This article will show you how to access the CoinGecko API endpoints in Python to retrieve live cryptocurrency information. You will use the pycoingecko and the Python requests library to fetch data from CoinGecko API.
The official CoinGecko API and pycoingecko libraries’ documentations lack concrete examples and explanations. Despite having over a decade of Python programming experience, It took me a substantial amount of time to execute scripts that fetch data from the CoinGecko API. I wrote this article so that you don’t have to go through the same hassle as I did.
You will see concrete examples of how to call the various CoinGecko API endpoints using the pycoingecko library to access digital currency prices, market volumes, exchanges, indexes, NFTs, etc. You will also study using the Python requests module to access the CoinGecko API.
Whether you are a beginner, an advanced trader, or a Python developer, this tutorial serves as the all-in-one reference guide to fetch data from the CoinGecko API.
What Is CoinGecko?
CoinGecko is a cryptocurrency information platform that provides live and historical insights into cryptocurrency prices, trading volume, market capitalization, exchanges and indexes, and much more. In addition, CoinGecko tracks on-chain metrics, community growth, major cryptocurrency events, and open-source code development.
Founded by TM Lee and Bobby Ong in 2014, CoinGecko is headquartered in Kuala Lumpur, Malaysia.
What Is the CoinGecko API?
CoinGecko API is a set of REST API functions that lets developers access and exchange CoinGecko data via a programming language.
CoinGecko API has a free plan that lets you access the API endpoints without subscribing or signing up with CoinGecko. With the free plan, you can make between 10-50 API calls per minute.
To access the CoinGecko advanced plans (analyst, lite, pro), you must sign up and subscribe with the CoinGecko API. To do so, go to the CoinGecko API pricing page, and select the subscription option that suits your needs.
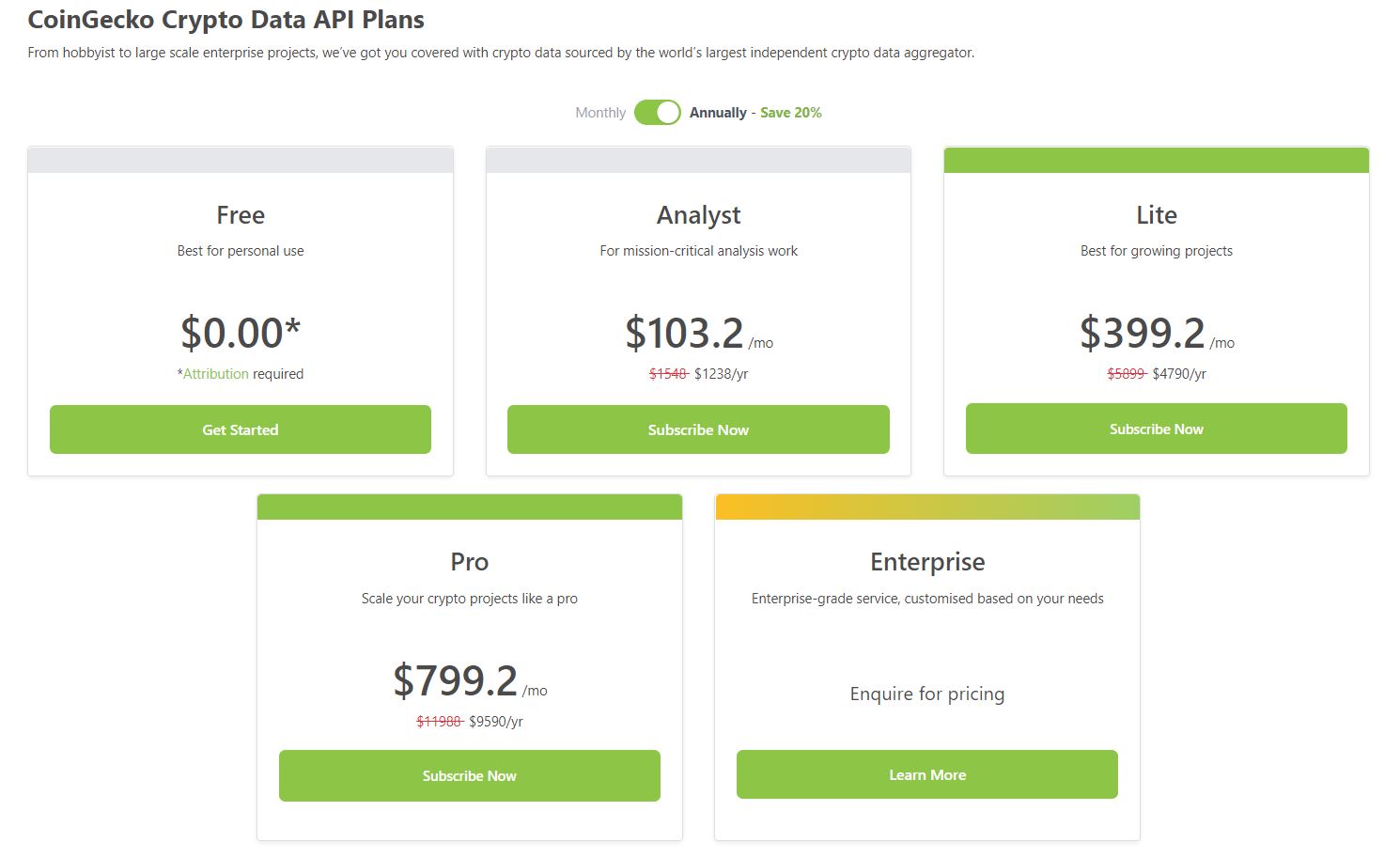
Image Source: CoinGecko API Plans
CoinGecko Pros and Cons
Pros:
- It has a free plan.
- Frequent API updates.
- Provides the latest cryptocurrency information with a recency rate of between 1 and 2 minutes.
- It contains a large number of crypto assets.
- No listing fees resulting in a large number of available coins.
- Highly transparent methodology of aggregating cryptocurrency information.
- Supports multiple languages.
Cons:
- The information is often incorrect.
- It has a limited number of endpoints.
- Has no official client libraries.
- Users report bugs in CoinGecko mobile apps.
How to Get Started with the CoinGecko API in Python?
With the CoinGecko free plan, you don’t need to sign up for a CoinGecko account. To use pro features, you must sign up with CoinGecko and subscribe to one of their advanced plans.
Simple Example Using CoinGecko API in Python
CoinGecko doesn’t have an official Python client. However, third-party Python libraries allow you to fetch data via API calls to the CoinGecko API. The other option is to use the Python requests() method to make API calls to the CoinGecko API. You will see both approaches in this section.
CoinGecko API pycoingecko Library Example
The Python pycoingecko wrapper for the CoinGecko API allows you to call the API endpoints. The following pip command installs this library.
# install the library with the following command
pip install pycoingecko
The first step is to import the CoinGeckoAPI class from the pycoingecko module.
from pycoingecko import CoinGeckoAPI
cg = CoinGeckoAPI()
If you have a pro subscription, you need to initialize the CoinGeckoAPI class with your API key, as shown in the following script.
# for users with Pro API Key
from pycoingecko import CoinGeckoAPI
cg = CoinGeckoAPI(api_key='YOUR_API_KEY')
Once you initialize the CoinGeckoAPI class, you can call any CoinGecko API endpoint function.
For instance, the following code uses the get_price() method to get Bitcoin’s current USD price.
cg.get_price(ids='bitcoin', vs_currencies='usd')
{'bitcoin': {'usd': 19337.76}}
Some of the API functions require you to pass parameter values. To see the details of the required parameters for each function, see the official CoinGecko API documentation.
