Embark on a thrilling voyage to master the IEX Cloud data using Python! Leveraging the PyEX and Python requests libraries, this guide navigates the intricacies of API endpoints while avoiding common pitfalls associated with third-party Python clients.
Whether you’re a trader dissecting financial trends or a Python developer innovating solutions, you’ll emerge with a robust command over the IEX Cloud API, revolutionizing your data-driven projects. Get ready for a transformative journey through the vast seas of IEX Cloud data.
If you’re trying to decide if IEX Cloud is right for you, check out my exhaustive IEX Cloud Review.
What Is IEX Cloud?
IEX (Investors Exchange) cloud is an online financial data provider platform connecting developers and financial data creators. IEX Cloud allows users to retrieve live and historical financial data such as stock prices, forex exchange rates, cryptocurrency prices, US and international exchange data, the latest financial news, etc.
IEX Cloud was founded in 2012 with Headquarters in New York, United States
What Is the IEX Cloud API?
IEX Cloud API is a REST API that allows developers to fetch data from IEX Cloud in the programming language of their choice. With IEX Cloud API, you can develop financial applications and trading bots, automating your trading activities. You can access IEX Cloud API in Python via the Python requests library or any third-party tool such as PyEX, IexFinance, etc.
IEX Cloud Pros and Cons
Pros
- 30-day free trial period for product evaluation.
- Has an easy-to-use API with client libraries in multiple languages.
Cons
- Streaming data is only available with a paid subscription.
- The confusing pricing scheme for accessing different endpoints.
- Some users report that the API documentation needs to include important information or be clearer to understand.
IEX Cloud Pricing Plans
The endpoints you can access using the IEX Cloud API depend upon your IEX cloud subscription plan.
Based on the features and the amount of data you want to access, IEX Cloud groups its pricing plans into three tiers:
- Launch – $49/Month.
- Grow – $599/Month.
- Scale – $3200/Month.
Setting Up IEX Cloud
To access the IEX Cloud API, you need to sign-up with IEX Cloud and get your API key.
Creating an Account
Go to the IEX Cloud sign-up page and fill out the account creation form. Click the “Create Account” button.
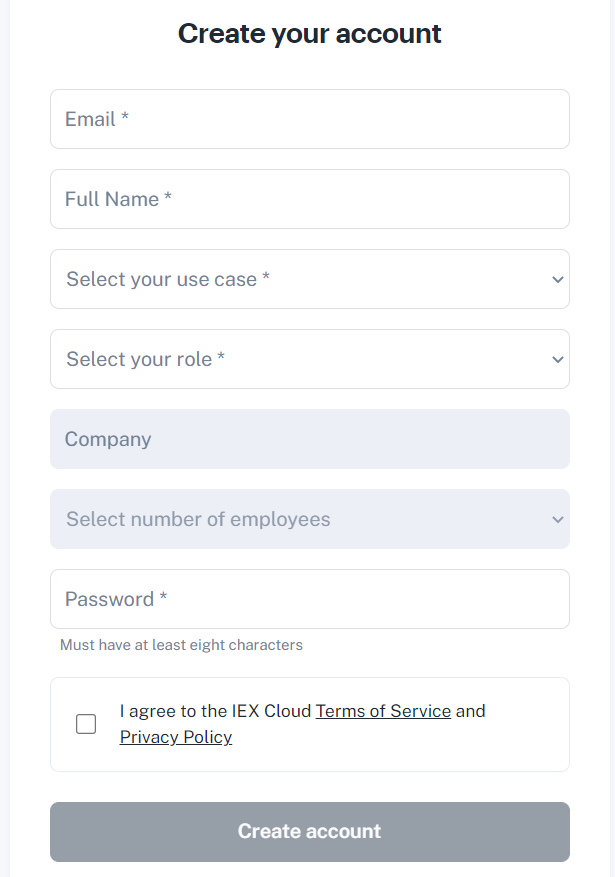
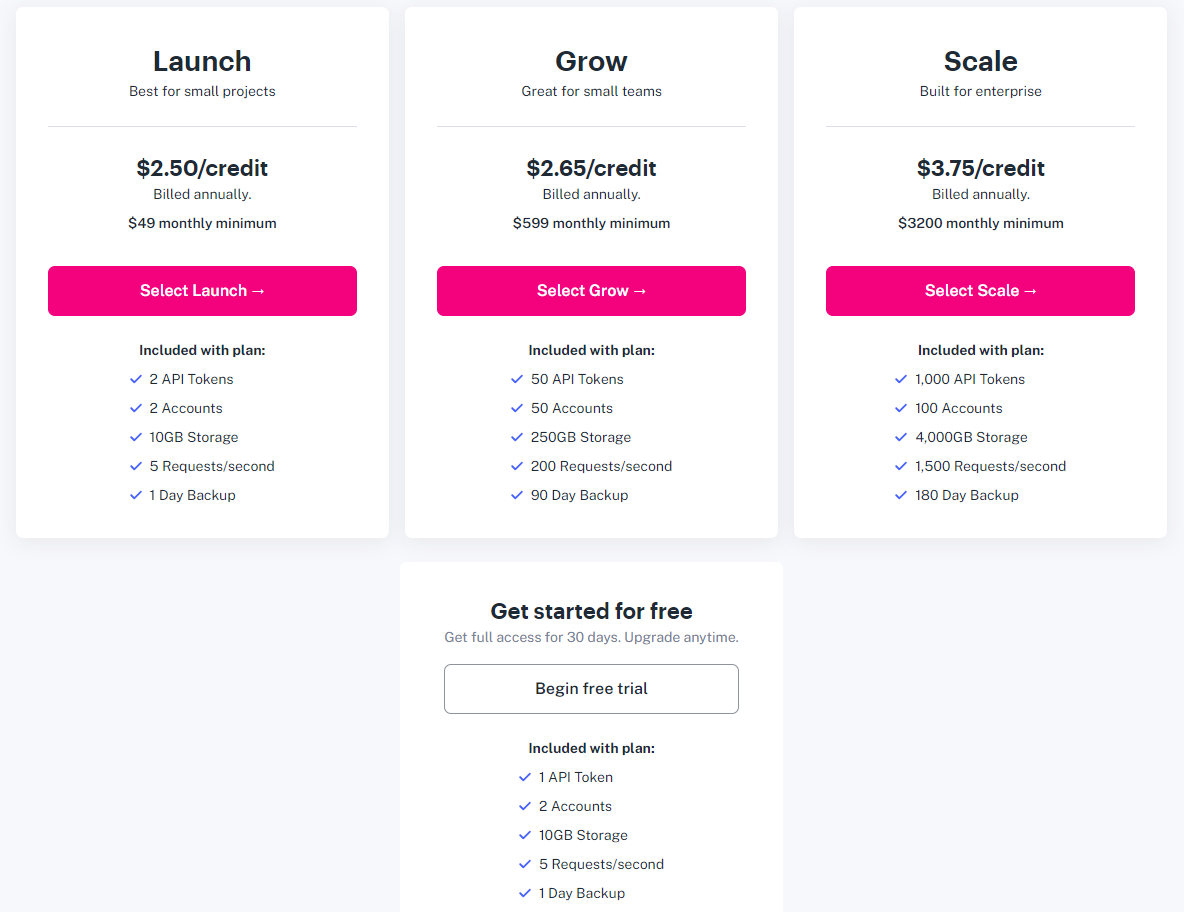
You can select any of the following paid plans. Sign up for a free trial to evaluate the product and see if it suits your needs.
Obtaining an API Key
Login to your IEX Cloud account and click the key icon from the left sidebar. You will see all your API tokens. The free tier allows only a single API token.
Click the “Reveal secret token” button to see your API secret, which is your API key. Save it at a safe place. I use environment variables to save my API keys.
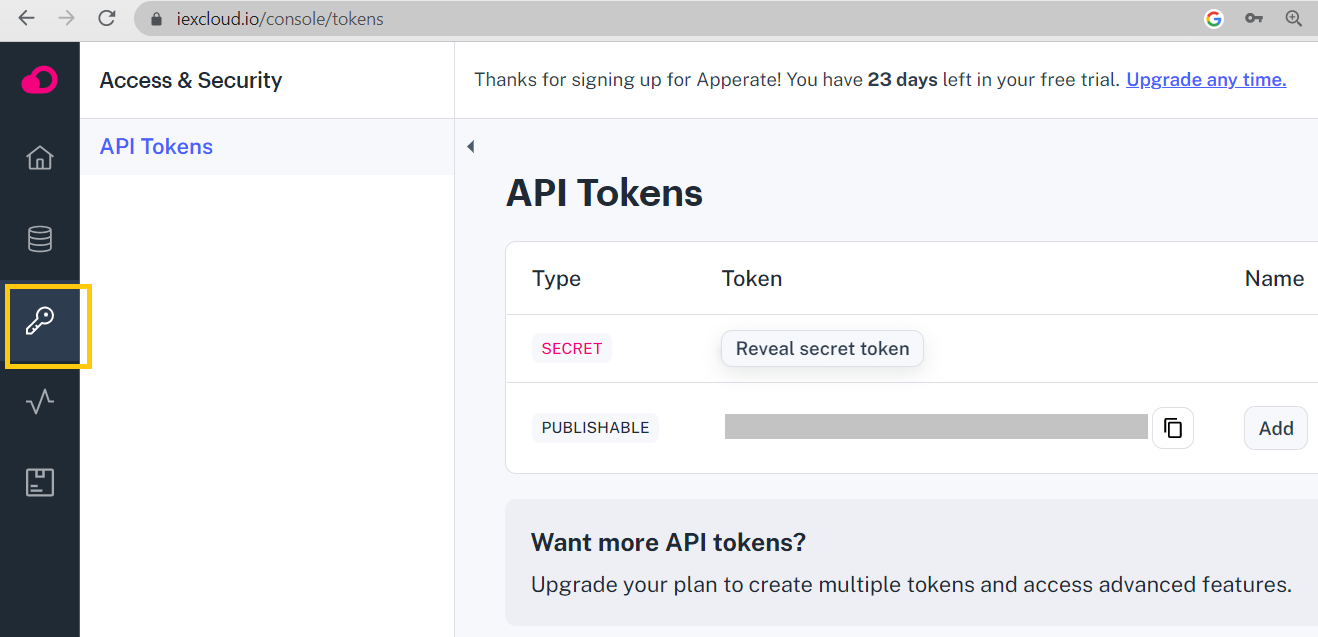
Fetching Data Using IEX Cloud API in Python
For convenience and ease of use, I recommend using PyEx library. Use the requests library if you can’t find a function for the IEX Cloud API in the PyEx library or if speed is your primary concern.
IEX Cloud PyEX Library Example
There are three steps to access an IEX Cloud API endpoint via the PyEX library:
- Install PyEx library
- Import the pyEX module and connect to the IEX Cloud API via the Client class
- Call IEX Cloud API endpoints via Client class methods
Installing the Library
The following command installs the PyEx library.
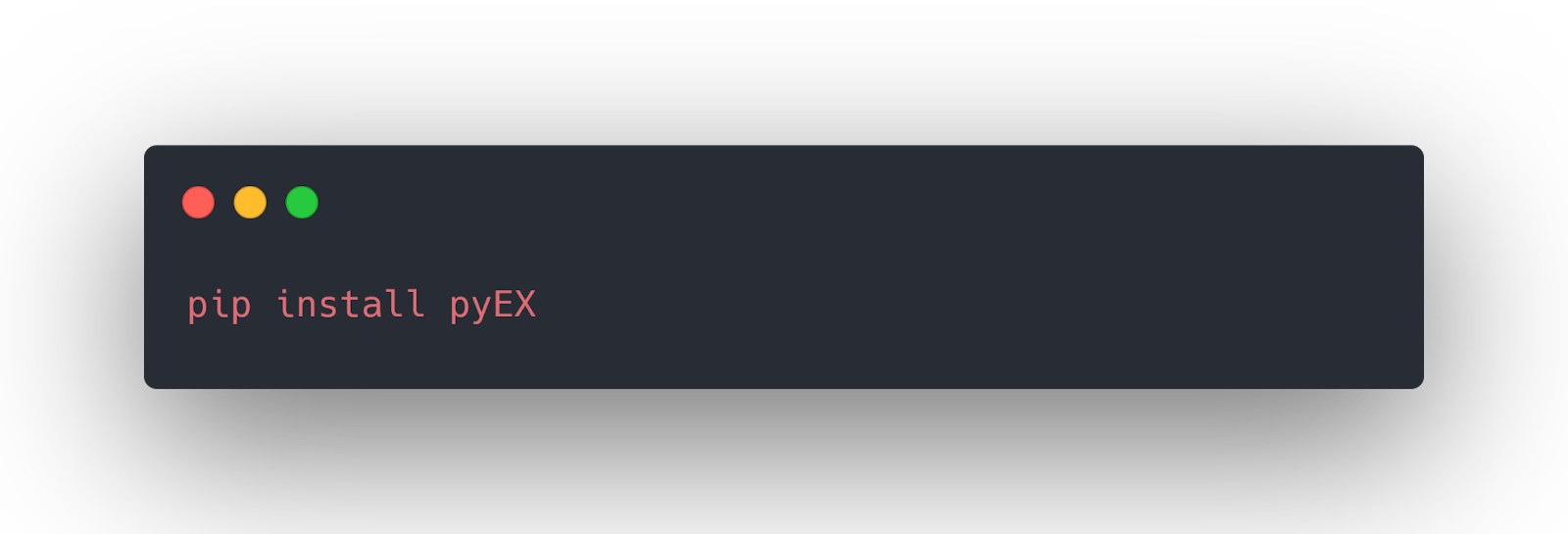
Connecting to the IEX Cloud and Making API Calls
Import the pyEX module and create an object of the Client class. Pass your API Key to the Client class constructor.
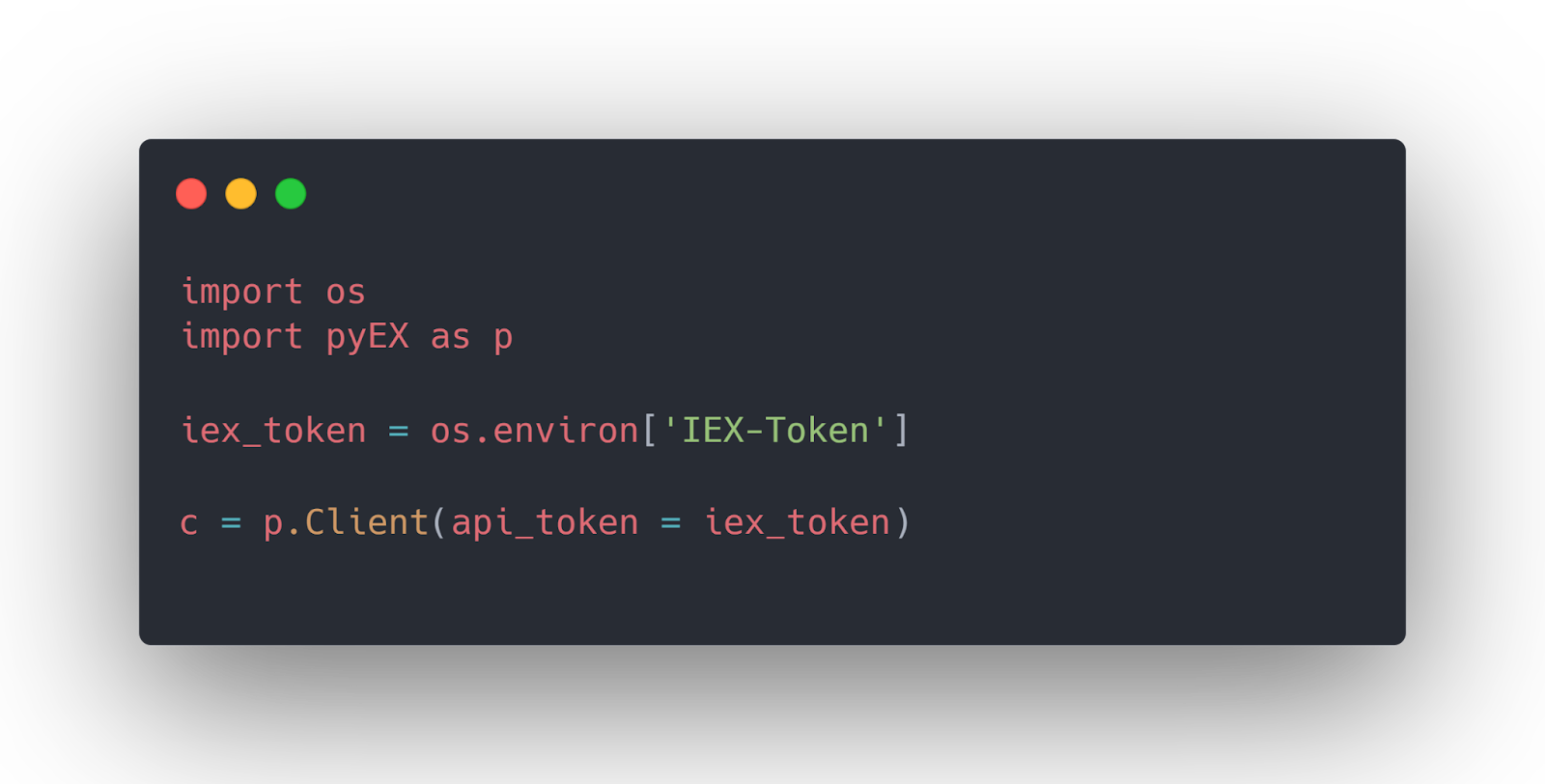
Next, you can call any Client class methods to access the corresponding IEX Cloud API endpoint.
For instance, you can access the IEX Cloud charts endpoint via the chart() method of the Client class. The method returns historical stock prices.
