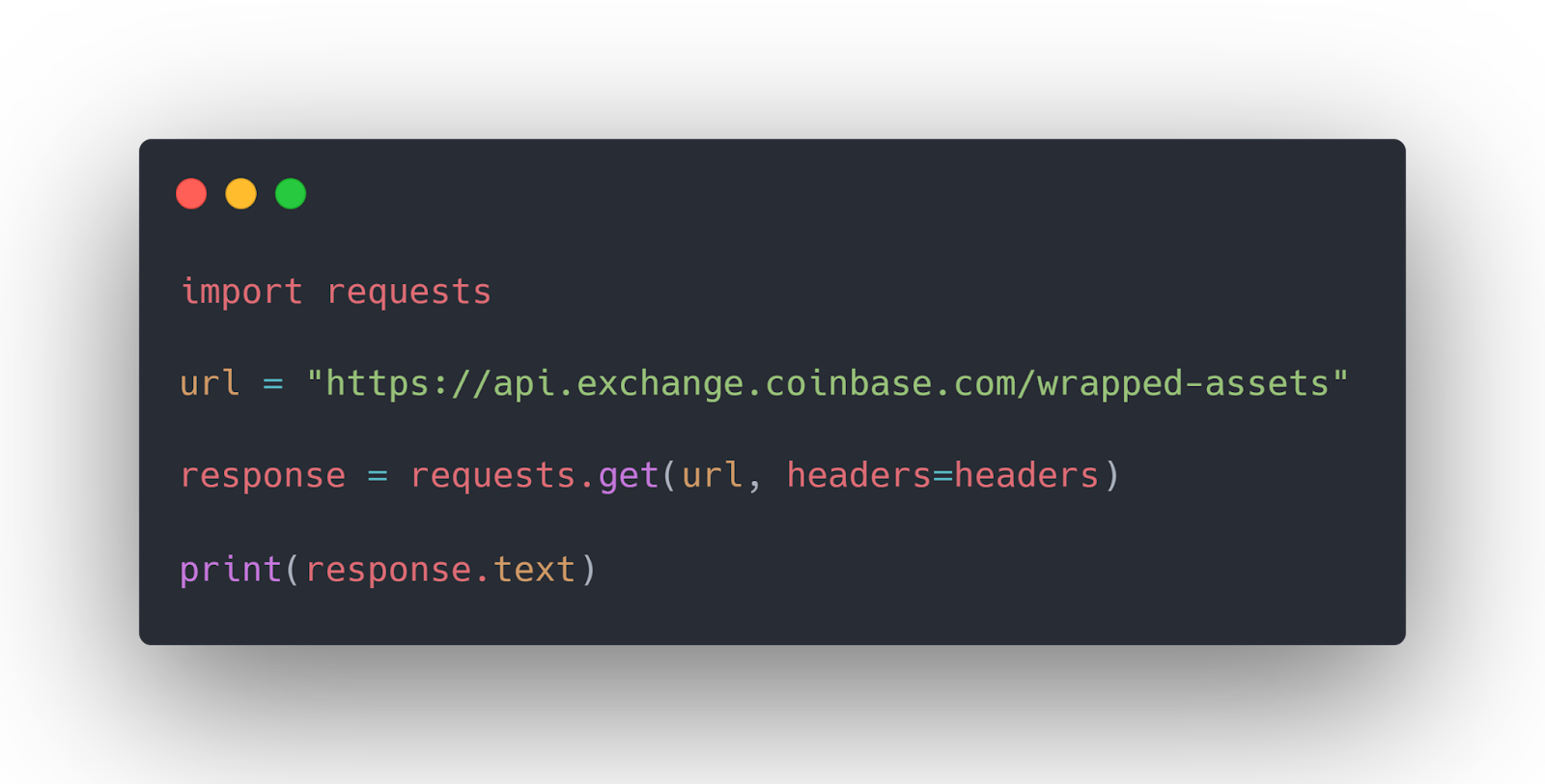
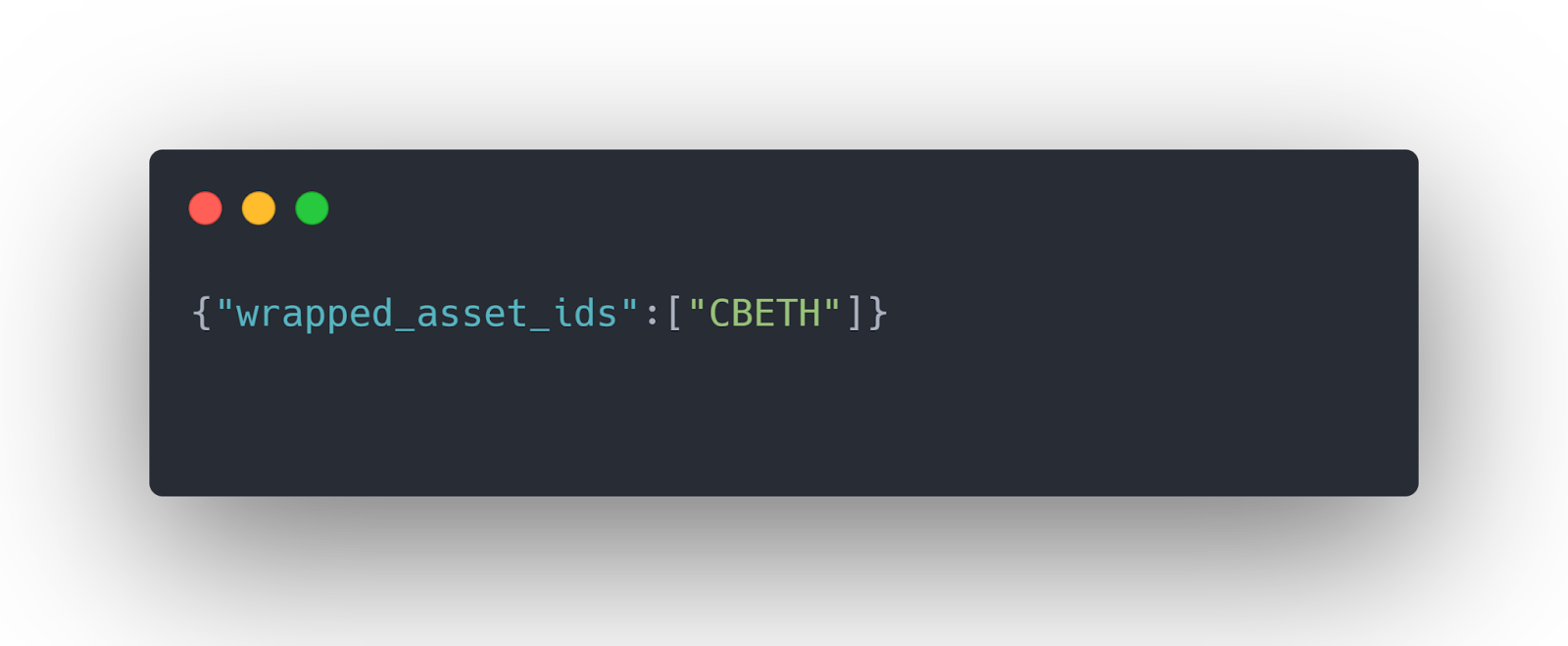
Get Conversion Rates of Wrapped Assets
The requests library function allows you to retrieve the conversion rate of a wrapped Coinbase asset:
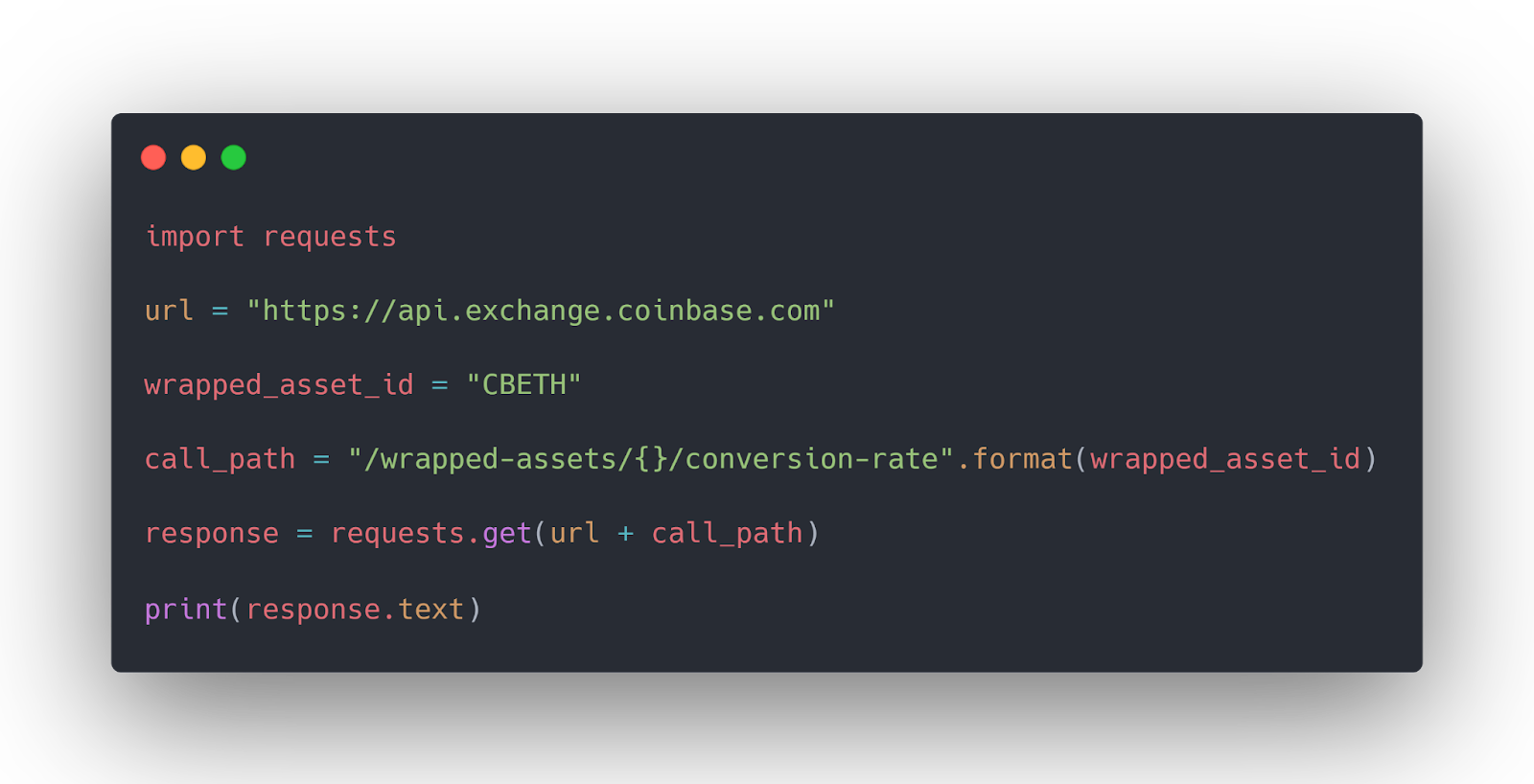
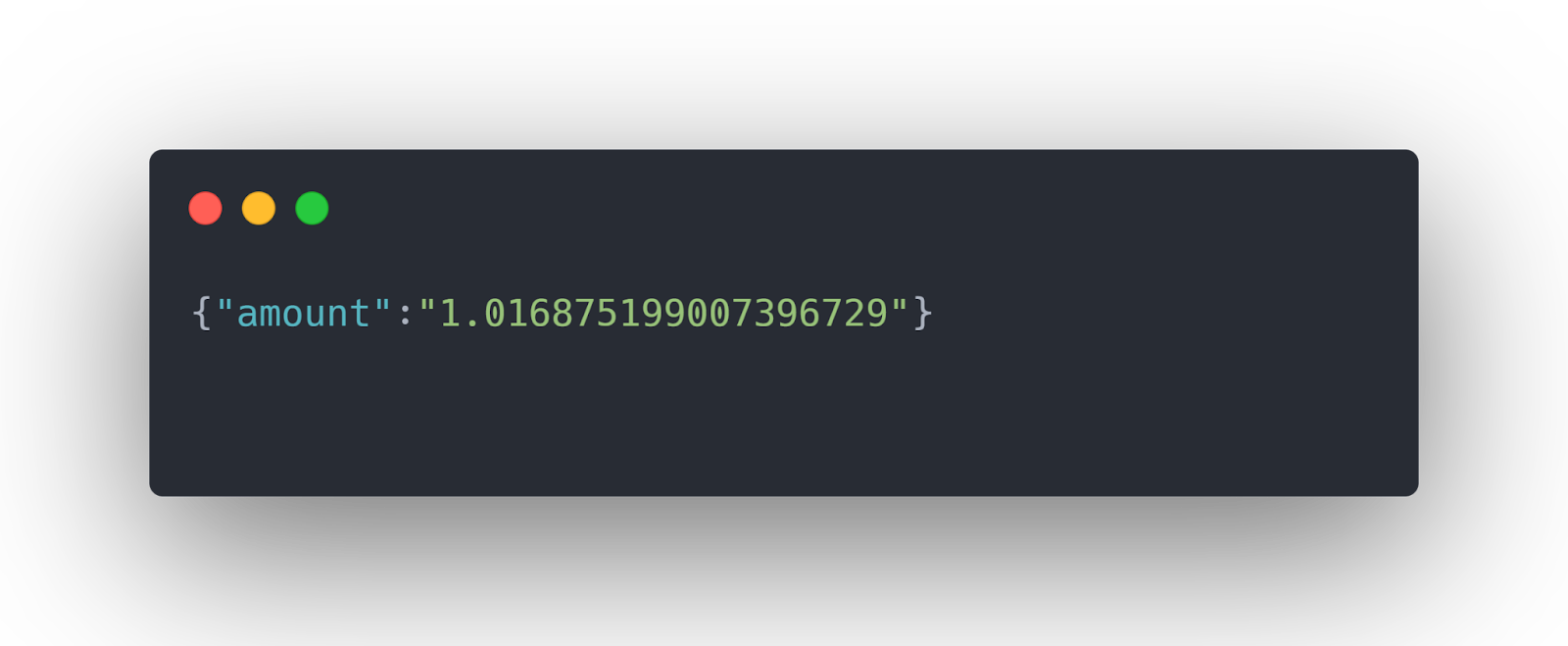
Accounts Endpoint
The accounts endpoint returns account information from the profile of the API key. The profile refers to the user profile to which the API key belongs. You must use an authenticated client to request data from this endpoint.
Get All Accounts for a Profile
The get_accouts() method of the AuthenticatedClient returns basic account details from the profile of the API key.
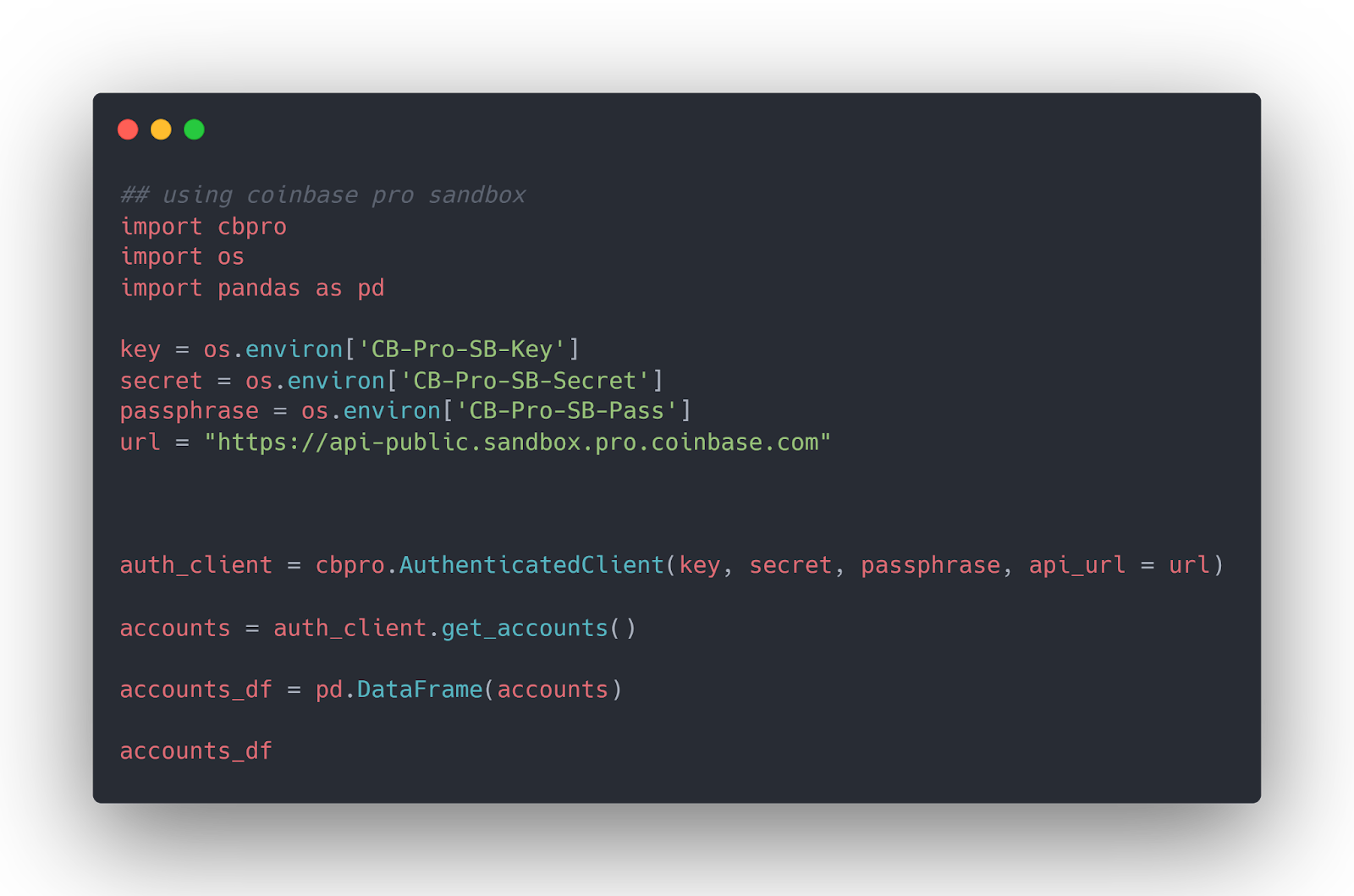
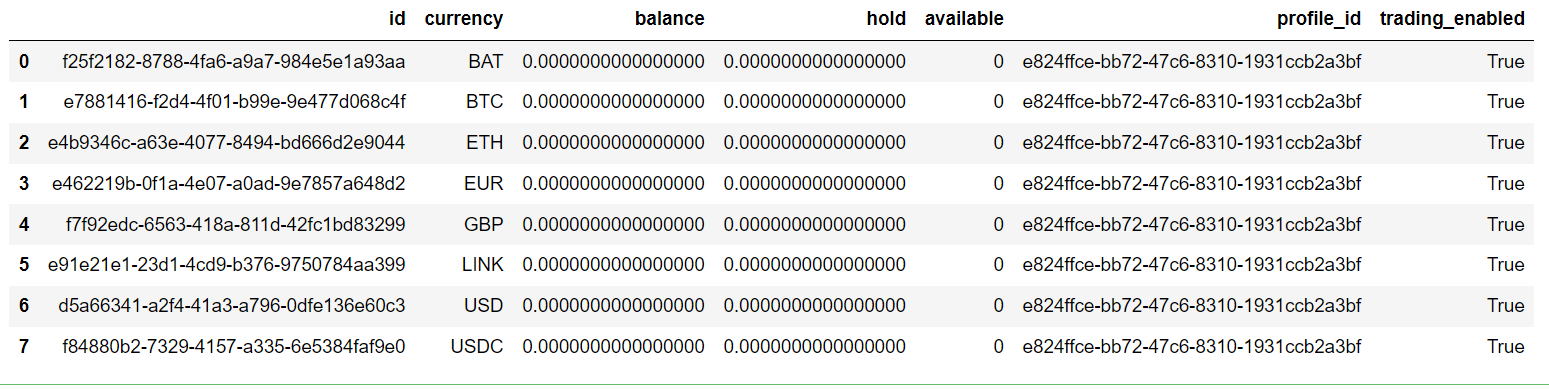
Get Coinbase Single Account Holdings
The get_account_holds() method returns a list of all holds of a an account belonging to the same profile as the API key.
