Looking to streamline financial data integration in your Python application as an algo-trader or quant analyst? Welcome! In this tutorial, I’ll simplify the Finnhub API for you with practical examples drawn from my extensive Python and Finnhub experience. We’ll go from API basics to creating robust Python applications that revolutionize your financial data analysis.
If you’re interested in using Finnhub as your market data API, check out my detailed Finnhub Review.
Let’s dive in!
What Is Finnhub?
Finnhub is a financial data provider that offers real-time and historical stock data, cryptocurrency data, news, and financial statements for publicly traded companies. The platform provides access to data from various global stock exchanges, including the New York Stock Exchange (NYSE), NASDAQ, London Stock Exchange (LSE), and Tokyo Stock Exchange (TSE), among others.
With the Finnhub API Python plugin, you can seamlessly integrate historical and real-time financial data into your Python applications via REST API calls and WebSockets.
Finnhub, headquartered in New York, USA, was founded in 2018.
Setting Up Finnhub API Python Plugin
To access Finnhub API in Python, register with a Finnhub account and retrieve your free API Key. You can then use the finnhub-python library or the Python requests module to call Finnhub API endpoints.
Signing Up for a Finnhub Account and Retrieving Your API Key
Sign-up with a Finnhub account, and by default, you will have access to all Finnhub API’s free endpoints.
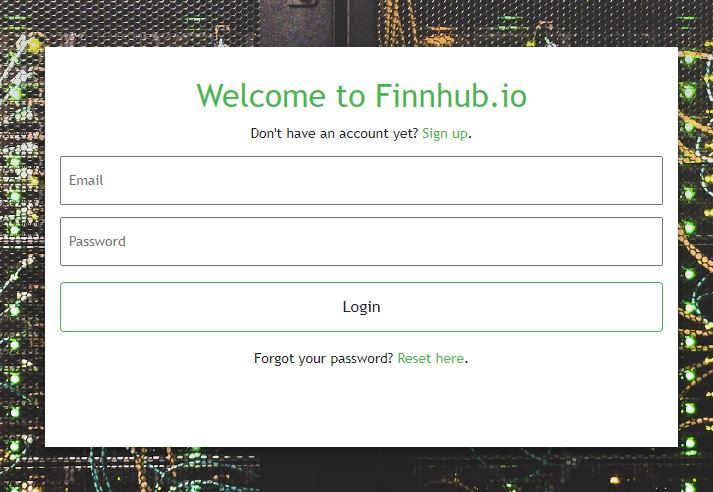
You will see the following dashboard once you log in to your Finnhub account. Copy and store your API key in a safe place. I recommend environment variables for saving your API key.
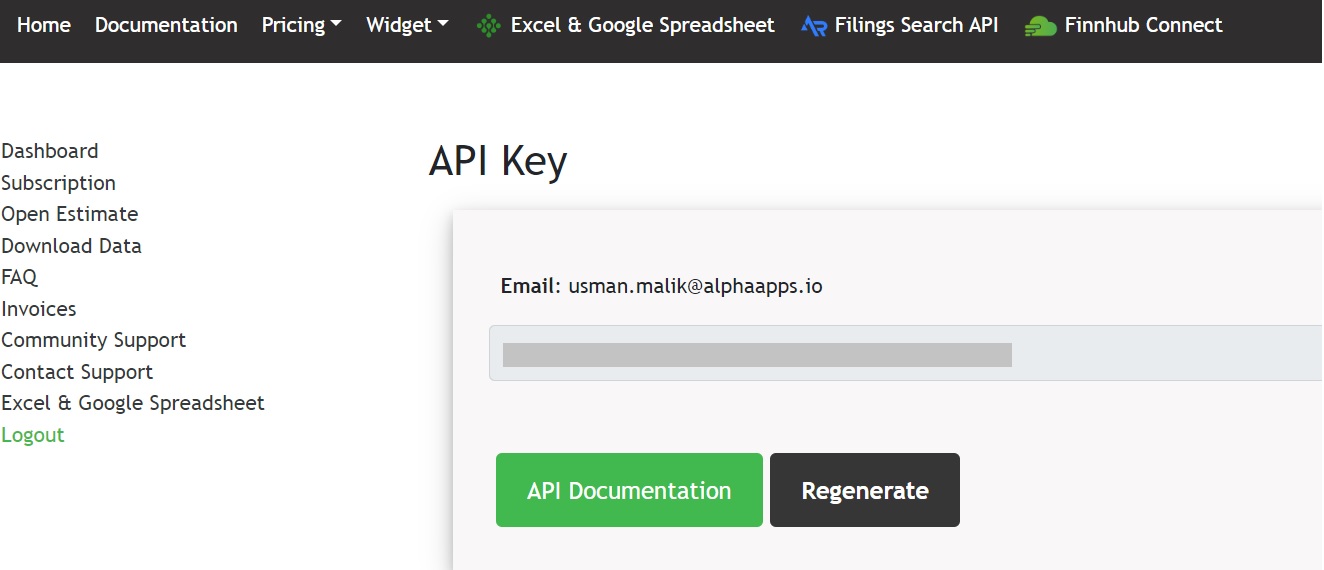
Fetching Data Using Finnhub API in Python
Fetching Data Using finnhub-python Plugin
Finnhub provides an official Python plugin to access Finnhub API endpoints. The plugin provides wrapper functions for REST API calls. The plugin allows easy access to Finnhub. Since the plugin adds a layer of code over the REST API calls, it can be slower than the Python requests module.
You can download the plugin with the following pip command.
pip install finnhub-python
To call Finnhub API via the Python plugin, import the finnhub module, and create an object of the client class. You must pass your Finnhub API key to the api_key attribute of the client class constructor.
Behind the scene, the client class methods call Finnhub REST API functions.