Let’s see another example. The following script returns current prices for Bitcoin, Litecoin, and Ethereum in USD and Euros.
price_dict = cg.get_price(ids='bitcoin,litecoin,ethereum', vs_currencies='usd,eur')
price_dict
Output:
{'bitcoin': {'usd': 19337.76, 'eur': 19728.39},
'ethereum': {'usd': 1331.82, 'eur': 1358.72},
'litecoin': {'usd': 53.51, 'eur': 54.59}}
For a better view, you can convert the output from some of the functions to a Pandas dataframe:
import pandas as pd
price_df = pd.DataFrame(price_dict)
price_df.head()
Output:
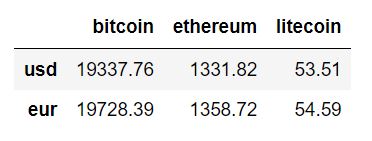
CoinGecko API Requests Module Example
Third-party client wrappers can be slower, and they often do not immediately implement API updates. If speed is a concern, I recommend using the Python request() function to call the CoinGecko API.
The following script shows how to get current cryptocurrency prices using the Python requests module.
import json import requests import os import pandas as pd api_url = 'https://api.coingecko.com/api/v3/' request = 'simple/price' # make API request res = requests.get(api_url + request, params={'ids': 'bitcoin,litecoin,ethereum', 'vs_currencies': 'usd,eur'}) # convert to pandas dataframe price_df = pd.read_json(res.text) price_df.head()
import json
import requests
import os
import pandas as pd
api_url = 'https://api.coingecko.com/api/v3/'
# make API request
request = 'simple/price'
res = requests.get(api_url + request,
params={'ids': 'bitcoin,litecoin,ethereum', 'vs_currencies': 'usd,eur'})
# convert to pandas dataframe
price_df = pd.read_json(res.text)
price_df.head()
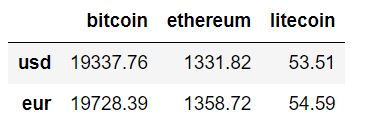
In the rest of this article, I will use the pycoingecko library functions. For the cases where the pycoingecko library has no functions for an endpoint, I will switch to the default Python requests module.
It is important to note that the pycoingecko library doesn’t provide detailed documentation linking its functions with CoinGecko API calls. However, the CoinGeckoAPI class is well commented, where you can see the API calls made by the CoinGeckoAPI class functions.
For example, the following screenshot depicts the implementation of the get_price() method of the pycoingecko library. You can see that this method falls under the Simple endpoint category and calls the simple/price API function.
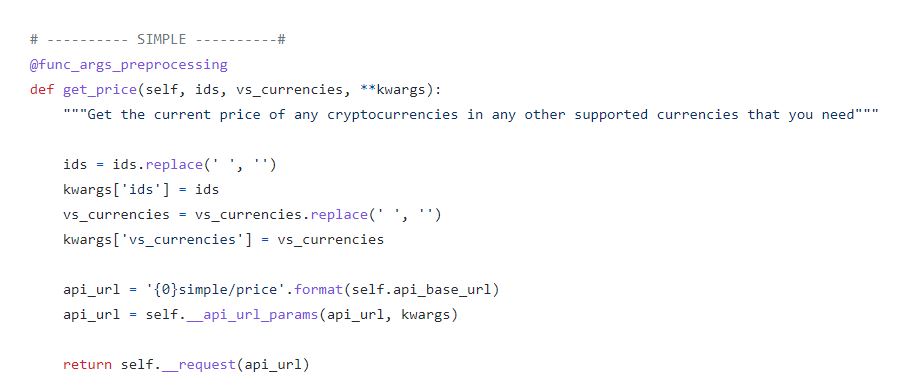
What Are Coingecko API Endpoints?
The following endpoints are available with the CoinGecko API:
- ping
- simple
- coins
- contract
- asset_platforms
- categories
- exchanges
- indexes
- derivatives
- nfts (Beta)
- exchange_rates
- search
- trending
- global
- companies (beta)
Ping Endpoint
The ping endpoint contains one function that returns the CoinGecko API server status.
How to Check CoinGecko API Server Status?
You can check API server status for the CoinGecko API using the ping() method.
from pycoingecko import CoinGeckoAPI
cg = CoinGeckoAPI()
cg.ping()
from pycoingecko import CoinGeckoAPI
cg = CoinGeckoAPI() cg.ping()
If you see the following response, the server is up and running.
{'gecko_says': '(V3) To the Moon!'}
Simple Endpoint
The simple endpoint provides functions to get basic cryptocurrency information, e.g., supported coins’ price values and a list of supported currencies.
How to Get CoinGecko Cryptocurrency Prices
You can call the get_price() method to get real-time cryptocurrency prices in other supported currencies. You must pass coin ids and the other currency values to the get_price() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
price_dict = cg.get_price(ids='bitcoin,litecoin,ethereum',
vs_currencies='usd,eur',
include_market_cap = True,
include_24hr_vol = True)
price_df = pd.DataFrame(price_dict)
price_df.head(10)
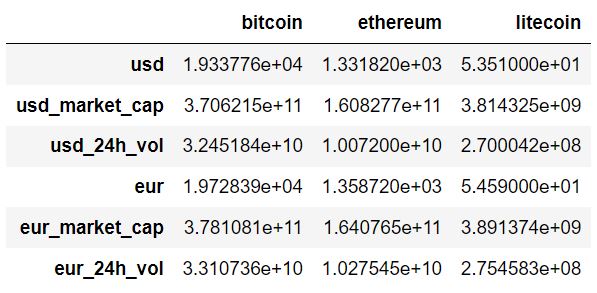
To get a list of all currencies that support interconversion, you can use the get_supported_vs_currencies() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
vs_currencies = cg.get_supported_vs_currencies()
vs_currencies_df = pd.DataFrame(vs_currencies)
vs_currencies_df.head(10)
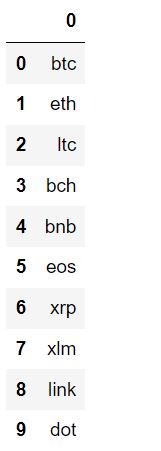
Coins Endpoint
The coins endpoint functions return information such as coins list, historical data, coins market data, etc.