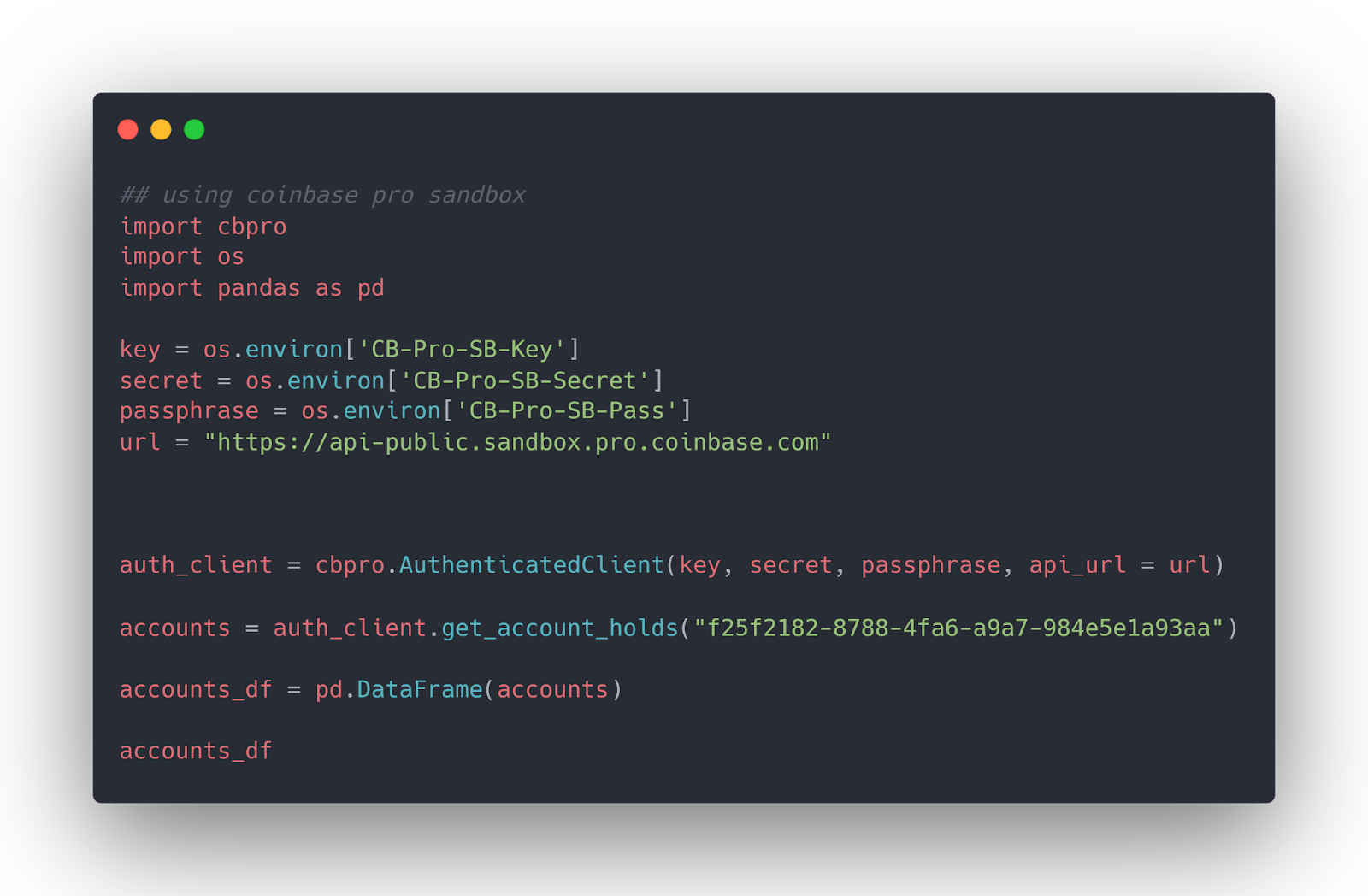
Address Book Endpoint
The address book endpoint contains only a single function that allows you to retrieve all your addresses in your account address book. Address book addresses allow you to send and receive digital assets safely.
Get All Address Book Addresses
The coinbase-pro library doesn’t implement a function for this endpoint. As the following script demonstrates, you can rely on the requests library to call the address book endpoint.
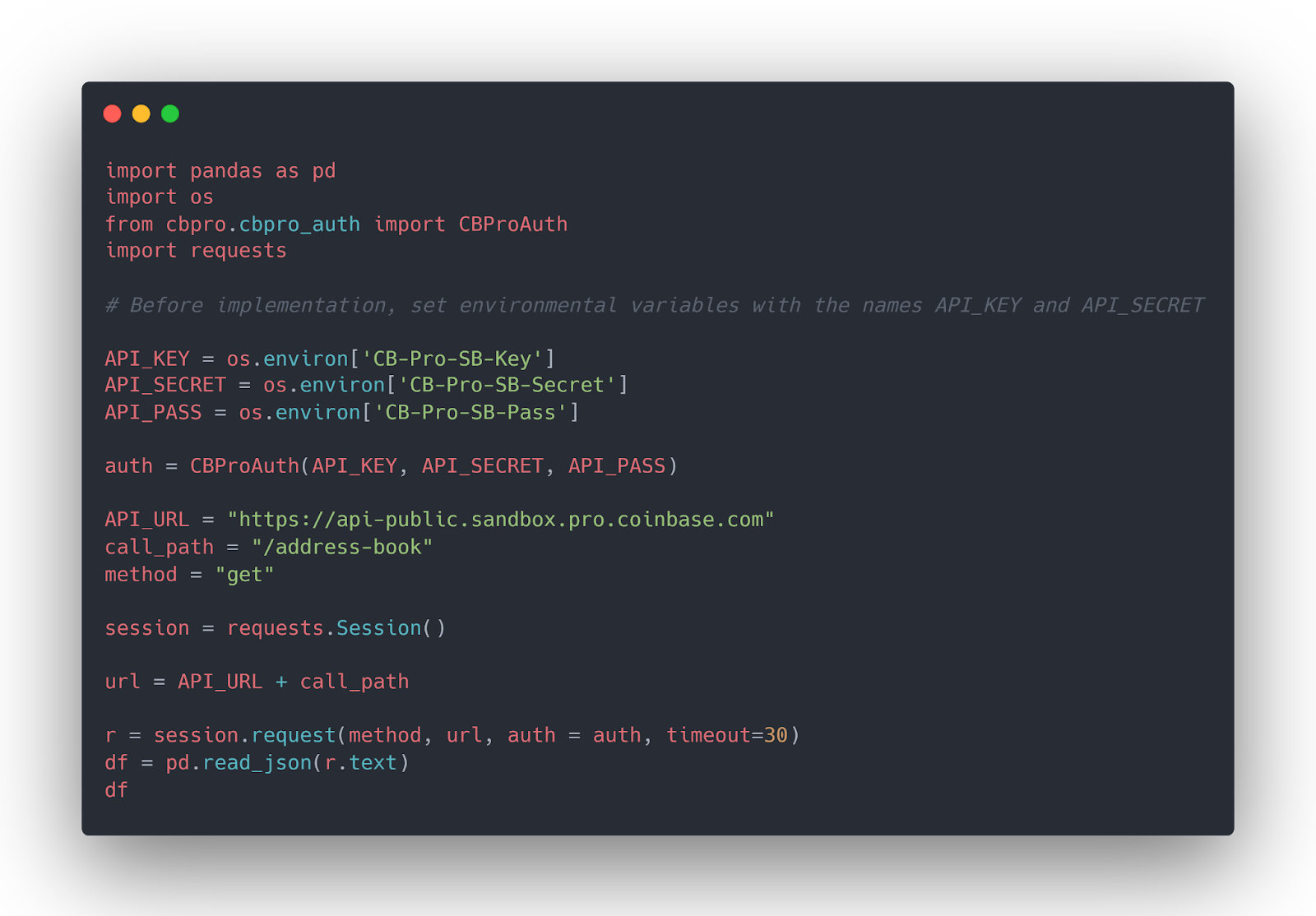
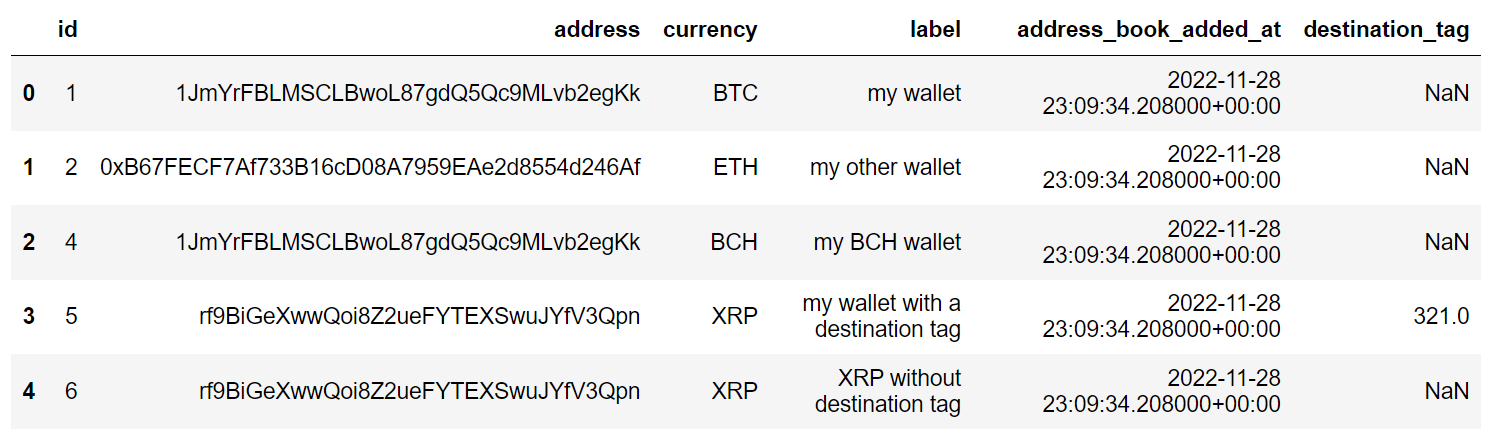
Coinbase Accounts Endpoint
Get All Coinbase Wallets
The get_coinbase_accounts() method returns a list of all your Coinbase wallets that you can use for buying and selling on www.coinbase.com.
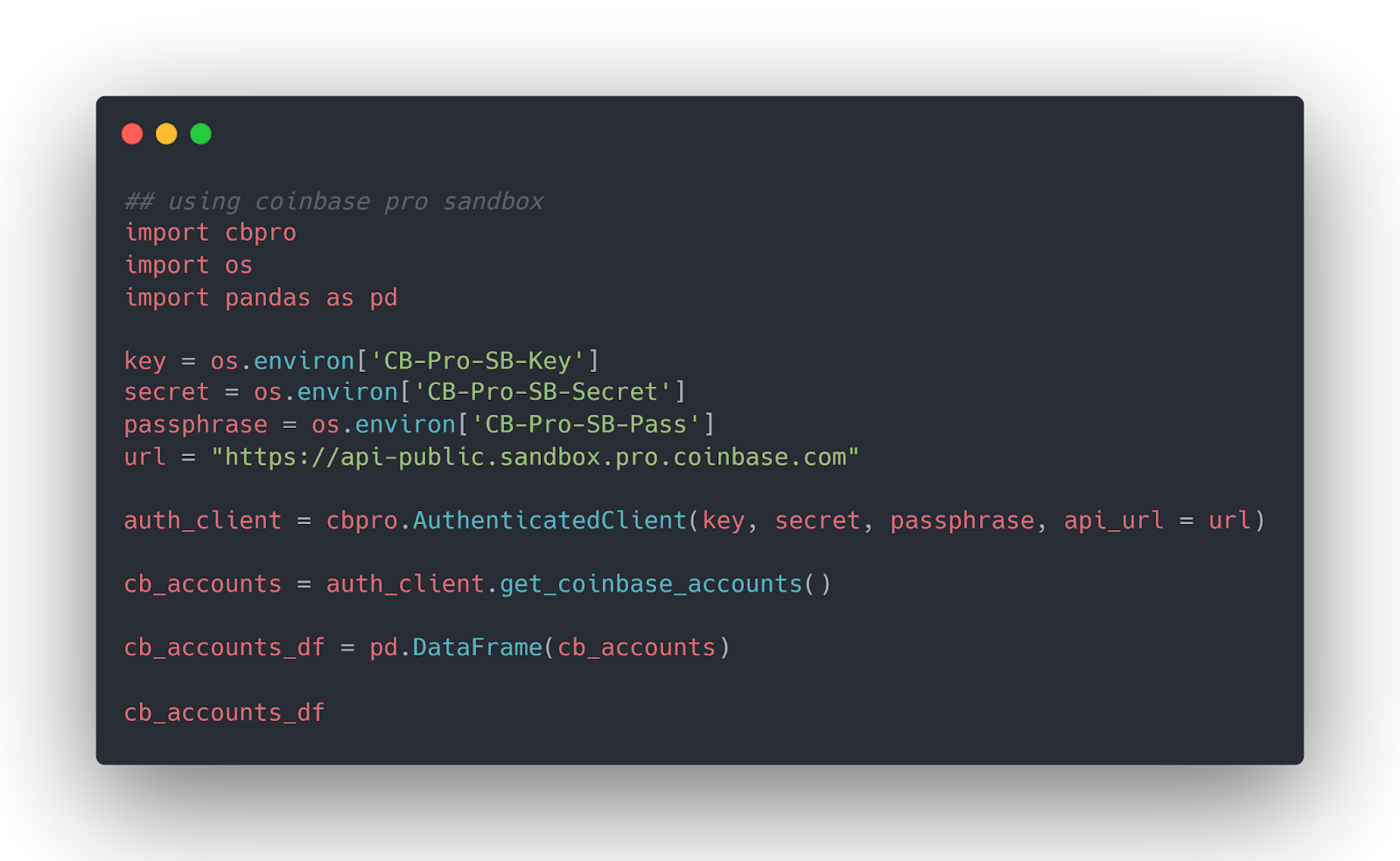
You can see a list of wallet ids, names, balances, currency types, etc. in the output:
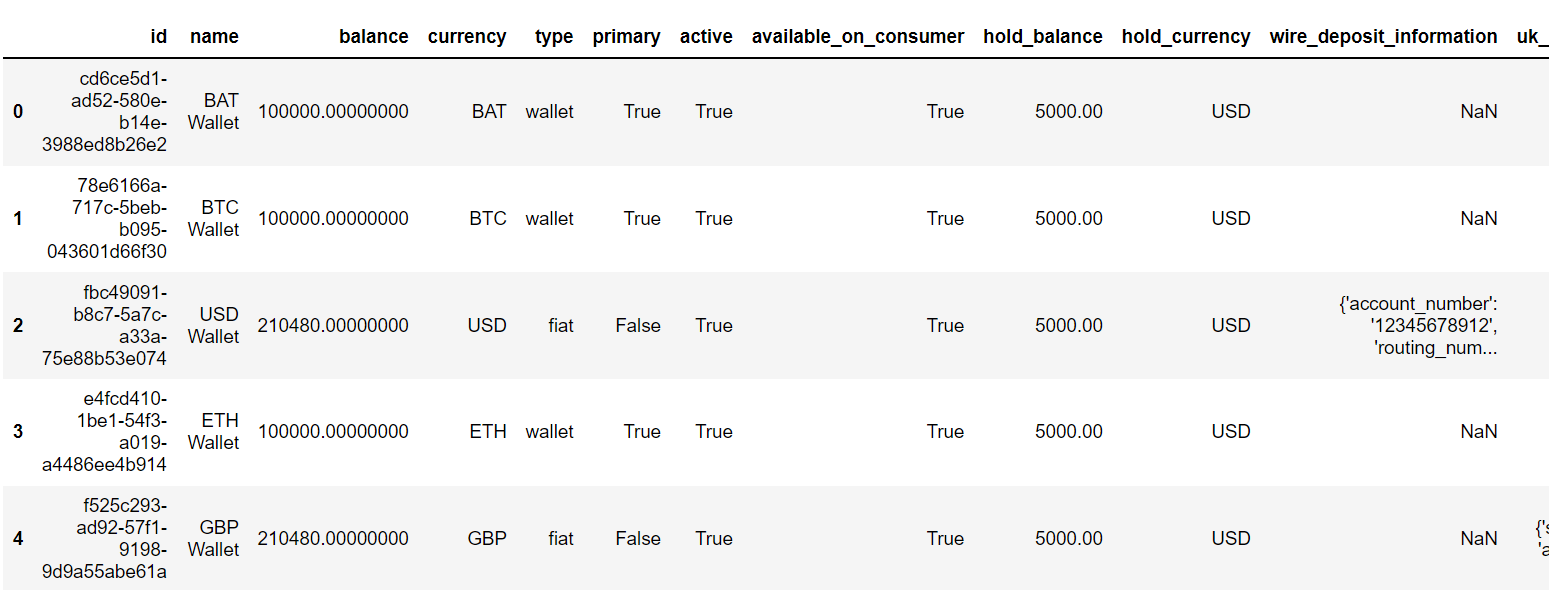
Generate Crypto Addresses
The coinbase-pro library doesn’t implement a function for this endpoint.
Using the requests library, you can generate a one-time crypto address for depositing your cryptocurrencies. You must pass the Coinbase wallet id to the API call for generating a crypto address.
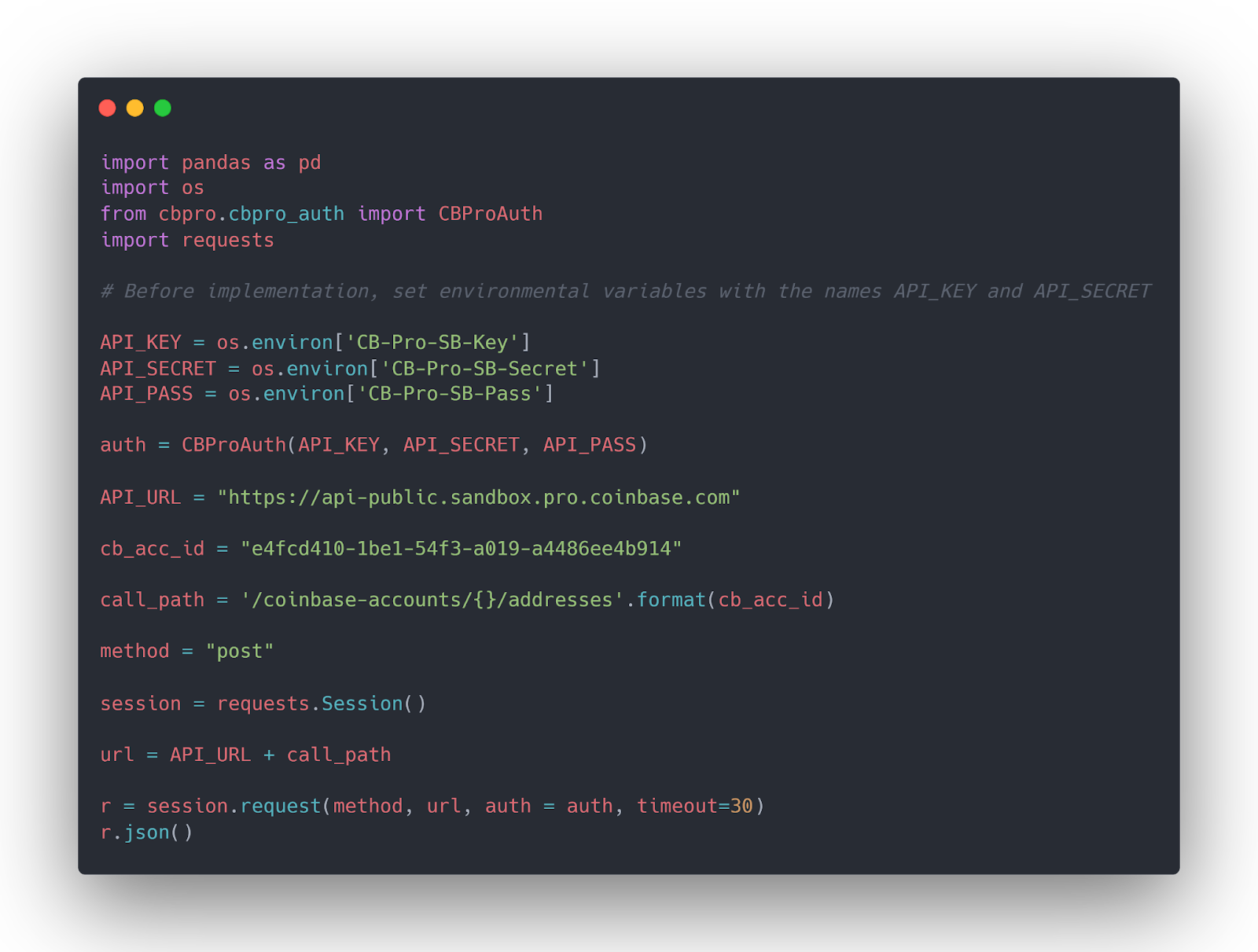
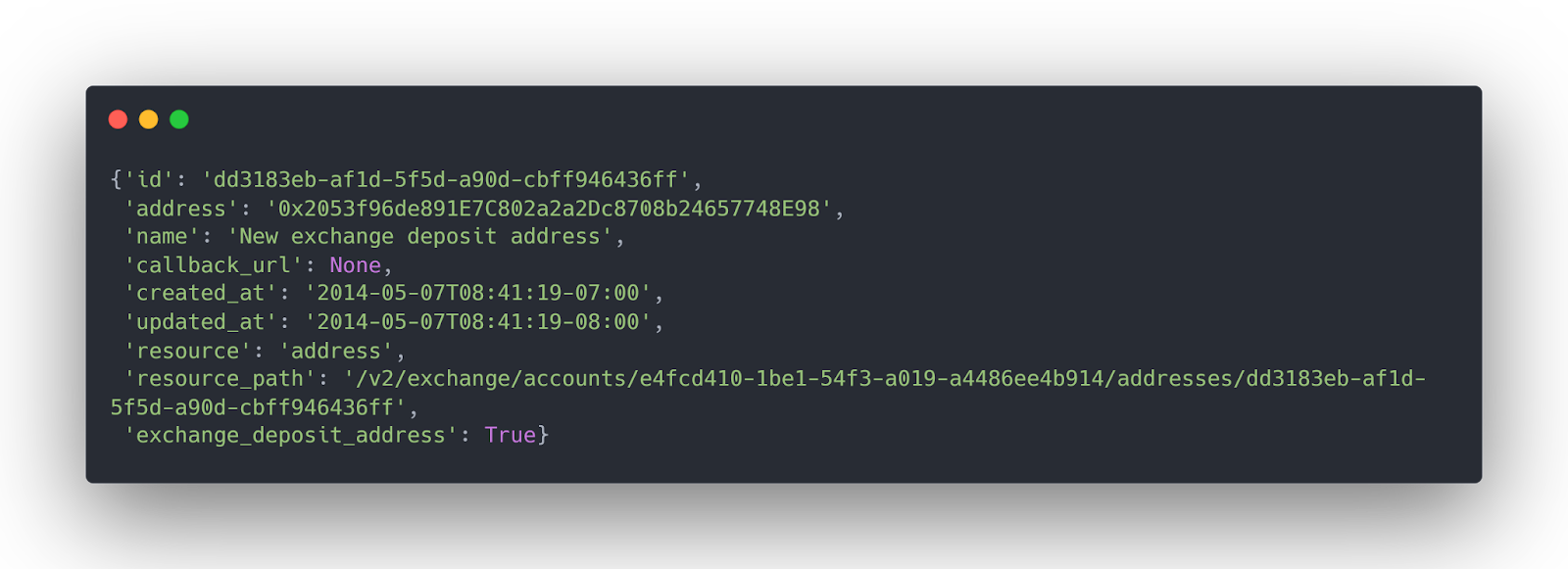
Fees Endpoint
The fees endpoint allows you to get maker and taker fees applicable to your transactions.