For example, in the following Python code, the client class’s stock_candles() method returns candlesticks data for Microsoft. The stock_candles() method makes an API call to the /stock/candle endpoint URL behind the scenes.
import finnhub
import os
api_key = os.environ['FH_KEY']
finnhub_client = finnhub.Client(api_key = api_key)
symbol = 'MSFT'
interval = 'D' # for daily stock candle values
start_date = 1609455600 # 2021-01-01
end_date = 1612047600 # 2021-01-31
response = finnhub_client.stock_candles('MSFT',
'D',
start_date,
end_date)
print(response)
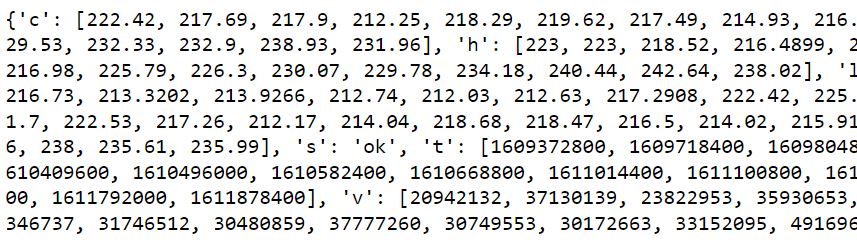
The stock_candles() method returns data in a Python dictionary, which you can convert to a Pandas dataframe using the following script.
import pandas as pd
response_df = pd.DataFrame(response)
response_df.head()
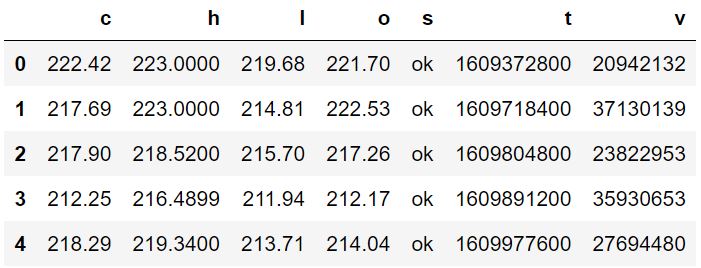
Fetching Data Using Python Requests Module
The Python requests module is a general-purpose module allowing calls to REST API functions. Use the requests module to access Finnhub API endpoints if speed and data latency affect your applications.
You must pass a Finnhub API endpoint URL to the get() method of the requests module.
A Finnhub API URL consists of three parts:
- Base URL: which will always be https://finnhub.io/api/v1.
- Endpoint URL: which changes depending upon the endpoint. For example, the endpoint URL in the following script is: /stock/candle?
- List of attributes, separated by ampersand (&) signs. The attribute name for passing the API key is token.
import requests
base_url = 'https://finnhub.io/api/v1'
endpoint = '/stock/candle?'
symbol = 'MSFT'
resolution = 'D'
start_date = '1609455600'
end_date = '1612047600'
query = 'symbol={}&resolution={}&from={}&to={}&token={}'.format(symbol,
resolution,
start_date,
end_date,
api_key)
response = requests.get(base_url + endpoint + query)
print(response.json())
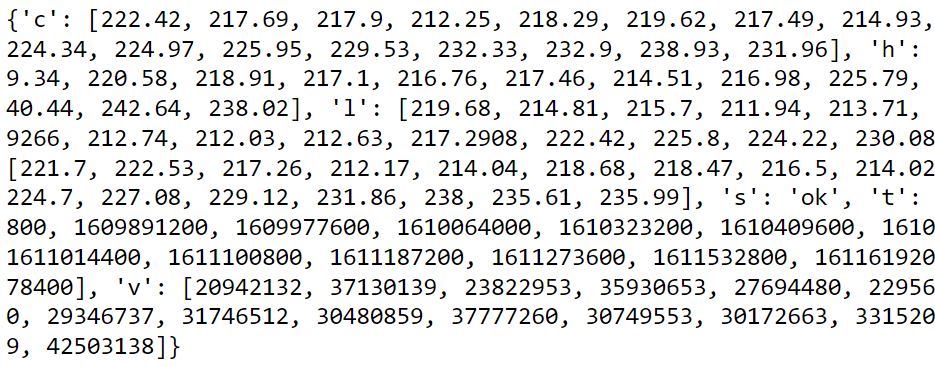
The requests module returns a response object in JSON format, which you can convert to a Pandas dictionary using the json() method. Using the following script, you can convert a Python dictionary into a Pandas Dataframe.
response_df = pd.DataFrame.from_records(response.json())
response_df.head()
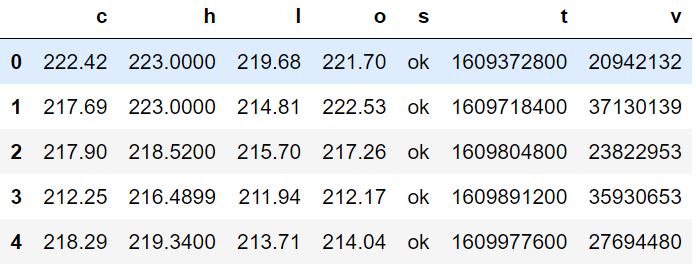
Finnhub API Endpoints
Per the official documentation, here is a list of Finnhub API endpoints. Unfortunately, most functions in each endpoint are only accessible with a paid subscription. In the following sections, you will see examples of retrieving data from free endpoints.
- Stock Price
- Stock Fundamentals
- Stock Estimates
- ETFS & Indices
- Forex
- Crypto
- Technical Analysis
- Alternative Data
- Economic
- Global Filings Search
- Mutual Funds
- Bonds
- WebSocket
Stock Price
The stock price endpoint allows retrieving quotes, candles, historical data, dividend information, etc., for a stock.
Quote
The quote() method retrieves real-time stock prices for US stocks. Only enterprise accounts allow access to real-time stock prices for international markets.
For example, the following script returns the latest stock prices for Microsoft.
response = finnhub_client.quote('MSFT')
response
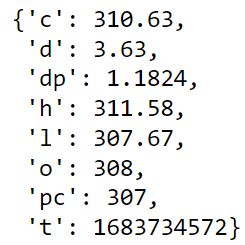
If you consistently want real-time stock prices, I recommend using WebSockets instead of the quote(). I will explain how to do that in the section on WebSockets.
Candles Data
You can retrieve candlesticks data for a stock using the stock_candles() method. You have already seen this method in action in a previous section. The stock_candles() method returns OHLCV data for a stock, along with the time and request status information. Supported interval values are 1, 5, 15, 30, 60, D, W, M.