List All CoinGecko Coins
The get_coins_list() method returns id, symbol, and name for all the supported coins at CoinGecko.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
all_coins_list = cg.get_coins_list()
all_coins_list_df = pd.DataFrame(all_coins_list)
all_coins_list_df.head()
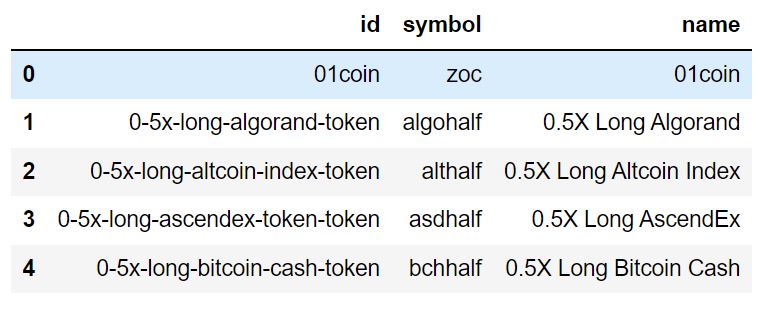
You can get more detailed information, e.g., name, market price, and location info for all coins using the get_coins() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
all_coins = cg.get_coins()
all_coins_df = pd.DataFrame(all_coins)
all_coins_df.head()
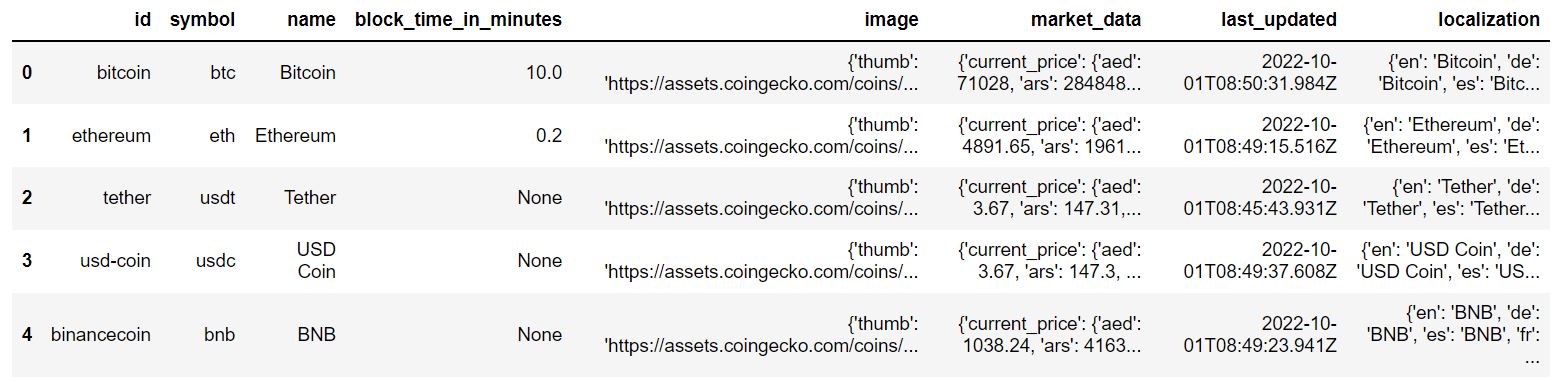
Get CoinGecko Market Data
The get_coins_market() method returns market-related data, e.g., current price, market cap, total volume, etc., for a coin. Here is an example that retrieves market data for a coin in USD.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
all_coins_market = cg.get_coins_markets(vs_currency ='usd')
all_coins_market_df = pd.DataFrame(all_coins_market)
all_coins_market_df.head()
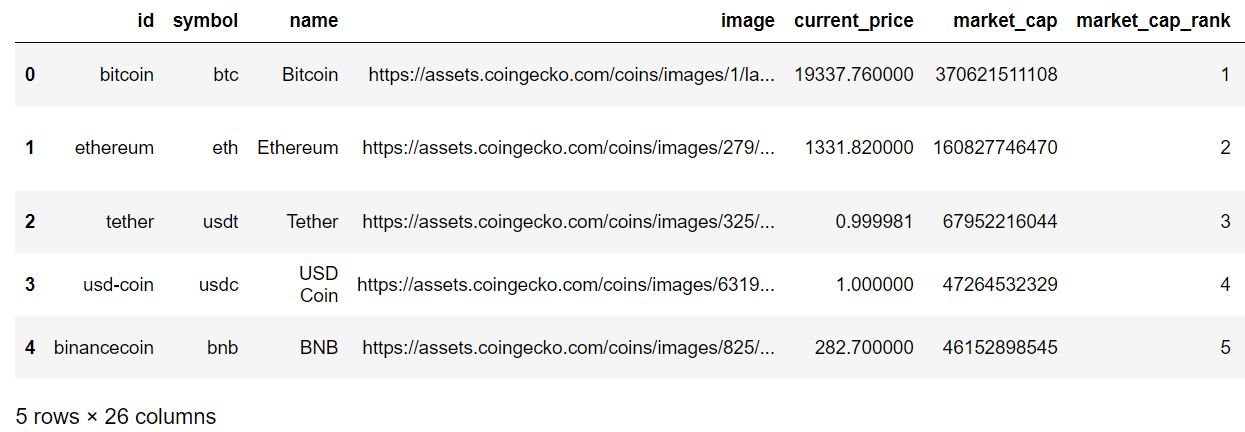
Get CoinGecko Coin Historical Data
The get_coin_history_by_id() method returns historical data such as prices, market caps, trading volume for a coin on a particular date.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
# you can obtain coin id using the get_coins() method
coin_history = cg.get_coin_history_by_id(id ='ethereum',
date = '29-09-2022',
localization = False)
coin_history_df = pd.DataFrame(coin_history)
coin_history_df.head()
Output:
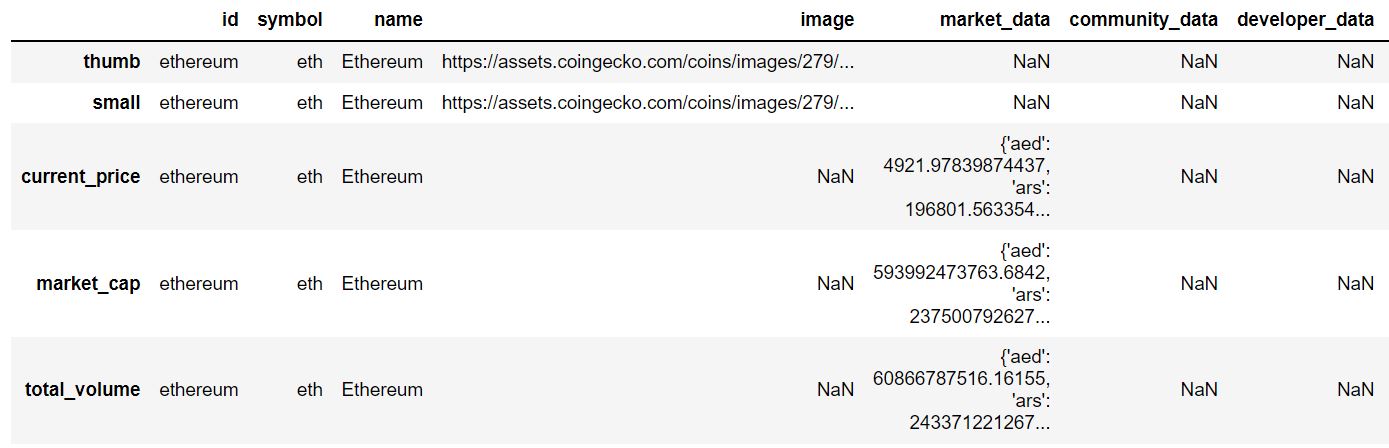
If you want to retrieve historical market data for a coin for a certain number of previous days from the current date, you can use the get_coin_market_chart_by_id() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI() # you can obtain coin id using the get_coins() method
coin_history = cg.get_coin_market_chart_by_id(id ='ethereum',
vs_currency = 'usd' ,
days = 3,
localization = False) coin_history_df = pd.DataFrame(coin_history)
coin_history_df.head()
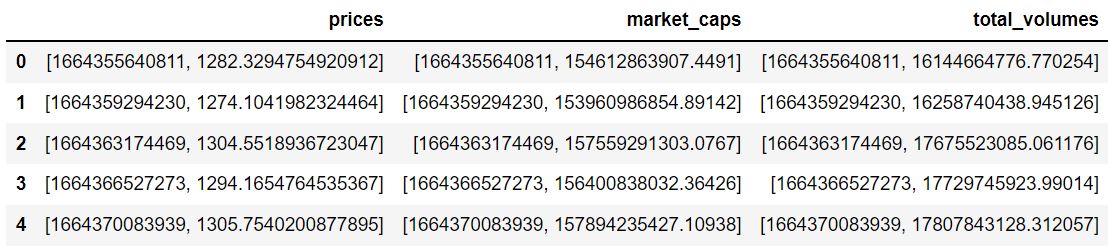
Finally, you can get historical market data between two dates using the get_coin_market_chart_range_by_id() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI() # you can obtain coin id using the get_coins() method
coin_history = cg.get_coin_market_chart_range_by_id(id ='ethereum',
vs_currency = 'usd' ,
from_timestamp = '1661990400', # September 1, 2022
to_timestamp = '1664582399', # September 30, 2022
localization = False)
coin_history_df = pd.DataFrame(coin_history)
coin_history_df.head()
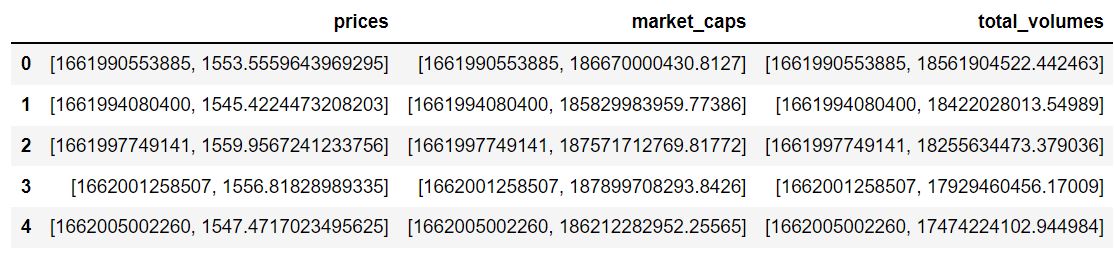
Get CoinGecko OHLC Values