Get Coinbase Fees
Since the coinbase-pro library doesn’t implement a function for this endpoint, you must rely on the requests library to get all Coinbase Pro fees.
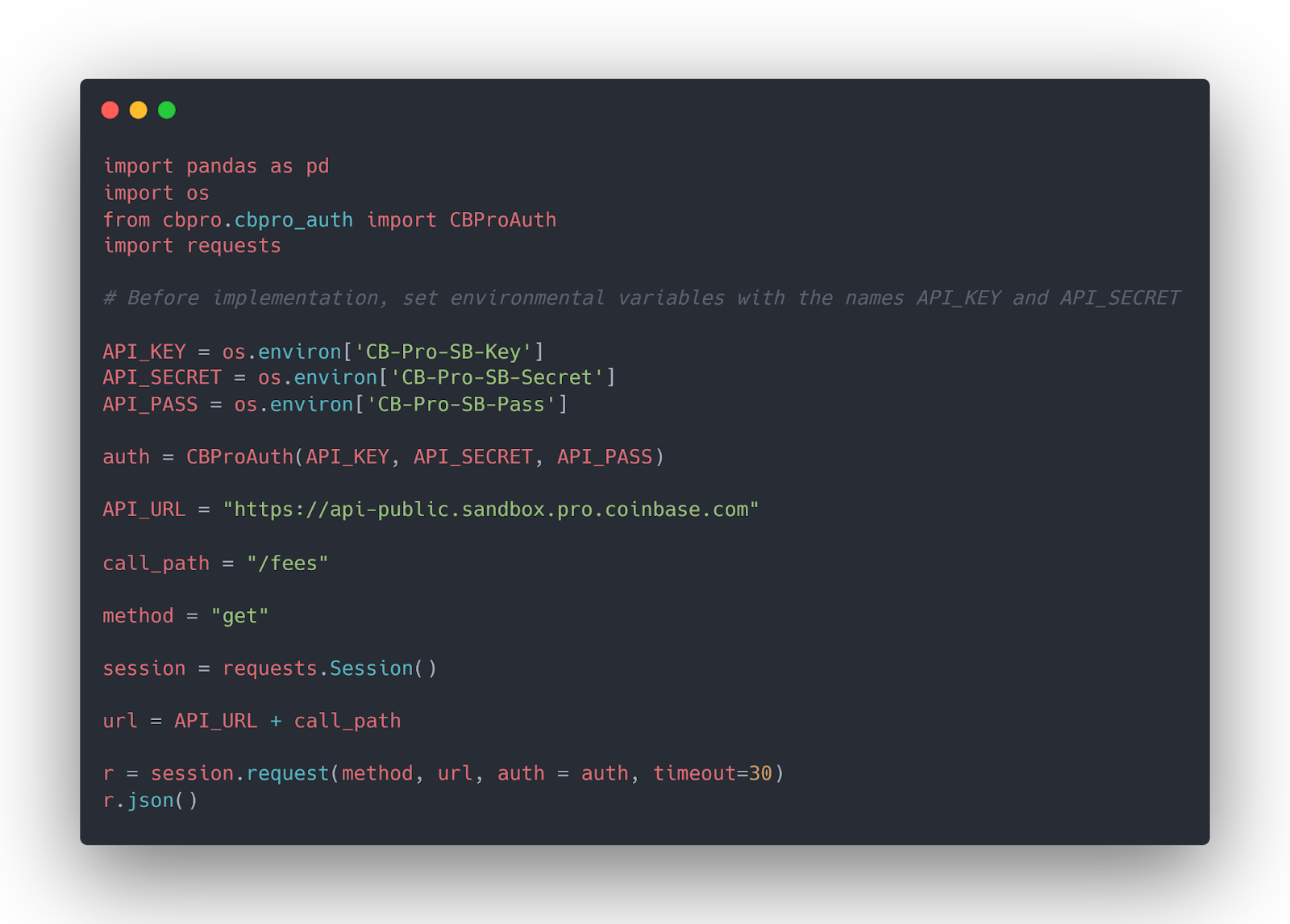
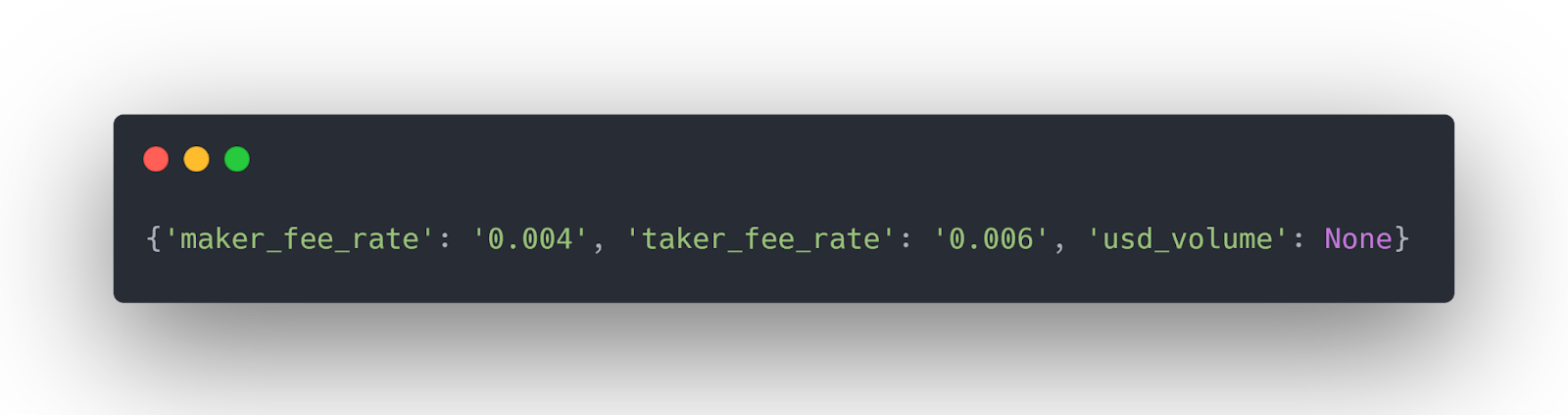
Coinbase Price Oracle Endpoint
The Coinbase price oracle API endpoint contains a function that returns signed price data for various cryptocurrencies. Anyone can retrieve the signed price and publish them on-chain.
Get Coinbase Signed Prices
You can retrieve Coinbase signed prices using the Python requests library.
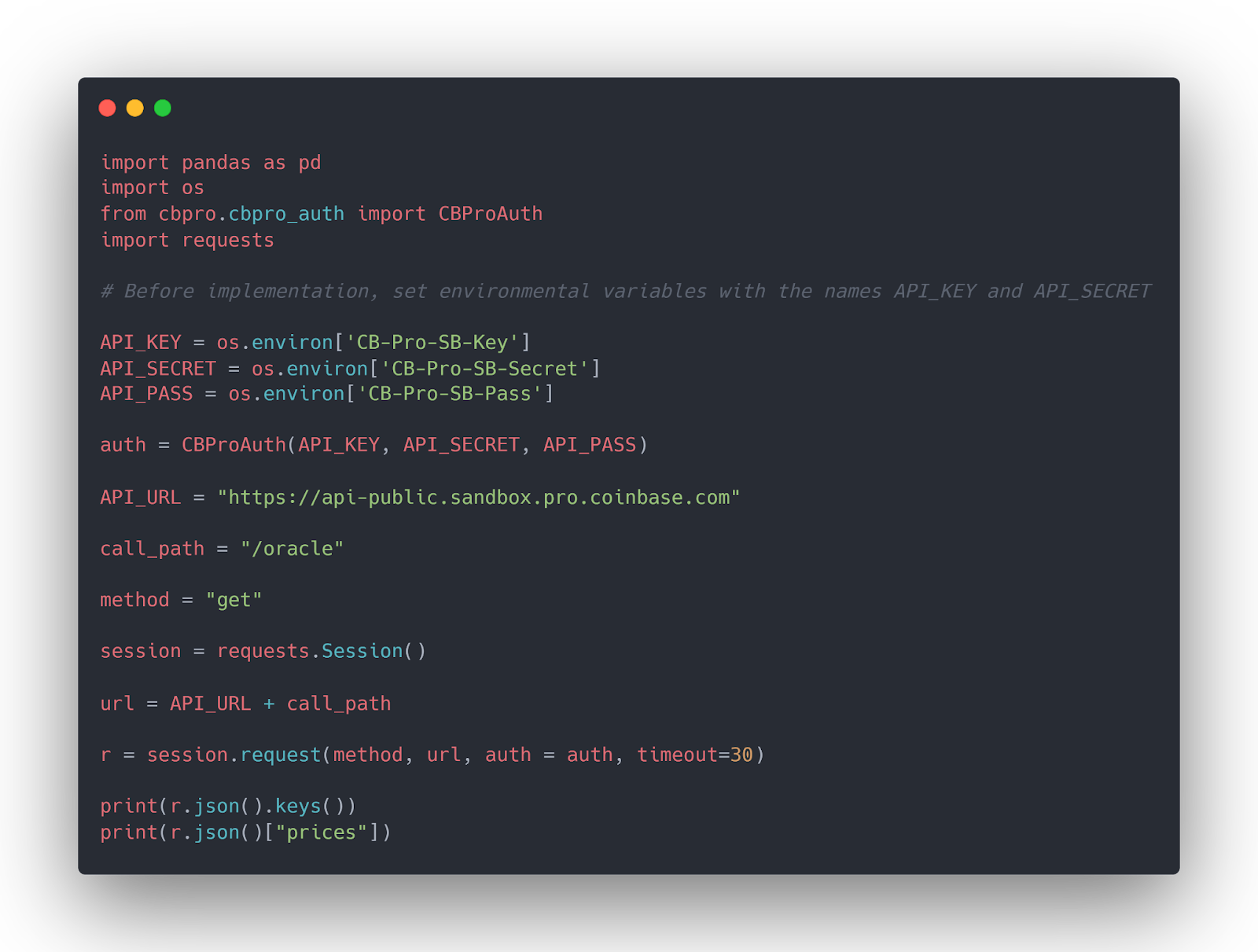
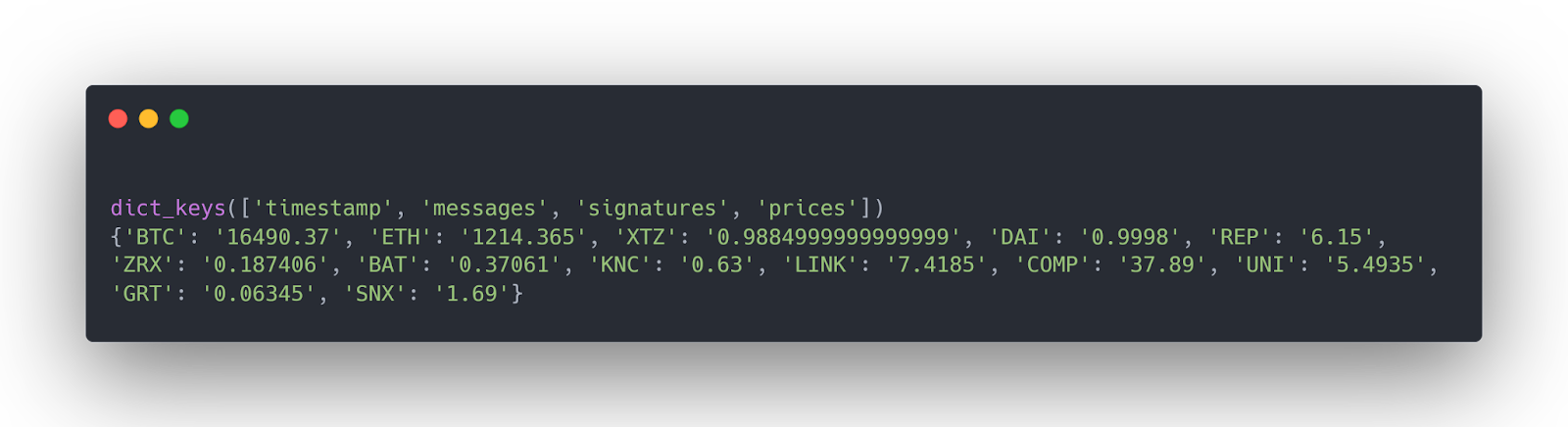
Users
The users endpoint contains one function that returns information on the user’s buy/sell limits per currency and payment method transfer limits.
Get User’s Exchange Limit Information
The coinbase-pro library doesn’t implement a function for this endpoint. You can use the Python requests library instead.
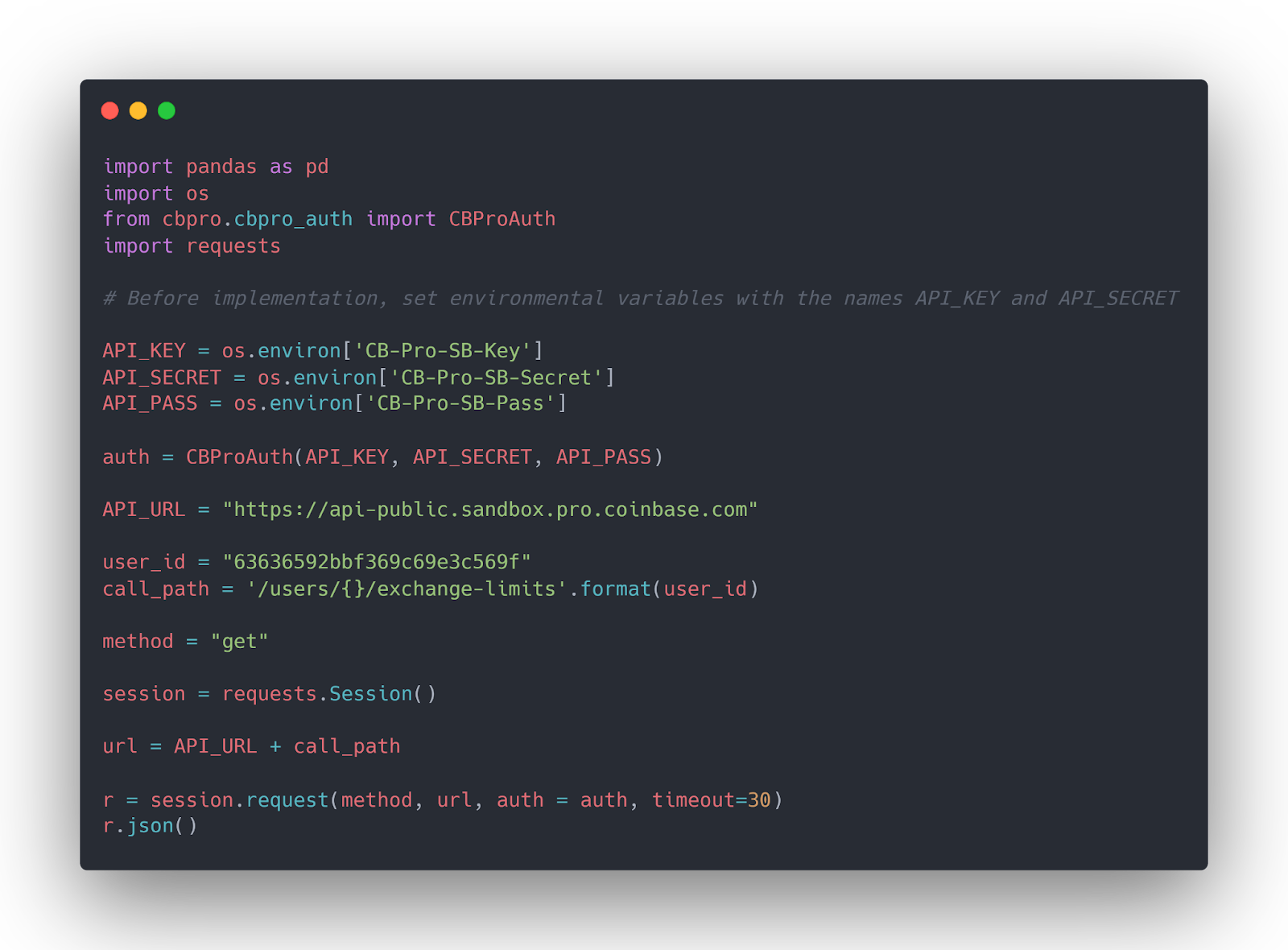
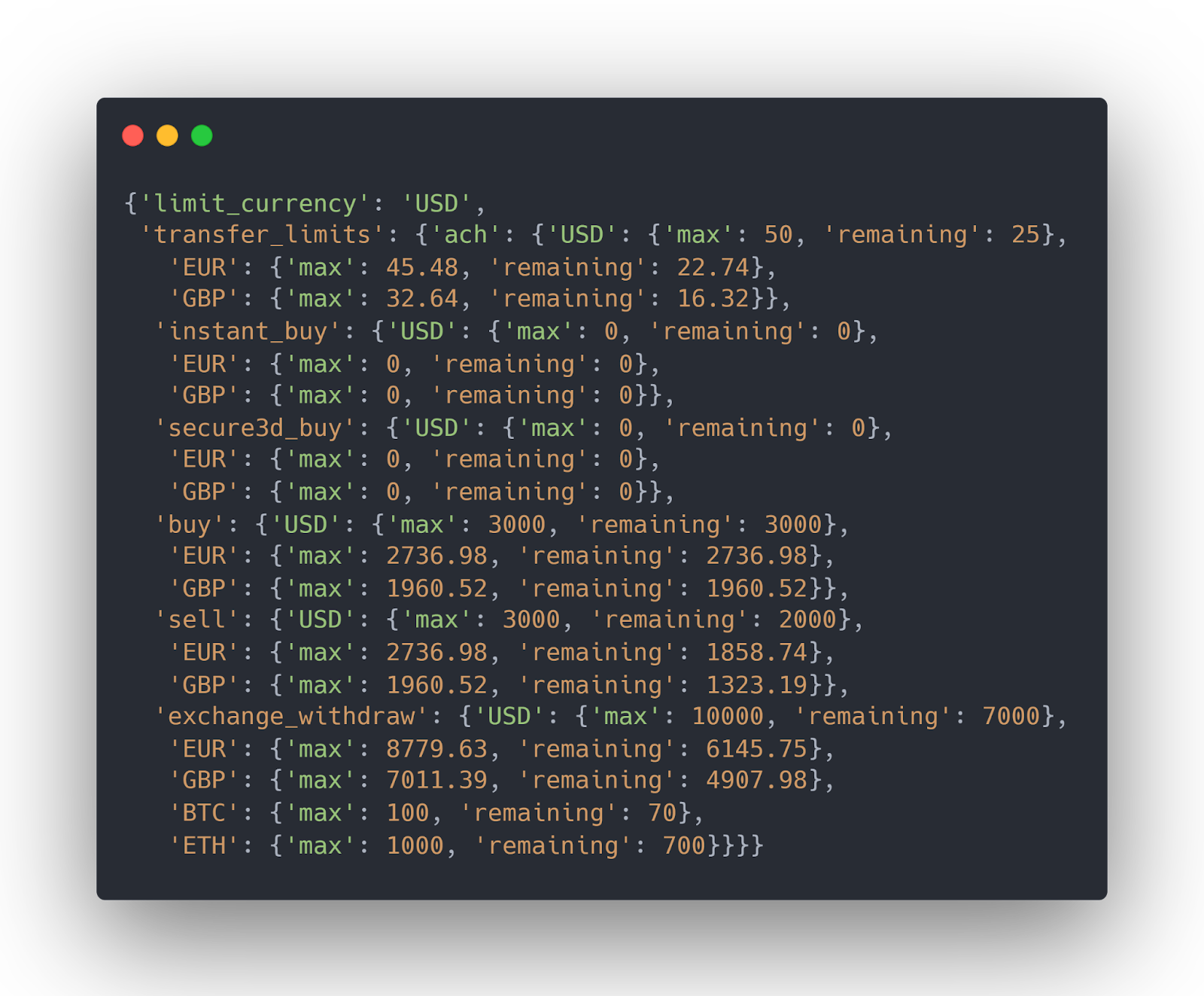
Reports Endpoint
Reports endpoint allows you to generate different reports for your Coinbase digital assets. You can see all your digital transactions and other information for a digital asset.