While working with this method data; I noticed that you need to pass the start and end time for the candle data in the UNIX format. It is almost impossible to remember the UNIX format for a specific date.
I wrote a method date_to_unix() which converts the ISO date format to UNIX. With this method, you can convert the start and end dates from ISO to UNIX formats and retrieve the corresponding candlestick data.
import datetime
def date_to_unix(date_string):
date_obj = datetime.datetime.fromisoformat(date_string)
unix_timestamp = int(date_obj.timestamp())
return unix_timestamp
symbol = 'MSFT'
interval = 'D' # for daily stock candle values
start_date = date_to_unix("2021-01-01")
end_date = date_to_unix("2021-01-31")
response = finnhub_client.stock_candles('MSFT',
'D',
start_date,
end_date)
The response from the stock_candles() method contains the time information in UNIX format. You can convert that information back to ISO format using the following script.
def date_to_iso(response):
t = int(response['t'])
date_obj = datetime.datetime.fromtimestamp(t)
date_string = date_obj.isoformat()
return date_string
response_df = pd.DataFrame.from_records(response)
response_df['t'] = response_df.apply(date_to_iso, axis=1)
response_df.head()
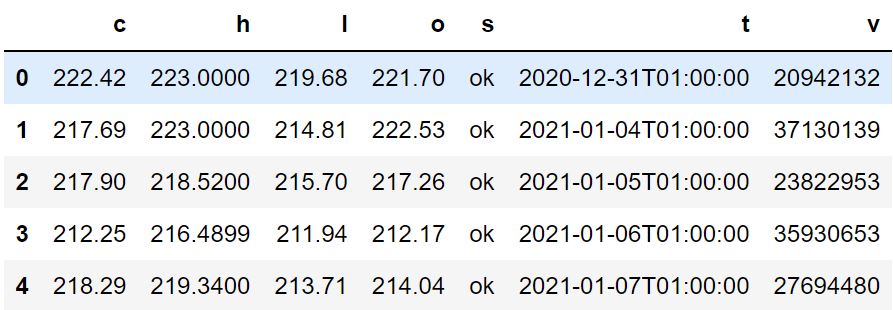
To plot stock candlesticks data, I recommend converting the date column to date format and setting it as the index column of the dataframe.
response_df['t'] = pd.to_datetime(response_df['t'])
response_df.set_index('t', inplace=True)
response_df.head()
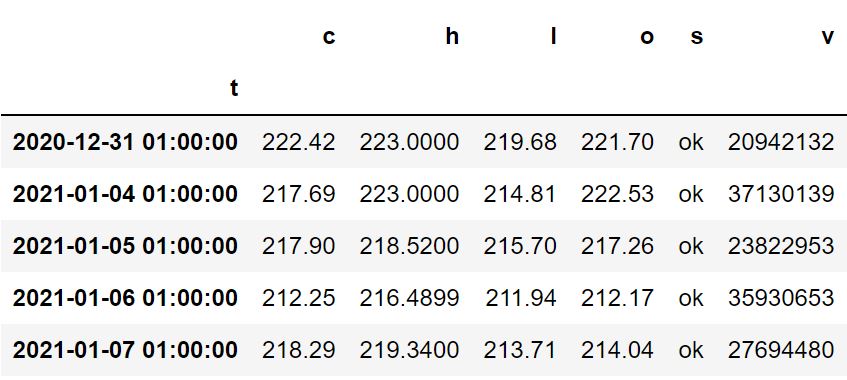
You can then plot a line plot using the plot() method of the Pandas dataframe.
import matplotlib.pyplot as plt
import seaborn as sns
sns.set_style("darkgrid")
sns.set_context("notebook")
response_df['c'].plot(figsize=(8,6))
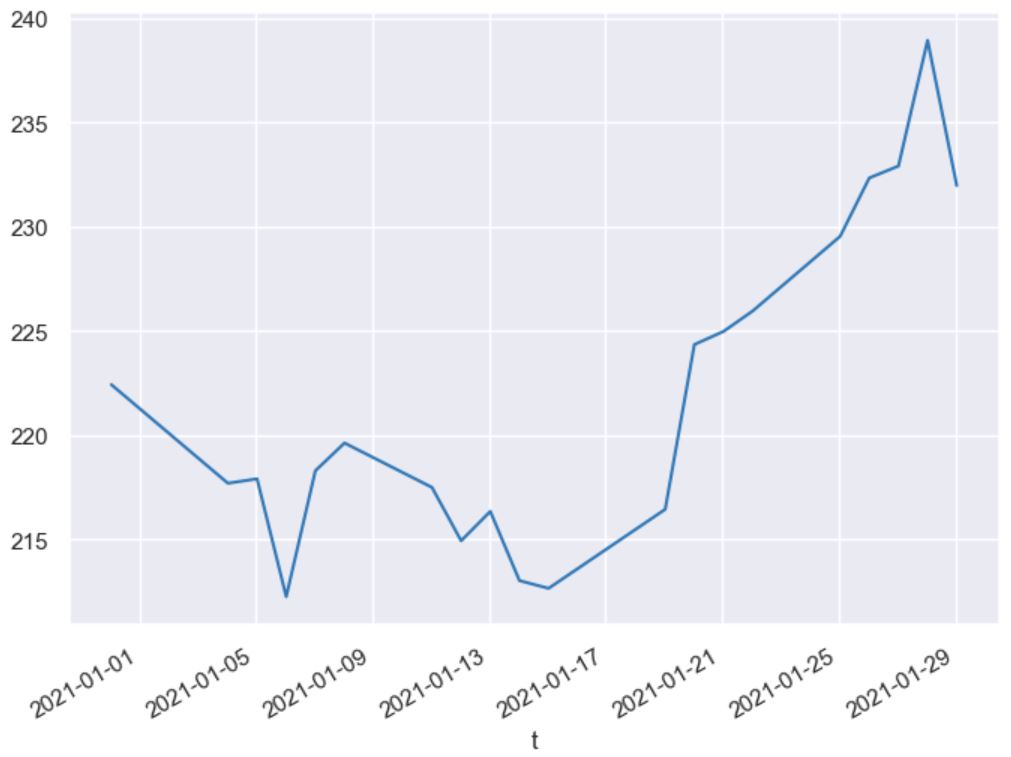
Stock Fundamentals
Stock fundamentals endpoint allows you to search for stock symbols, get company profiles and news, basic financial information, insider statements, etc., for a stock.