You can retrieve the open, high, low, and closing values for a coin for a certain number of past days from the current date using the get_coin_ohlc_by_id() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
coin_ohlc = cg.get_coin_ohlc_by_id(id ='bitcoin',
vs_currency = 'usd' ,
days = 1)
coin_ohlc_df = pd.DataFrame(
coin_ohlc, columns = ['Time', 'Open', 'High', 'Low', 'Close'])
coin_ohlc_df.head()
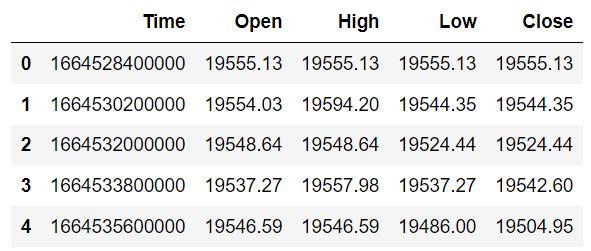
Contract Endpoint
The contract endpoint returns coin information using the contact addresses Contract address is a unique address generated when a contract is deployed on the blockchain and is used to identify a particular transaction or a set of transactions. A contract address is generated after a transaction or a contract. Whereas the regular wallet address is used to send or receive cryptocurrencies and exists before a transaction or a contract takes place.
Get Coin Info from Contract Address with Coingecko API
The get_coin_info_from_contract_address_by_id() method returns coin info, e.g., price values, market cap, trading volume, liquidity score, CoinCecko score, and much more for a specific coin using a contract address. Here is an example:
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
coin_info = cg.get_coin_info_from_contract_address_by_id(id ='ethereum',
contract_address = '0xc00e94cb662c3520282e6f5717214004a7f26888',
localization = False)
coin_info
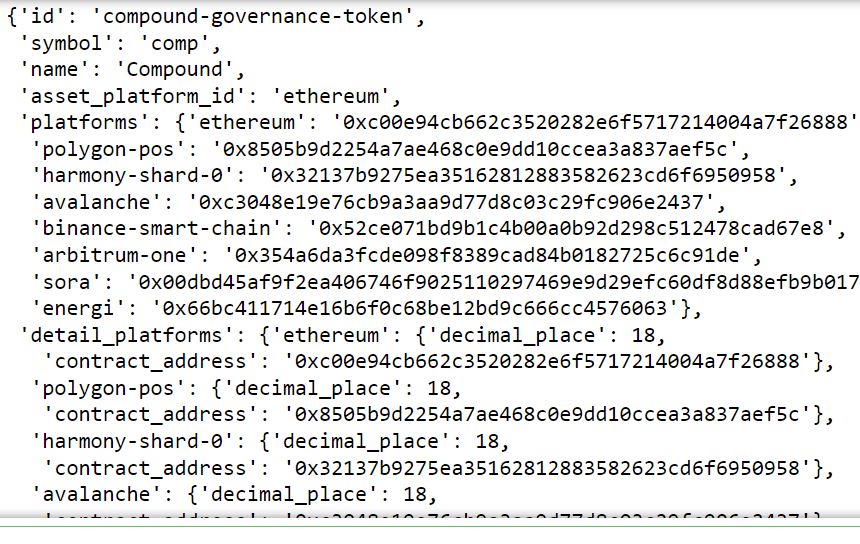
I did not convert the output response to a Pandas dataframe in this case since the Python dictionaries returned by the get_coin_info_from_contract_address_by_id() are asymmetrical. You should also be careful about the type of response you get from an API call before converting it to another format. You will get an exception if you try to convert asymmetrical dictionaries to Pandas dataframes.
Get CoinGecko Historical Coin Info from Contract Address
The get_coin_market_chart_from_contract_address_by_id() method returns historical market data for a coin using a contract address The number of previous days from the current data is specified in the days attribute. For example, the script below returns data from three previous days from the current date.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
coin_info = cg.get_coin_market_chart_from_contract_address_by_id(id ='ethereum',
contract_address = '0xc00e94cb662c3520282e6f5717214004a7f26888',
vs_currency = 'usd' ,
days = 3)
coin_info
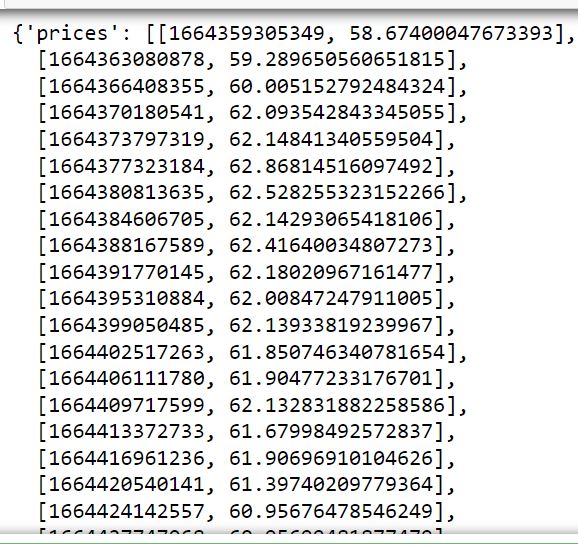
Asset Platforms Endpoint
This endpoint retrieves all asset platforms (blockchain networks) supported by CoinGecko, e.g., cosmos, openledger, etc. You can use this endpoint to see if CoinGecko supports a blockchain network that you want to explore further.