Create a Report
The create_report() method from the AuthenticatedClient class allows you to create reports. You must pass to the method, the report type, start and end dates, and the product id of the digital asset for which you want to generate the report. You can optionally pass an email address where you want to receive the report.
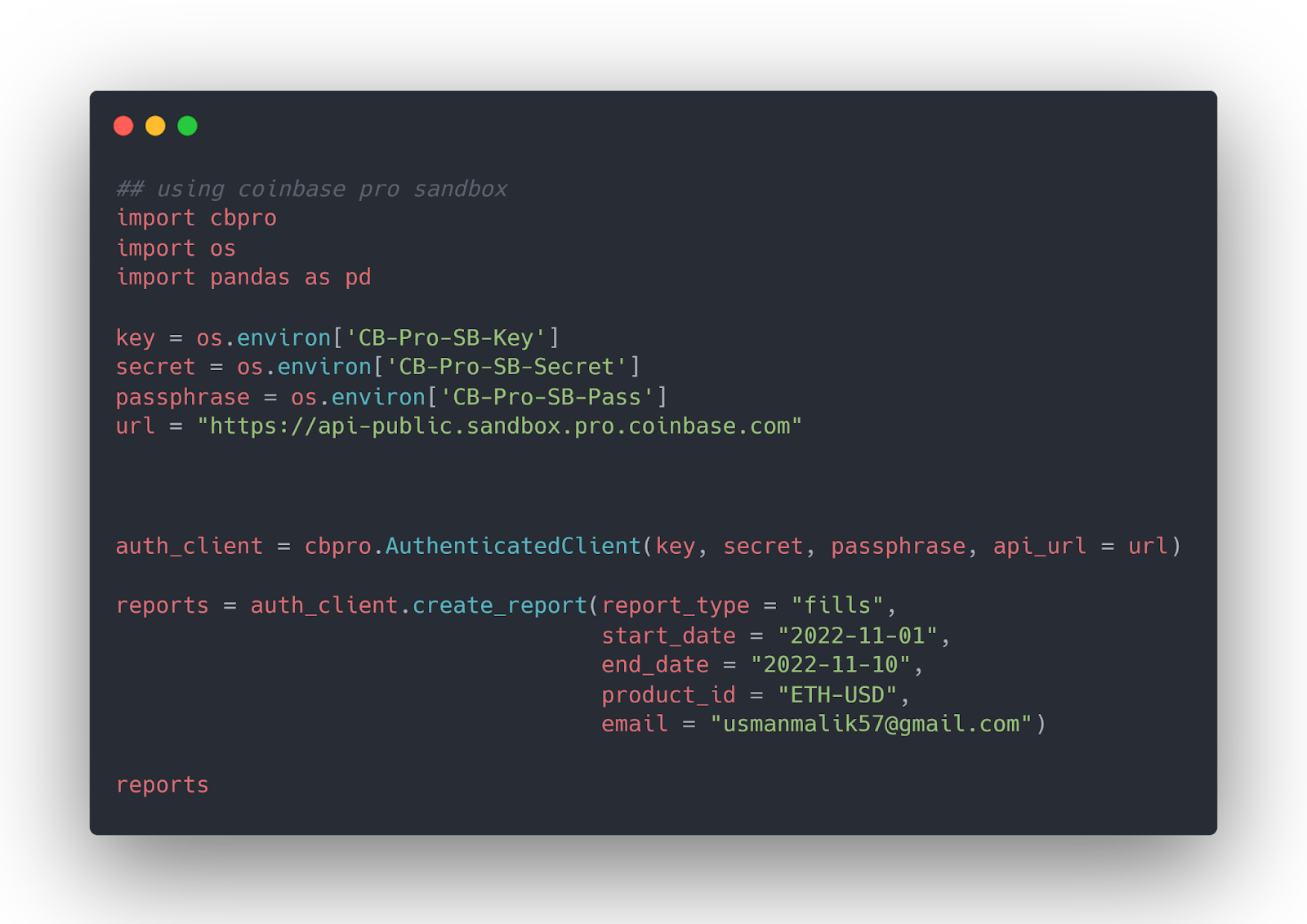
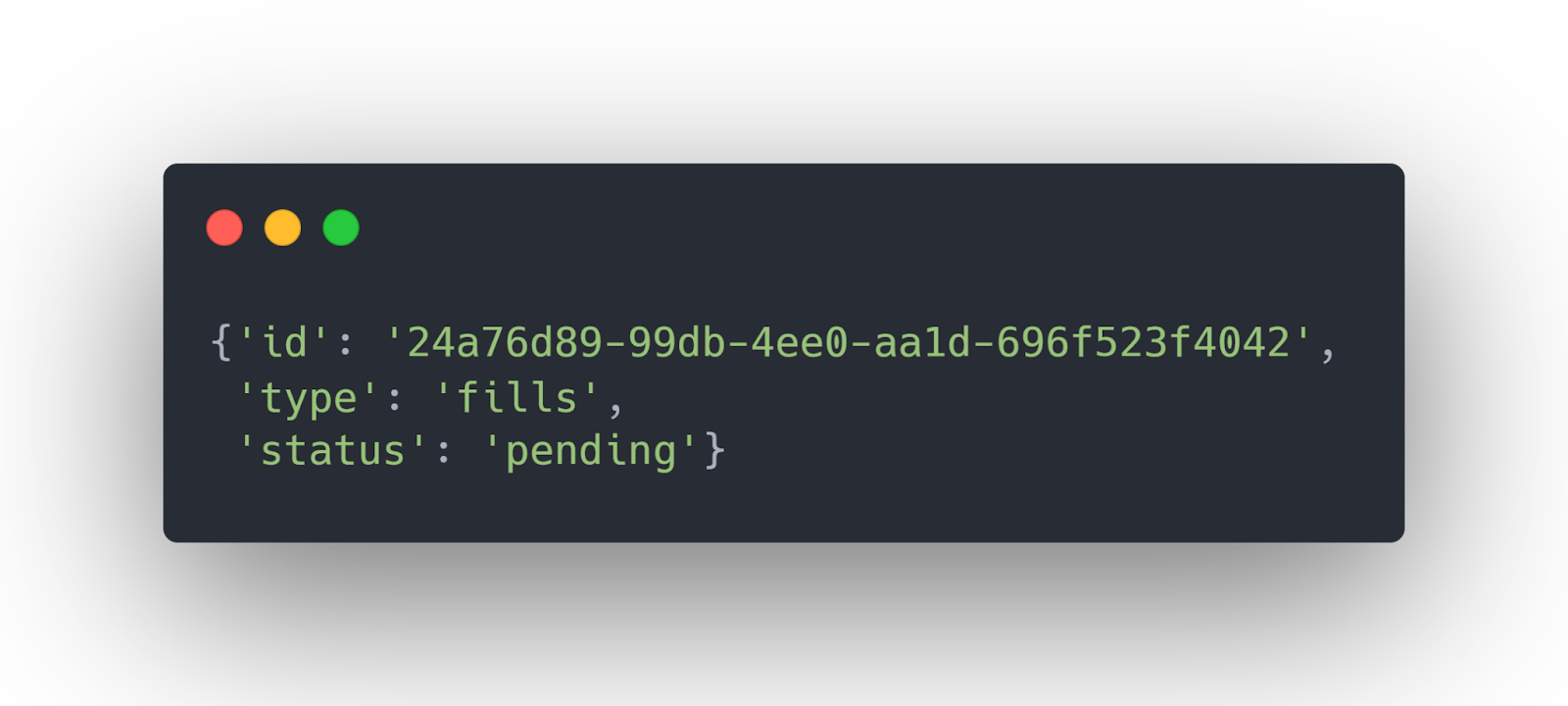
The report looks like that in the email. Since I did not have any trade activity for “ETH-USD” between the given dates, the report is empty.
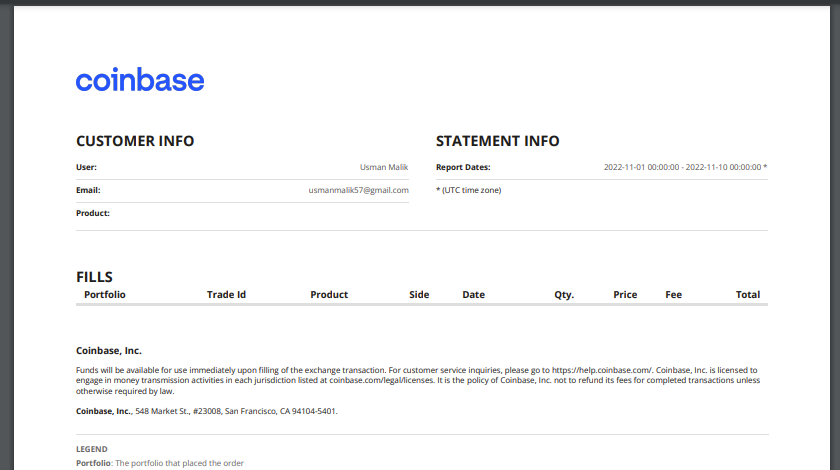
Get a Report
The create_report() method returns the id of the report, which you can use to get the report details using the get_report() method.
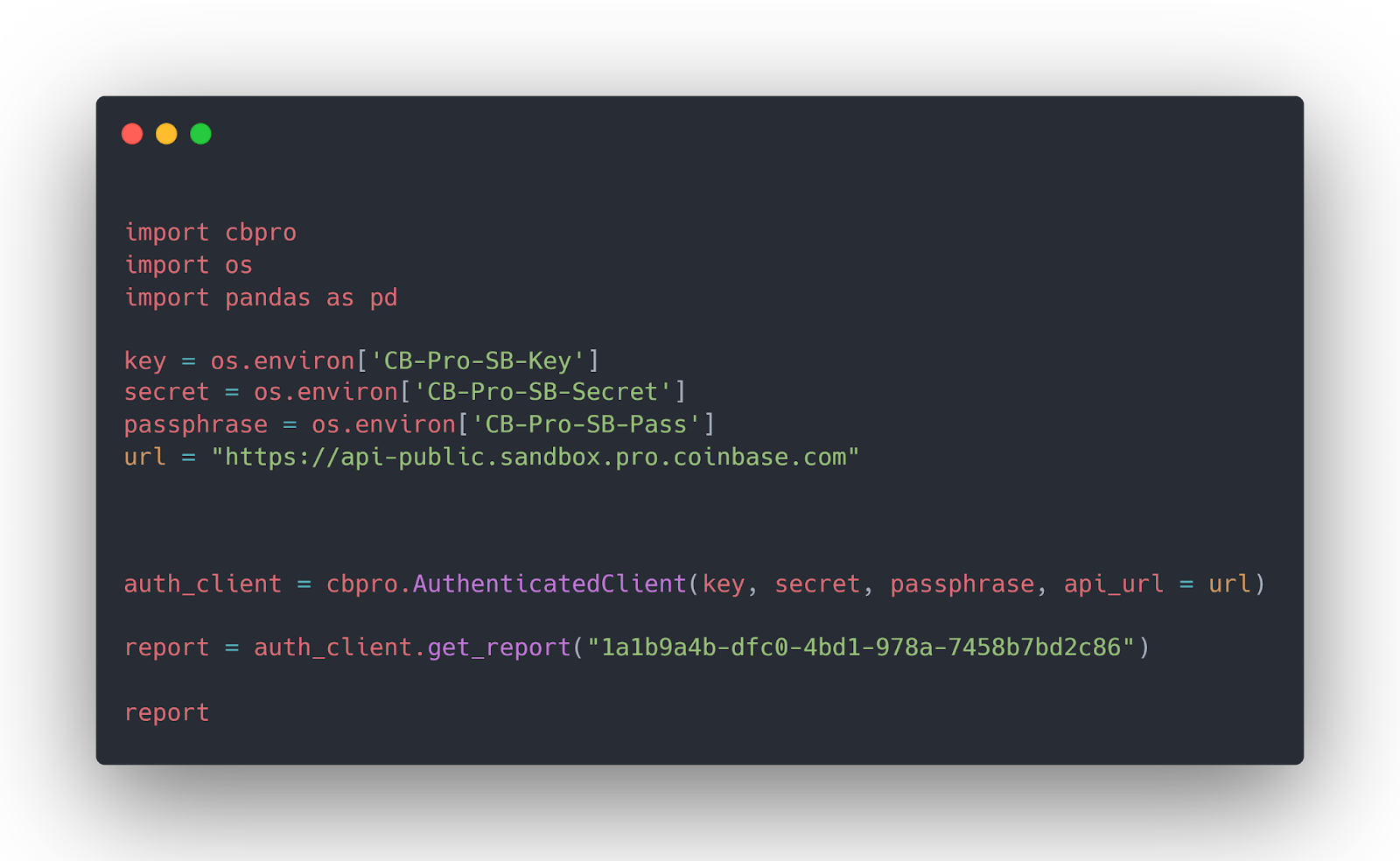
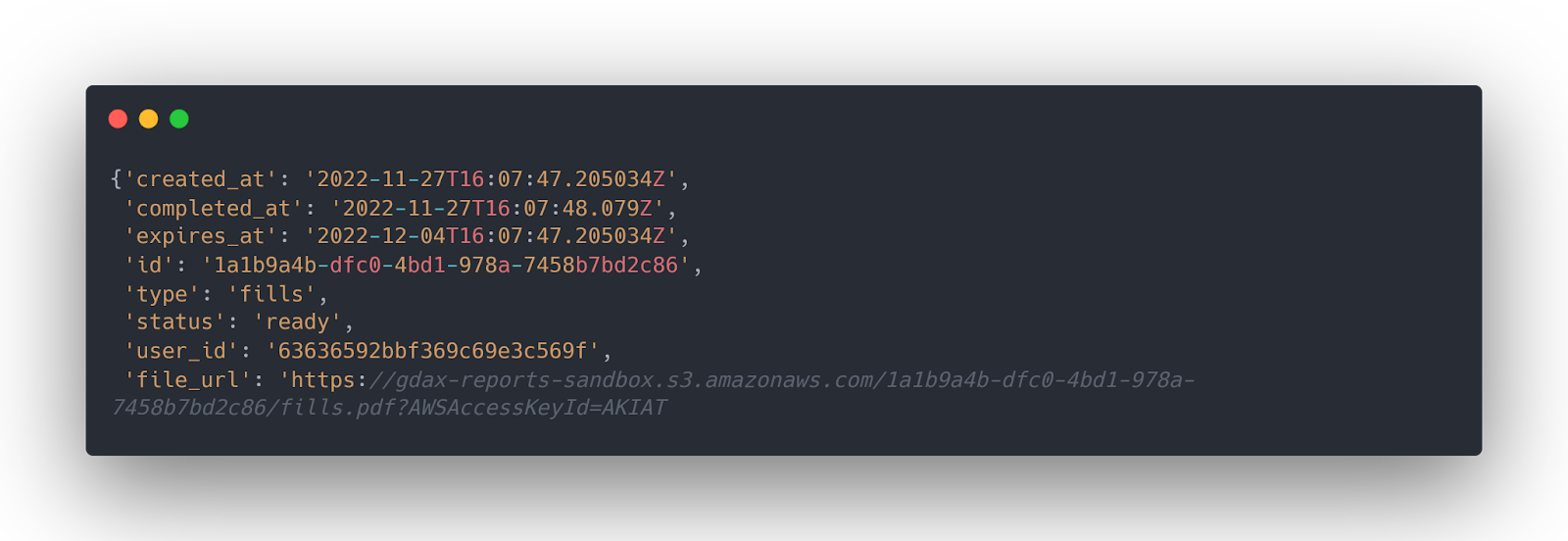
Get All Coinbase Reports
If you want to retrieve all Coinbase reports, you will have to rely on the requests library since the coinbase-pro library doesn’t implement a function for this.