Symbol Lookup
You can search for a symbol, security, ISIN, and chip information using the symbol_lookup() method, which returns the best-matching symbols based on your input query.
response = finnhub_client.symbol_lookup('micros')
response_df = pd.DataFrame.from_records(response['result'])
print(response_df.shape)
response_df.head()
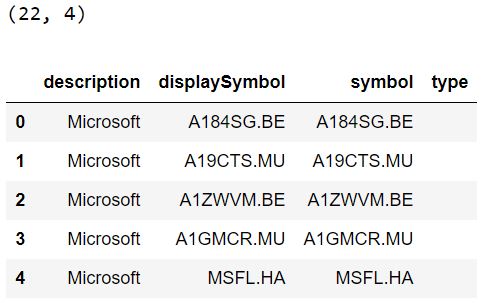
Stock Symbol
The stock_symbols() method returns a list of stocks from one or a set of exchanges. You must pass one of the supported exchange codes to the stock_symbols() method.
finnhub_client.stock_symbols('US')
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
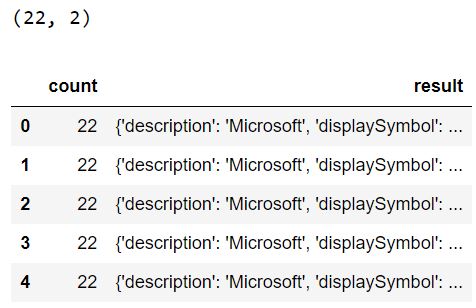
Company Profile
The company_profile2() method returns a company’s basic information e.g., country, currency, exchange(s) where the company operates, etc.
response = finnhub_client.company_profile2(symbol = 'MSFT')
response
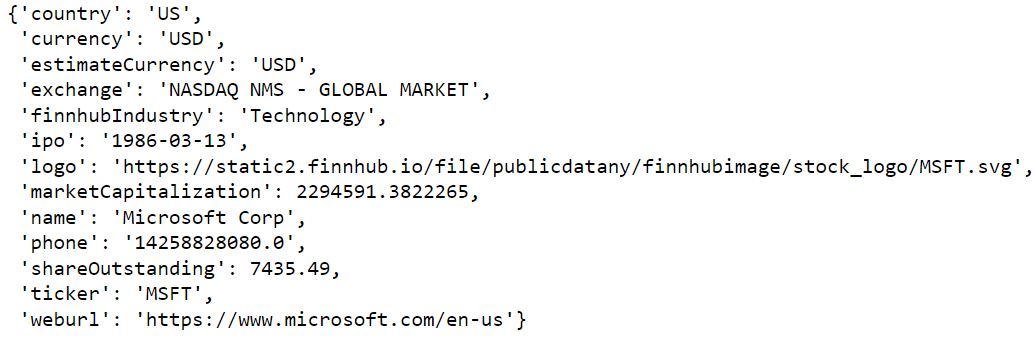
Market News
The general_news() method returns latest market news about stock market. You must pass the news type to the general_news() method. The acceptable news values are general, forex, crypto, and merger.
response = finnhub_client.general_news('general')
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
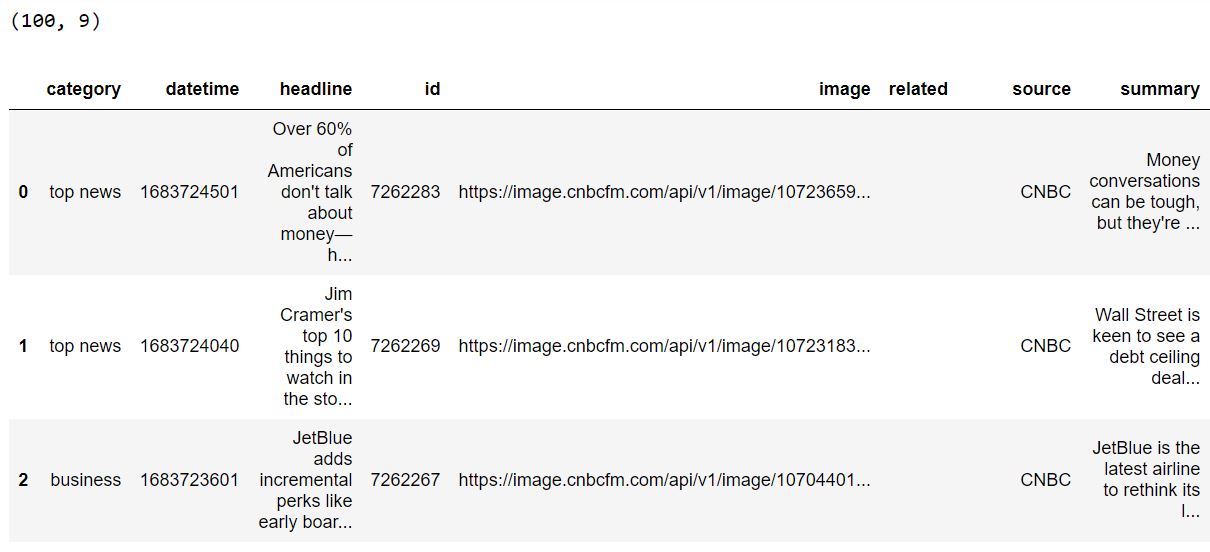
Company News
The company_news() method returns the latest news about a particular company. You must pass the company symbol and optional start and end dates to filter the news. This endpoint only returns information about North American companies.