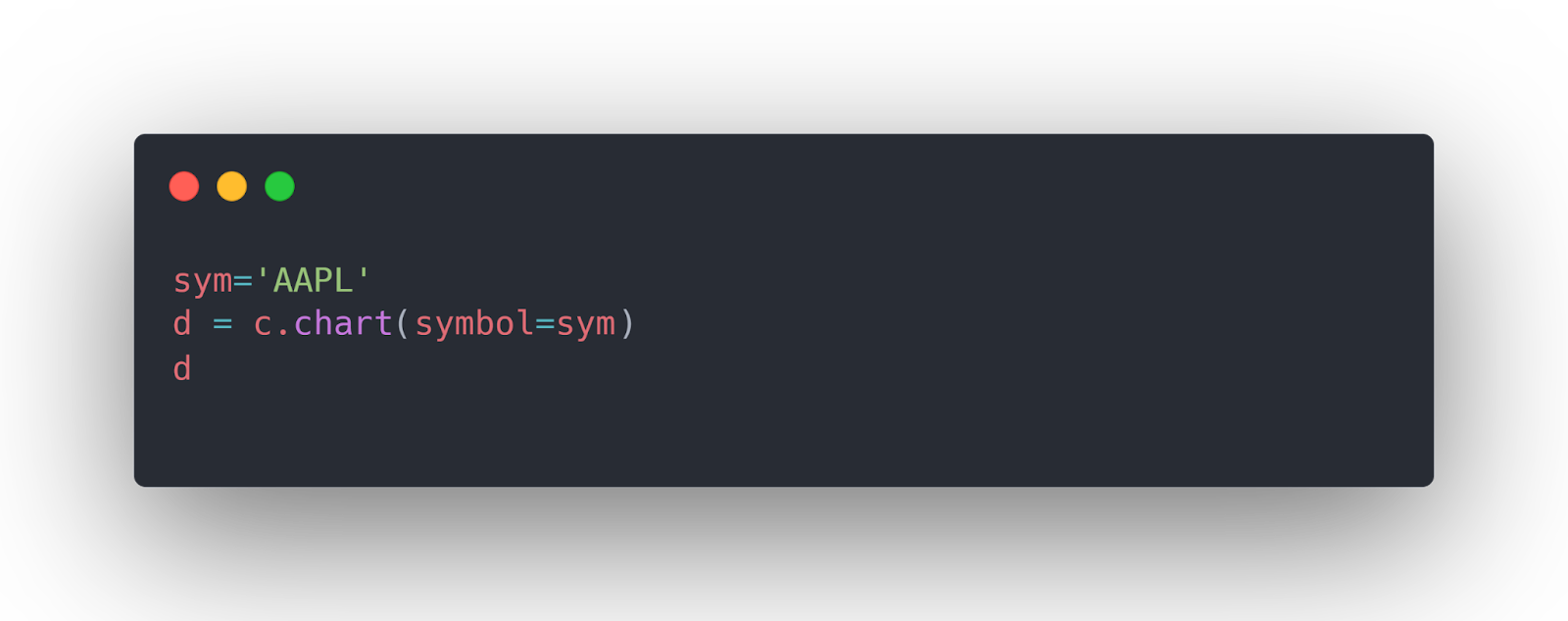
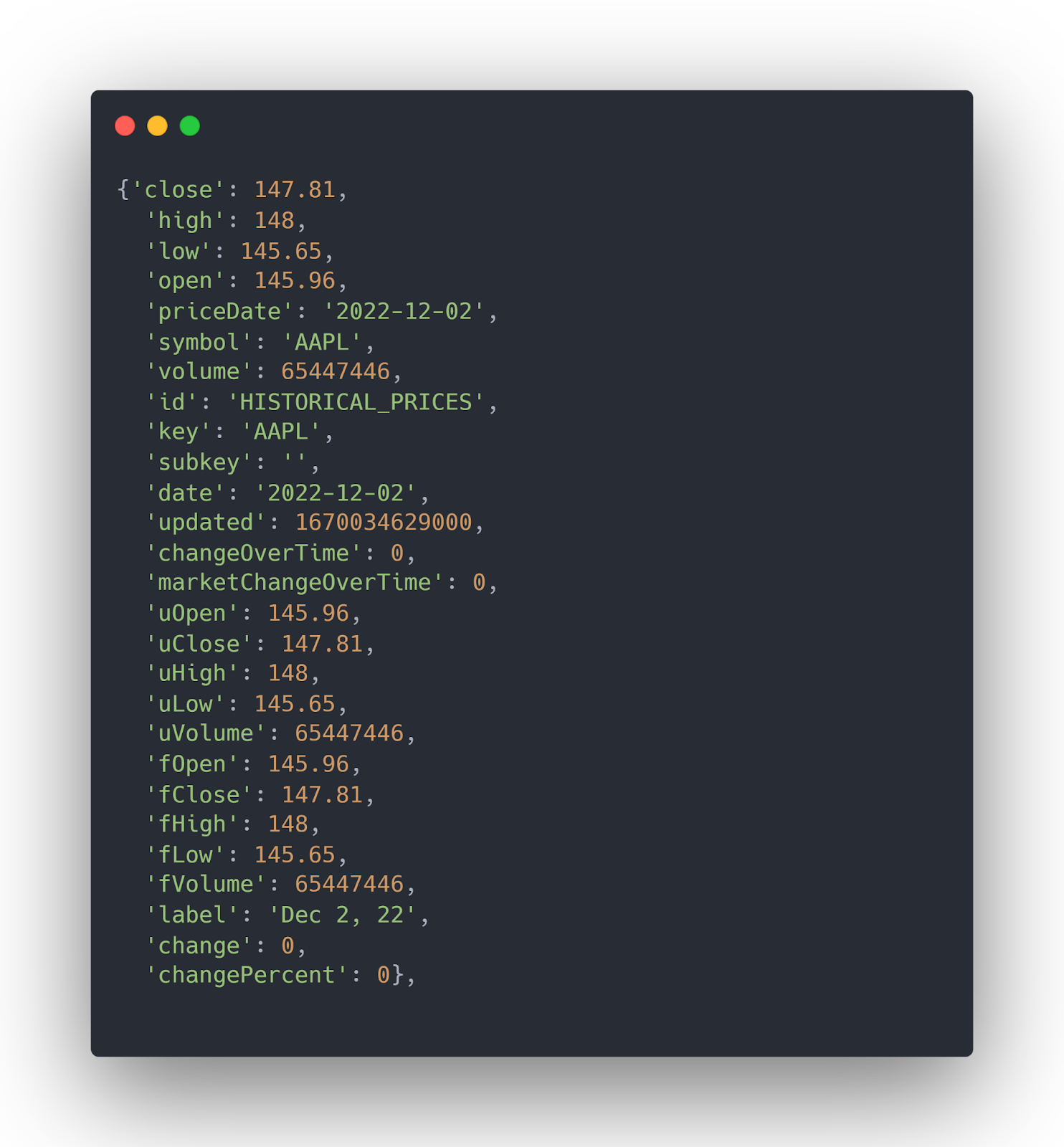
IEX Cloud Requests Library Example
The requests library’s get() method retrieves data from REST APIs. To access the IEX Cloud API function, you must pass the API URL to the get() method. You must pass the API key in a Python dictionary to the params attribute of the get() method.
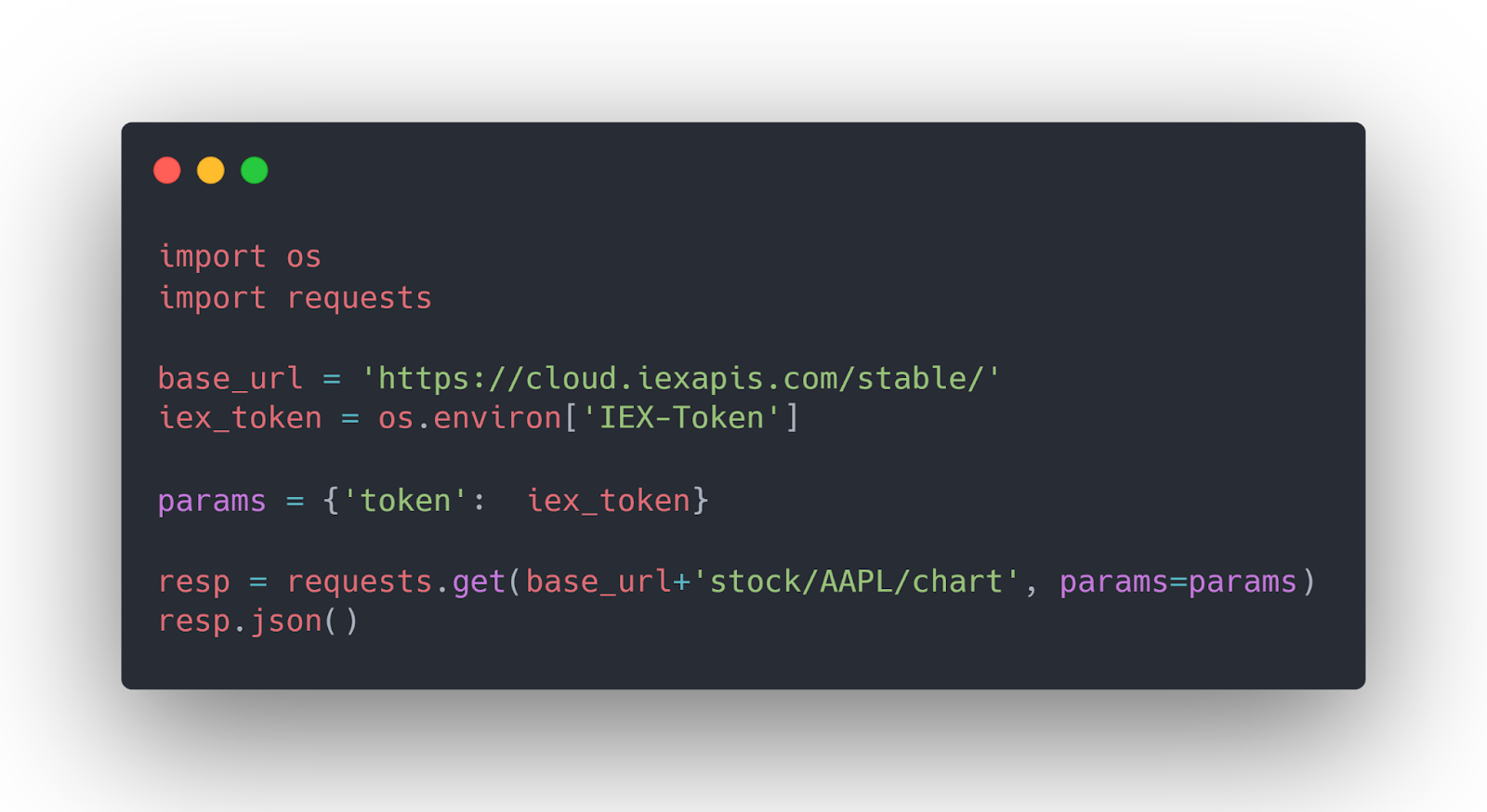
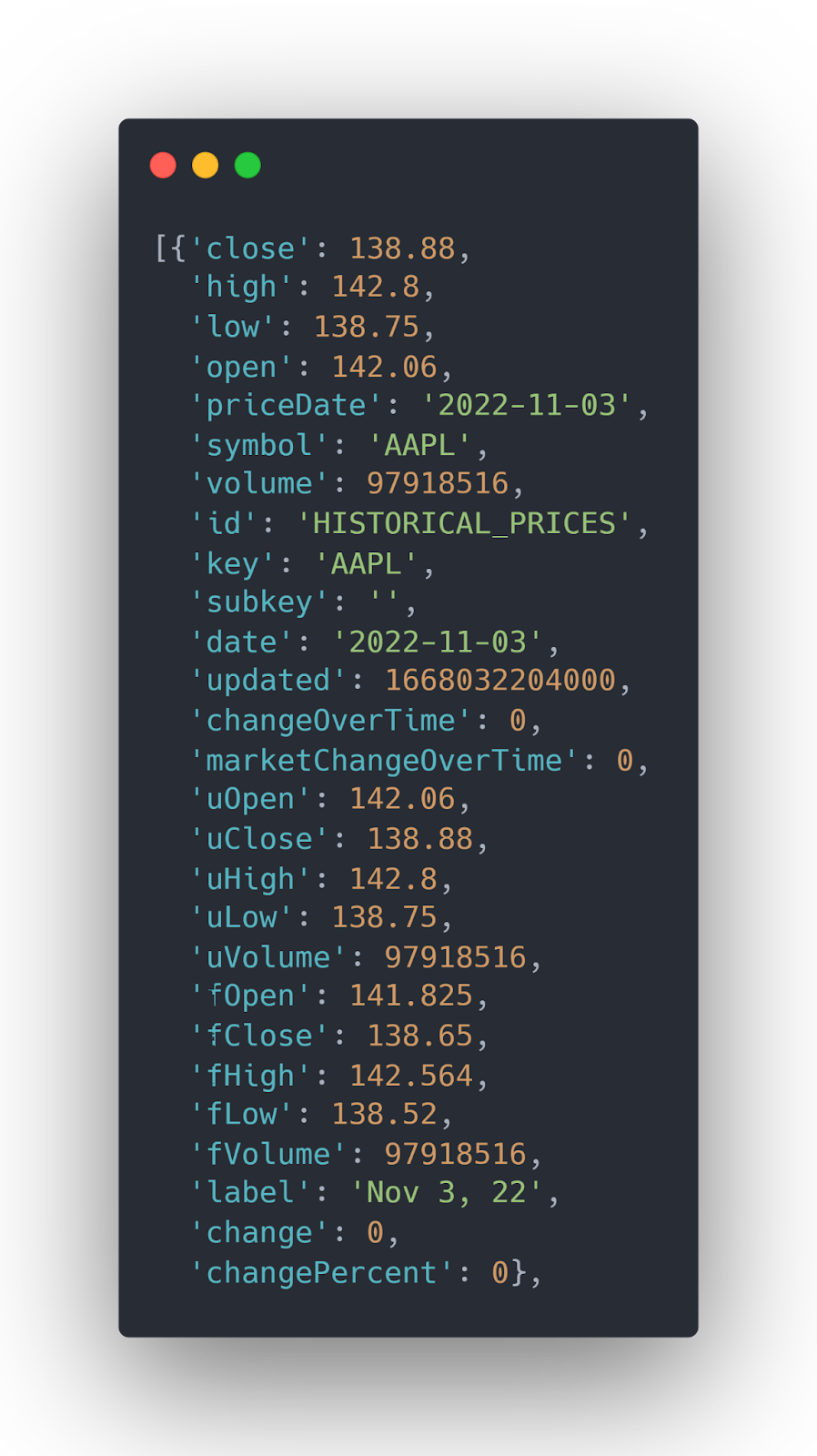
Working with Data
The PyEX library can fetch data in JSON format and Pandas DataFrame.
The Client class implements a method for JSON and Pandas Dataframe format. For more detail, see the list of all Client class methods.
Parsing JSON Responses
To fetch data in JSON format, call the Client class API methods without the trailing “DF.” For instance, you can use the chart() method to plot historical stock prices.
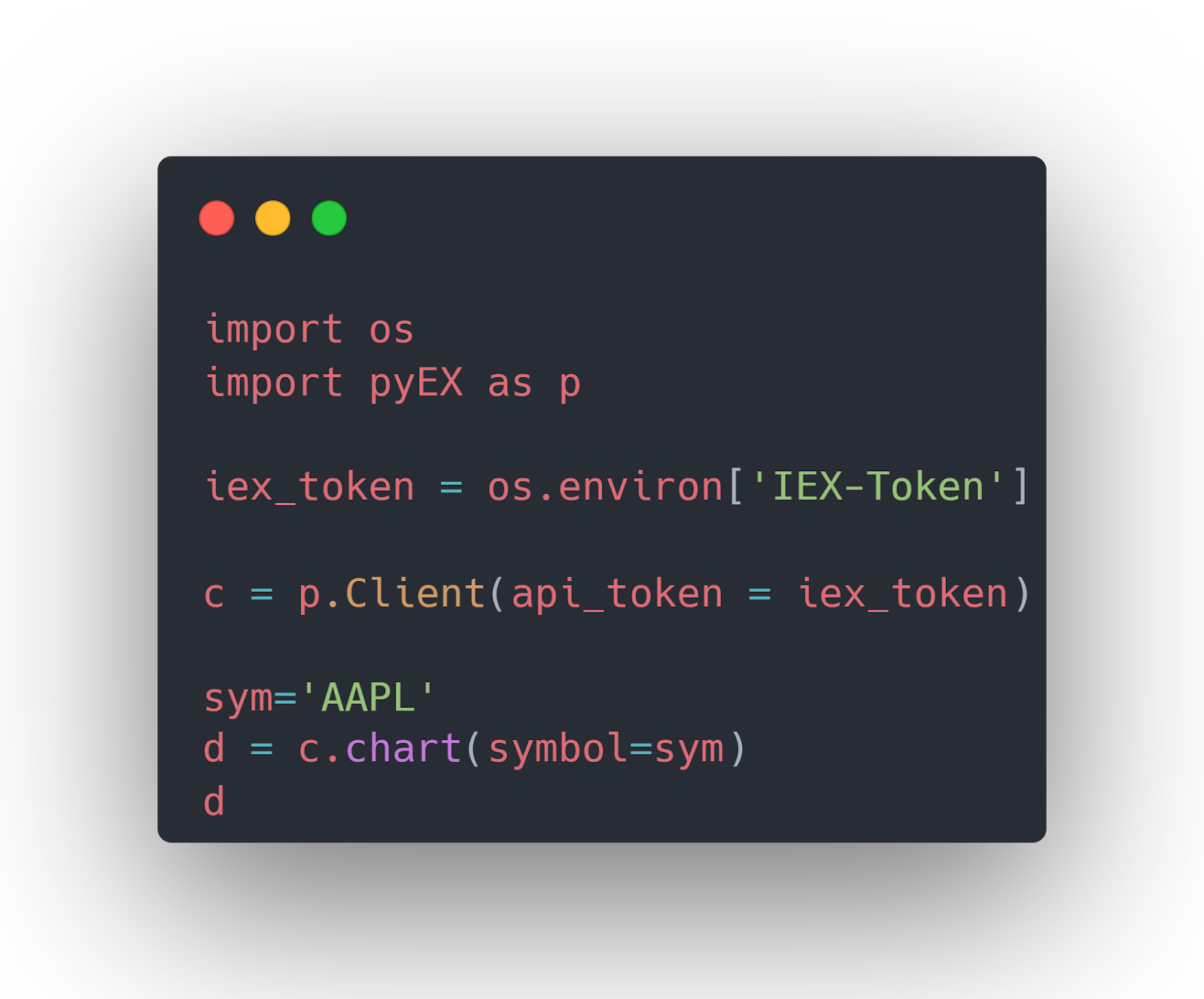
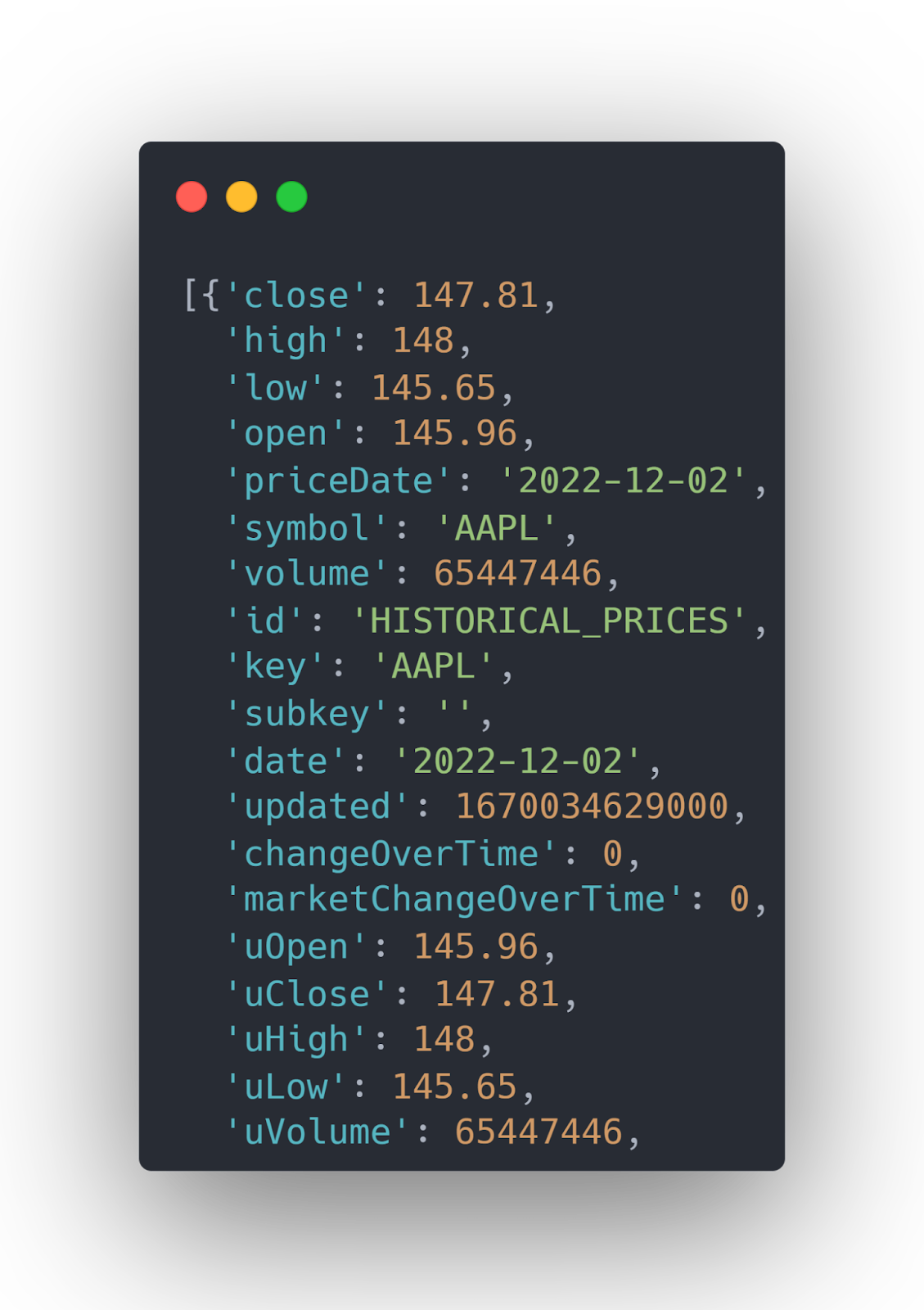
The requests library always fetches data in JSON format, which you can convert to a Python dictionary using the json() method. Here is an example:
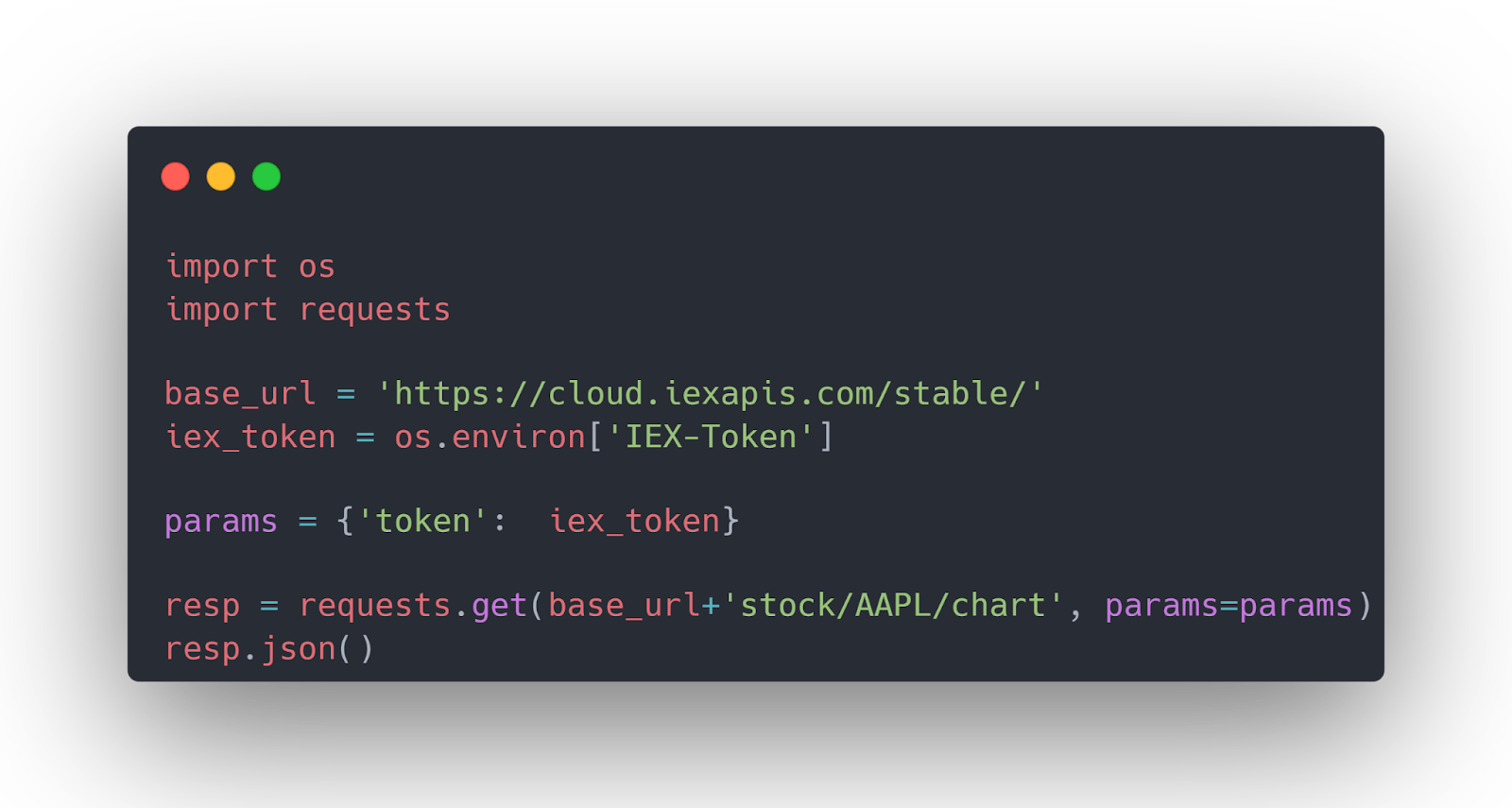
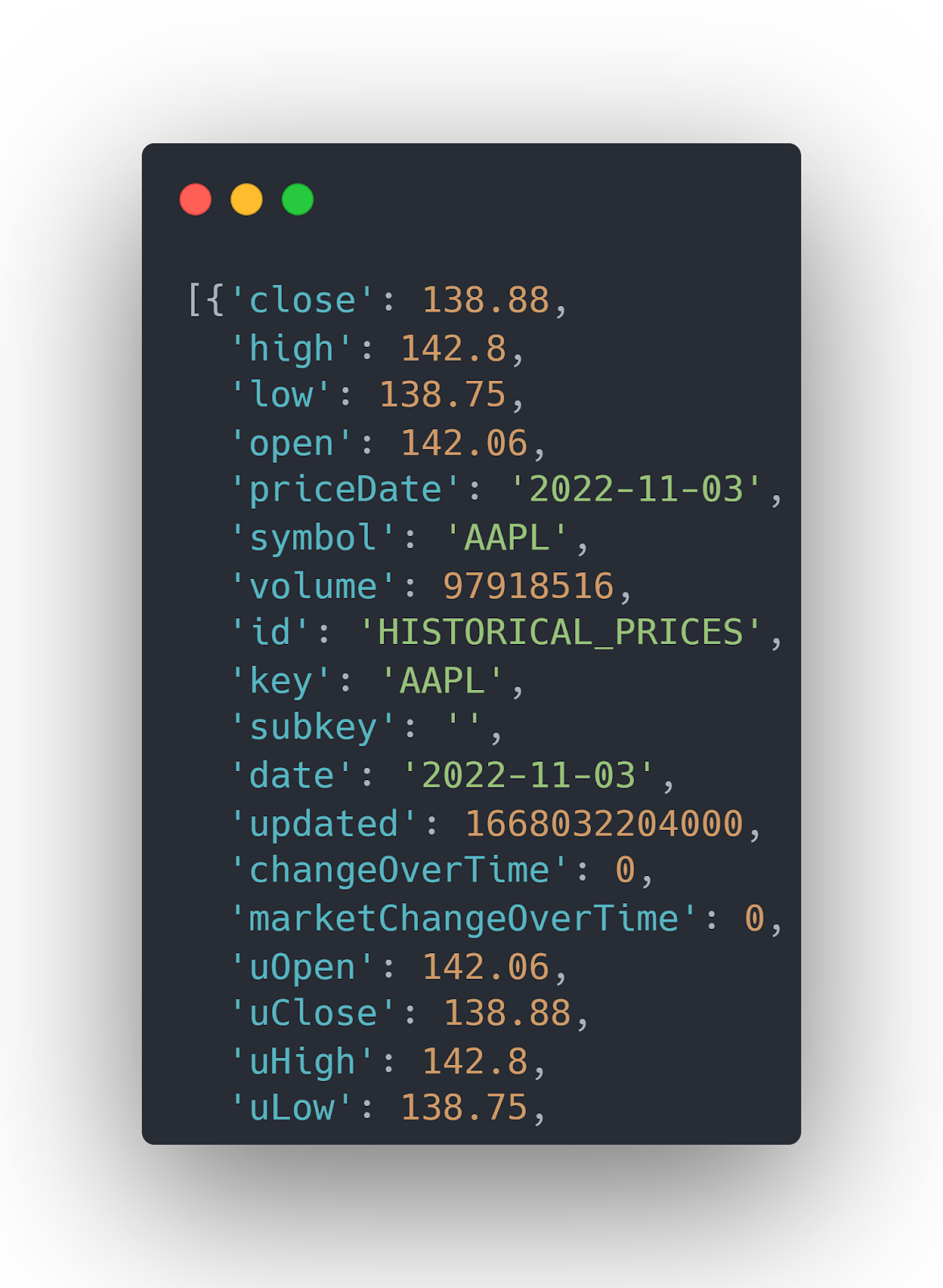
Working with DataFrames
The Client class methods that end in a trailing DF return data in a Pandas DataFrame object.
For instance, the chartDF() method returns historical stock prices in a Pandas DataFrame.
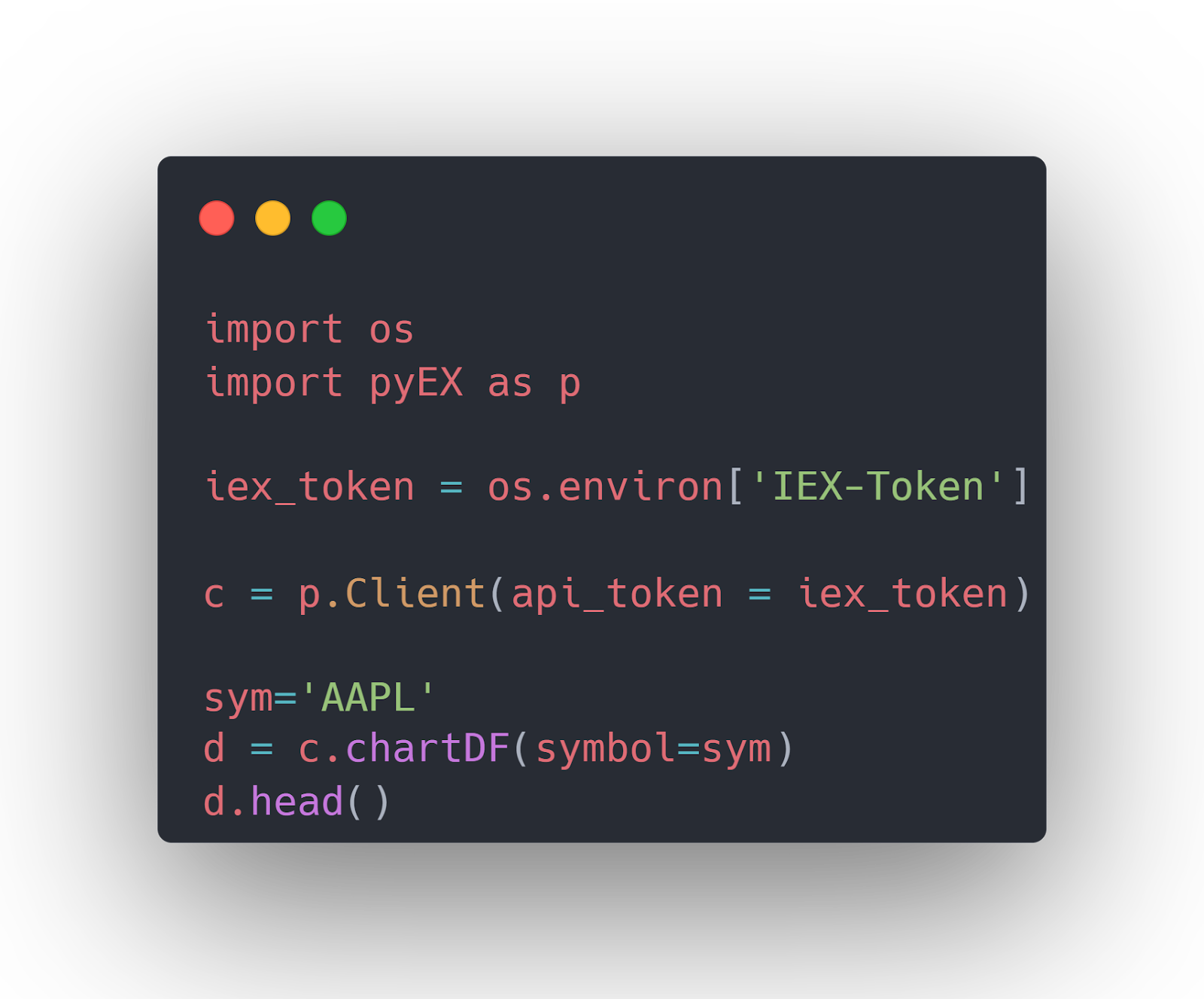
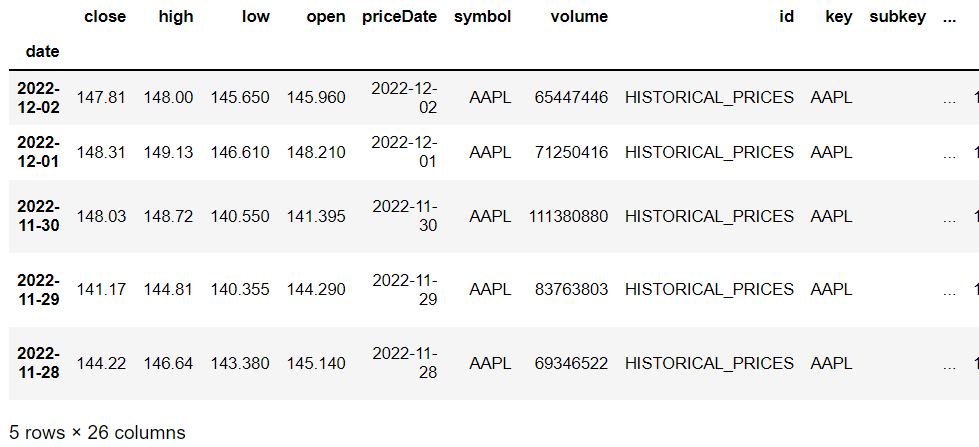
You can convert the data returned by the requests library’s get function into a Pandas DataFrame by passing the Python dictionary into the DataFrame class.
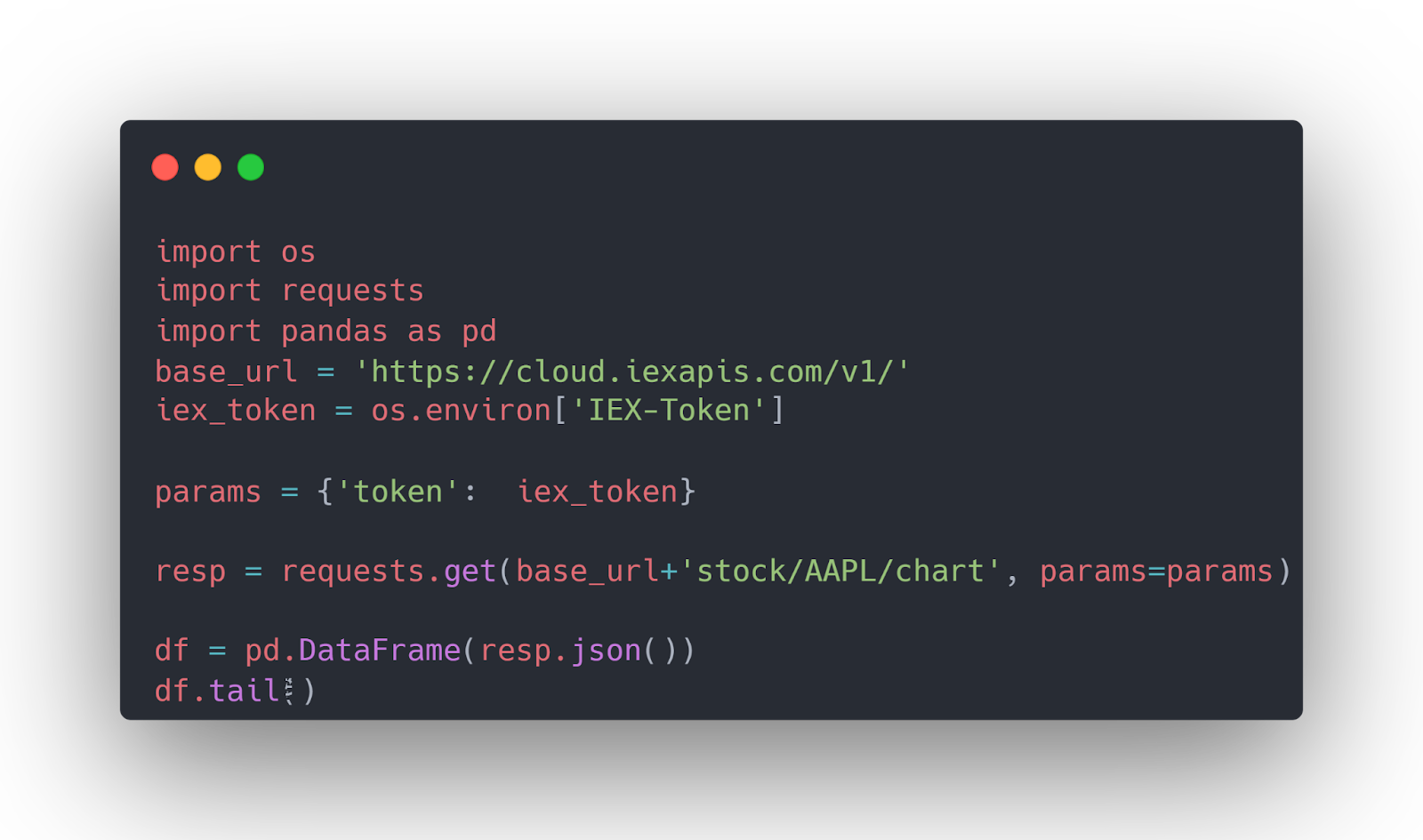
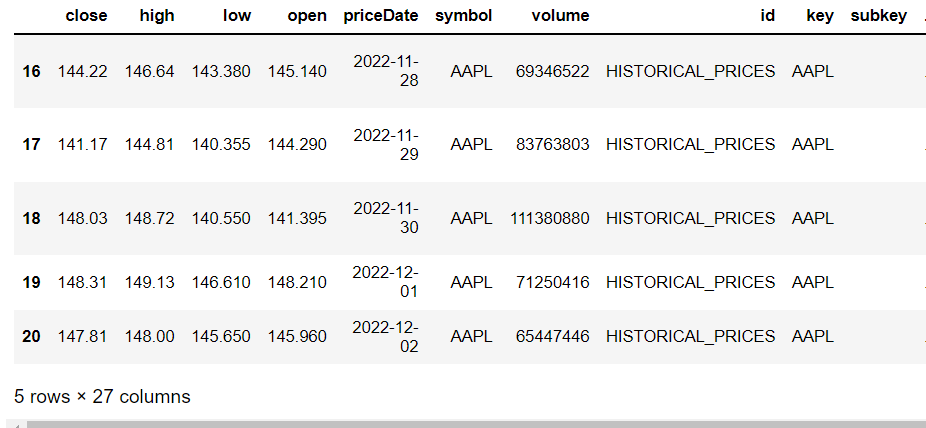
You can set the priceDate column of the above dataframe as the index column.
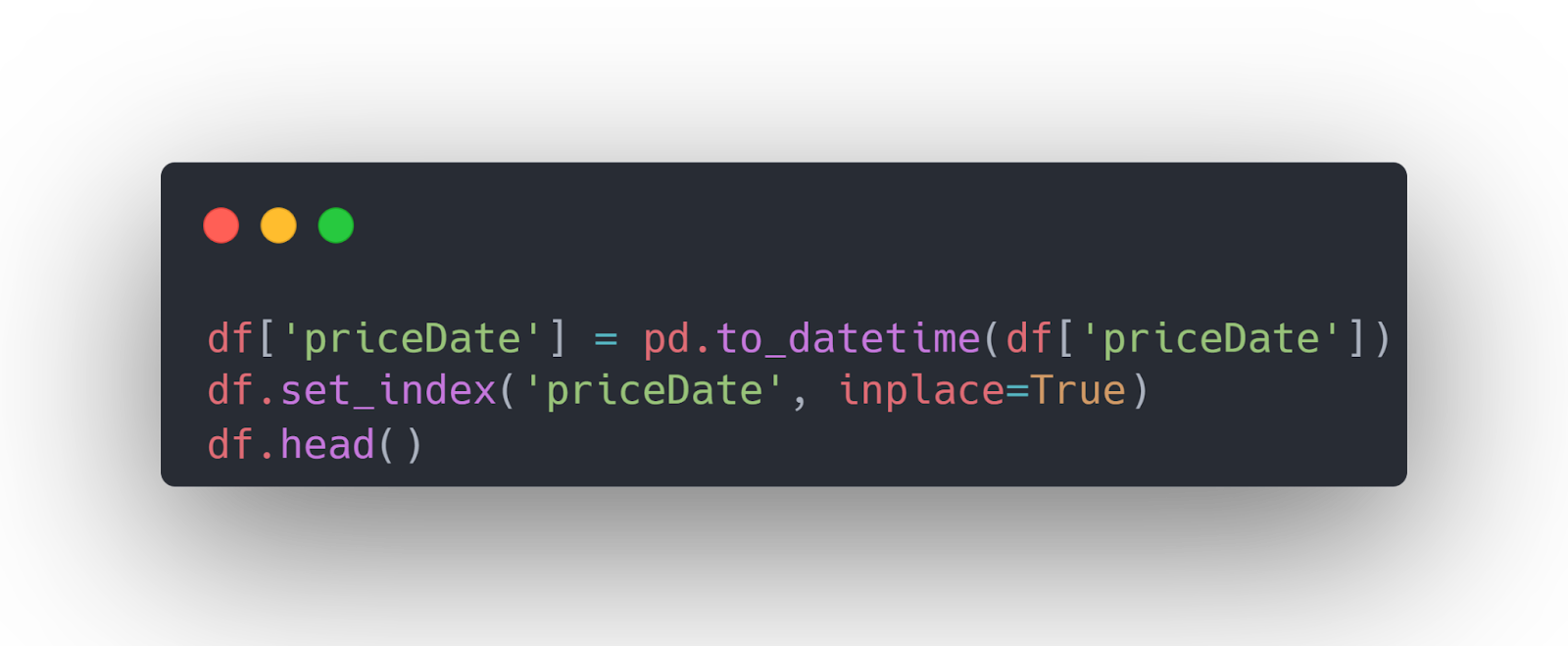
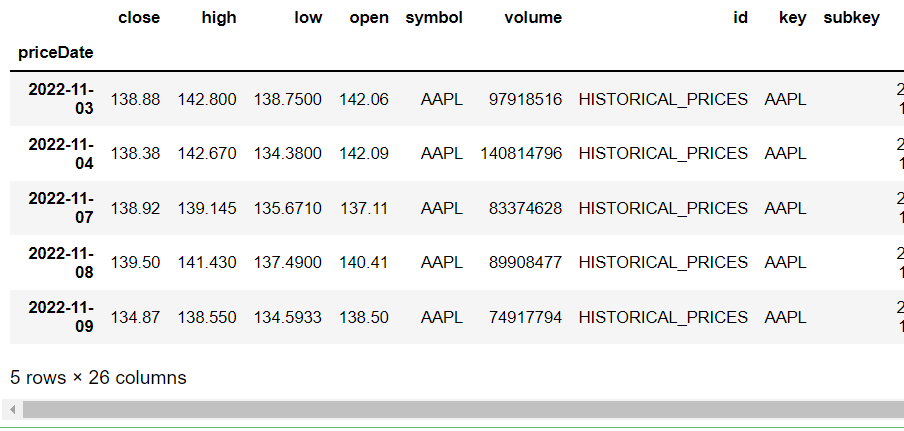
Visualizing Data
I always prefer to store the IEX Cloud API response in a Pandas DataFrame and then visualize the data using the DataFrame functions. For example, you can plot a line-plot showing a stock’s historical closing prices using the following script.