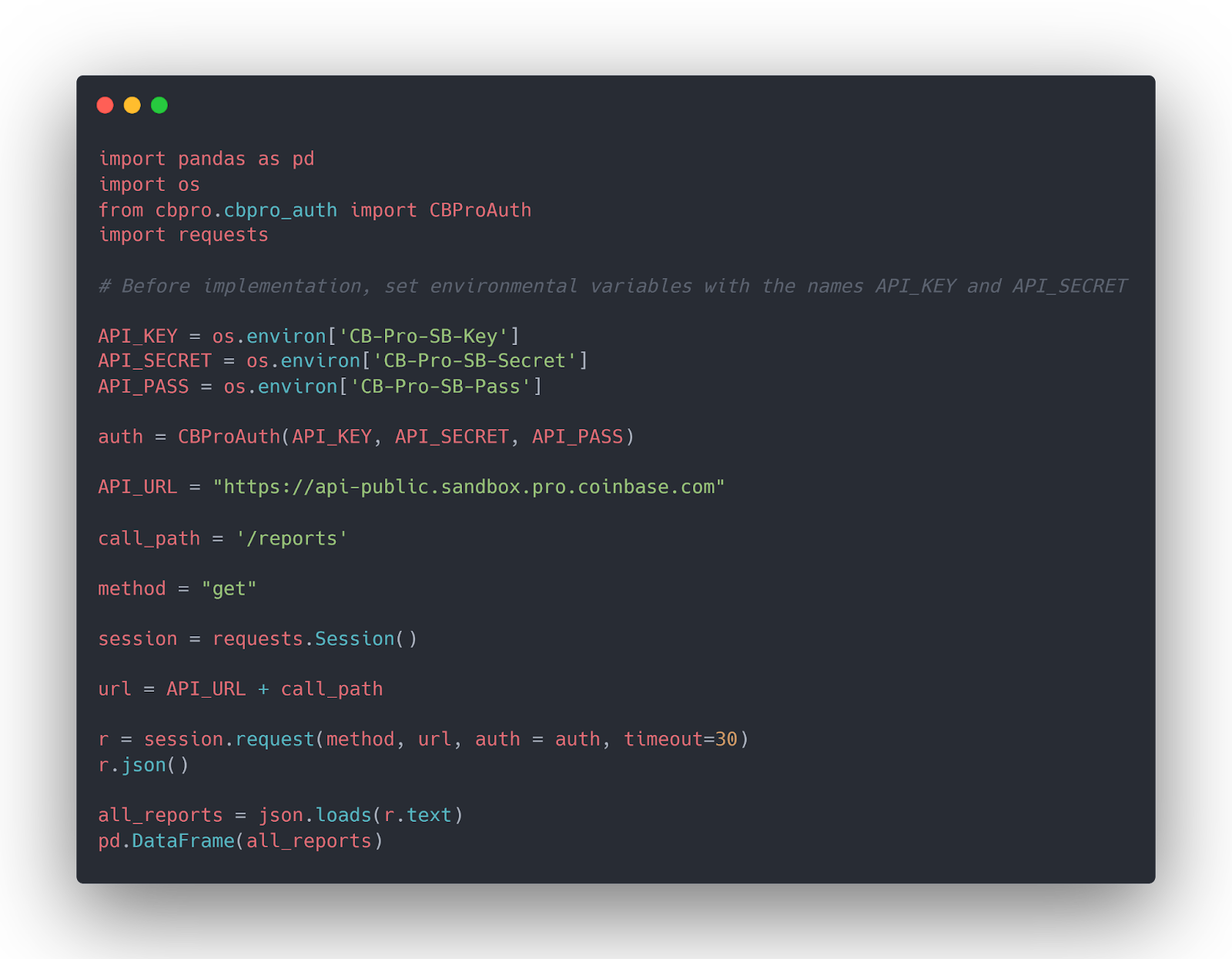
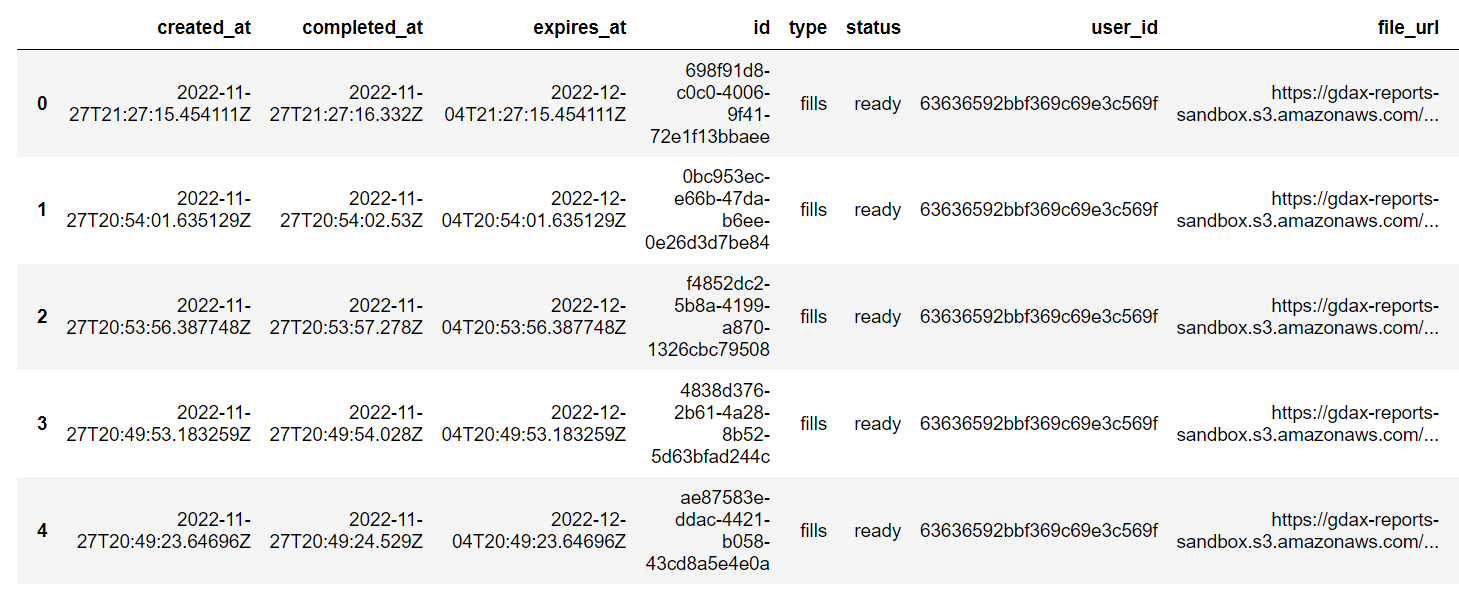
Conversions Endpoint
The conversion endpoint functions allow you to convert currencies in your account.
Convert Currency using Coinbase
You can use the requests library to convert currencies in your Coinbase account. You must pass the profile id of your account, source and destination currency, and the amount of currency.
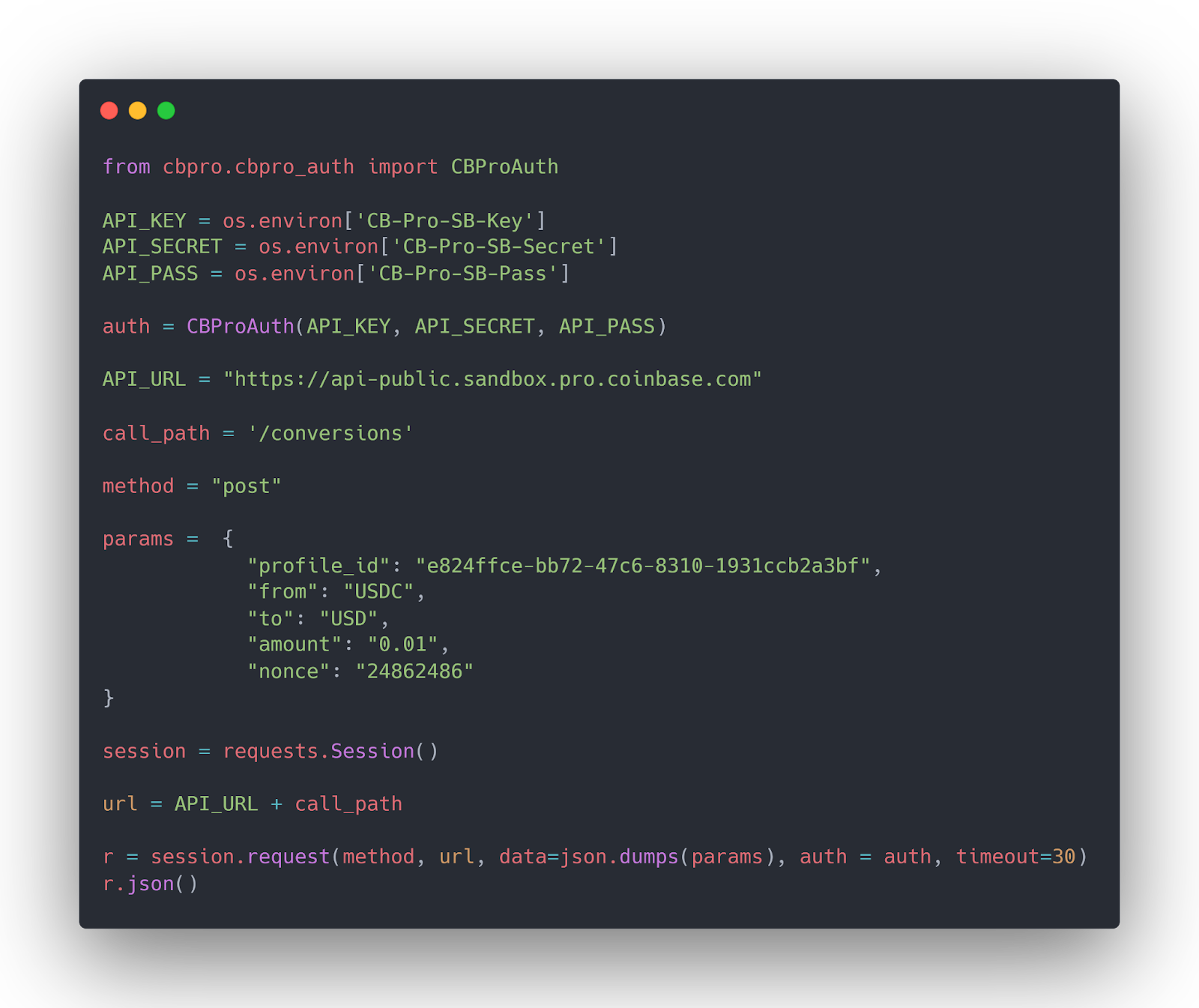
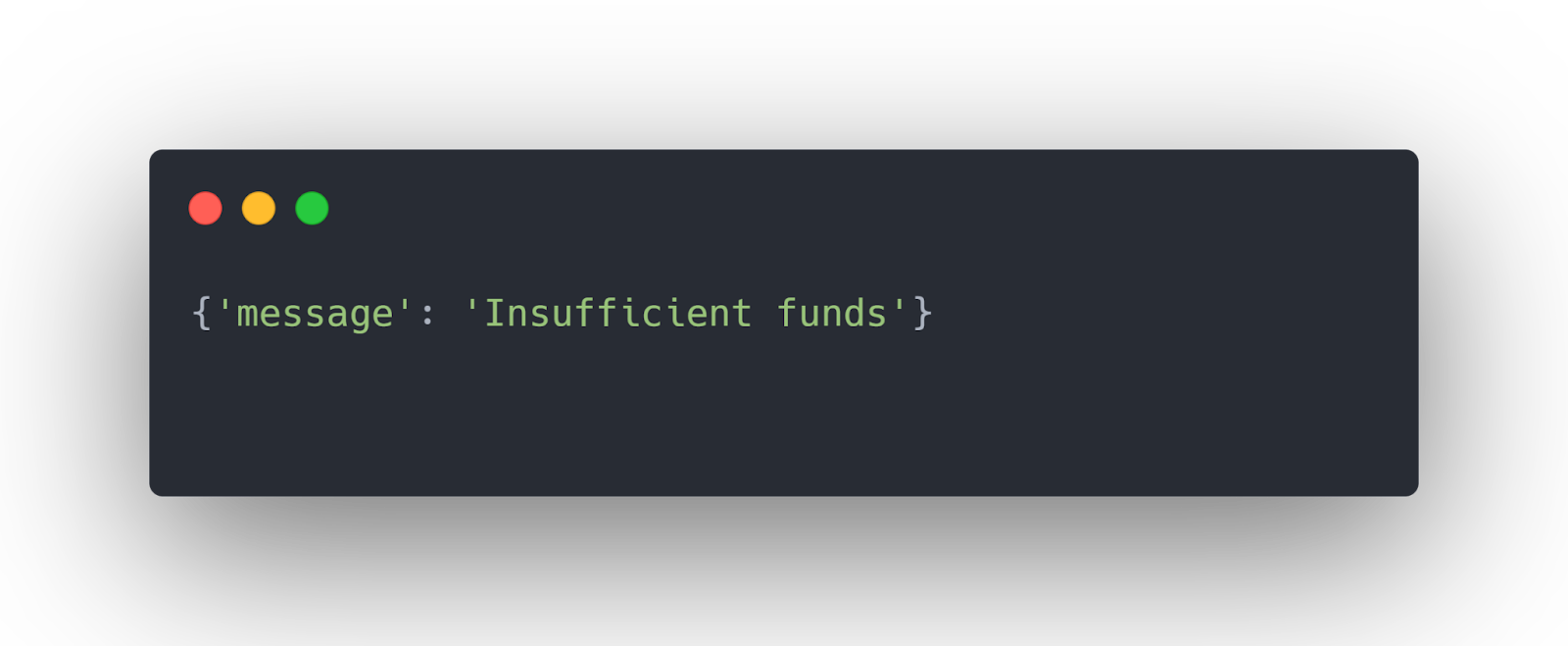
Get a Conversion
The requests library’s get() function allows you to get a conversion using its id:
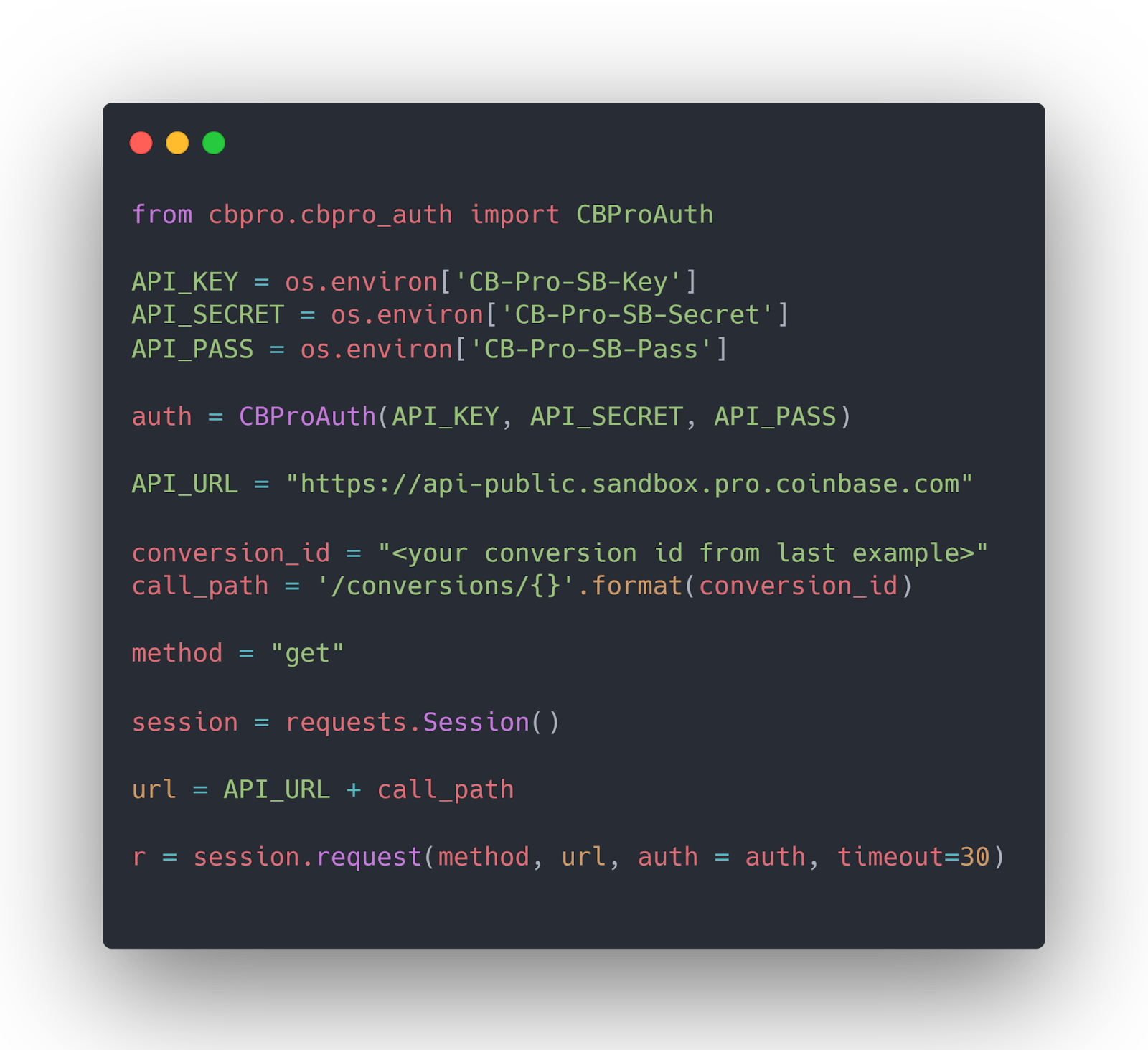
Transfers Endpoint
Transfers endpoint allows you to deposit and withdraw funds from your Coinbase wallet and payment methods, e.g., a bank account, into your Coinbase profile and vice-versa.
Deposit Funds from Coinbase Account
The coinbase_deposit() method from the AuthenticatedClient class deposits funds from one of your Coinbase wallets to your Coinbase account profile. You must pass the amount, the type of currency, and the profile id to which you want to deposit funds.
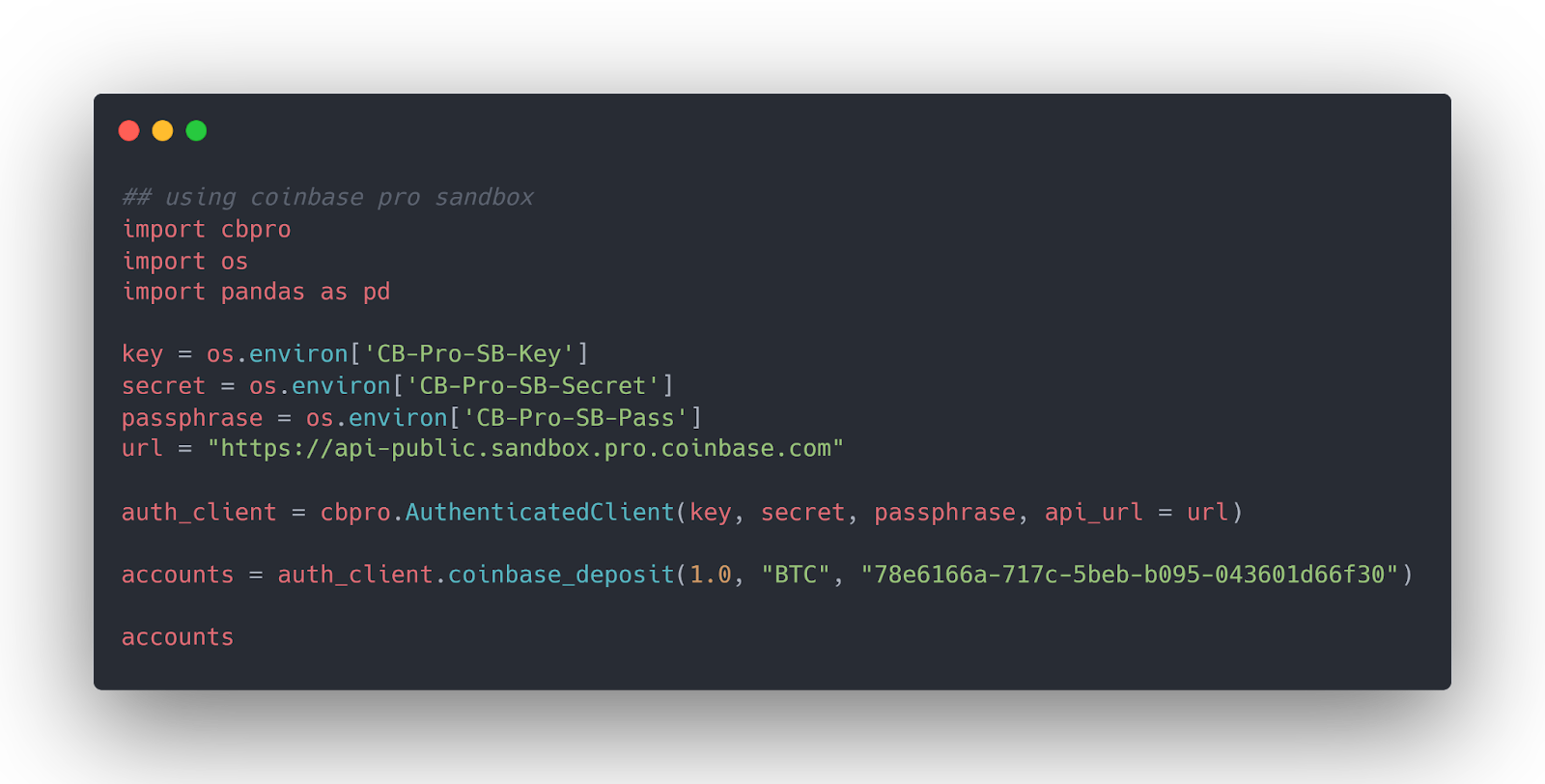
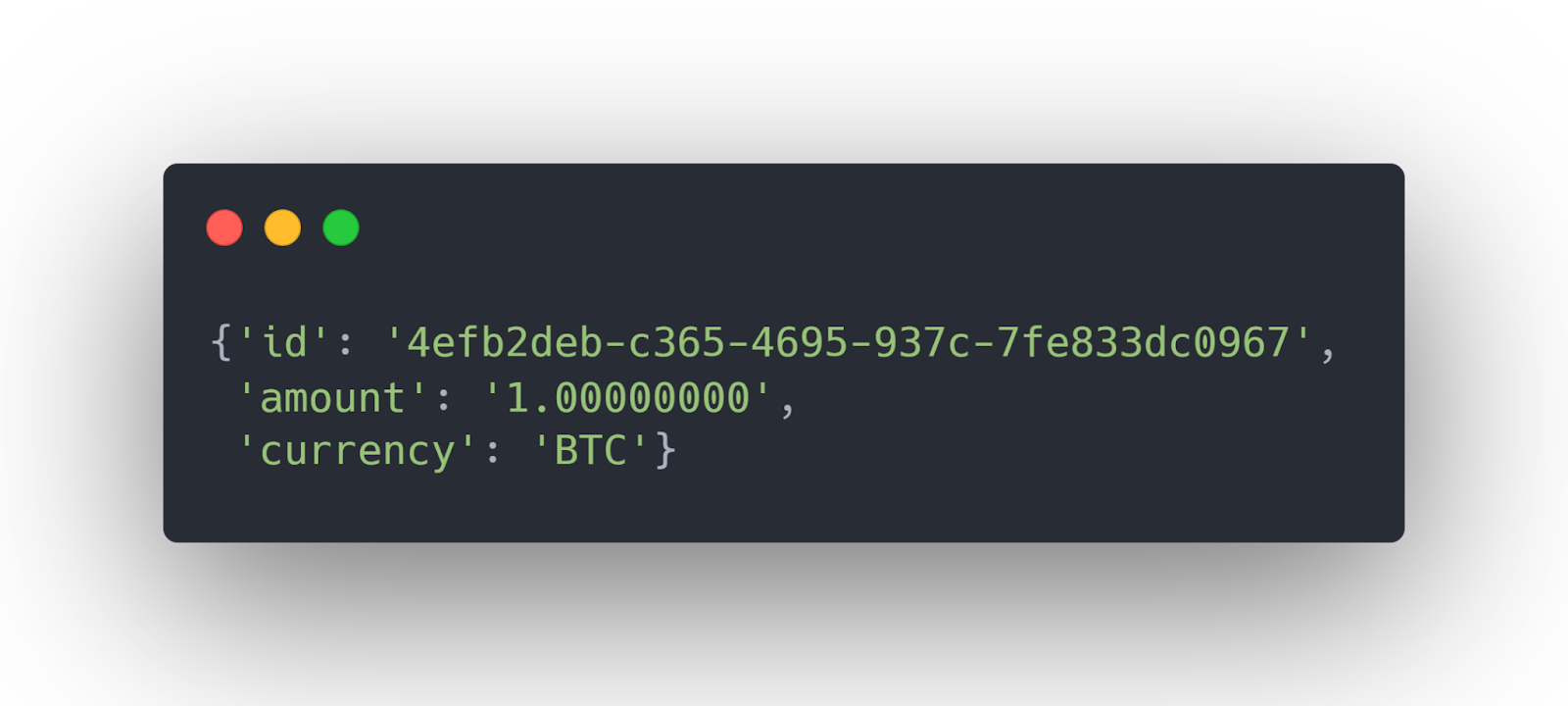
Withdraw Funds to Coinbase Wallet
You can withdraw funds from your Coinbase account to any of your Coinbase wallets using the coinbase_withdraw() method.