response = finnhub_client.company_news('MSFT', _from="2022-01-01", to="2022-12-31")
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
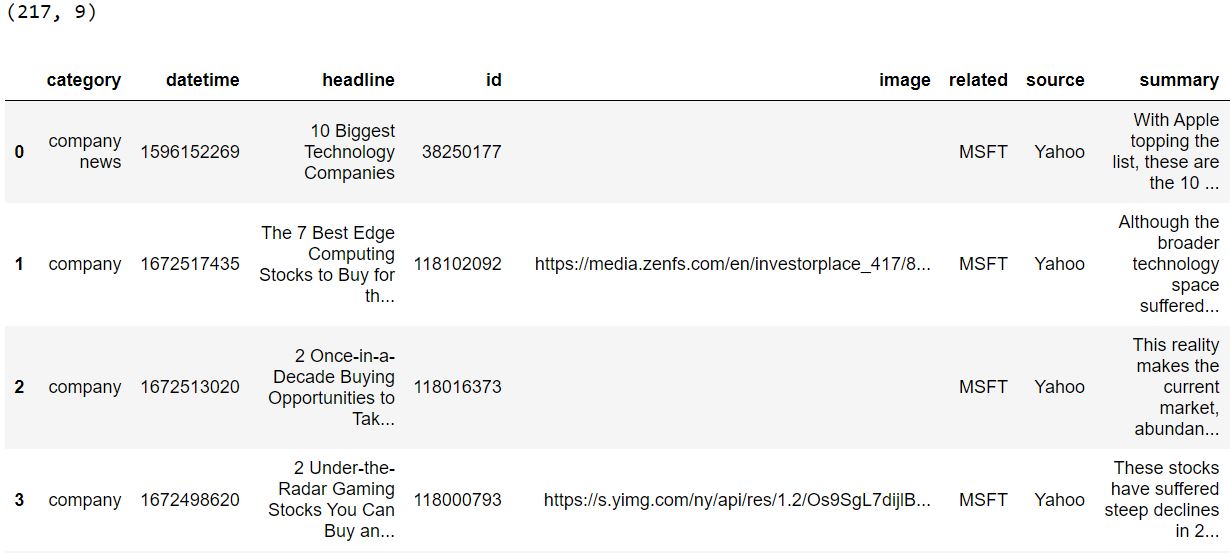
Basic Financials
You can retrieve all basic financial information about a company using the company_basic_financials().
response = finnhub_client.company_basic_financials('MSFT', 'all')
response
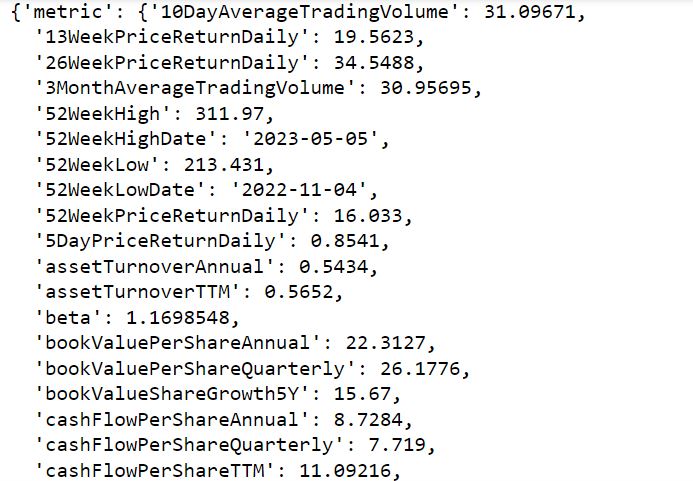
Insider Sentiment
The stock_insider_sentiment() method returns insider sentiment information for US companies using approaches discussed in this blog.
The MSPR values in the output convey sentiment information, ranging from -100 to 100, with -100 being the most negative and 100 being the most positive.
response = finnhub_client.stock_insider_sentiment('MSFT', '2022-01-01', '2022-12-31')
response_df = pd.DataFrame.from_records(response['data'])
print(response_df.shape)
response_df.head()
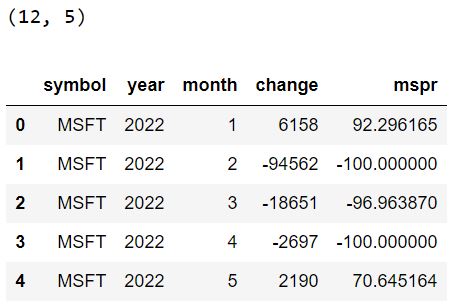
Stock Estimates
The stock estimates endpoint functions return future speculations and estimates for a company, e.g., analyst recommendations, price targets, earnings surprises, etc.
Recommendation Trends
The recommendation_trends() returns analyst recommendations. The method returns the number of recommendations that fall into the buy, hold, strong buy, and strong sell categories.
response = finnhub_client.recommendation_trends('MSFT')
response_df = pd.DataFrame.from_records(response)
response_df.head()
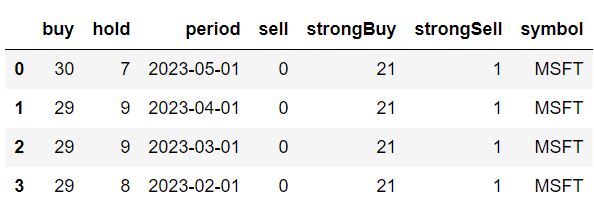
Earnings Surprises
The company_earnings() method returns estimated quarterly earnings surprises for a company. Earnings surprise is the difference between actual and estimated future earnings.
response = finnhub_client.company_earnings('MSFT', limit=10)
response_df = pd.DataFrame.from_records(response)
response_df.head()
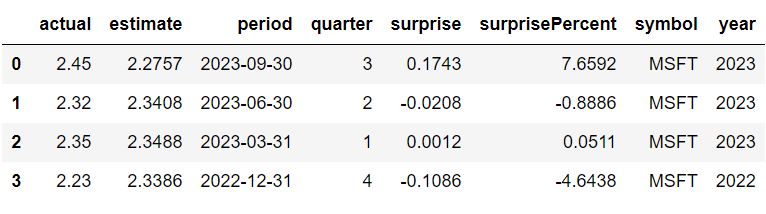
ETFS & Indices
This endpoint contains function returning indices constituents, Exchange Traded Funds (ETFs) profiles, holdings, sectors, and countries, data.