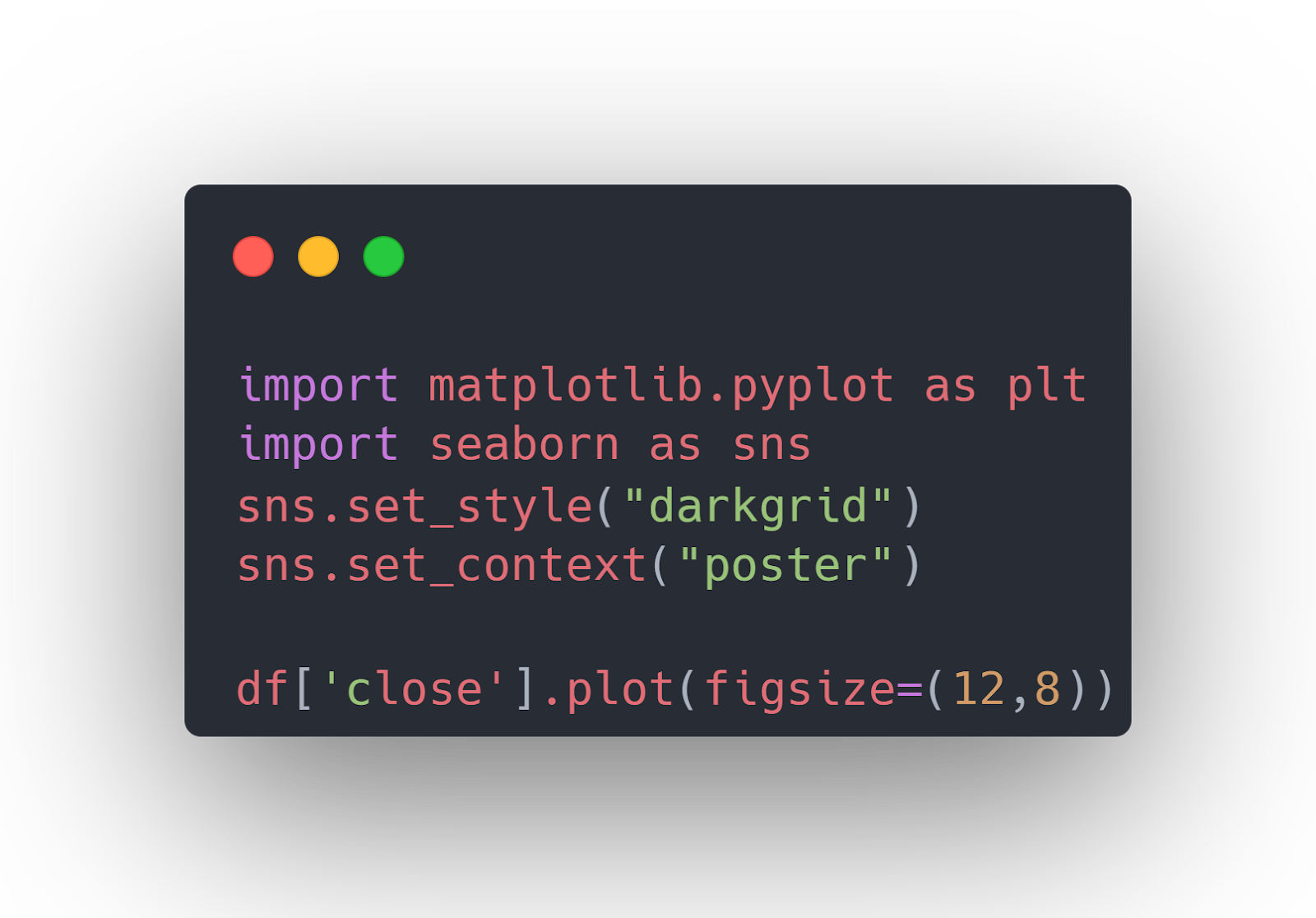
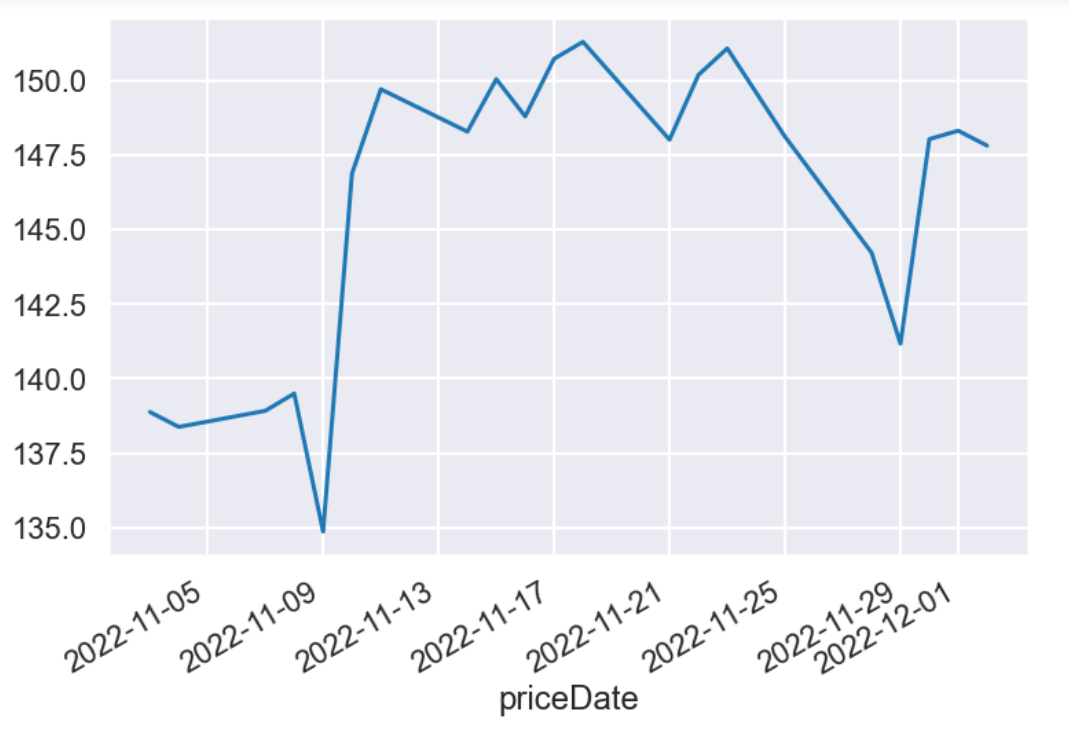
IEX Cloud API Endpoints
- Crypto
- Forex/Currencies
- Options
- Points
- Time Series
- Rates
- Stocks
- Commodities
- Economic
- Accounts
- News
- Reference Data
- Investors Exchange Data
Cryptocurrency Endpoint
This cryptocurrency endpoint returns information such as cryptocurrency prices, quotes, books, etc.
The cryptoSymbolsList() method returns a list of all IEX Cloud cryptocurrency symbols.
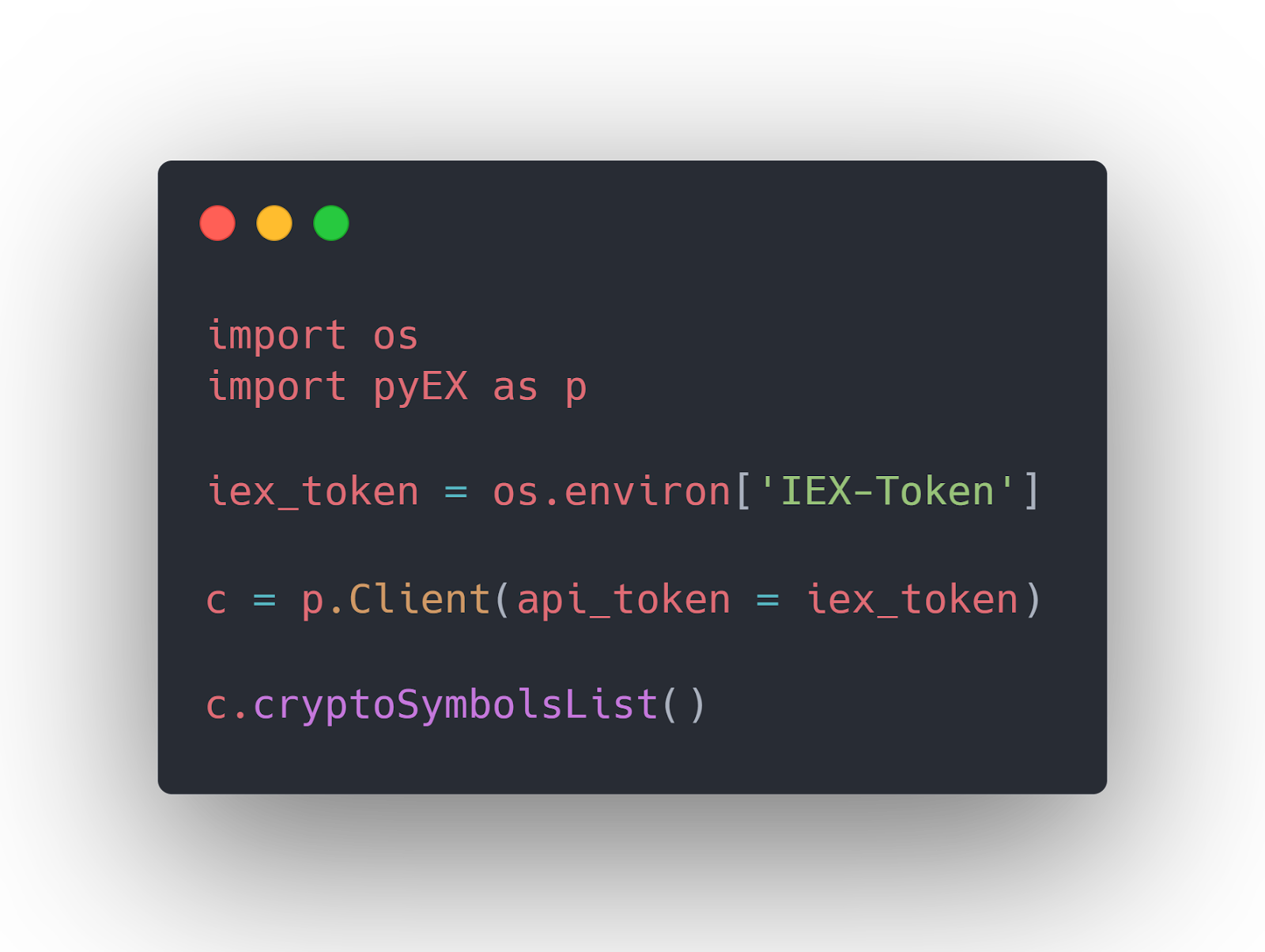
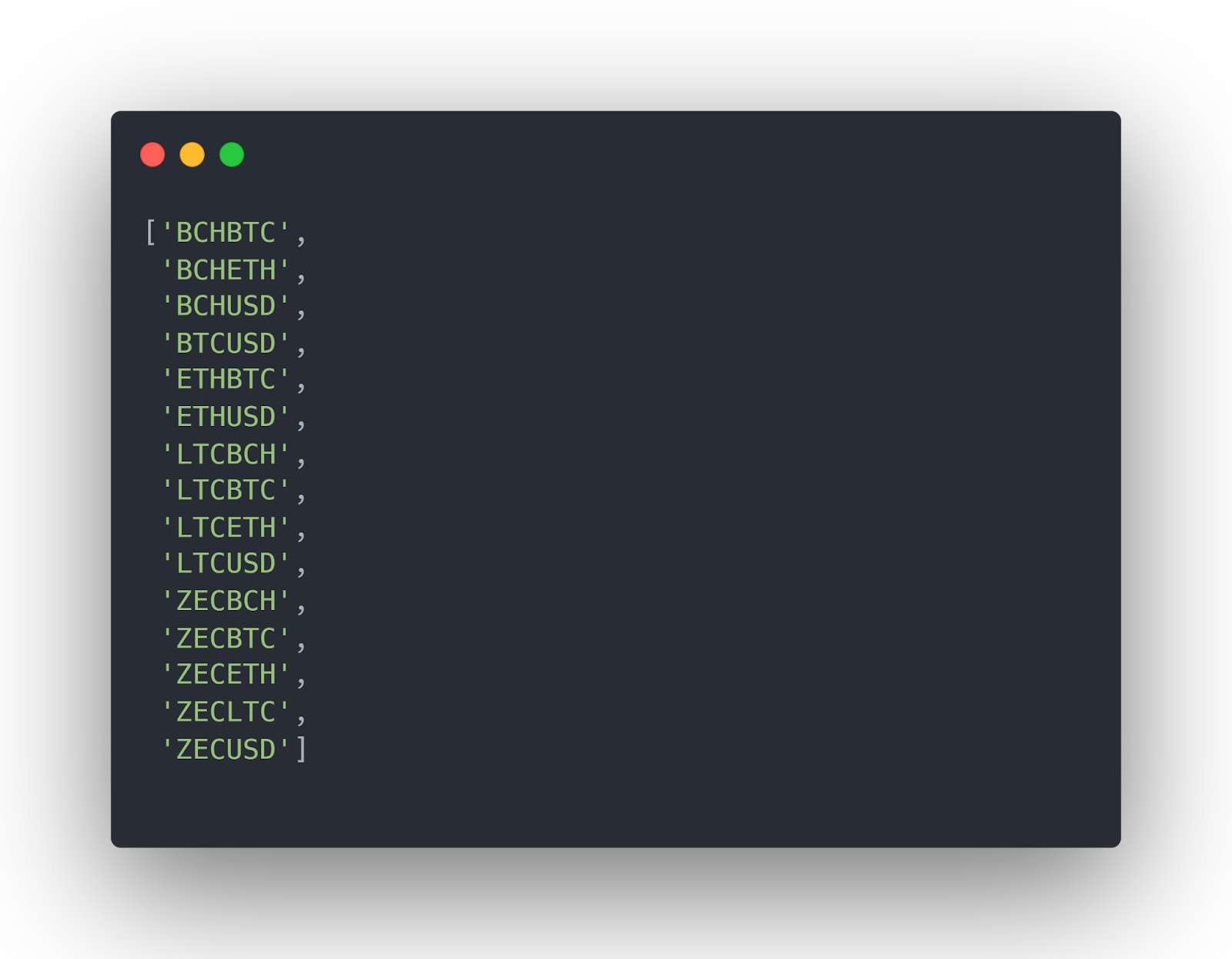
Get Cryptocurrency Prices
The cryptoPrice() method returns cryptocurrency prices using symbols. For instance, the “BTCUSD” symbol returns the price of Bitcoin in USD.
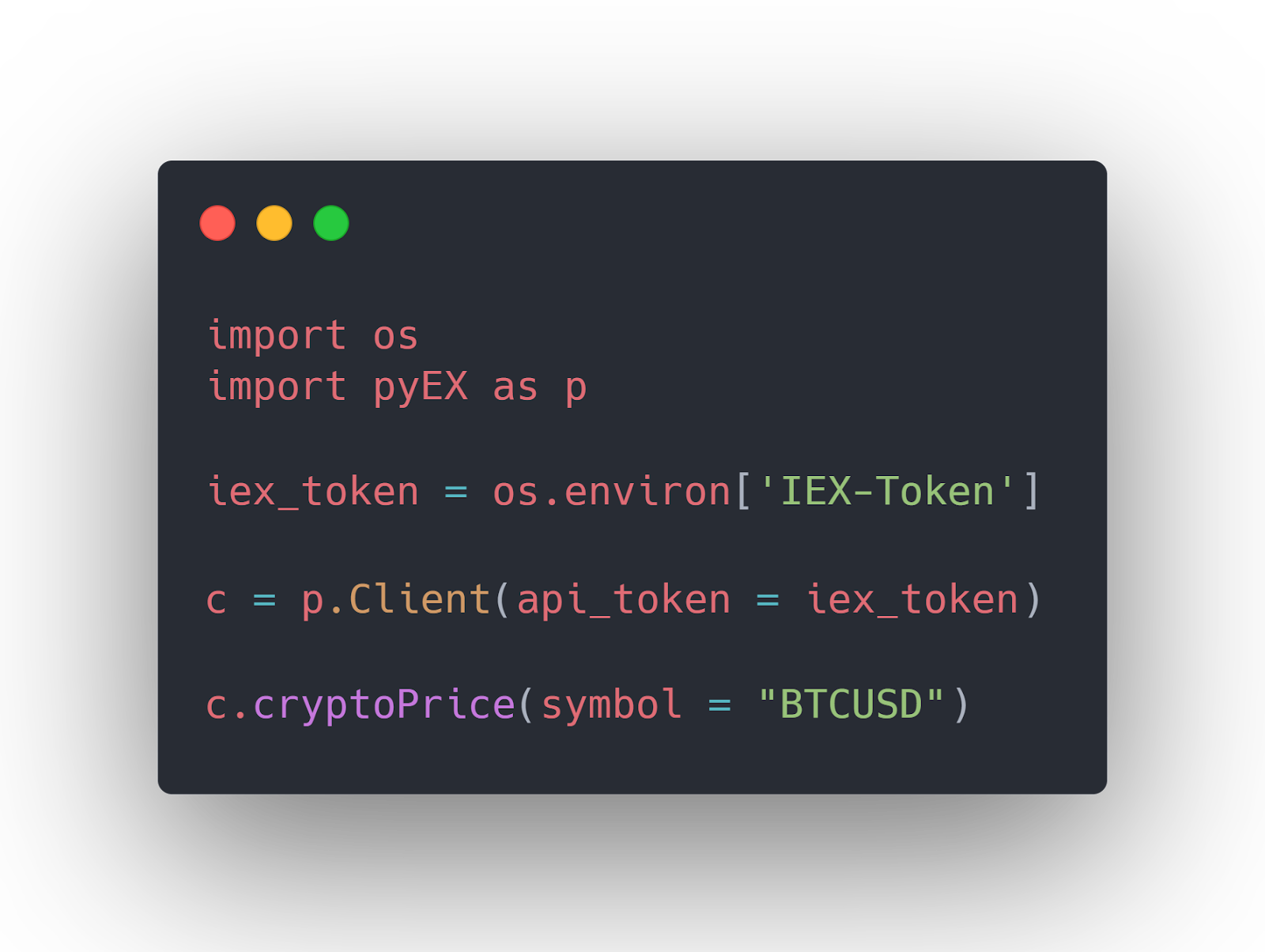
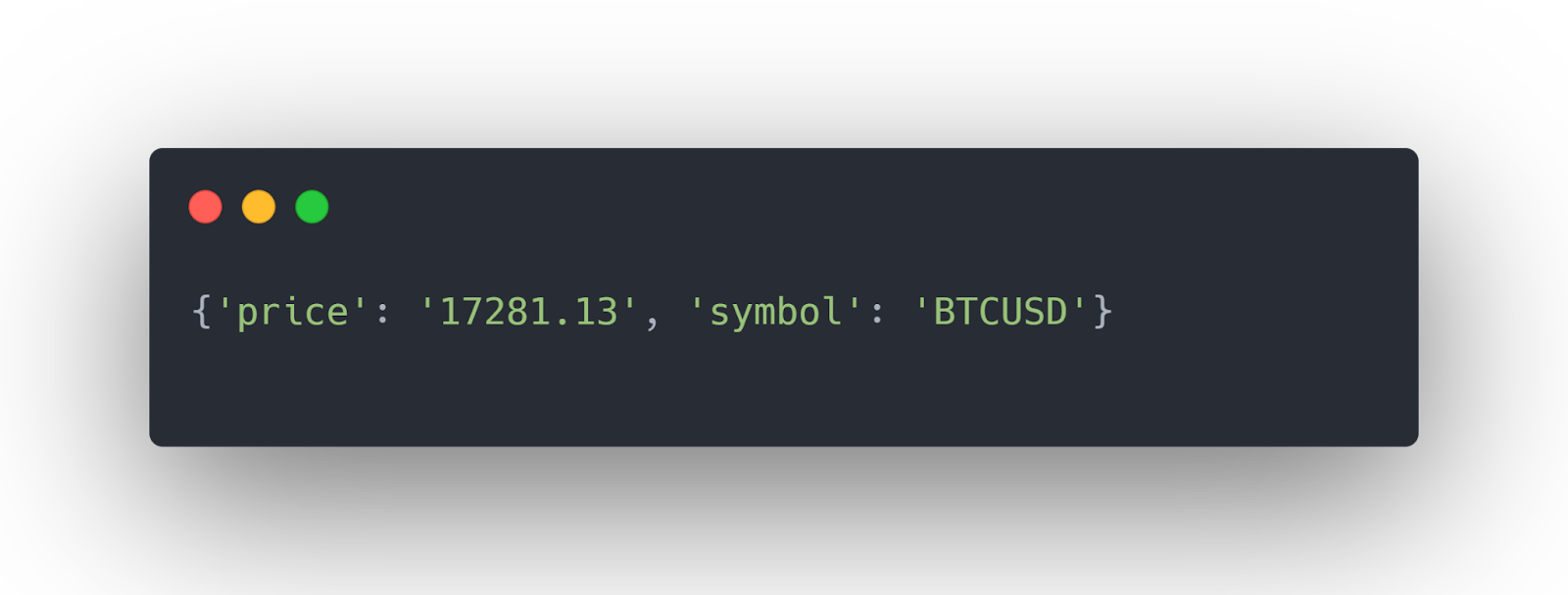
Get Cryptocurrency Quotes
The cryptoQuote() method returns cryptocurrency quotes. The following script returns quotes for Bitcoin in USD.