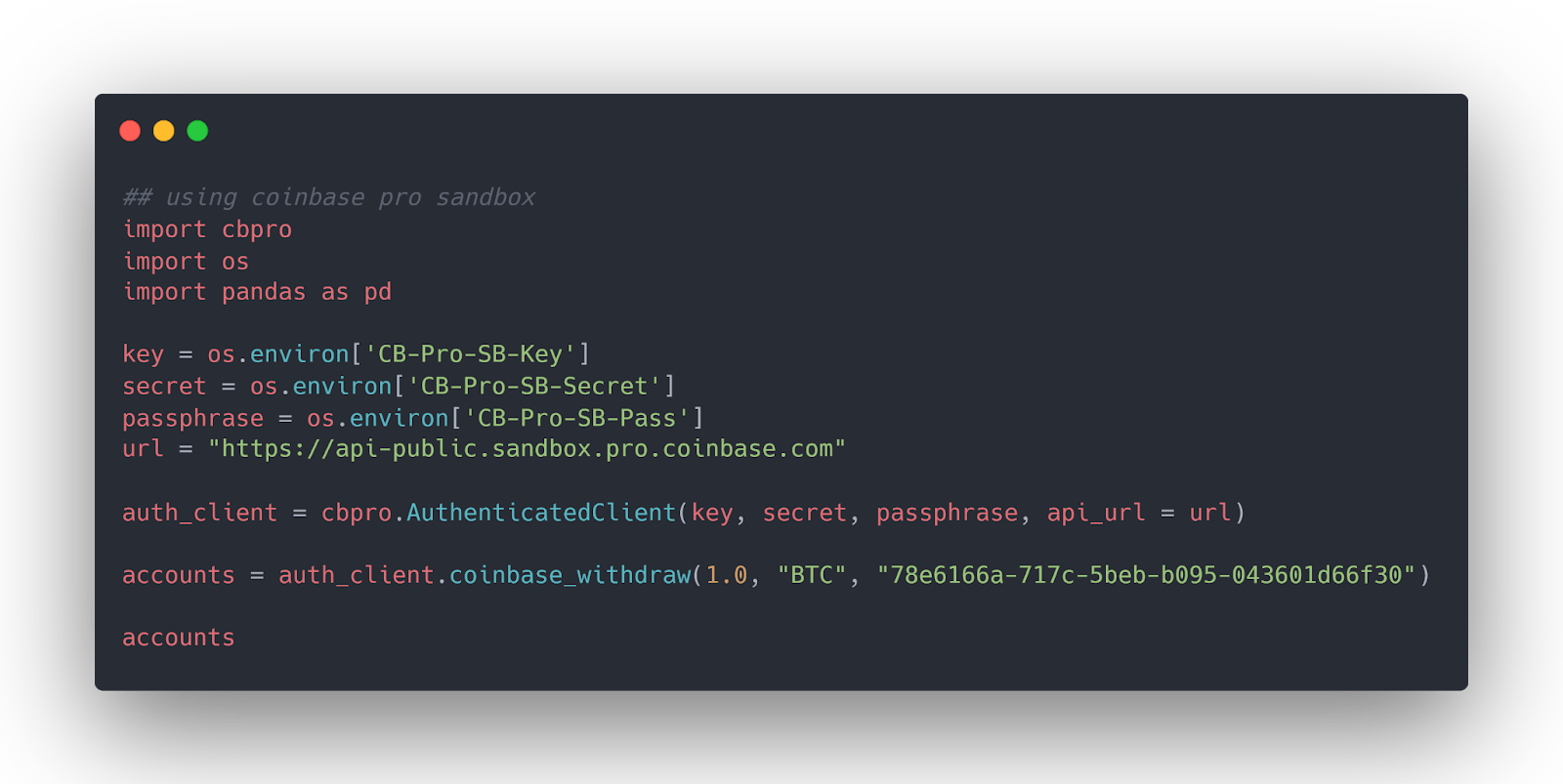
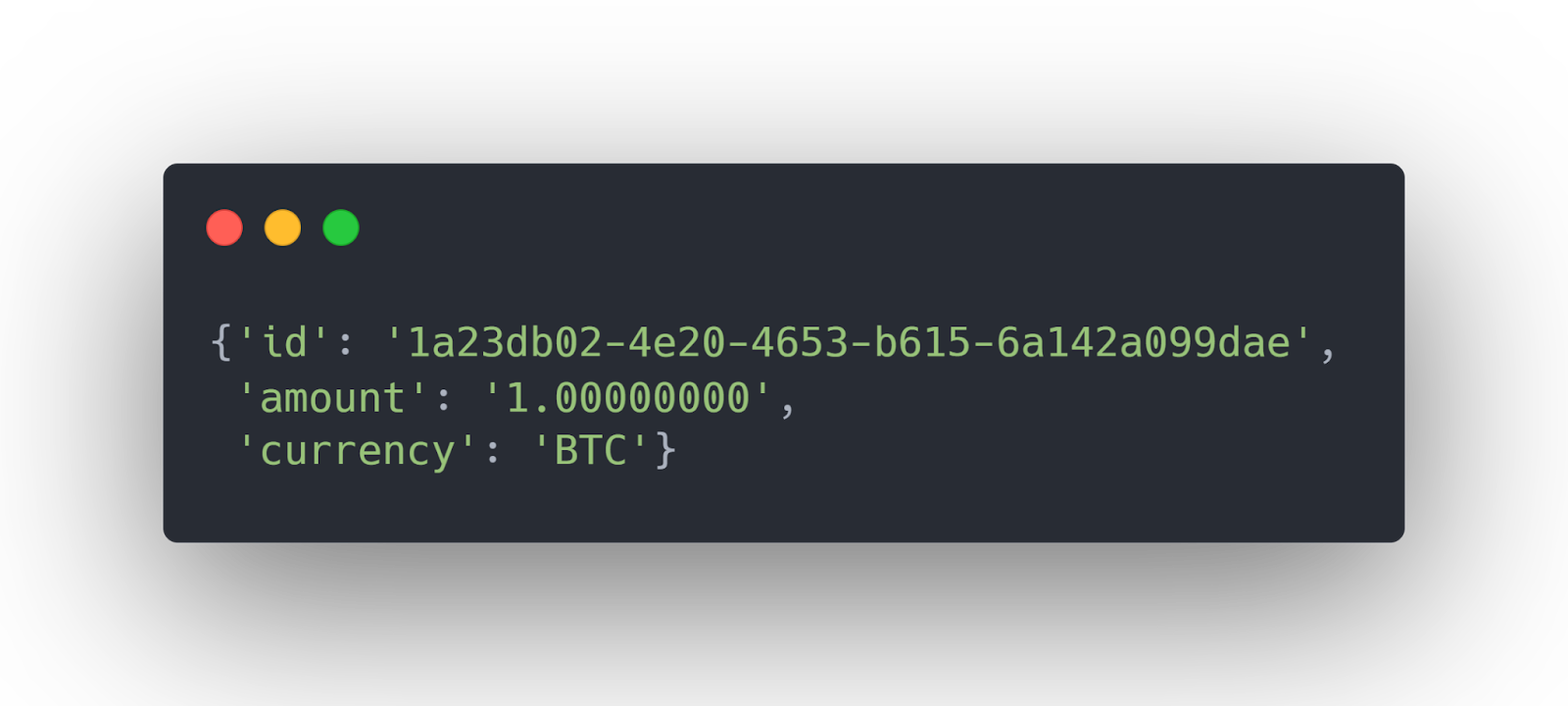
Get All Coinbase Transfers
You can retrieve a list of all your transfers between Coinbase wallets and between Coinbase wallets and Coinbase accounts using the requests library’s get() function.
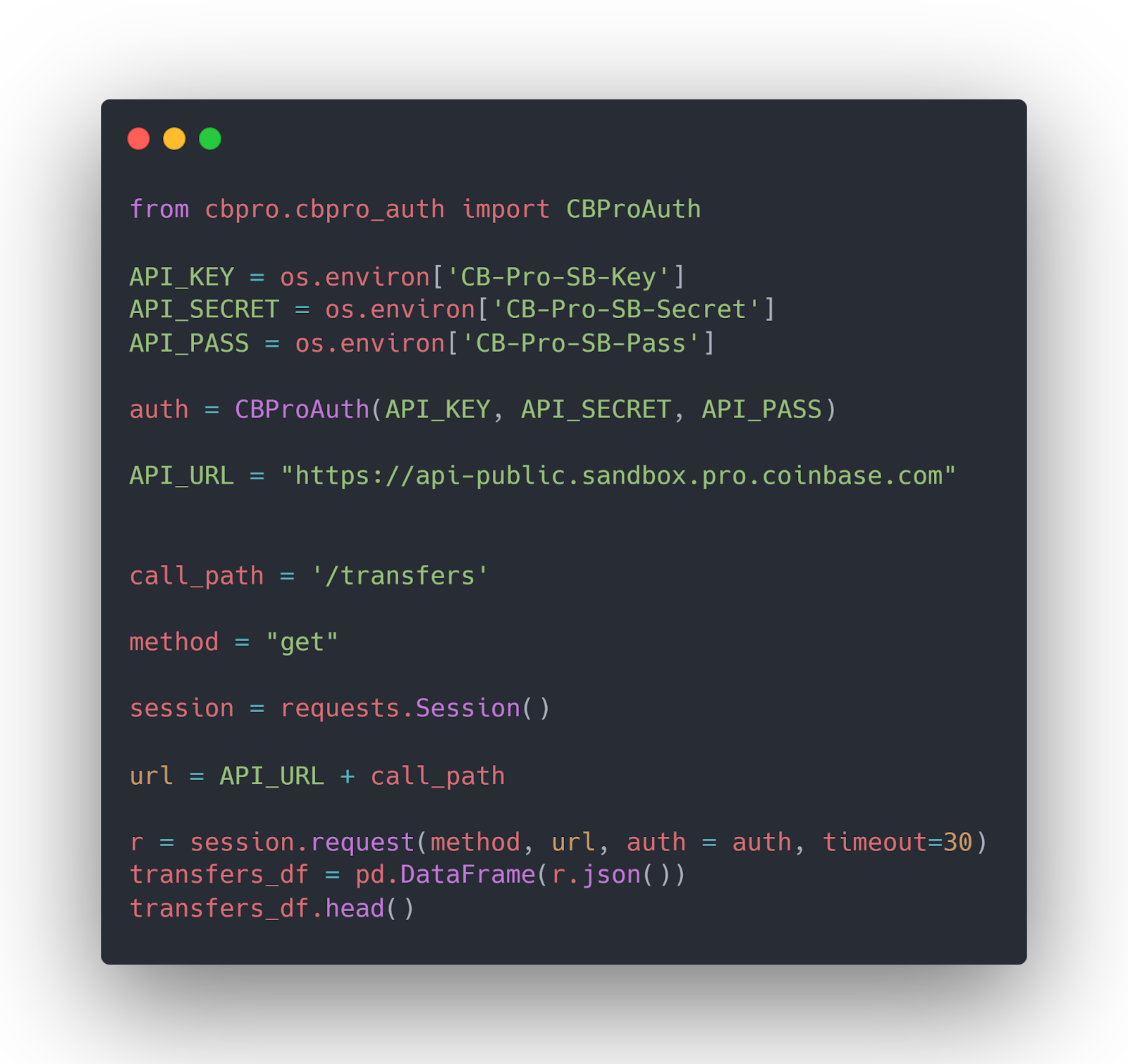
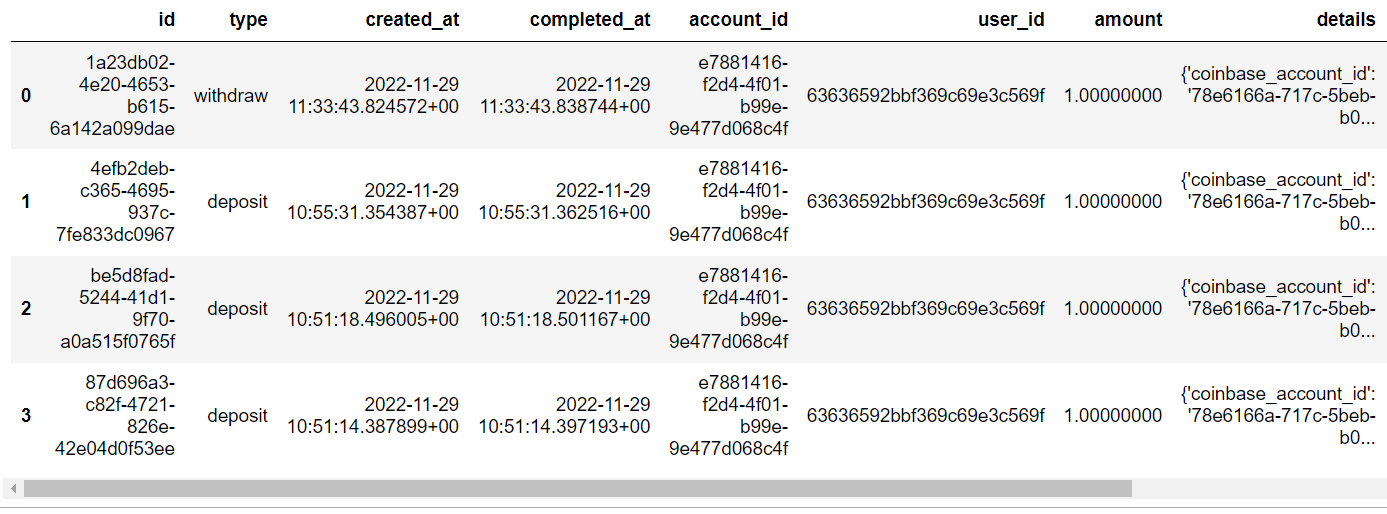
Get a Single Transfer
You can retrieve details of a single transfer by passing the transfer id to the request library’s get() method that calls the corresponding Coinbase Pro API function.