Indices Constituents
The indices_const() method is the only free method in this endpoint. This method returns a list of stocks for an index. You must pass one of the Finnhub-supported indices to this method.
response = finnhub_client.indices_const(symbol = "^OEX")
response
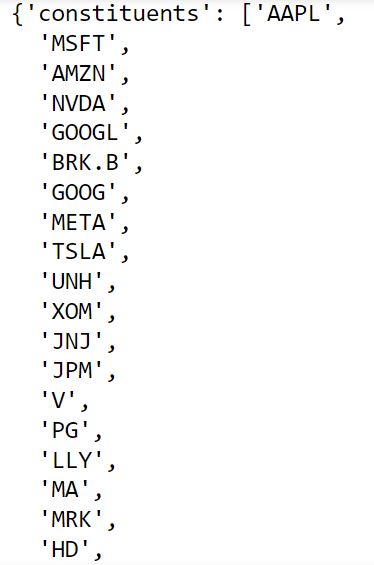
Forex
The forex endpoint returns the list of supported forex exchanges, symbols, and candlesticks data.
Forex Exchanges List
The forex_exchanges() method returns a list of all supported forex exchanges.
finnhub_client.forex_exchanges()

Forex Symbols List
You can retrieve a list of all forex symbols within a forex exchange using the forex_symbols() method. The method accepts forex exchange as a parameter value.
response = finnhub_client.forex_symbols('forex.com')
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
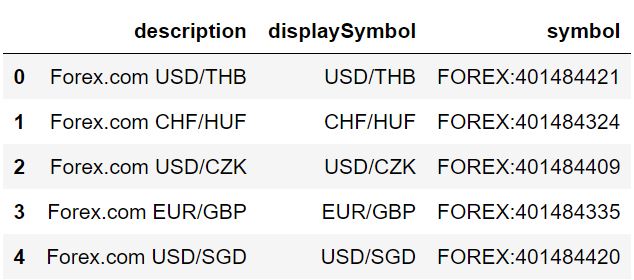
Crypto
The crypto endpoint returns lists of cryptocurrency exchanges, symbols, and cryptocurrency candlestick data.
Crypto Exchanges List
The crypto_exchanges() method returns a list of all supported cryptocurrency exchanges.
finnhub_client.crypto_exchanges()
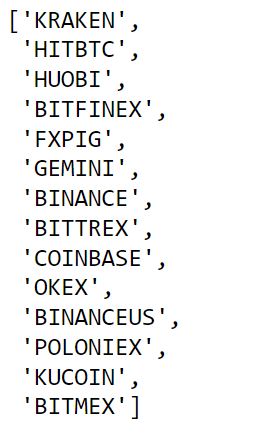
Crypto Symbols List
The crypto_symbols() method returns a list of all cryptocurrency symbols within a forex exchange. The method accepts the cryptocurrency exchange name as a parameter value.
response = finnhub_client.crypto_symbols('COINBASE')
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
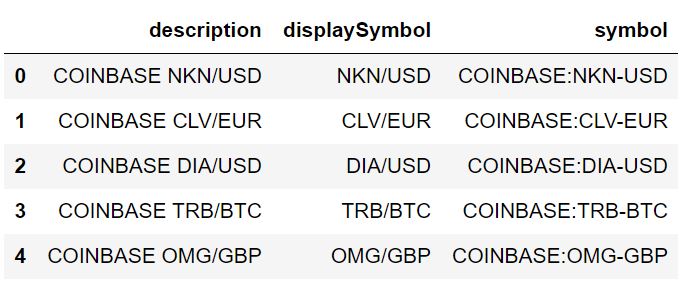
Technical Analysis:
This endpoint contains functions that return various technical stock indicators and allow you to perform technical analysis on a stock, such as pattern recognition and support/resistance calculations.
Technical Indicators
The technical_indicator() function is the only free function in this endpoint. The function returns various technical indicators for a stock.
start_date = 1609455600 # 2021-01-01
end_date = 1612047600 # 2021-01-31
symbol = "MSFT"
resolution = "D"
indicator = "sma" # simple moving average
indicator_fields = {"timeperiod": 5}
response = finnhub_client.technical_indicator(symbol = symbol,
resolution = resolution,
_from = start_date,
to = end_date,
indicator = indicator,
indicator_fields = indicator_fields)
response_df = pd.DataFrame.from_records(response)
response_df['t'] = response_df.apply(date_to_iso, axis=1)
response_df.head()
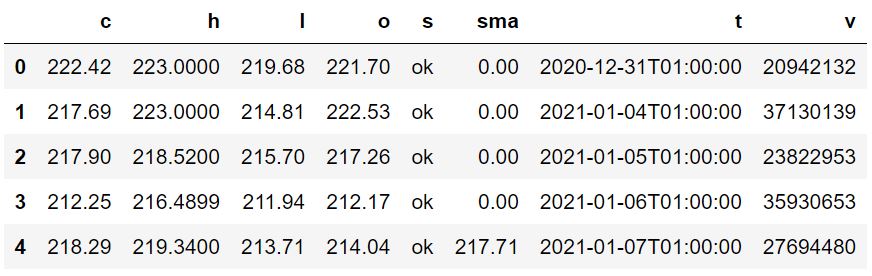
Alternative Data
The alternative data endpoint returns information such as stock social sentiments, USA spending, senate lobbying, and other miscellaneous information.