List All CoinGecko Asset Platforms (Blockchain Networks)
The get_asset_platforms() method returns a list of all blockchain networks along with their ids, chain identifiers, names, and short names, as shown in the following script.
from pycoingecko import CoinGeckoAPI
import pandas as pd cg = CoinGeckoAPI()
asset_platforms = cg.get_asset_platforms()
asset_platforms_df = pd.DataFrame(asset_platforms)
asset_platforms_df.head()
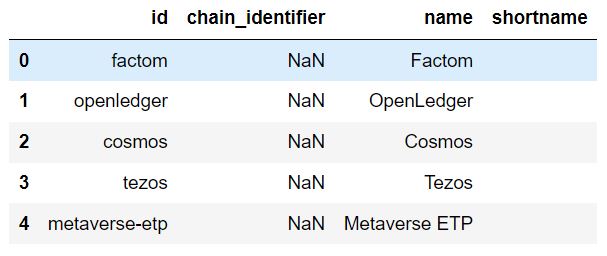
Categories Endpoint
You might be interested in retrieving coin information from a specific type of CoinGecko coin category. A category in CoinGecko is collections of certain tokens. For example, the Cardano category contains tokens that belong to the Arbitrum category. How to Get CoinGecko Coin Categories
To get a list of all the supported coin categories, you can use the get_coins_categories_list() method, as demonstrated in the following code.
from pycoingecko import CoinGeckoAPI
import pandas as pd cg = CoinGeckoAPI()
categories = cg.get_coins_categories_list()
categories_df = pd.DataFrame(categories)
categories_df.head()
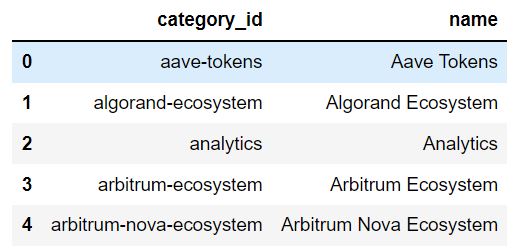
Similarly, you can use the get_coins_categories() method to get a list of all coin categories with market data. With this method, you can see the top 3 coins per category, the total market cap, the total market cap and volume change in the last 24 hours, and so on.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
categories = cg.get_coins_categories()
categories_df = pd.DataFrame(categories)
categories_df.head()
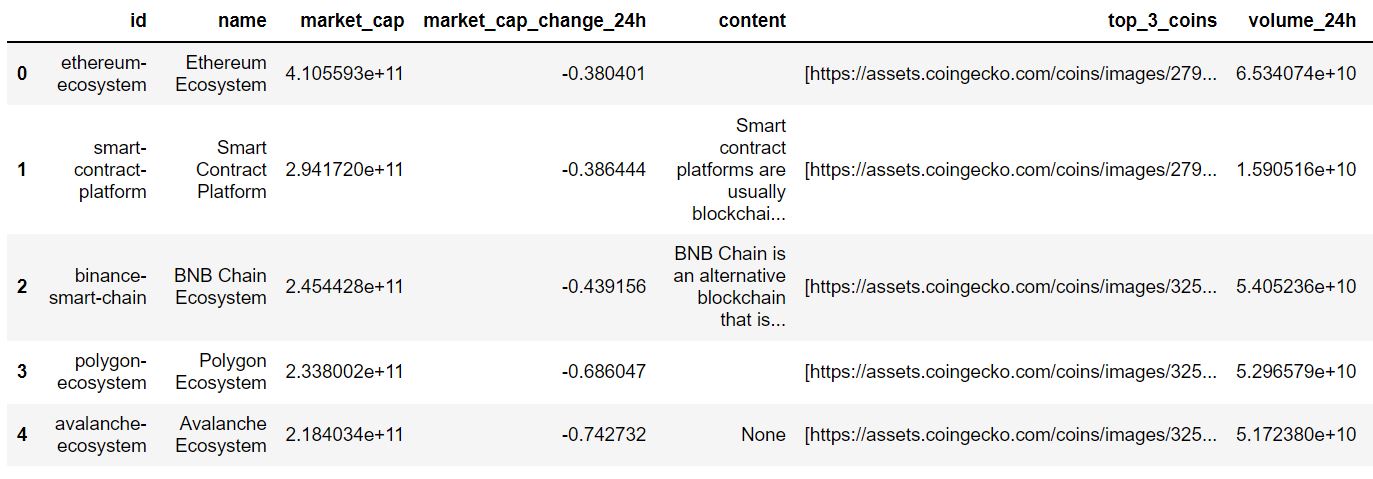
Exchanges Endpoint
The exchange endpoint allows you to get a list of all exchanges, exchange tickers, and volume information, etc. You can also see market caps and trading columns per exchange, along with each exchange’s trust score, which can help you decide the exchange you want to use for trading.
How to Get All Coingecko Listed Exchanges
The get_exchanges_list() method returns a list of all cryptocurrency exchanges supported by CoinGecko.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
exchanges = cg.get_exchanges_list()
exchanges_df = pd.DataFrame(exchanges)
exchanges_df.head()
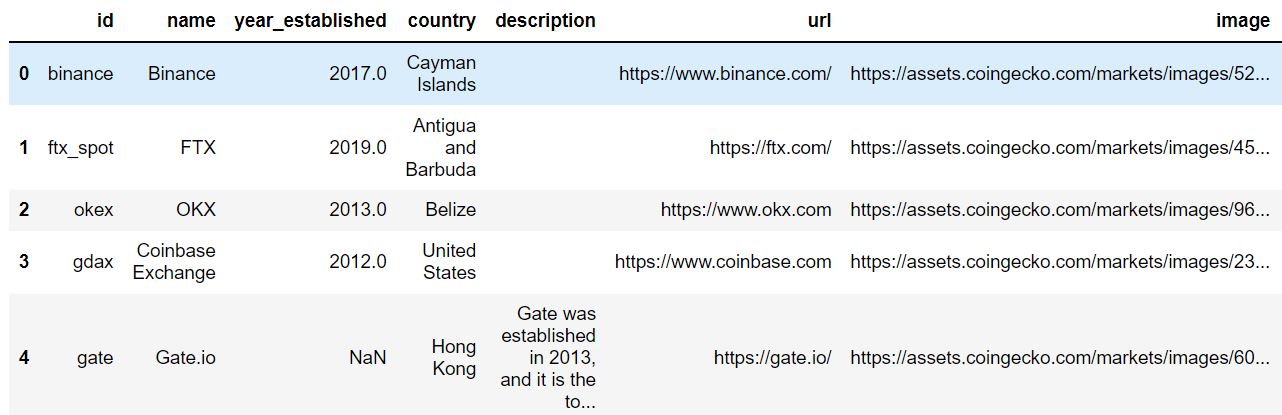
Get Exchange Volume of CoinGecko Top 100 Tickers in BTC
The get_exchanges_by_id() method returns exchange volume in BTC and the top 100 exchange tickers for an exchange. For instance, the following script returns the information for Binance.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
exchanges = cg.get_exchanges_by_id(id = 'binance')
exchanges_df = pd.DataFrame(exchanges['tickers'], columns = ['base','target','volume','trust_score'])
exchanges_df.head()
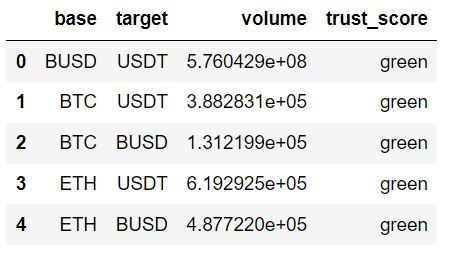
Get Volume Chart Data for an Exchange
Finally, you can retrieve exchange volume chart data for a certain number of previous days from the current date using the get_exchanges_volume_chart_by_id() method.