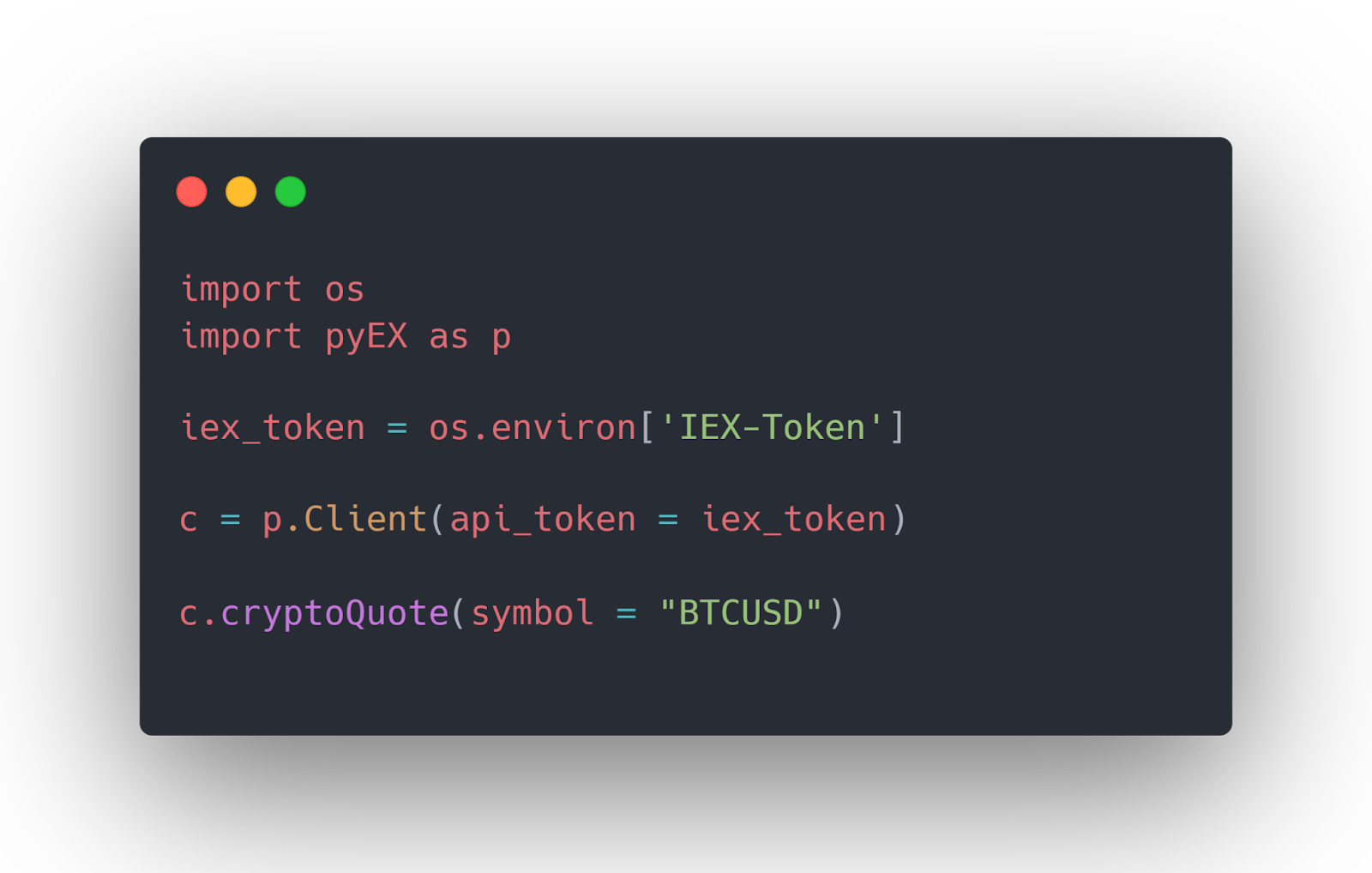
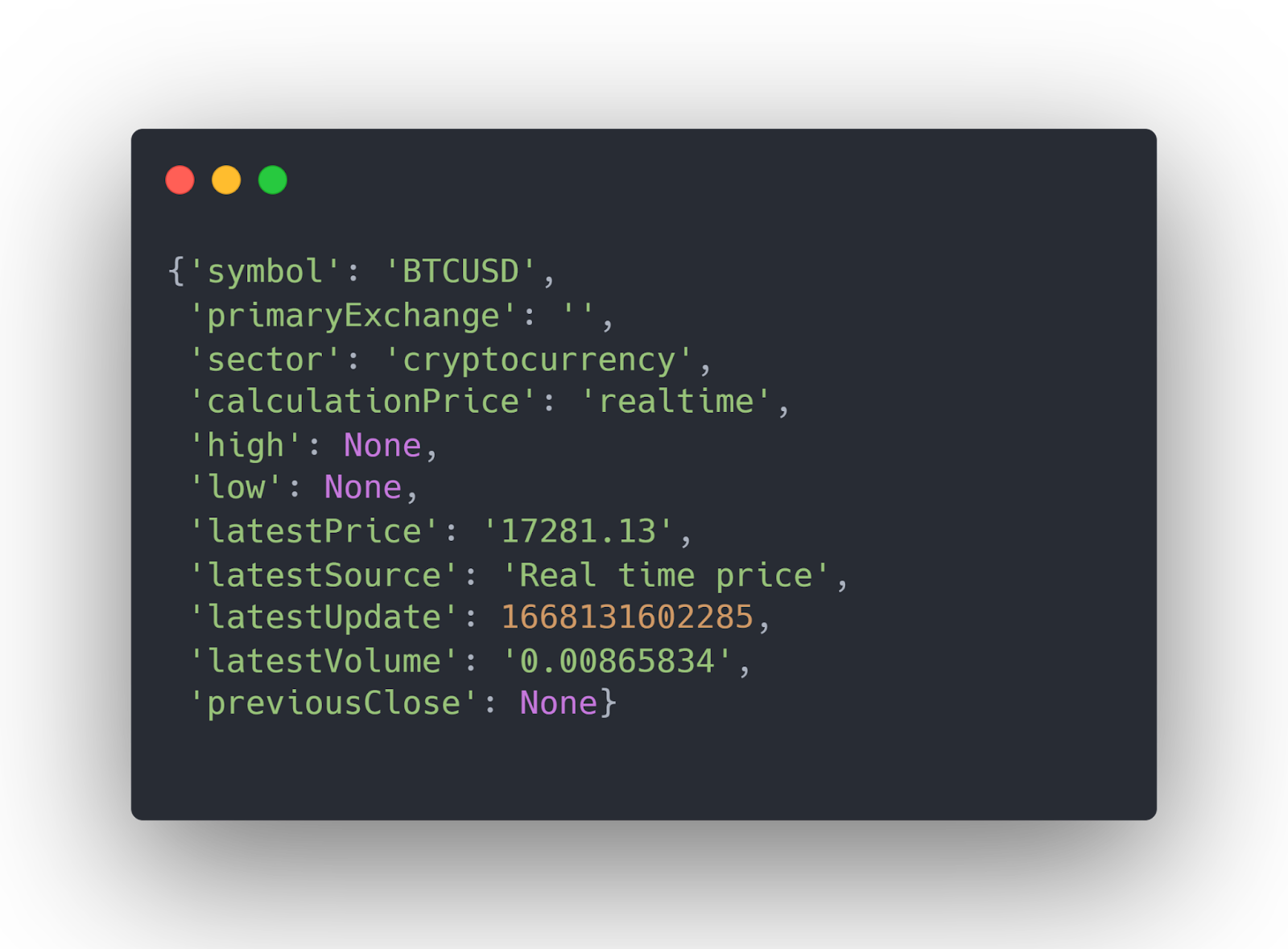
Get Cryptocurrency Book
The cryptoBookDF() method returns a list of all recent trades for a cryptocurrency. The method is not available with the free tier.
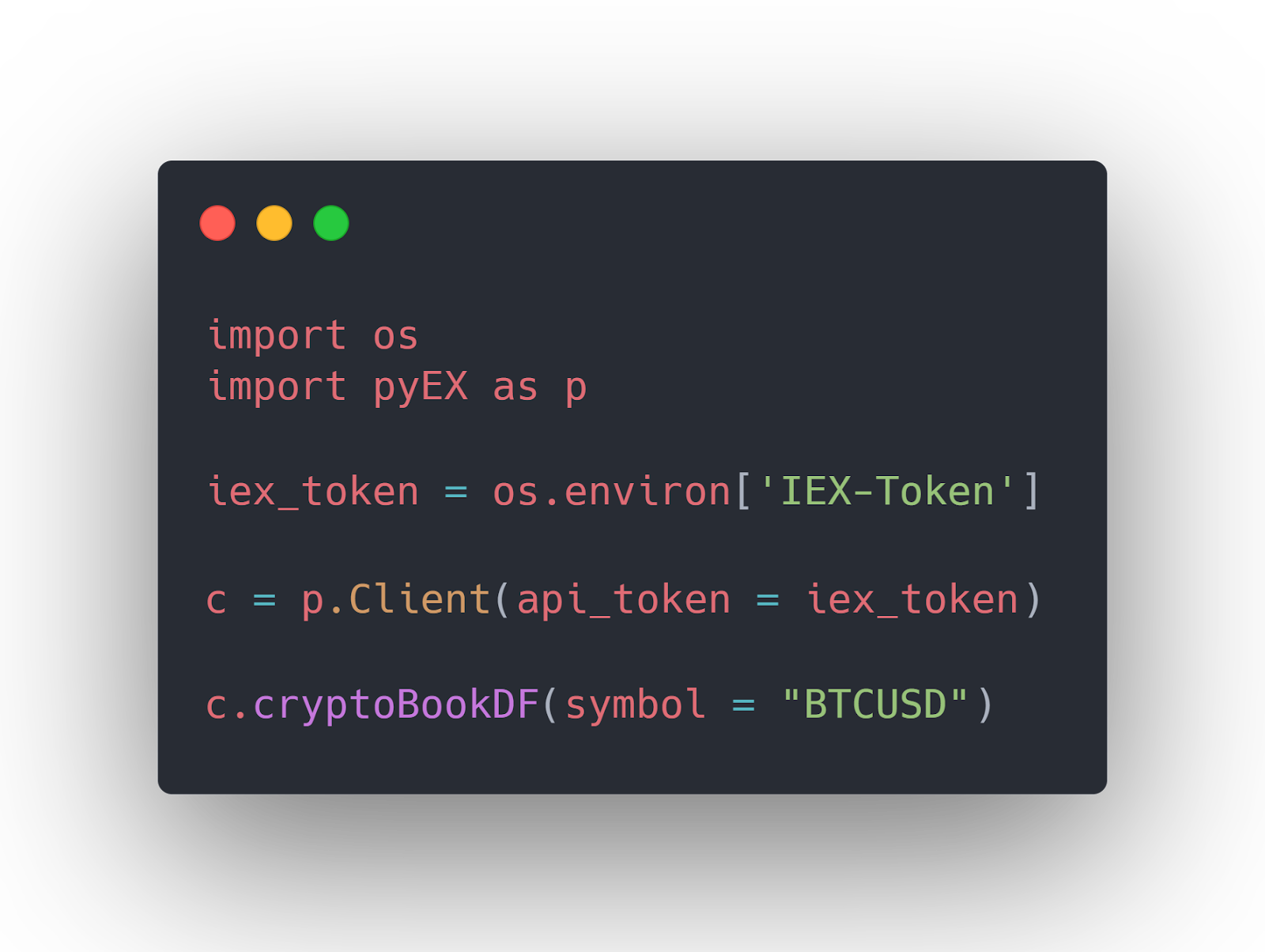
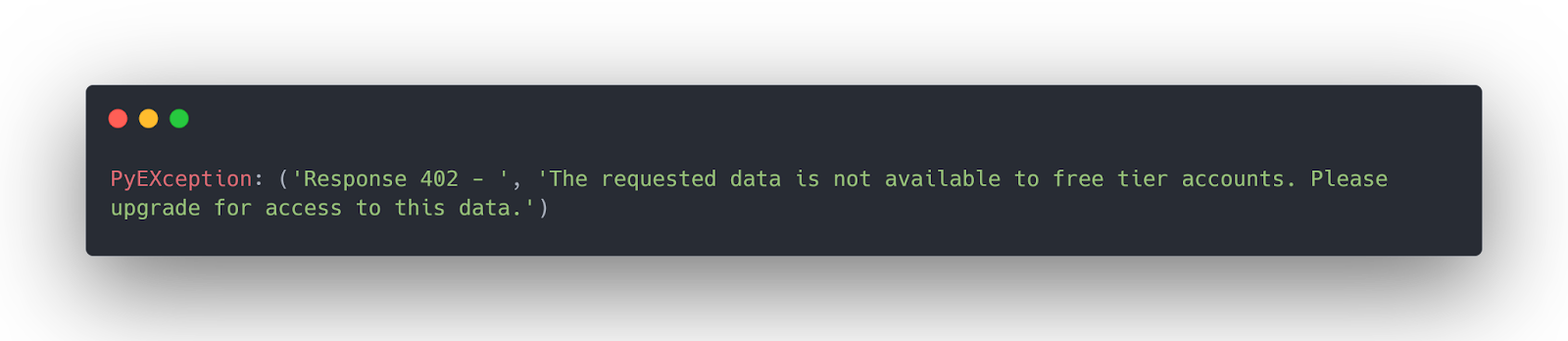
Forex/Currencies Endpoint
The forex endpoint fetches foreign exchange information such as foreign exchange rates, currency conversions, etc.
Convert Currencies
The convertFX() method converts one fiat currency into another. You must pass the source and destination currencies in a list to the symbols attribute and the amount to convert to the amount attribute. The endpoint is not available with the free tier.
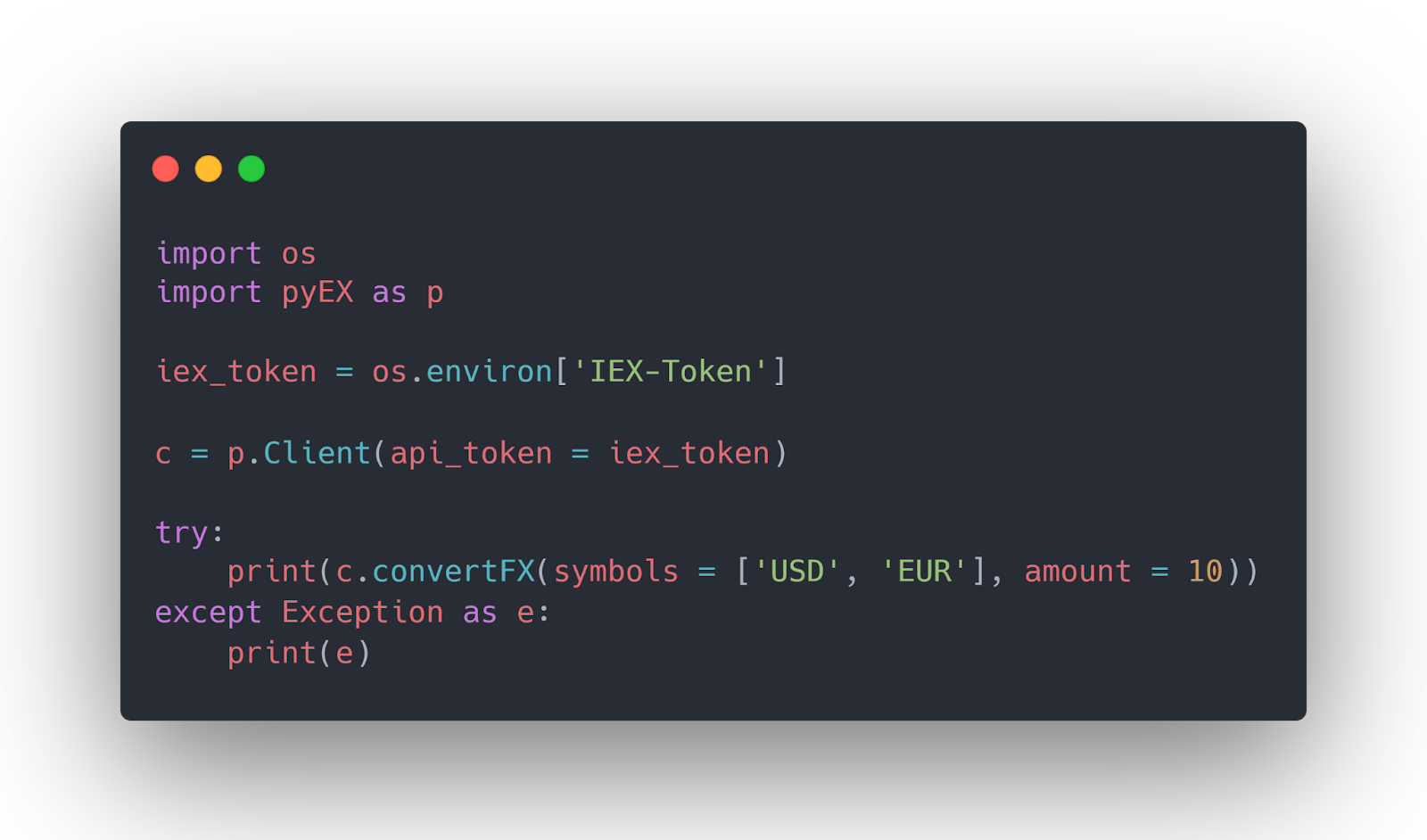
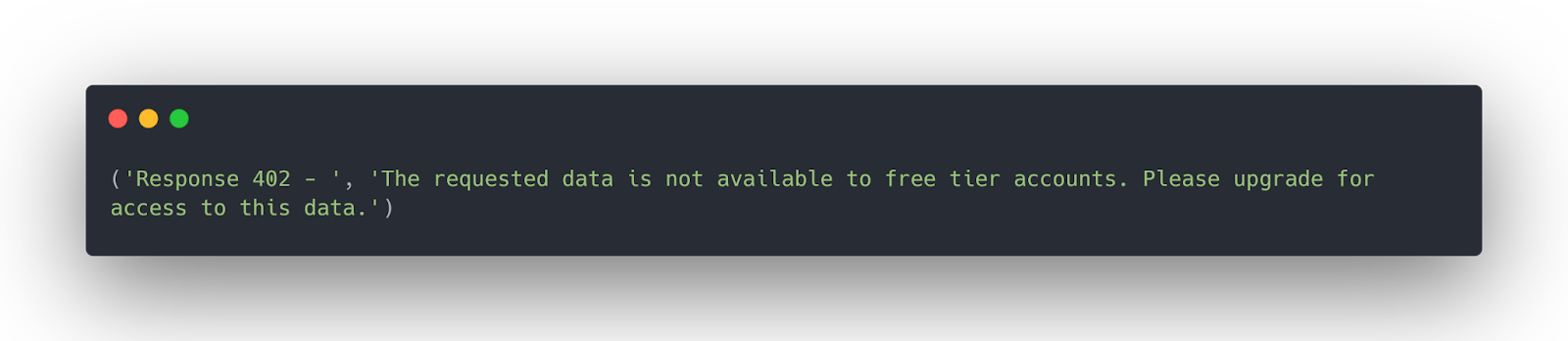
Get Realtime Foreign Exchange Rates
The latestFX() method retrieves real-time forex exchange rates for the data updated every 250 milliseconds. The method is not available with the free tier account.