Social Sentiment
The stock_social_sentiment() returns social sentiments about stocks from Reddit and Twitter.
response = finnhub_client.stock_social_sentiment('MSFT')
response_df = pd.DataFrame.from_records(response['reddit'])
print(response_df.shape)
response_df.head()
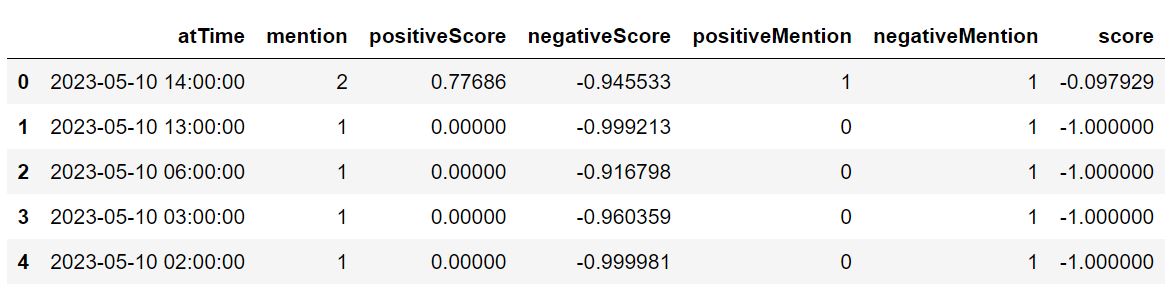
USA Spending
The stock_usa_spending() method returns a list of US government spending activities from the USASpending dataset. This information allows you to identify companies winning big government contracts.
response = finnhub_client.stock_usa_spending("MSFT", "2022-01-01", "2022-06-15")
response_df = pd.DataFrame.from_records(response['data'])
print(response_df.shape)
response_df.head()
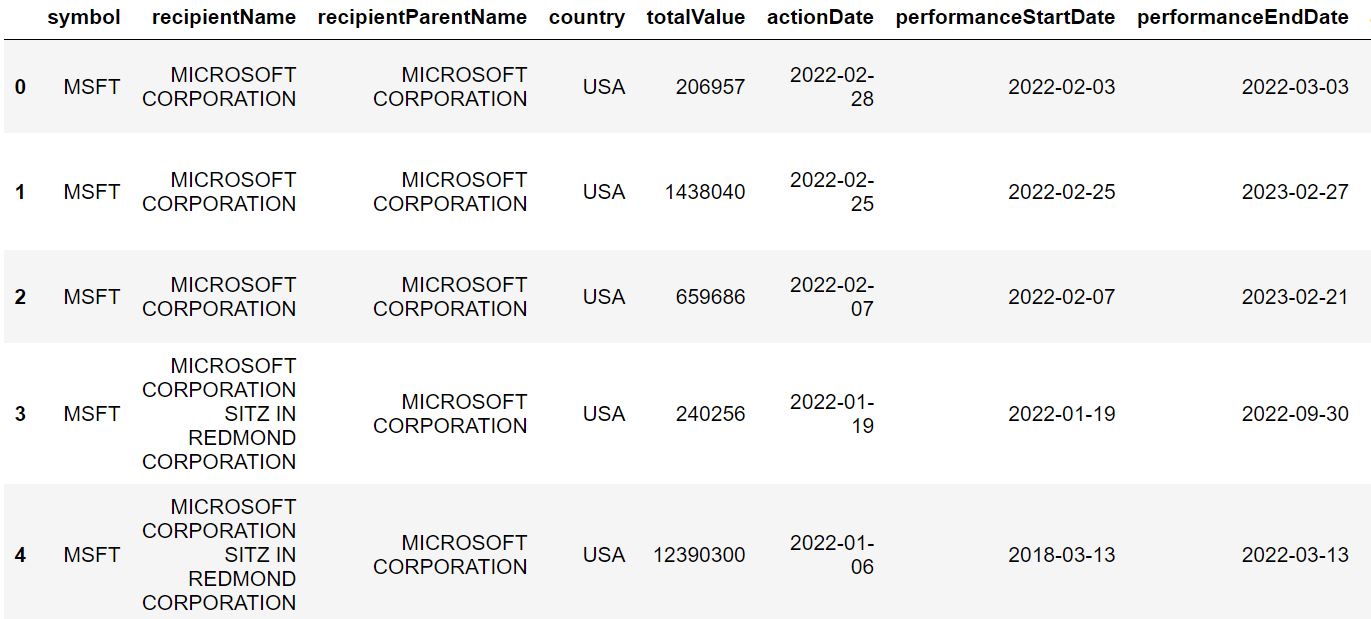
COVID-19
The covid19() method returns real-time updates on Covid-19 for all US states.
response = finnhub_client.covid19()
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
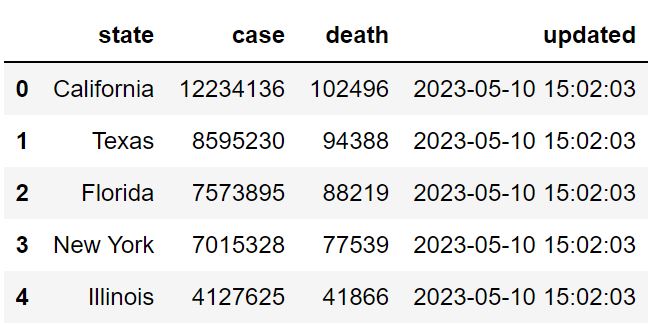
Economic
This endpoint returns country information and associated metadata, economic calendar, codes, and other miscellaneous economic data.
Country List
Only the country() method is free in this endpoint, which returns countries’ information and metadata.
response = finnhub_client.country()
response_df = pd.DataFrame.from_records(response)
print(response_df.shape)
response_df.head()
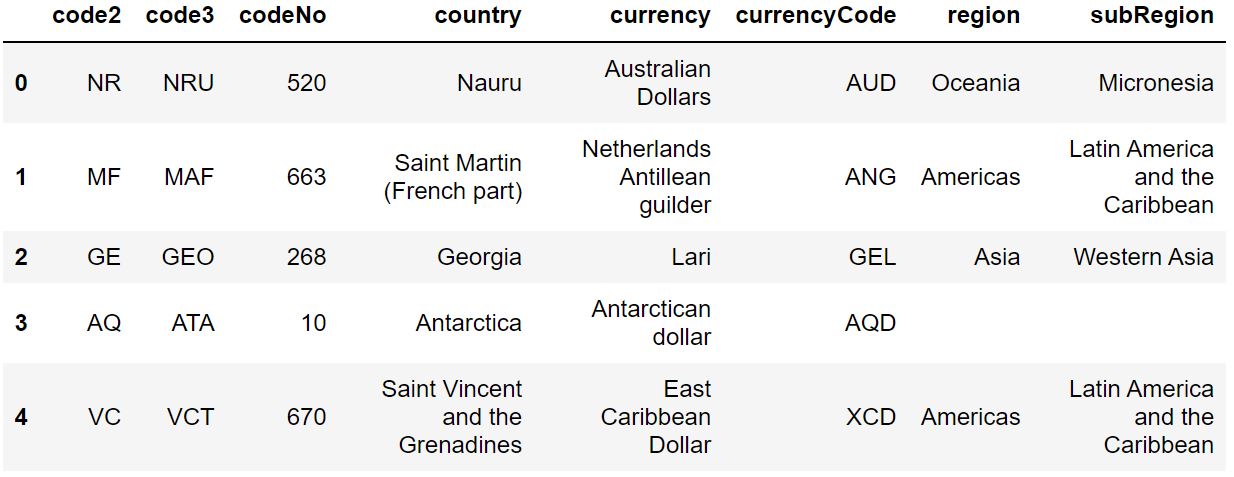
Mutual Funds and Bonds
These endpoints returns various information about mutual funds and bond. These endpoints have no free methods.