from pycoingecko import CoinGeckoAPI
import pandas as pd cg = CoinGeckoAPI()
exchanges = cg.get_exchanges_volume_chart_by_id(id = 'binance',
days = 4)
exchanges_df = pd.DataFrame(exchanges, columns =['Time', 'Volume'])
exchanges_df.head()
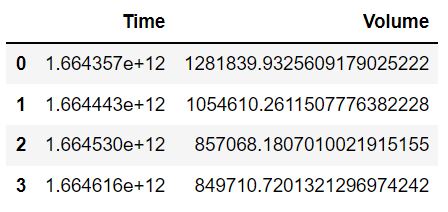
Indexes Endpoint
List All CoinGecko Market Indexes
You can use the get_indexes() method to list market index values using the CoinGecko API. Here is an example of how to do this.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
indexes = cg.get_indexes()
indexes_df = pd.DataFrame(indexes)
indexes_df.head()
Output:
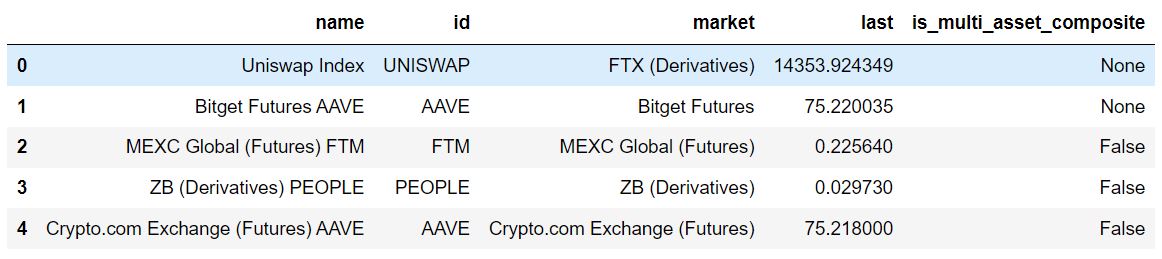
Derivatives Endpoint
A derivative in cryptocurrency is a product whose value is determined by an underlying asset. For example, a Bitcoin derivative relies on and obtains values from the value of Bitcoin. Crypto derivatives seek to adjust elements of risk associated with the volatile nature of cryptocurrencies.
List All CoinGecko Derivative Tickers
The get_derivatives() method returns a list of all derivative tickers. Here is an example:
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
derivatives = cg.get_derivatives()
derivatives_df = pd.DataFrame(derivatives)
derivatives_df.head()
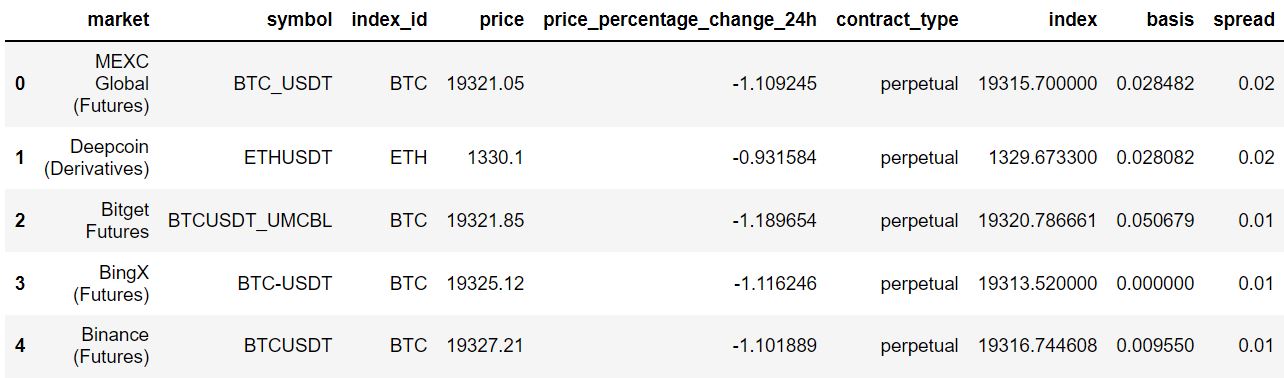
List All Derivate Exchanges
You can list all derivative exchanges using the get_derivative_exchanges() method. You can see the derivative exchange data for various exchanges below.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
derivatives = cg.get_derivatives_exchanges()
derivatives_df = pd.DataFrame(derivatives)
derivatives_df.head()
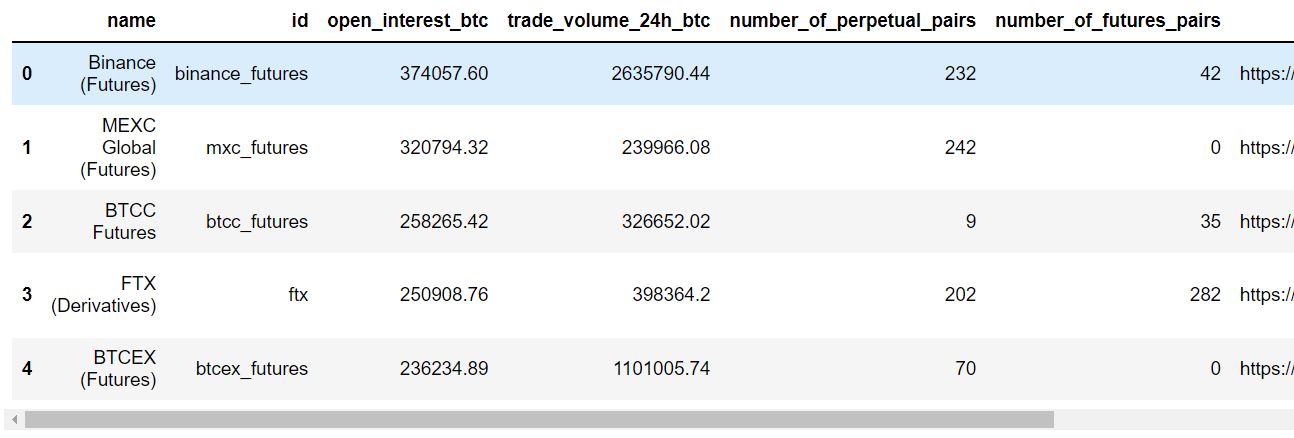
NFTs (Beta) Endpoint
CoinGecko has recently added the NFTs (Non-fungible-tokens) endpoint to its API. NFTs are crypto-assets with unique identification codes on blockchains. They can represent any digital or non-digital asset. NTS include movie or gaming avatars, digital collectibles, old or new tickets, domain names, etc.
List All Supported NFT IDs with CoinGecko
The Python pycoingecko library has not yet implemented a function that can access the NFTs endpoint. Therefore, I will use the Python requests module to access this endpoint. You will get NTFs ids, contract addresses, names, asset platform ids, and symbols in the output.