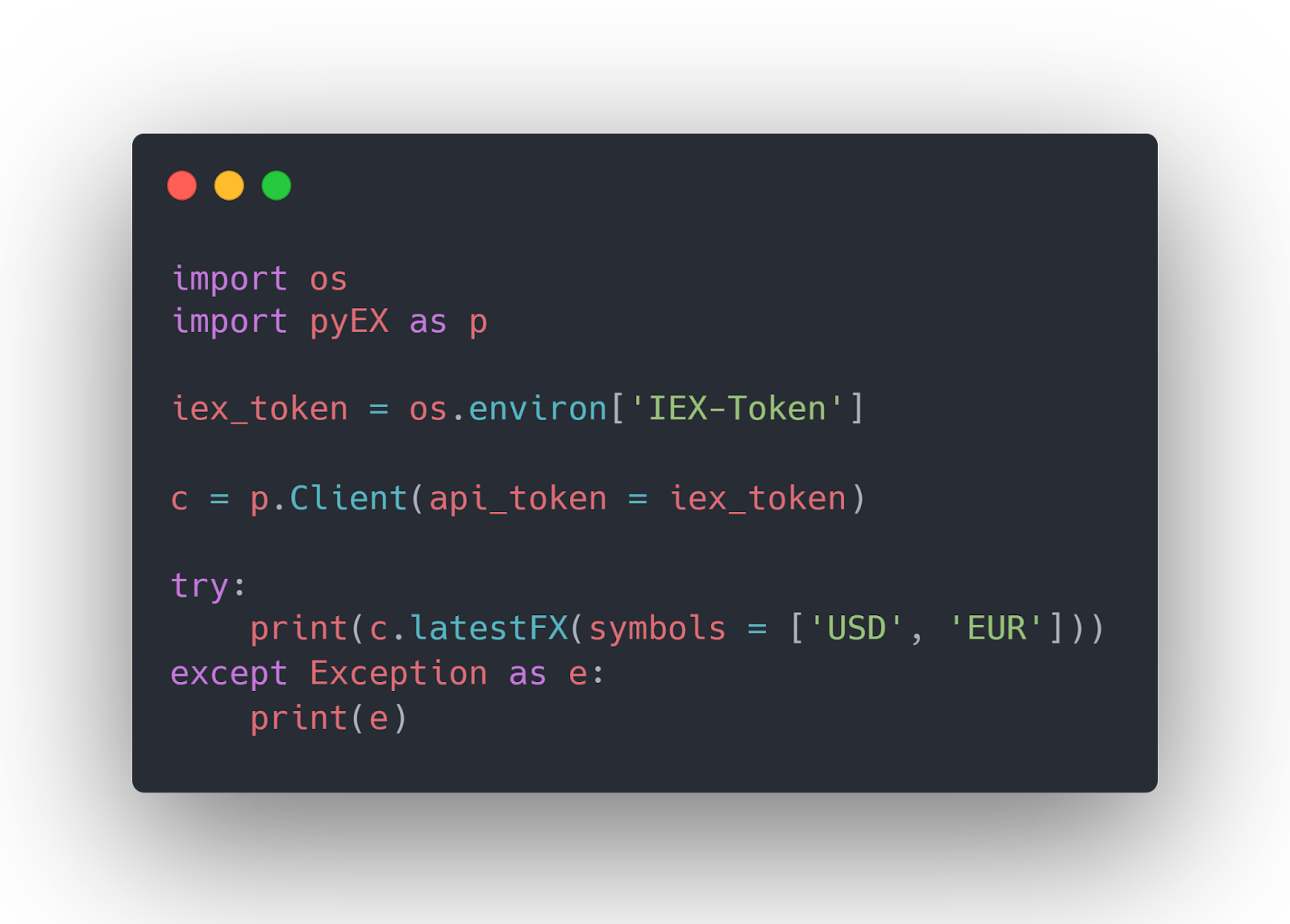
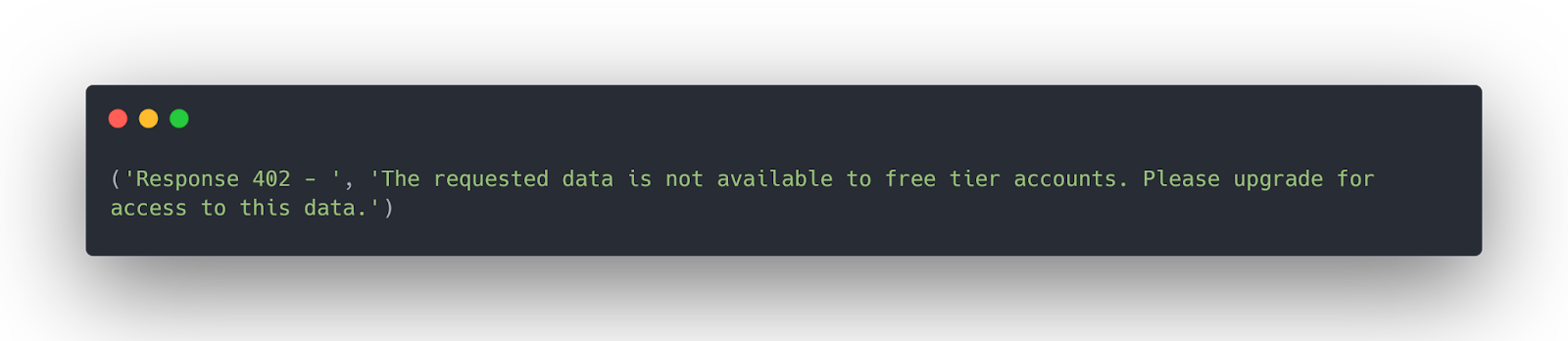
Options Endpoint
The options endpoint has a single function that returns end-of-day options data for a stock. Options data refers to the information on financial instruments that guide traders to make better purchasing or selling decisions on stocks.
Get End of Day Options Data
The optionExpirations() method returns end-of-day options data for a stock. You must pass the stock symbol to the symbol attribute.
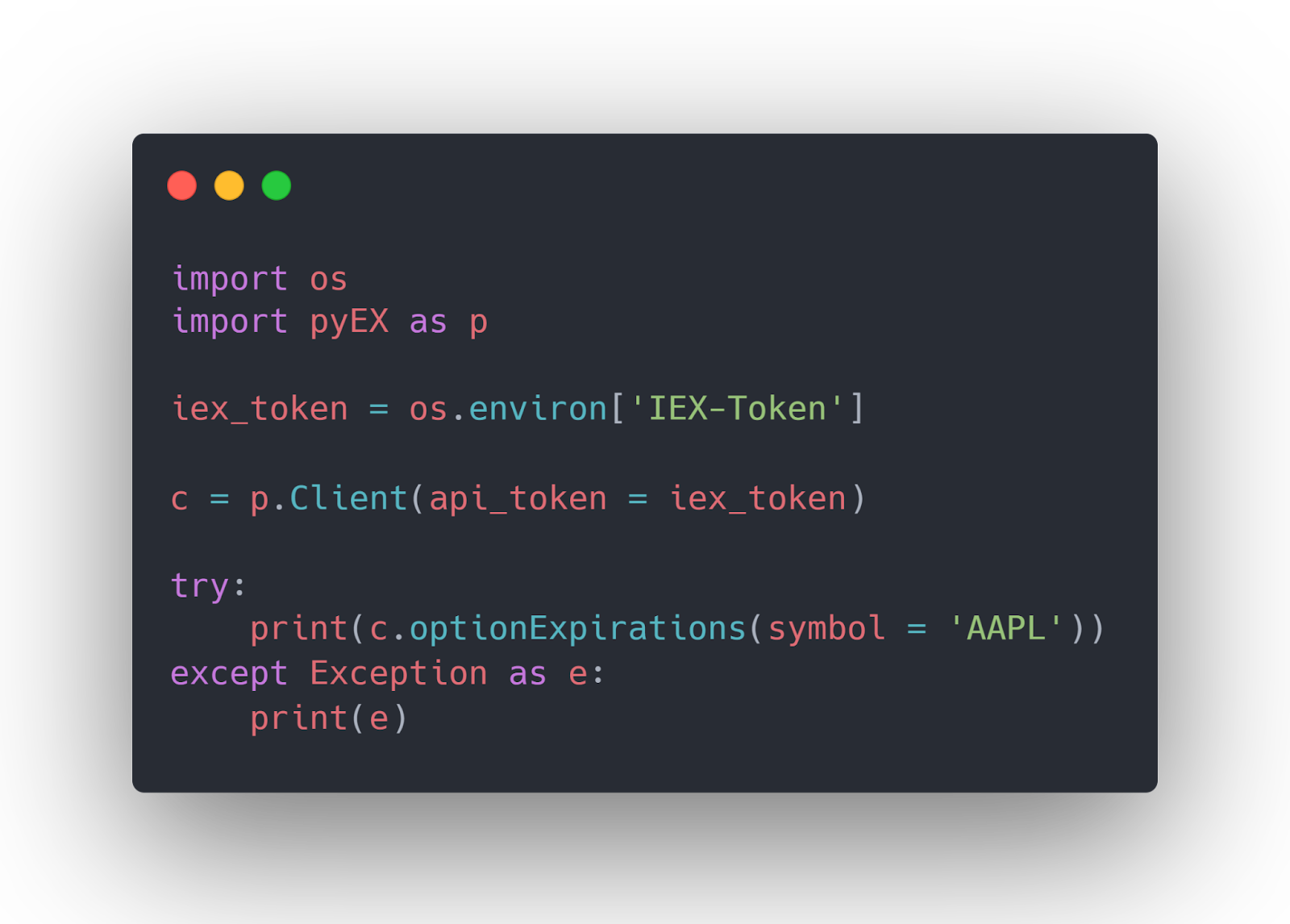
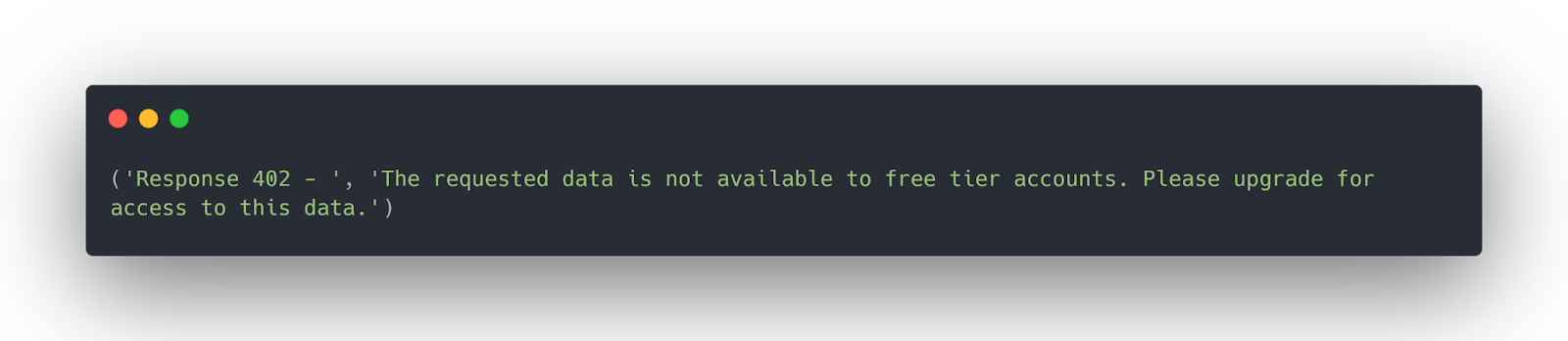
Points Endpoint
The point endpoint returns individual plain text values for a stock. Retrieving individual data is useful where a single, lightweight value is needed.
Get Individual Data Points
The points() method returns individual data points for a stock. In the output, you will see values for total liabilities, shareholder equities, retained earnings, capital expenditures, etc.
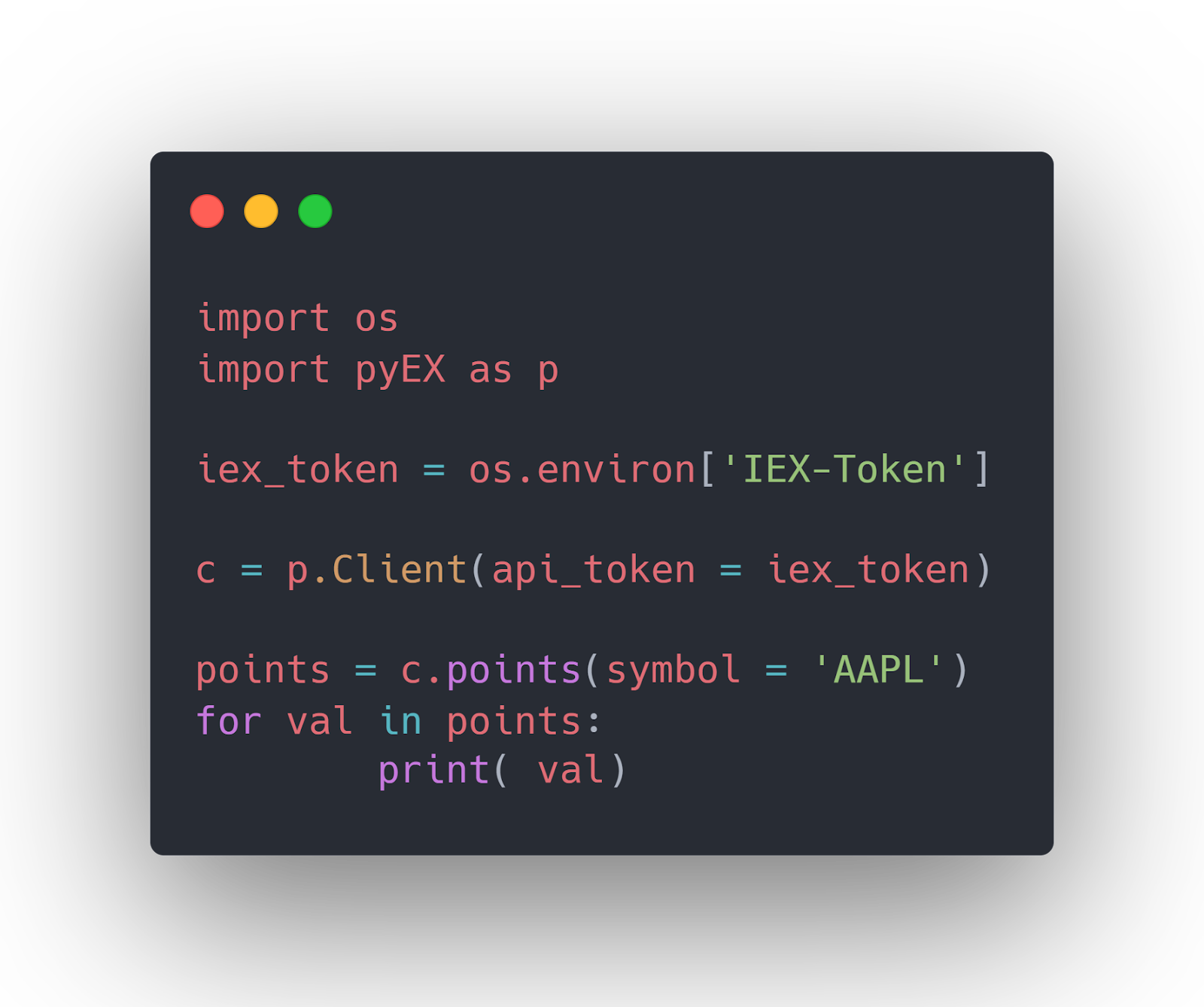
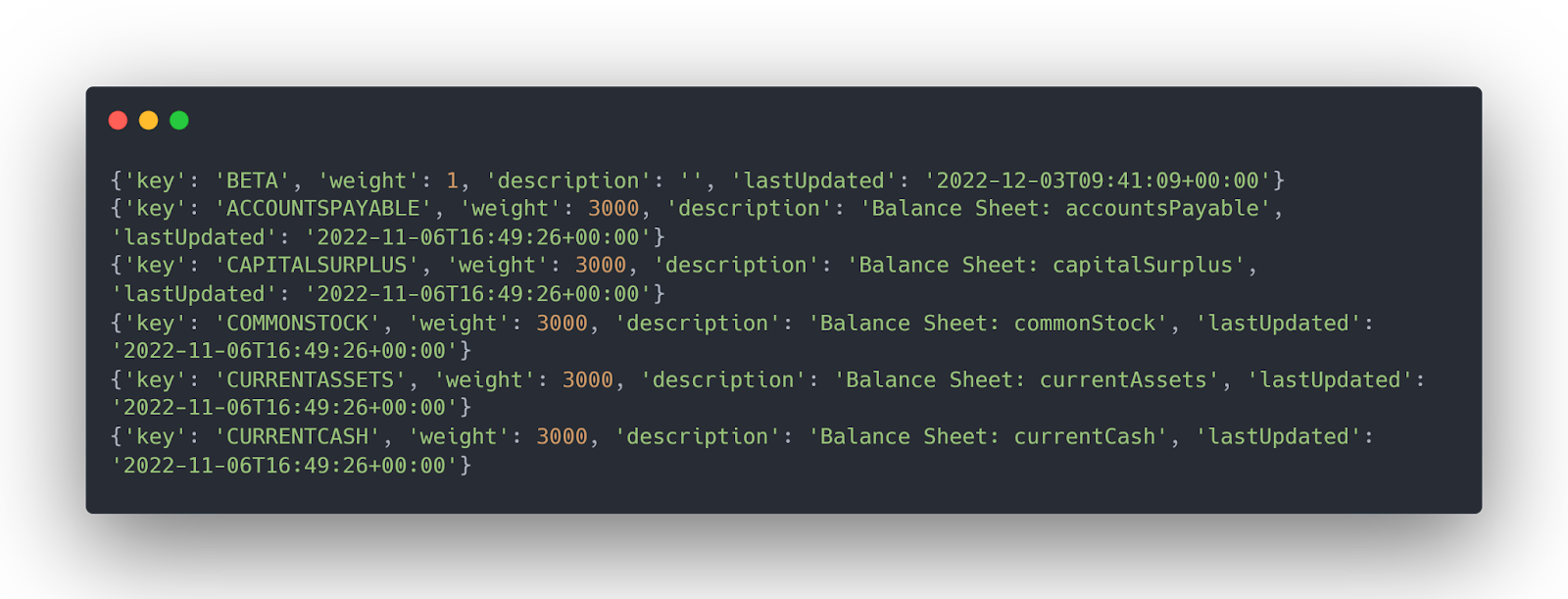
Time Series Endpoint
The time series endpoint allows you to retrieve data over a period of time. Using the time series data, you can plot line charts, candlesticks, and other visualizations.
Get Time Series Data
The timeseries() method returns time series data for a stock that you pass to the key attribute of the method. You must pass the data set id to the timeseries() method. For example, “REPORTED_FINANCIALS.” You can specify the number of time series data points to retrieve using the last or range attributes.
For instance, the following script returns Apple Inc’s last 20 reported financials.