WebSockets
I could not find a method in the finnhub-python library that implements Finnhub API WebSockets. You can use the Python websocket-client module to retrieve streaming data from Finnhub API. You can install the websocket-client library using the following pip command.
pip install websocket-client
Trades
The free plan allows you to retrieve real-time trades for only US stocks, forex, and crypto.
To implement WebSockets, you must import the websocket module and create an object of the WebSocketApp class.
Next, you need to define the methods for the on_open callback of the WebSocketApp class. This method executes as soon as the WebSocket opens. Inside this method, you must call the send() method and pass it the symbols that you want to subscribe to for real-time data.
You also need to define a method for the on_message callback, which is called whenever you receive a response from WebSocket. Inside this method, you can define the logic to process the response.
I print six response messages in the following script before closing the WebSocket.
import websocket
import json
counter = 0
def on_message(ws, message):
global counter
data = json.loads(message)
print(data)
counter += 1
print(" ============== Total messages received ================", counter)
if counter == 10:
ws.close()
def on_error(ws, error):
print(error)
def on_close(ws):
print("### closed ###")
def on_open(ws):
ws.send('{"type":"subscribe","symbol":"MSFT"}')
base_url = 'wss://ws.finnhub.io?'
query = 'token={}'.format(api_key)
if __name__ == "__main__":
websocket.enableTrace(True)
ws = websocket.WebSocketApp(base_url + query,
on_message = on_message,
on_error = on_error,
on_close = on_close)
ws.on_open = on_open
ws.run_forever()
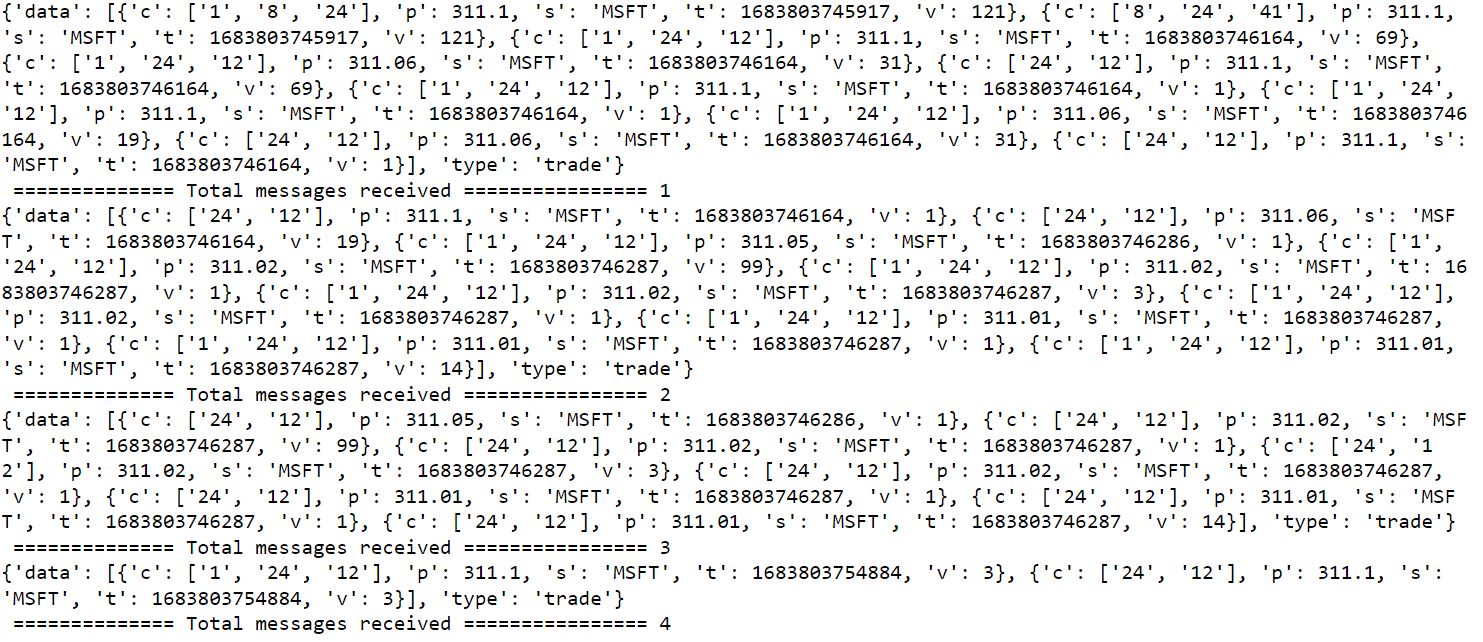
Finnhub Plugins
Besides Python, Finnhub supports official plugins for the following languages:
Finnhub Alternatives
Following are some alternative market data APIs:
The Bottom Line
Congratulations! You have completed the tutorial and gained a comprehensive understanding of integrating Financial Data into your Python applications using the Finnhub API. Armed with this knowledge, you can now develop robust models and tools to stay ahead of the game. With the Finnhub API, you have a powerful tool that can help you gain insights into the financial world. So why delay? Take the first step today, and start exploring the world of financial data to discover new opportunities and gain a competitive edge in the market.