The following script shows how to get a list of all supported NFTs using the nfts/list API call.
import json
import requests
import os
import pandas as pd
api_url = 'https://api.coingecko.com/api/v3/' request = 'nfts/list'
# make API request
res = requests.get(api_url + request)
# convert to pandas dataframe
nfts_token = pd.read_json(res.text)
nfts_token.head()
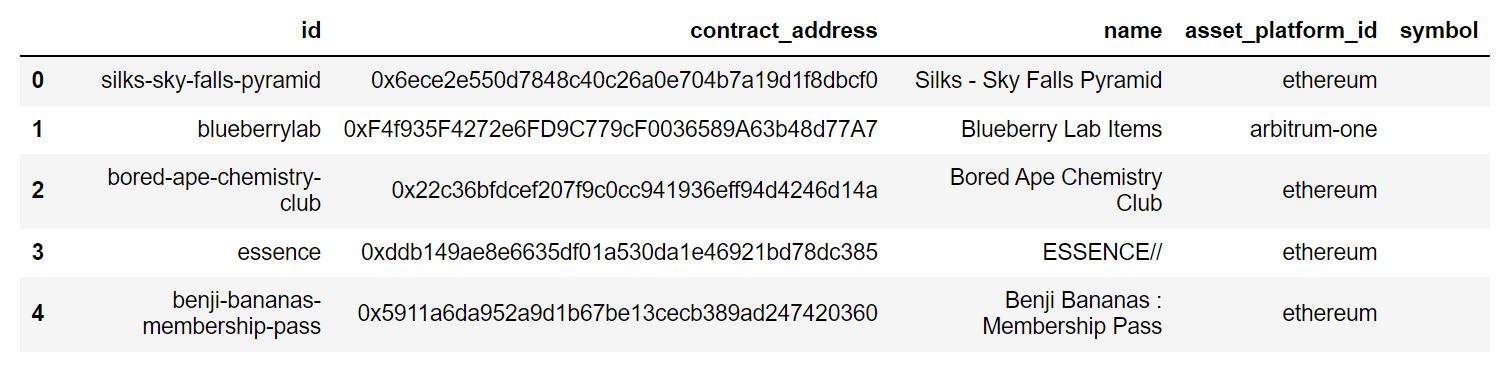
Exchange Rates Endpoint
The exchange rates endpoint contains a function that returns BTC-to-Currency exchange rates.
Get CoinGecko BTC Pair Exchange Rates
The get_exchange_rates() method returns the BTC-to-currency exchange rates.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
exchange_rates = cg.get_exchange_rates()
exchange_rates_df = pd.DataFrame(exchange_rates['rates'])
exchange_rates_df.head()
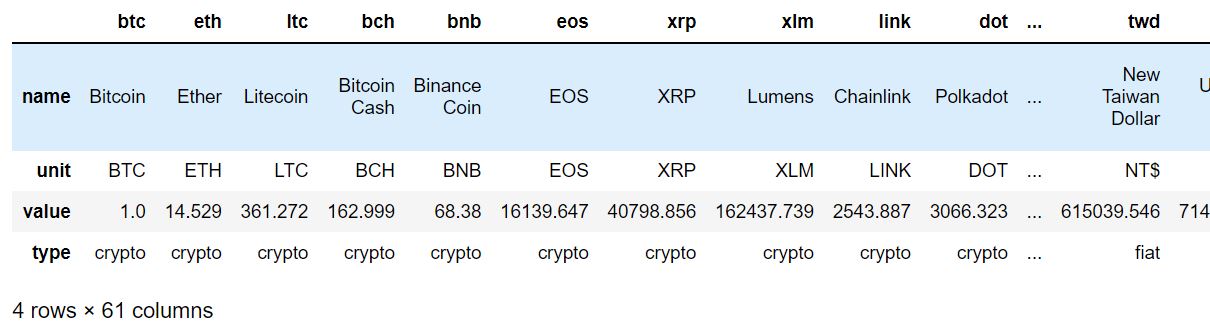
Search Endpoint
The search endpoint allows you to use a string query to search for all the coins, categories, and markets on CoinGecko. If you are unsure about a coin’s exact name, symbol, or id, you can try to search it via the search endpoint. In the output, you will see the names, ids, symbols, etc, of all the coins that match your search criteria.
Search CoinGecko Cryptocurrency Information
You can search for cryptocurrency information using the search() method. You need to pass the search query to the query parameter of the search() method. The following script searches for “Ethereum.”
from pycoingecko import CoinGeckoAPI
import pandas as pd cg = CoinGeckoAPI()
search_results = cg.search(query = "ethereum")
for i in search_results['coins']:
print(i)
In the output below, you can see all the Ethereum coins.
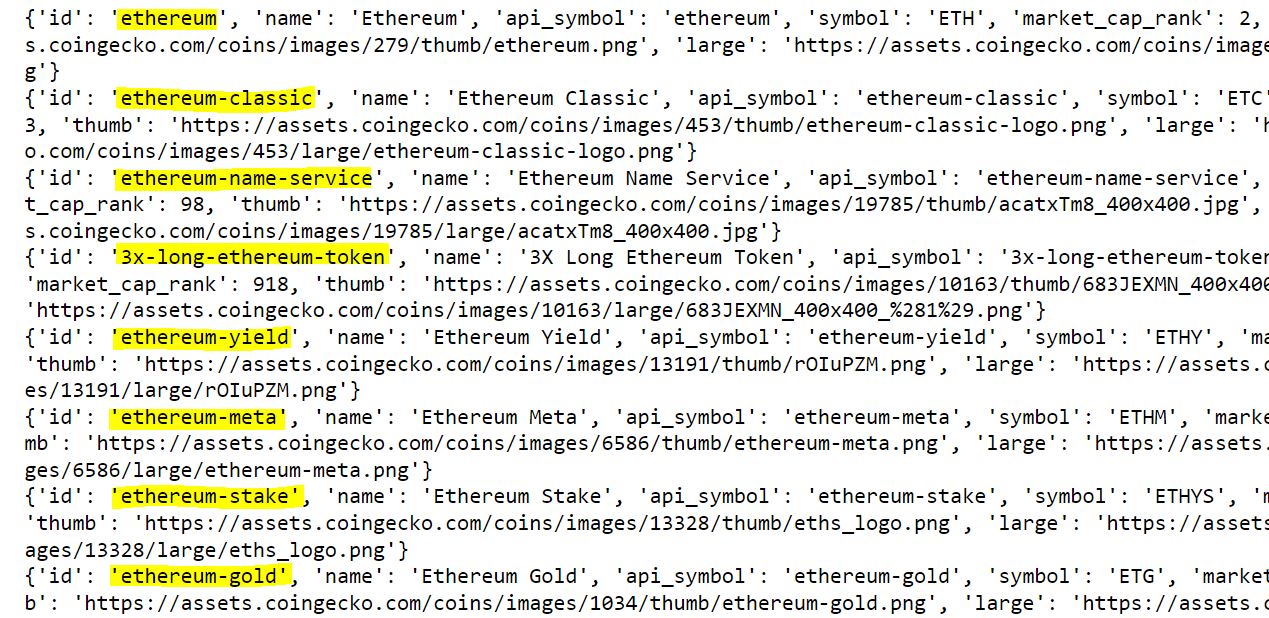
Trending Endpoint
The trending endpoint contains a function that returns the top-7 trending coins on CoinGecko based on people’s searches in the last 24 hours
Search for Trending Coins with CoinGecko API
The get_search_trending() method returns the trending coins on CoinGecko:
from pycoingecko import CoinGeckoAPI
import pandas as pd cg = CoinGeckoAPI()
trending = cg.get_search_trending()
for i in trending['coins']:
print(i)
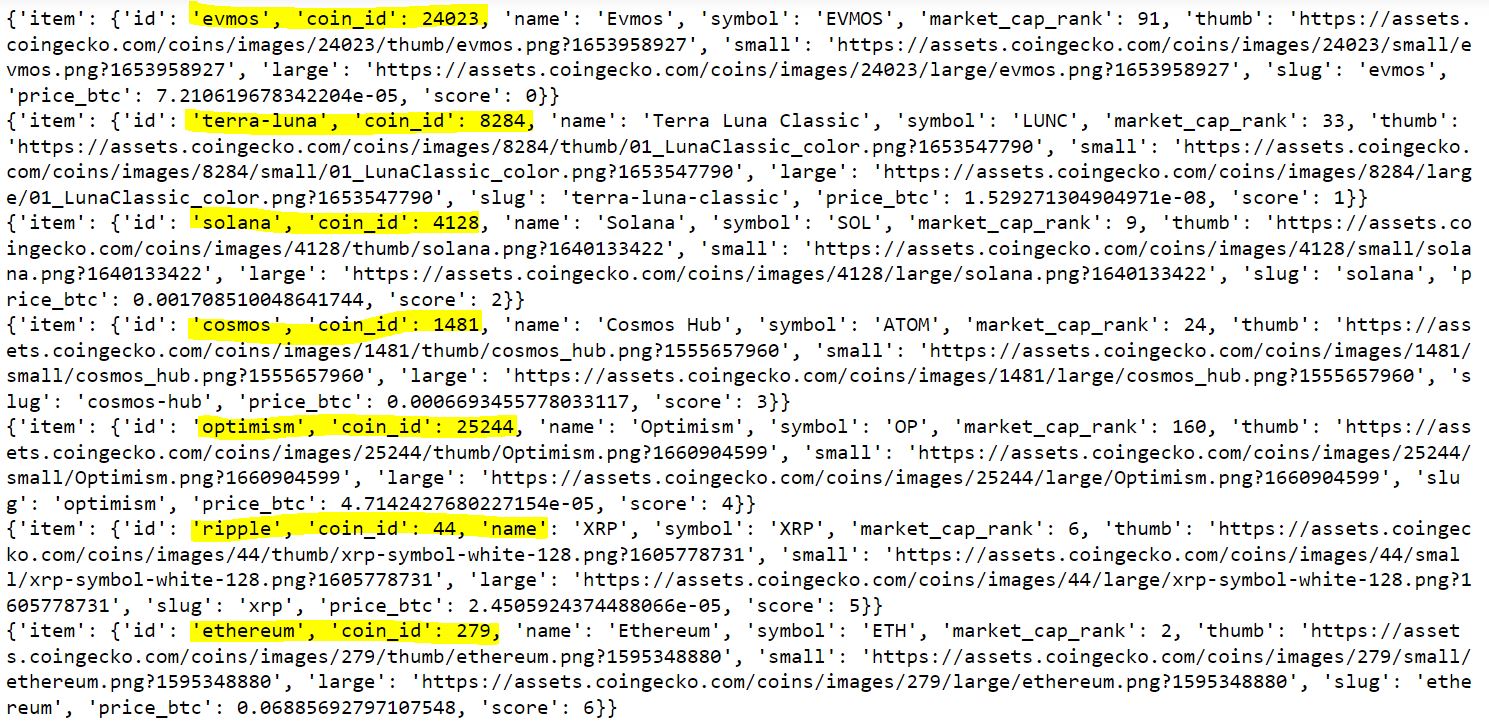
Global Endpoint
The Global endpoint contains functions that return global cryptocurrency information, such as the number of active cryptocurrencies, their market caps, trading volumes, etc.
CoinGecko Get Cryptocurrency Global Data
Here is an example of how to use the get_global() method to retrieve cryptocurrency global data.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
global_data = cg.get_global()
global_data_df = pd.DataFrame(global_data)
global_data_df.head()
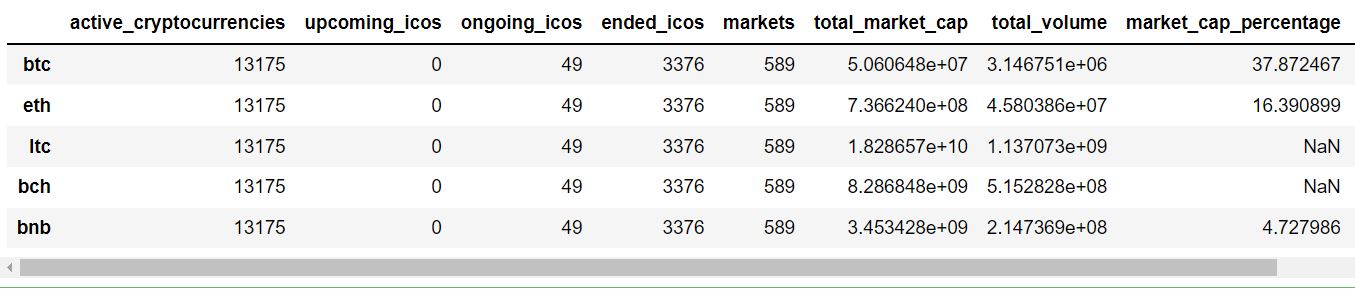
Get CoinGecko DeFi Data
Decentralized finance (DeFi) is an emerging cryptocurrency technology that lets traders trade via peer-to-peer transactions eliminating bank fees.