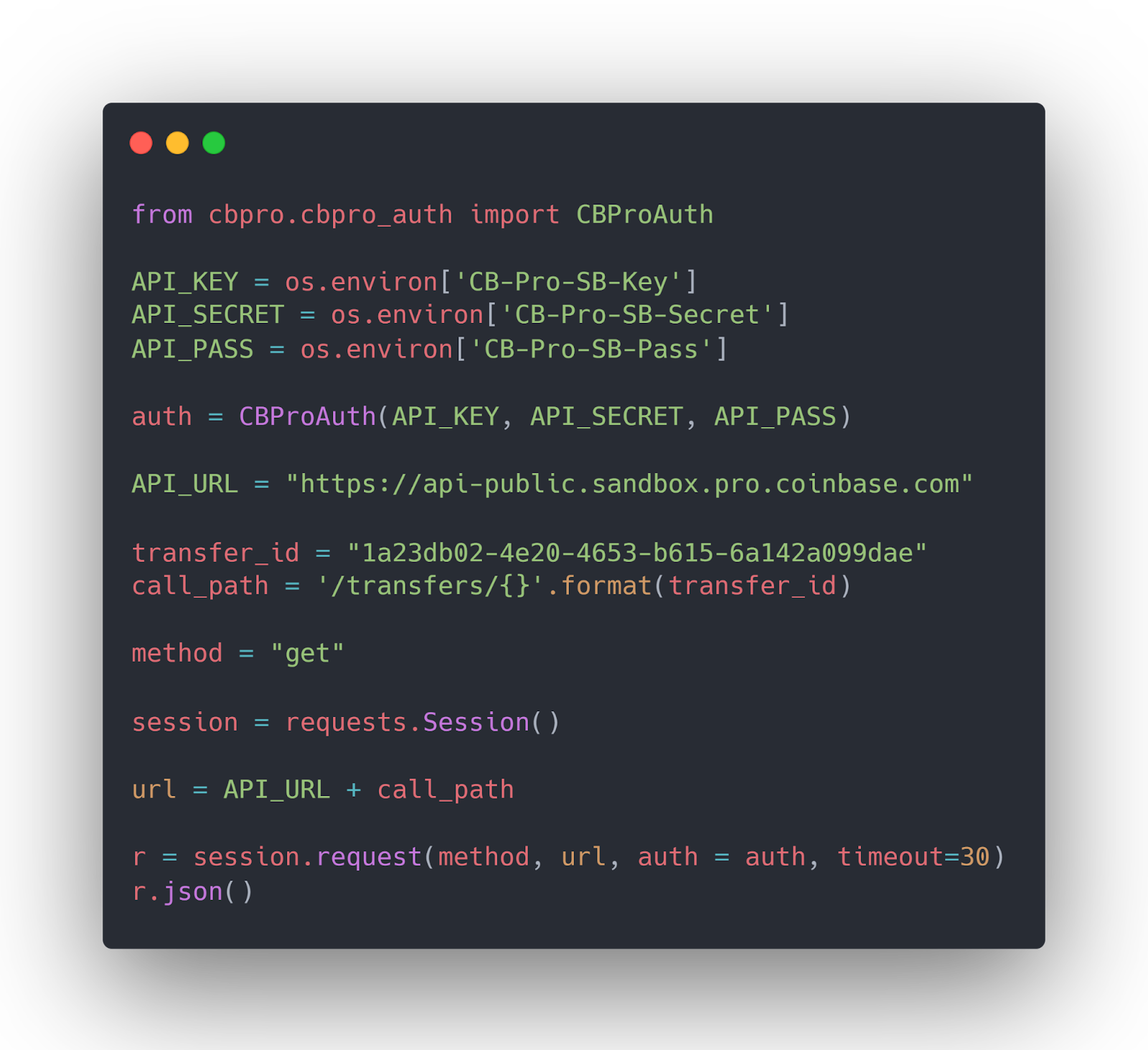
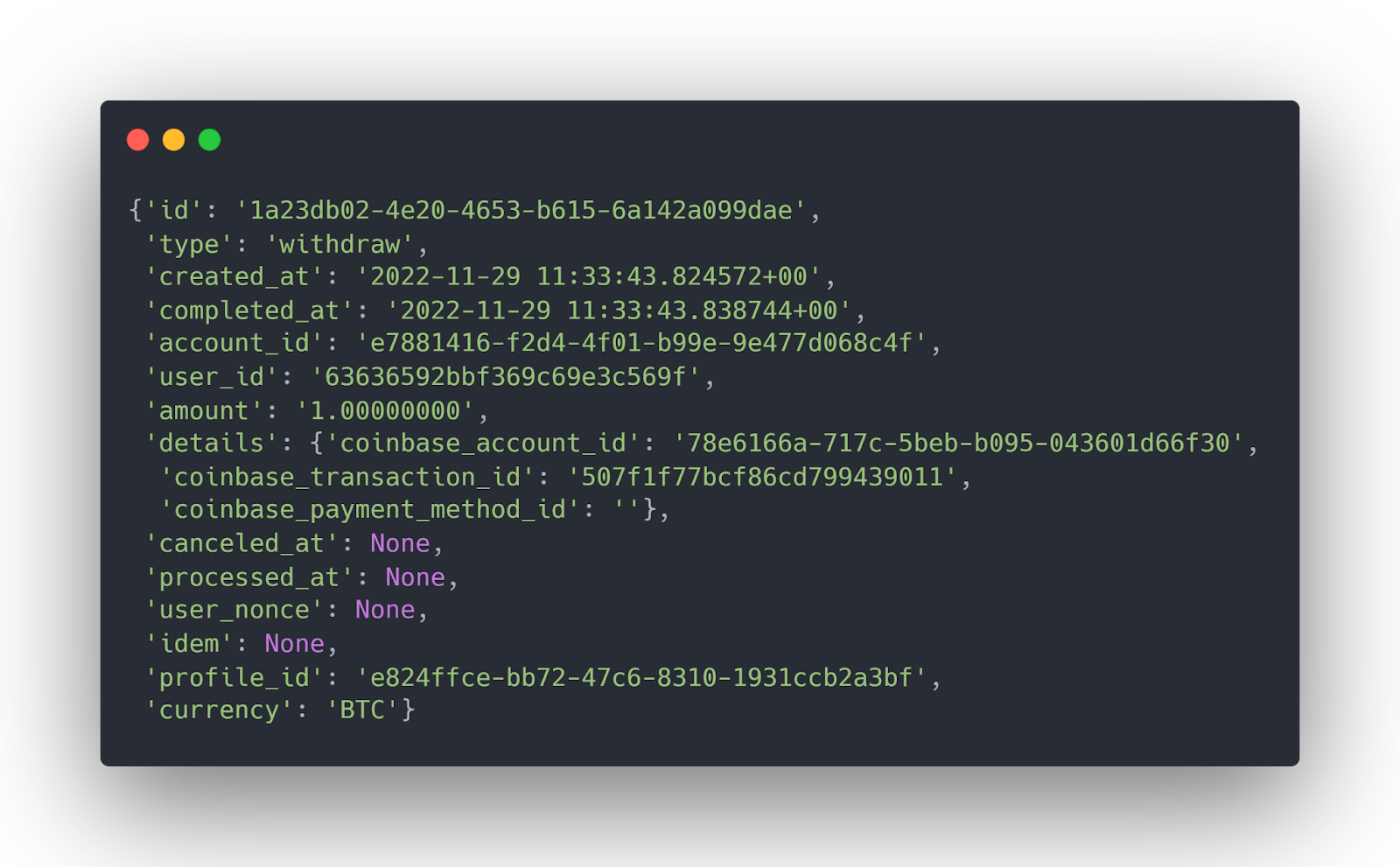
Orders Endpoint
The orders endpoint allows you to place new buying and selling orders for digital assets.
Create a New Order
You can use the place_order() method of the AuthenticatedClient class to create a new order. You must pass the product id, the order side (buy, sell), the amount of the order, and the order type (market, limit, stop) to the place_order() method.
The following script shows how to place a limit order.
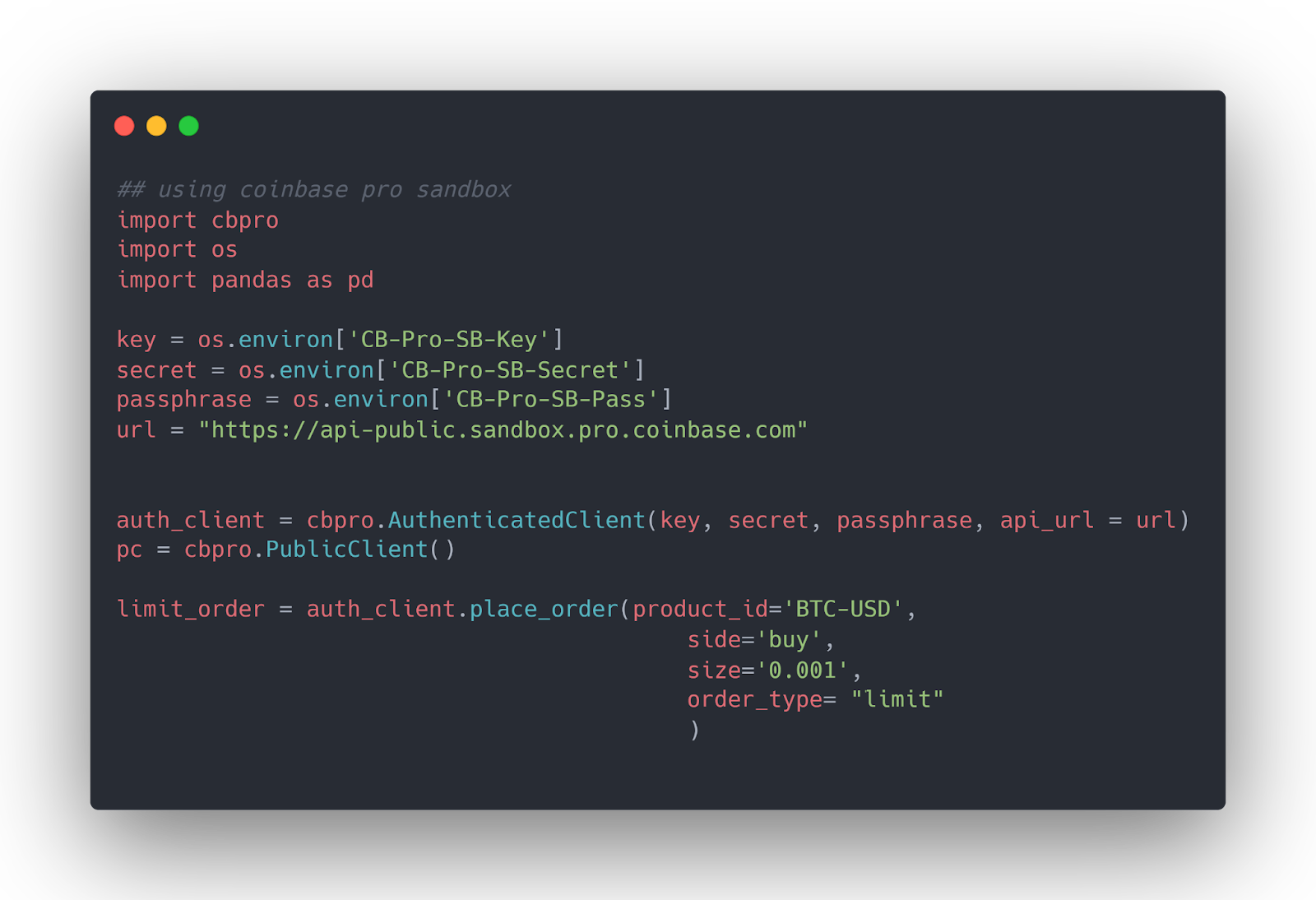
Get All Orders
The get_orders() method returns a list of all your orders, including buying, selling, pending, and completed orders.
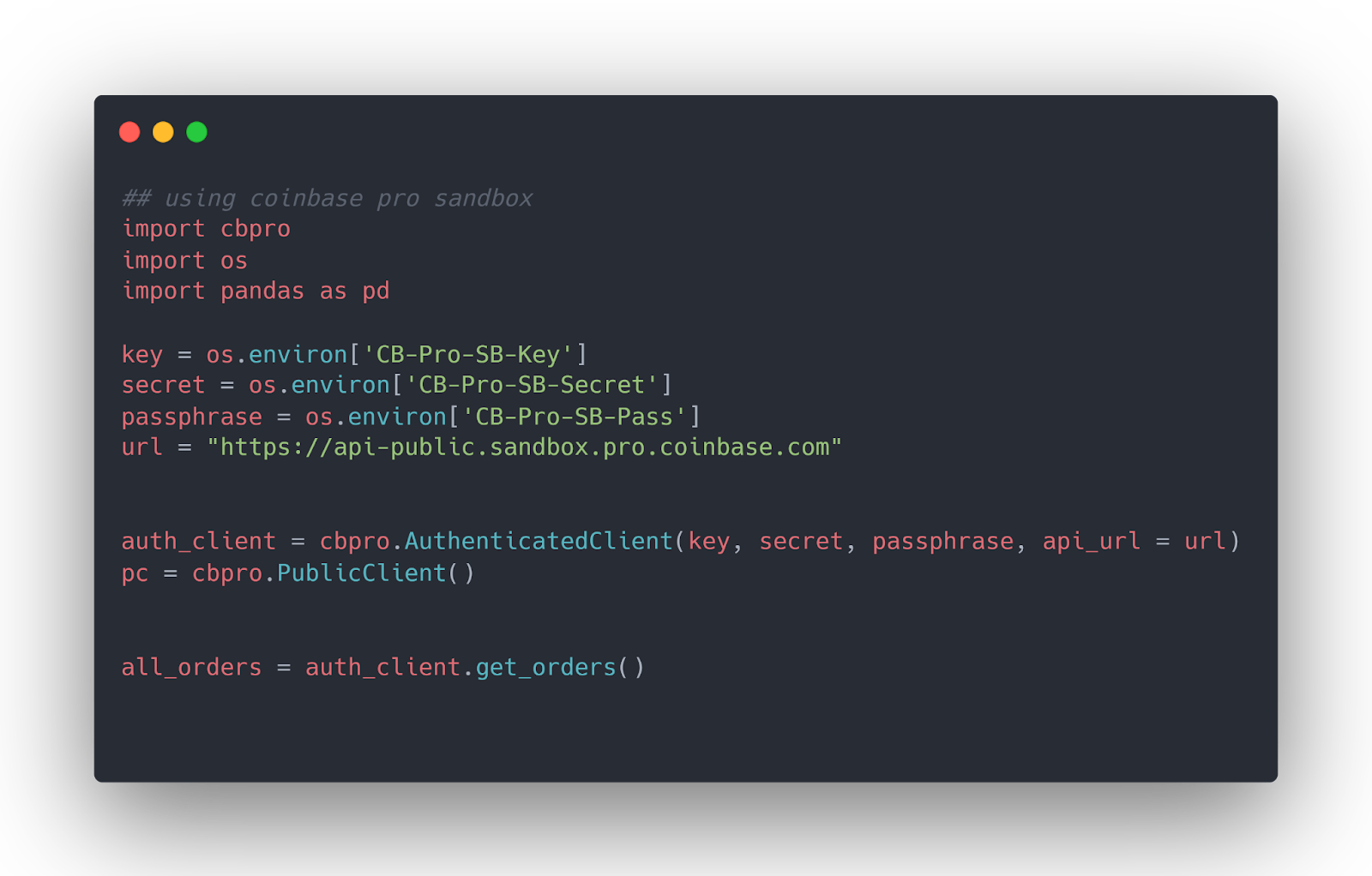
Cancel All Orders
The cancel_all() method allows you to cancel all your open orders:
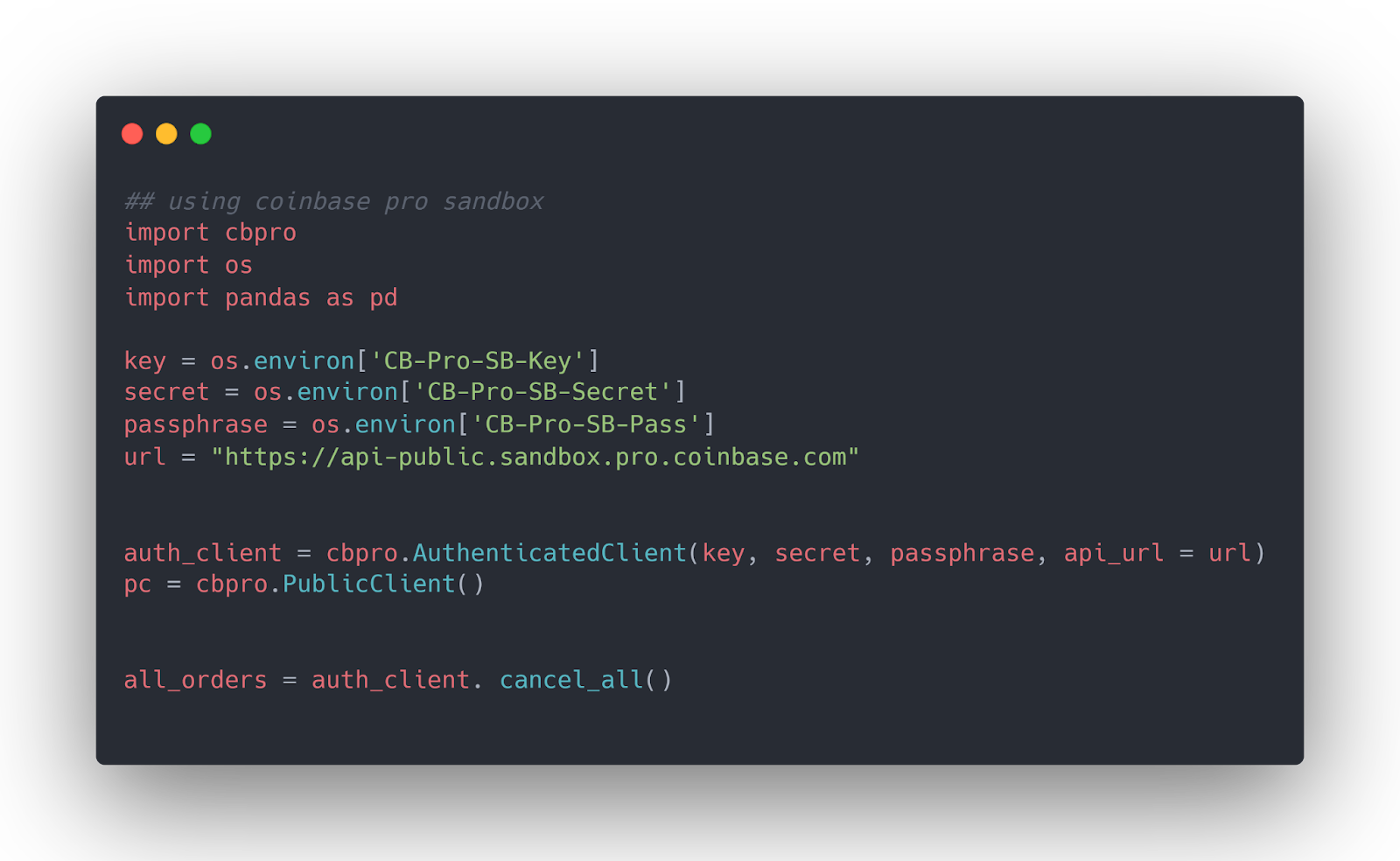
Profiles Endpoint
You can create and retrieve all user profiles for the API key using the profiles endpoint functions.
Get All Current User Profiles
To get all user profiles for a Coinbase account, you can use the requests library’s get() method, as the following script demonstrates.
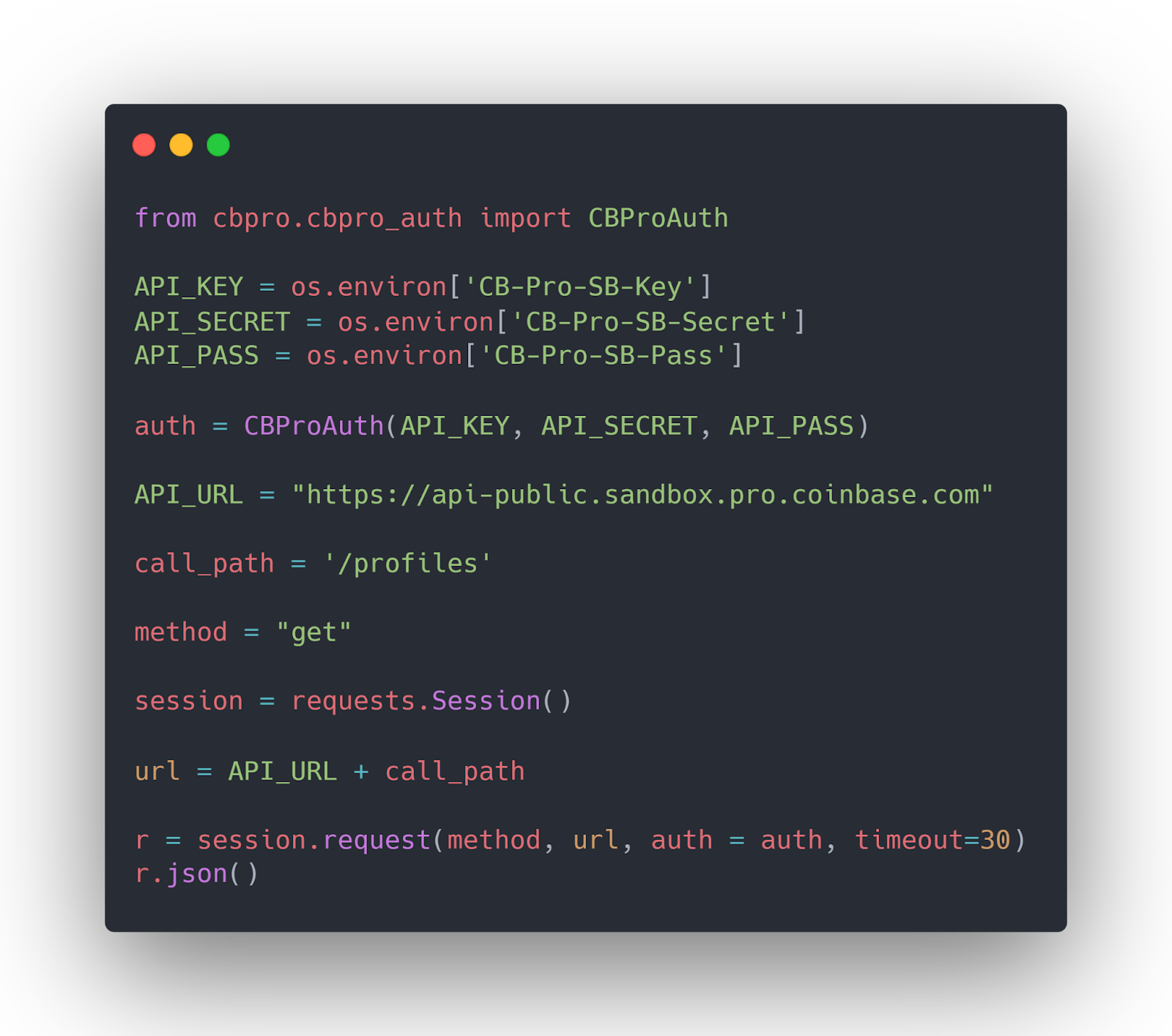
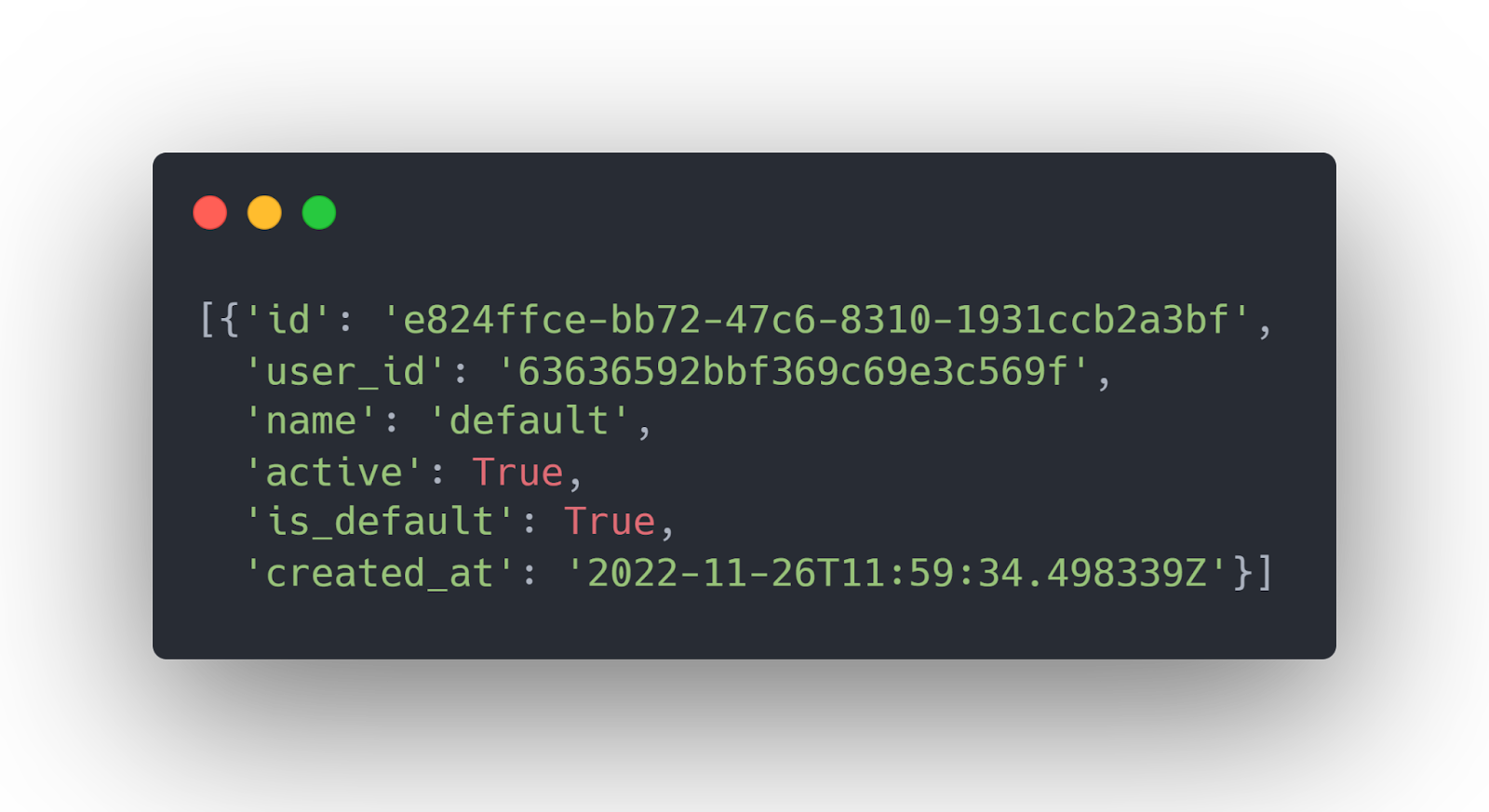
Get Profile by ID
To get details of any user profiles, you can use the request library’s get() function, which calls the corresponding Coinbase Pro API function. You must pass the profile id in the get() function’s URL.