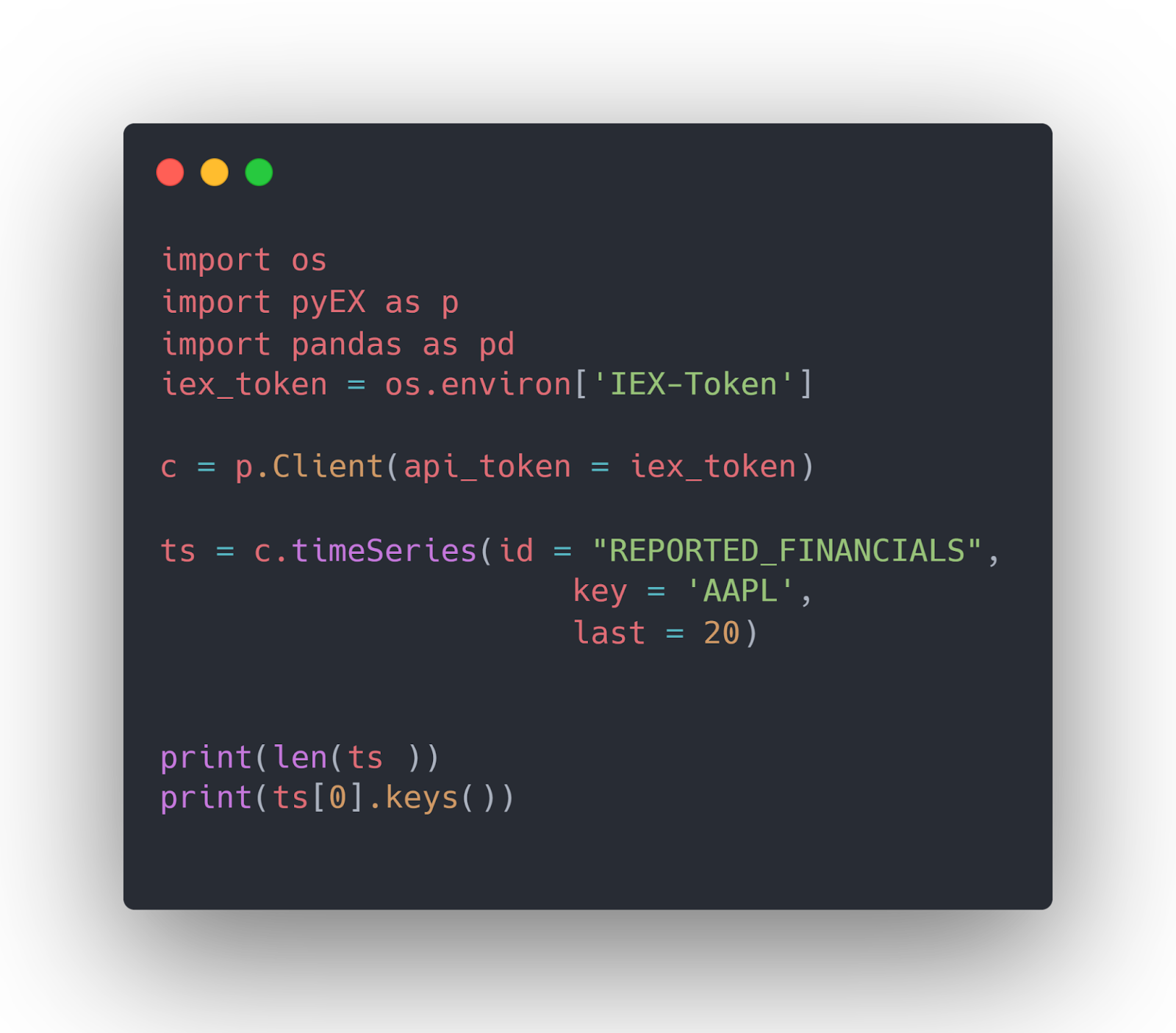
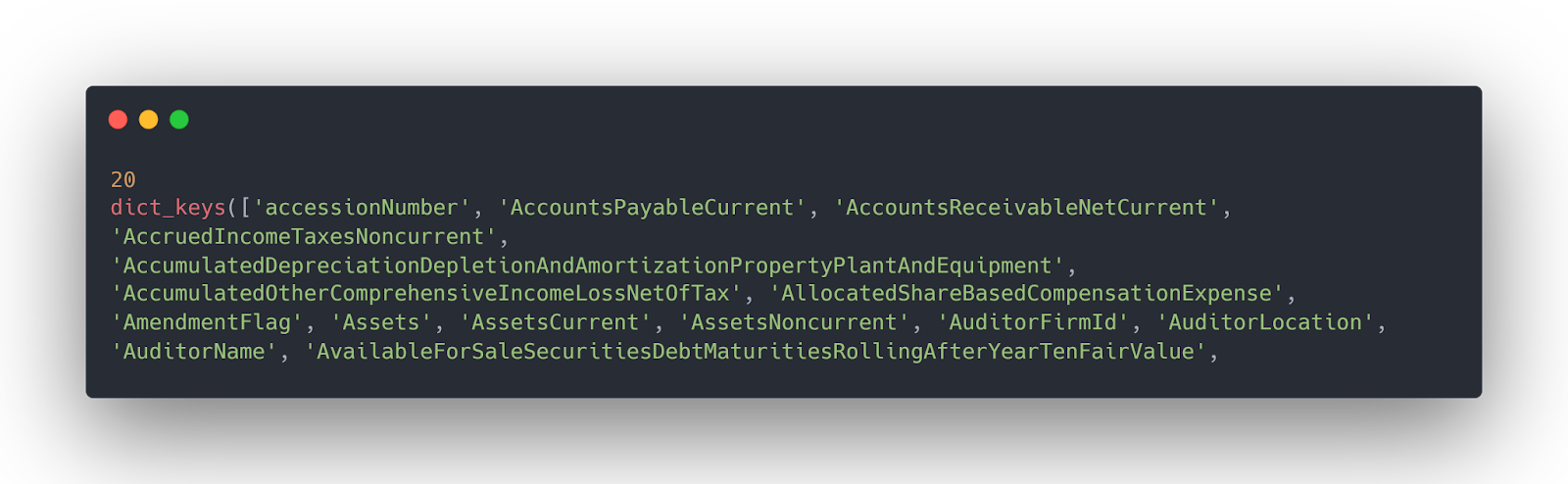
For the details of various range options, see the IEX time series documentation.
The following script demonstrates how to plot a line chart using the time series data from the IEX Cloud time series endpoint.
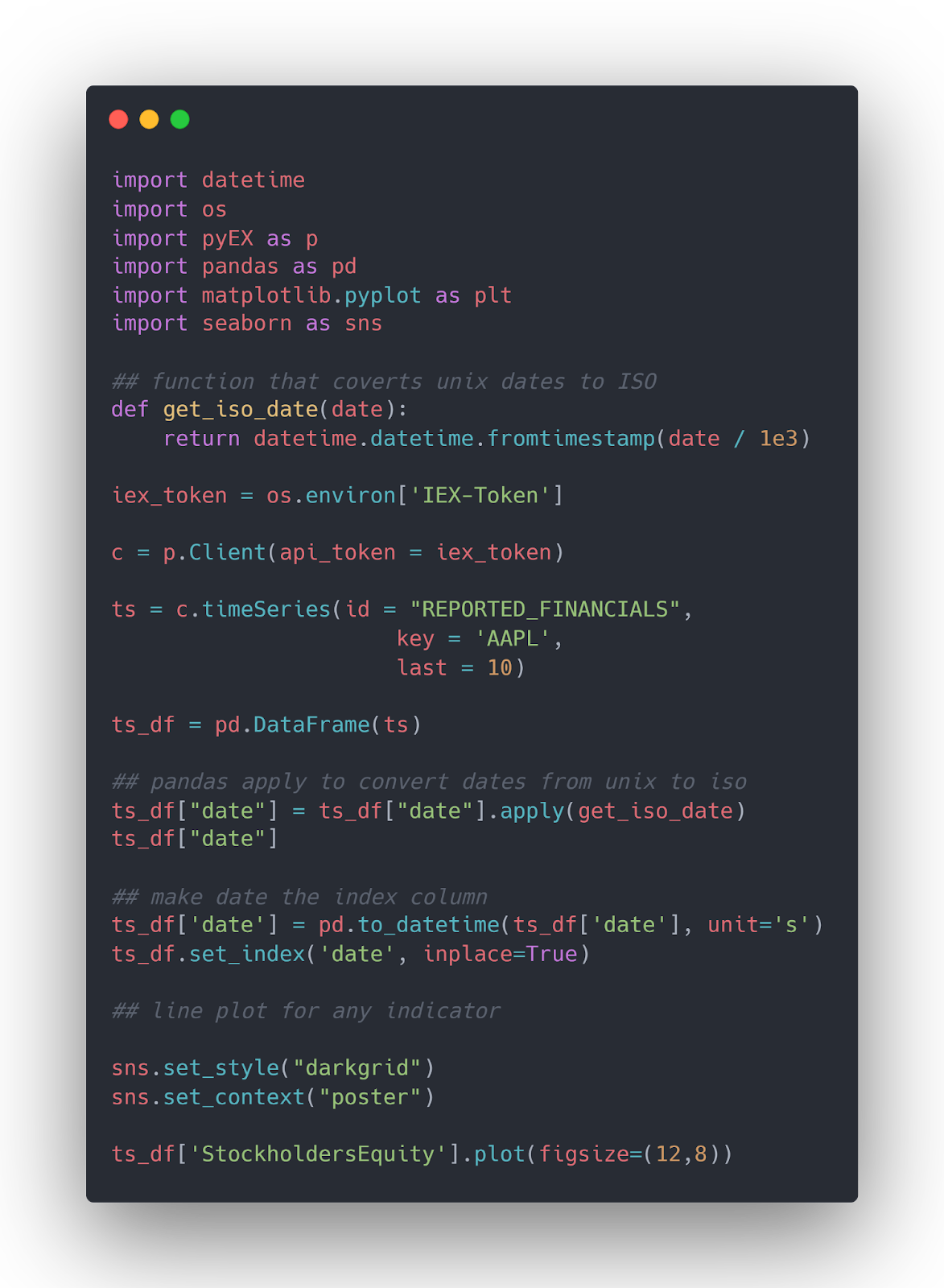
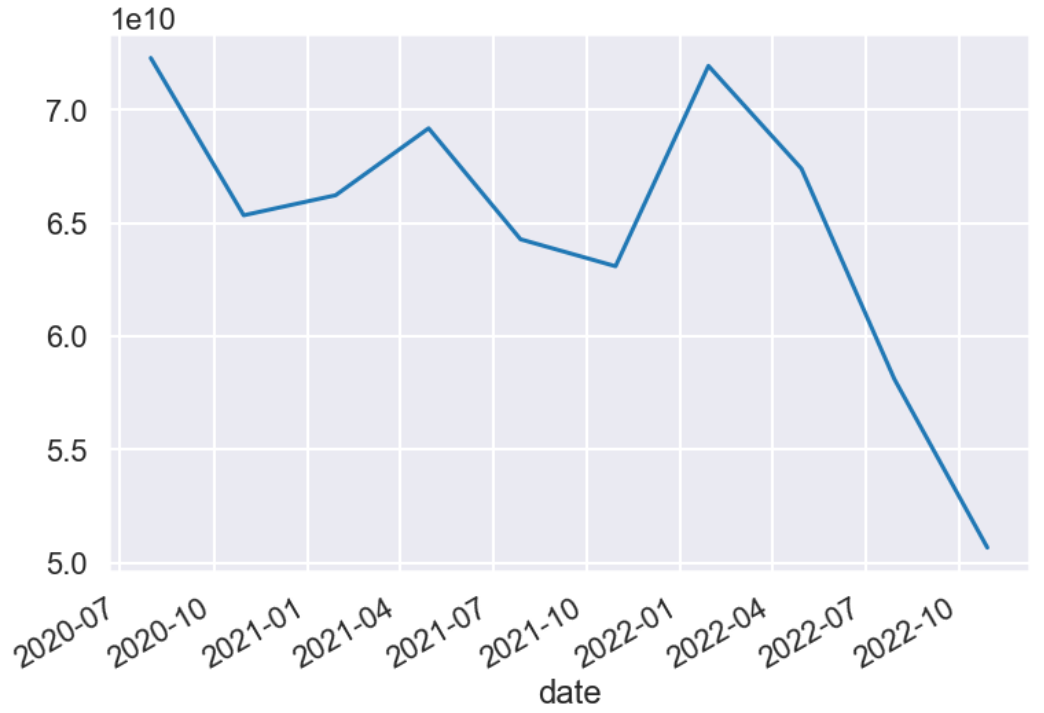
I couldn’t find any function in the documentation to list all financial ids for a stock. One way to do this is to call timeseries() method without any id. The method returns all the ids for a symbol. You can then use the “id” key of the response dictionary to see all id values.
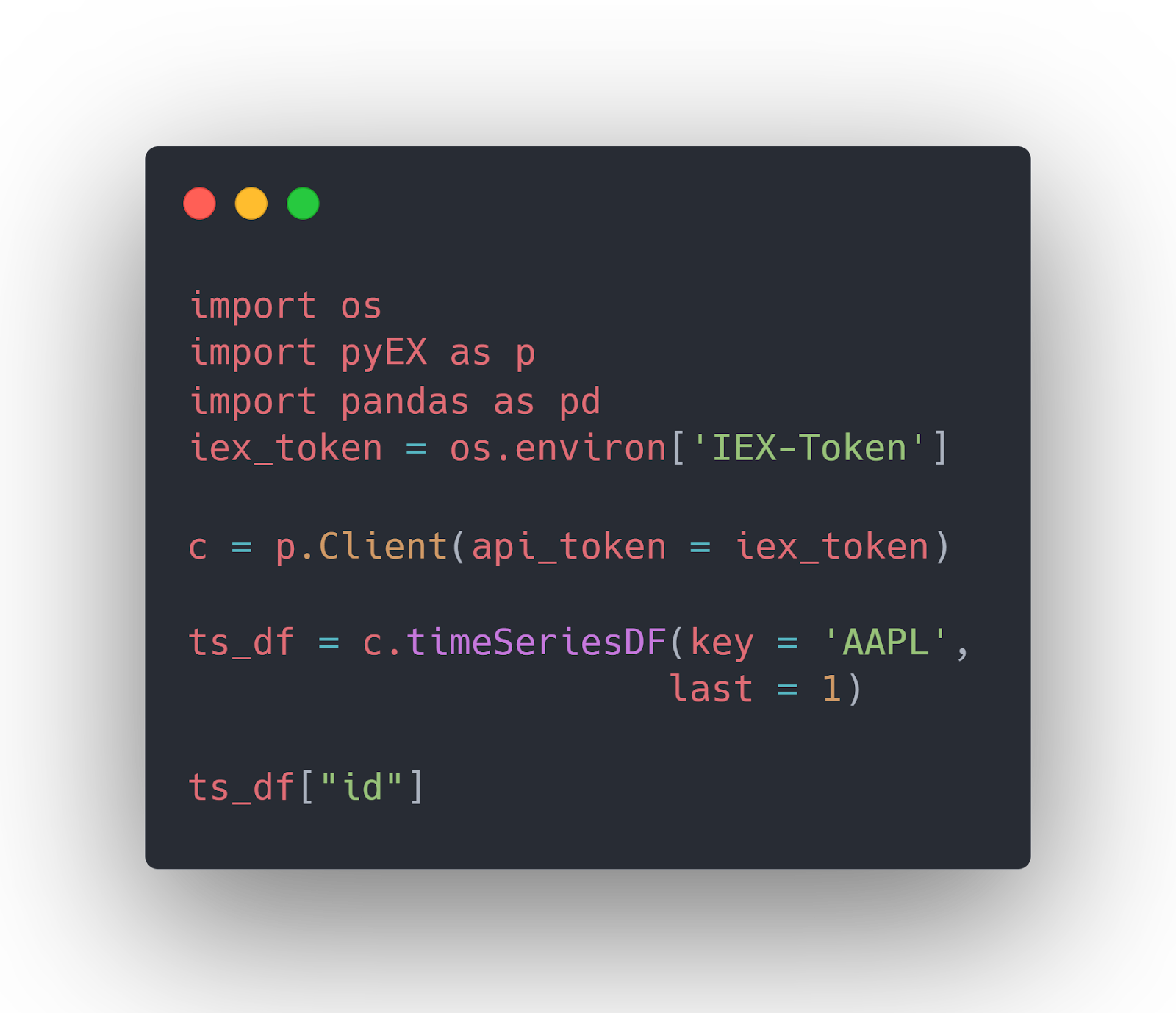
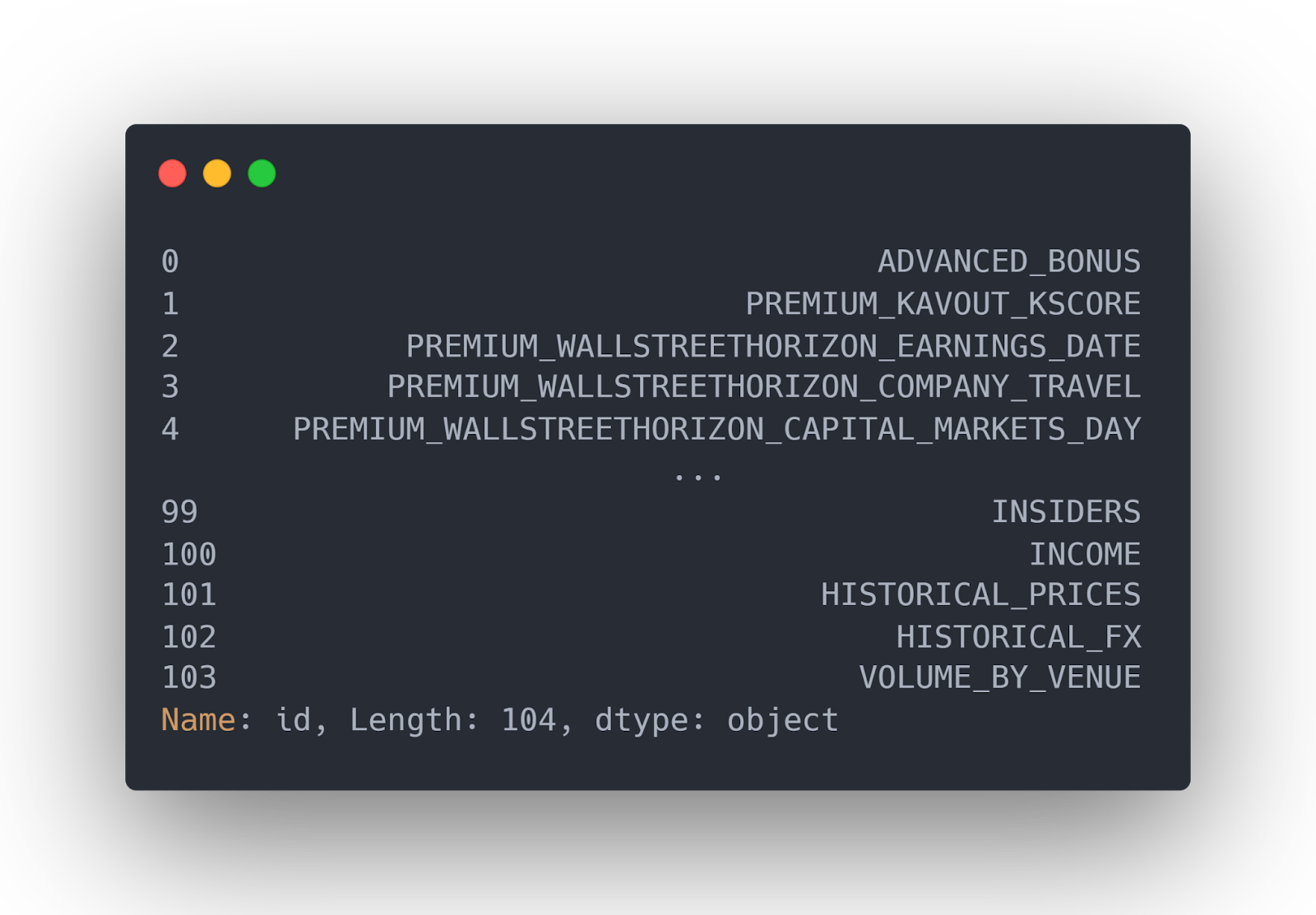
Rates Endpoint
The rates endpoint contains functions to return credit card interest and certificate of deposit (CD) rates.
Get Credit Card Interest Rates
The creditcard() method returns commercial banks’ credit interest rates.