The get_global_decentralized_finance_defi() method returns cryptocurrency global decentralized finance (data) as shown in the following example. The method returns the global DeFi market cap, trading volume, top DeFi coin name, etc.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
global_data = cg.get_global_decentralized_finance_defi()
global_data

Companies Endpoint
The companies endpoint contains a function that returns public companies’ holdings for Bitcoin or Ethereum.
Get Public Companies Bitcoin or Ethereum Holdings
You can use the get_companies_public_treasury_by_coin_id() method to retrieve public companies’ Bitcoin or Ethereum holdings. For example, the following script returns Ethereum holdings for public companies.
from pycoingecko import CoinGeckoAPI
import pandas as pd
cg = CoinGeckoAPI()
companies_holdings = cg.get_companies_public_treasury_by_coin_id(coin_id = "ethereum")
companies_holdings_df = pd.DataFrame(companies_holdings)
companies_holdings_df.head()

Frequently Asked Questions
Where Does Coingecko Get Its Prices?
CoinGecko calculates crypto prices based on pairings collected from various cryptocurrency exchanges. It then determines the final value using a global volume-weighted average price formula. You can check the CoinGecko methodology for calculating various cryptocurrency metrics for more information.
Is CoinGecko Real-Time?
CoinGecko claims to provide users with the most up-to-date cryptocurrency information. As per CoinGecko support, all the CoinGecko API endpoints are refreshed in around 1 to 2 minutes on average.
What Clients are Available for CoinGecko API?
CoinGecko doesn’t have an official client. However, in addition to Python clients, the following are other unofficial clients for the CoinGecko API.
– NodeJS
– C#
– Java
– R
– Cryptosheets
– WordPress
– Go
– PHP
– Kotlin
Is CoinGecko Trustworthy?
The Trustpilot user reviews for CoinGecko, with an average rating of 1.8/5.0, suggest that most users do not trust CoinGecko. Users report issues such as false or outdated data information and bugs in their mobile applications.
What Clients are Available for CoinGecko API?
CoinGecko doesn’t have an official client. However, in addition to Python clients, the following are other unofficial clients for the CoinGecko API.
- NodeJS
- C#
- Java
- R
- Cryptosheets
- WordPress Plugin
- Go
- PHP
- Kotlin
The Bottom Line
CoinGecko is one of the most widely-used platforms for tracking real-time cryptocurrency information. In addition to a graphical interface, the CoinGecko lets users access their data using a lightweight REST API.
This tutorial explains how to access the CoinGecko REST API in Python using a third-party client library and via the Python requests module. The information provided in this tutorial can help you develop your cryptocurrency tracking application.