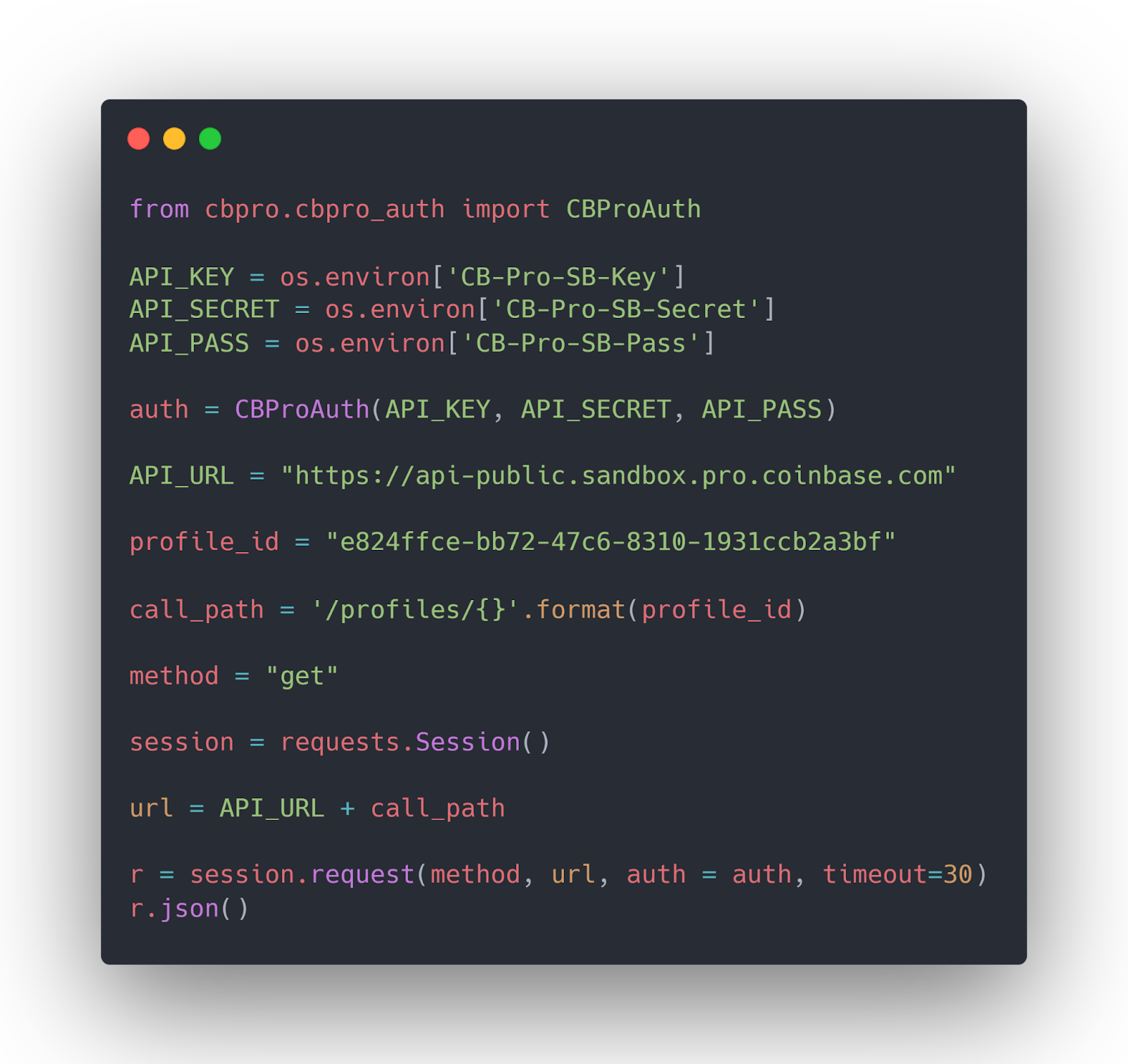
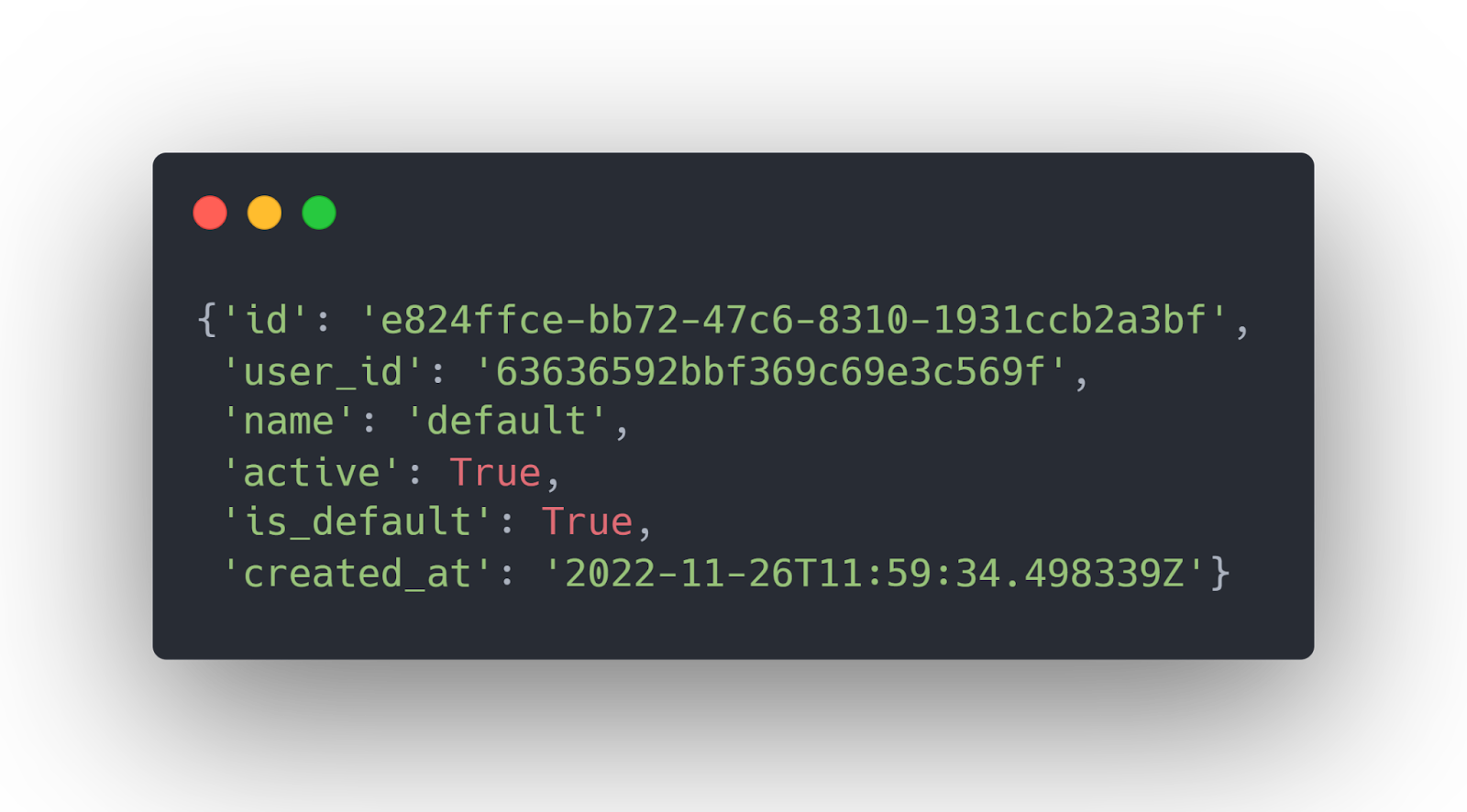
Common Errors and Issues Accessing Coinbase Pro API
Create a New Profile
I faced an issue while creating a new profile using the profiles endpoint. The coinbase-pro library doesn’t implement a function for this endpoint. I used the requests library and followed the instructions on the Coinbase Pro documentation.
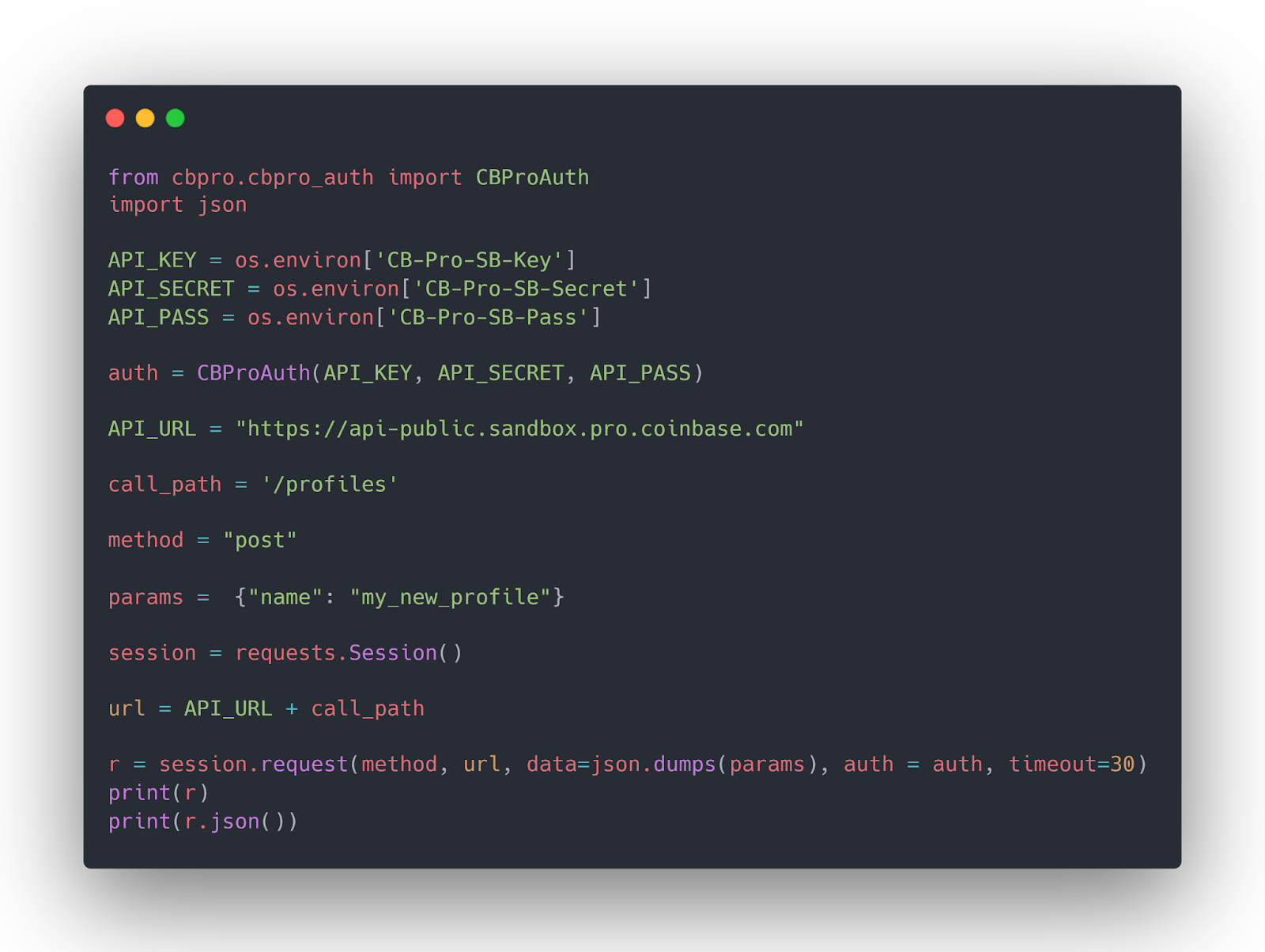
I receive the “Forbidden” message type with a 403 response, a status code for unauthorized scope.
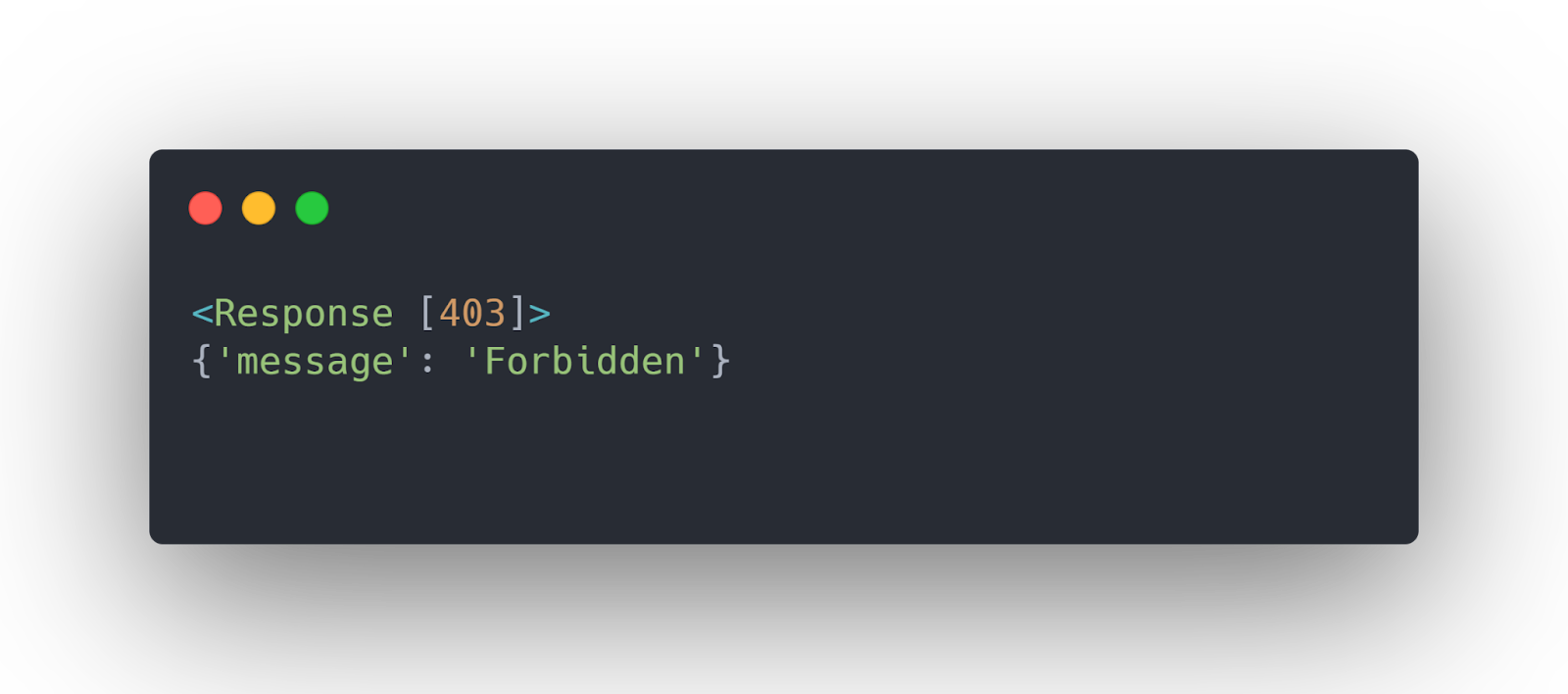
I get the same error when I try to run the above script with the real account instead of the sandbox account.
Coinbase Pro Alternatives
Frequently Asked Questions
Can You Short on Coinbase Pro?
Yes, you can short on Coinbase Pro. To do so, you must deposit money into Coinbase Pro and then open a short position at a price lower than the market price.
Is Coinbase Pro Shutting Down?
As per Coinbase’s official announcement from June 2022, Coinbase is shutting by 2022. Advance Trade will replace Coinbase Pro. However, Coinbase Pro API will continue to function indefinitely since Advance Trade API development is in progress.
Does Cointracker Work for Coinbase Pro?
Yes, CoinTracker works for Coinbase Pro and is free for 3000 transactions.
Can You Use Trading Bots on Coinbase Pro?
Coinbase Pro doesnt have a proprietary trading bot. However, you can integrate third party bots such as CryptoHopper and Bitsgap with Coinbase Pro.
Does Coinbase Pro Have Learn and Earn?
Coinbase Pro has a learning rewards system where you can earn a crypto balance for learning Coinbase Pro features.
Is Binance Better than Coinbase Pro?
Binance and Coinbase Pro have very high feature similarities between them. Binance has lower fees than Coinbase Pro, which makes Binance a better option. However, Binance is not available in the US. You can use Binance US, but it has fewer crypto options, making Coinbase Pro the better option for US customers.
Does Coinbase Pro Track Losses?
You cannot directly track your losses on Coinbase Pro. Coinbase recommends downloading your transaction history from the statements section of Coinbase Pro and tracking your gains and losses by connecting your account to CoinTracker.
Can I Withdraw Crypto Immediately on Coinbase Pro?
In most cases, cashouts to bank accounts take place within 60 seconds. However, in some cases, it can take up to 24 hours.
What Network Does Coinbase Pro Use for ETH?
Coinbase supports all Ethereum and EVM-compatible networks. See the complete list.
The Bottom Line
Coinbase Pro is a one-stop online exchange for buying, selling, and trading activities. You have the option to access Coinbase via a GUI interface or a REST API. With the latter, you can develop customized financial software for yourself or your clients.
This article explained how to call the Coinbase Pro API endpoints in Python. You can use a third-party coinbase-pro Python library or the Python requests library to call the Coinbase Pro API endpoints. Owing to its ease of use, I recommend using the coinbase-pro library to access the Coinbase Pro API. For the scenarios where you cannot use the coinbase-pro library, you can revert to the requests library.