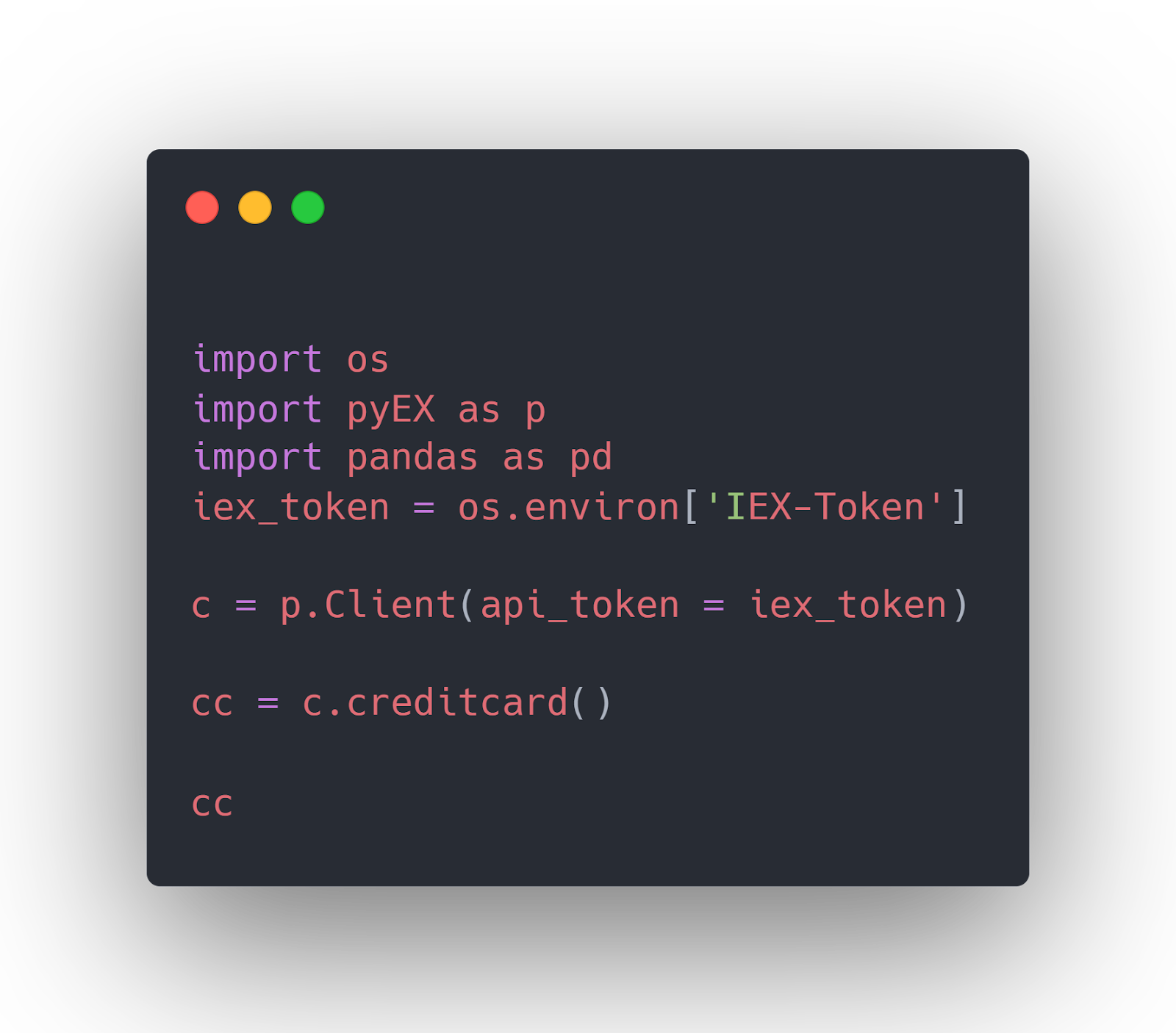
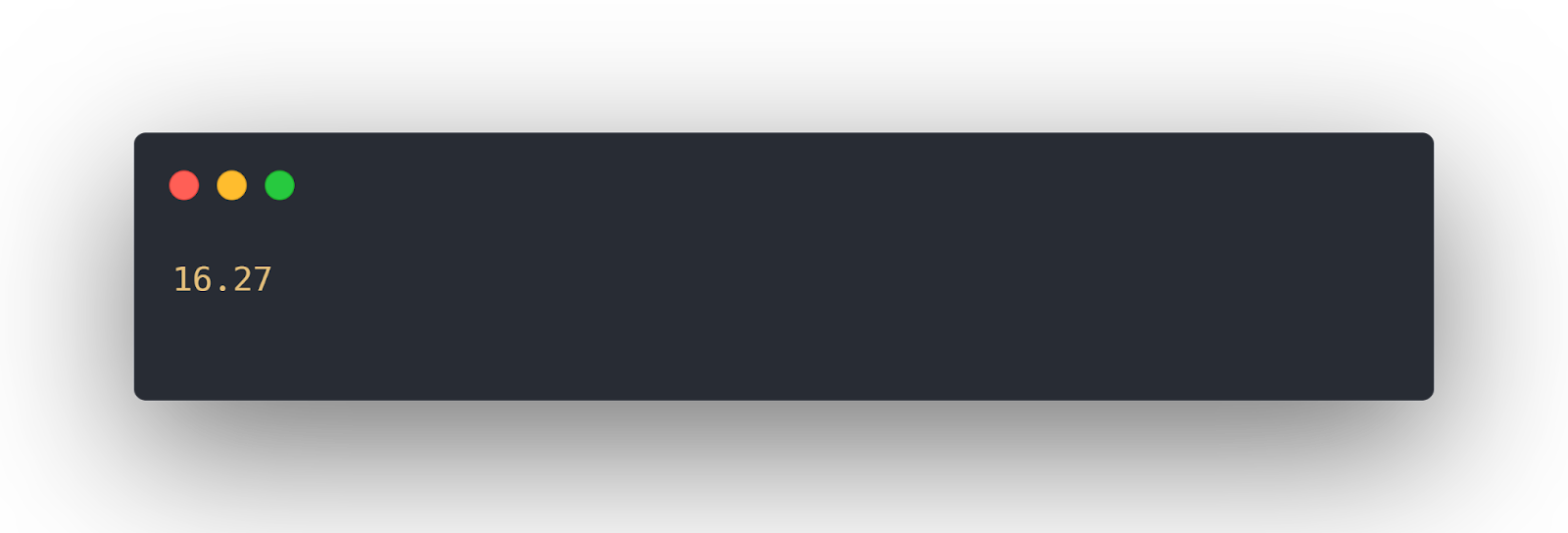
Stocks Endpoint
The stocks endpoint is one of the most valuable endpoints from IEX Cloud and allows you obtain data for current and historical stock prices, balance sheets, cash flow, dividends, etc.
Get Historical Stock Prices
The chartDF() method returns historical and intraday prices for a stock in a Pandas DataFrame. You must pass the stock symbol to the symbol attribute.
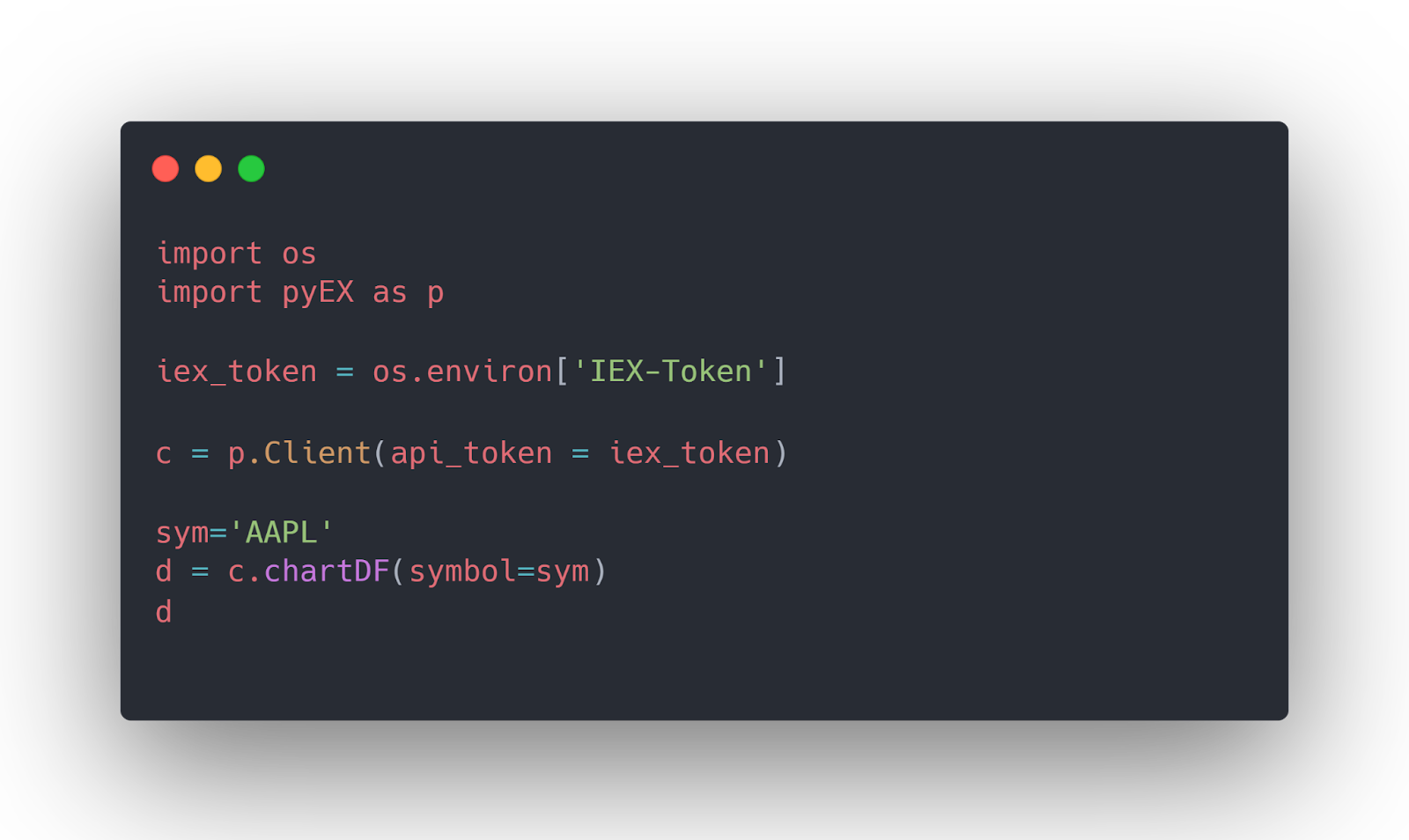
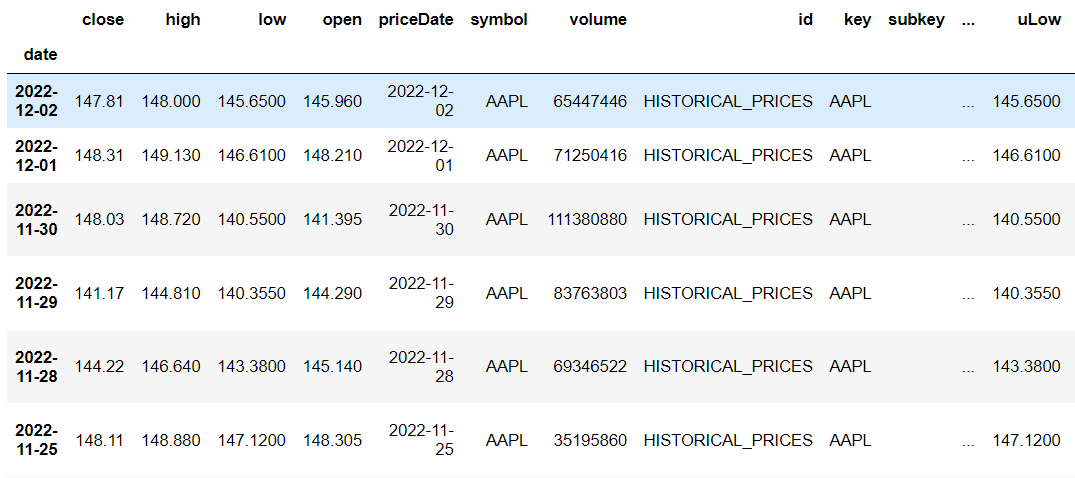
You can obtain historical data for various time ranges. See the official documentation for all range values.
The timeseries() method from the time series endpoint also returns historical stock prices. You must pass the “HISTORICAL_PRICES” as the value for the “id” attribute.
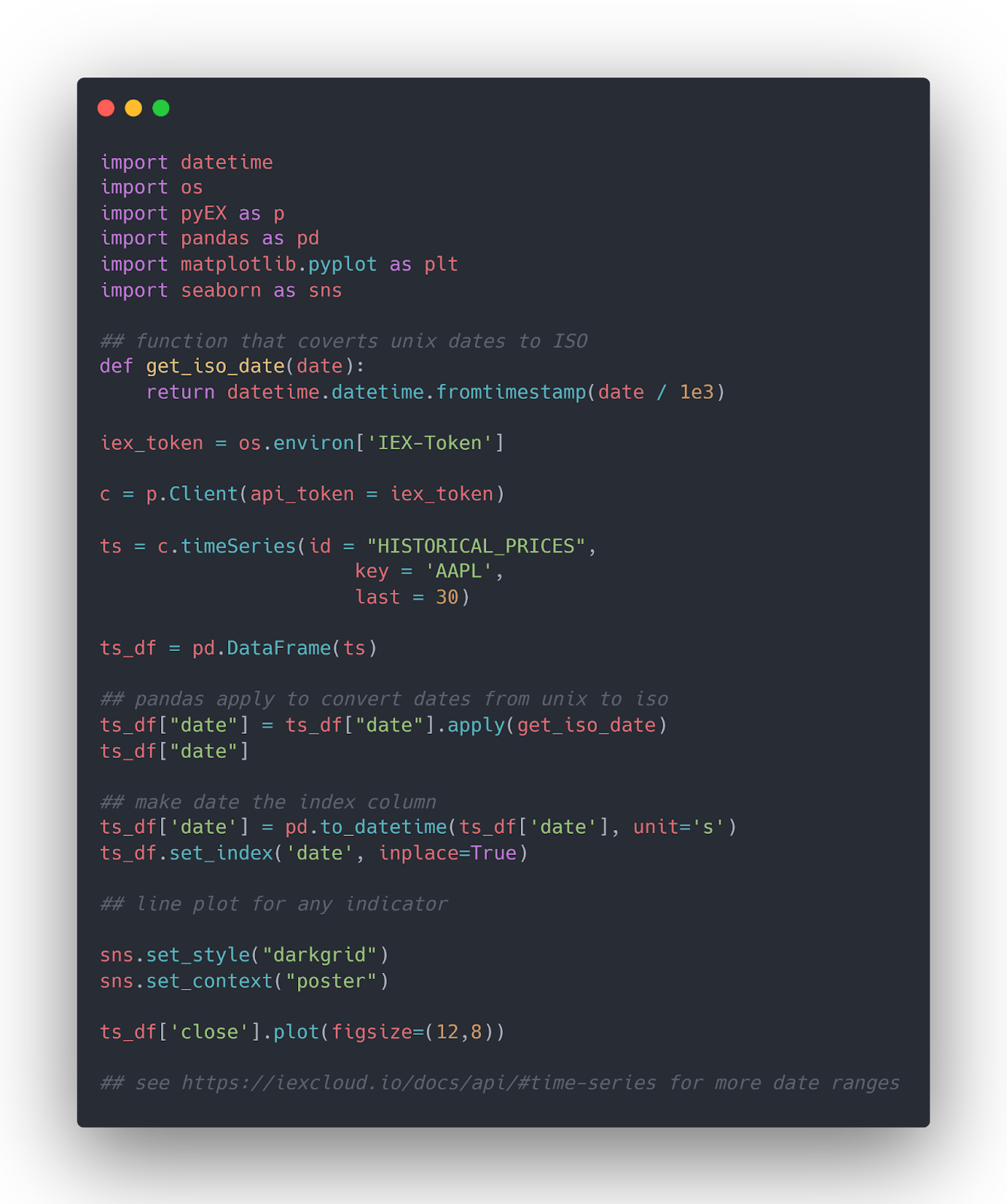
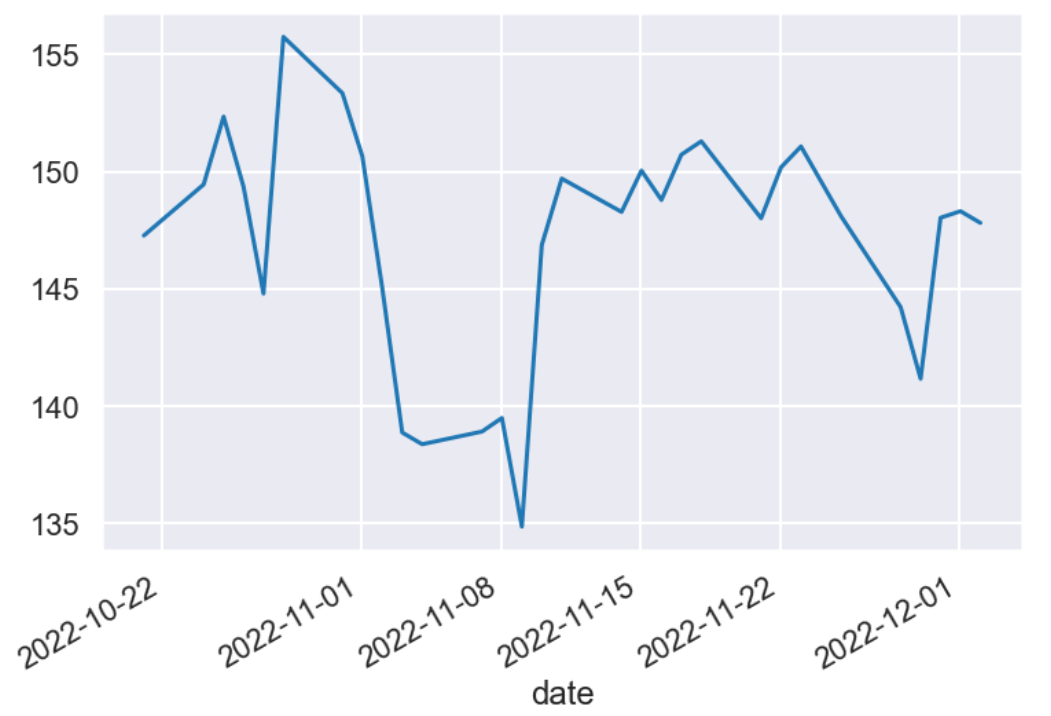
Get Stocks Fundamentals
The fundamentals() method returns a company’s stock fundamentals as reported in a company’s financial statement, income statement, cash flow statement, balance sheet, etc. This method is not available with the free tier account.
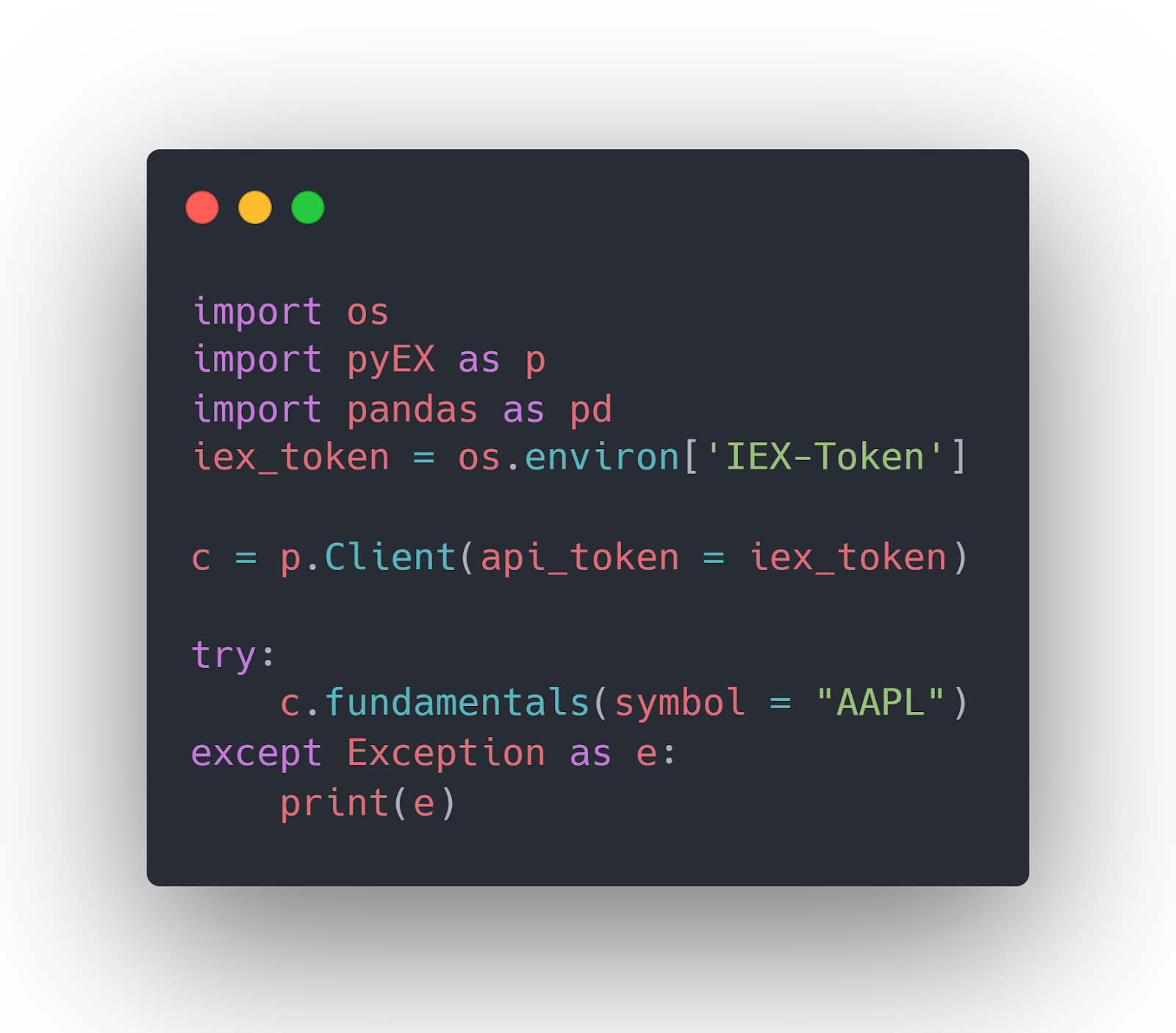
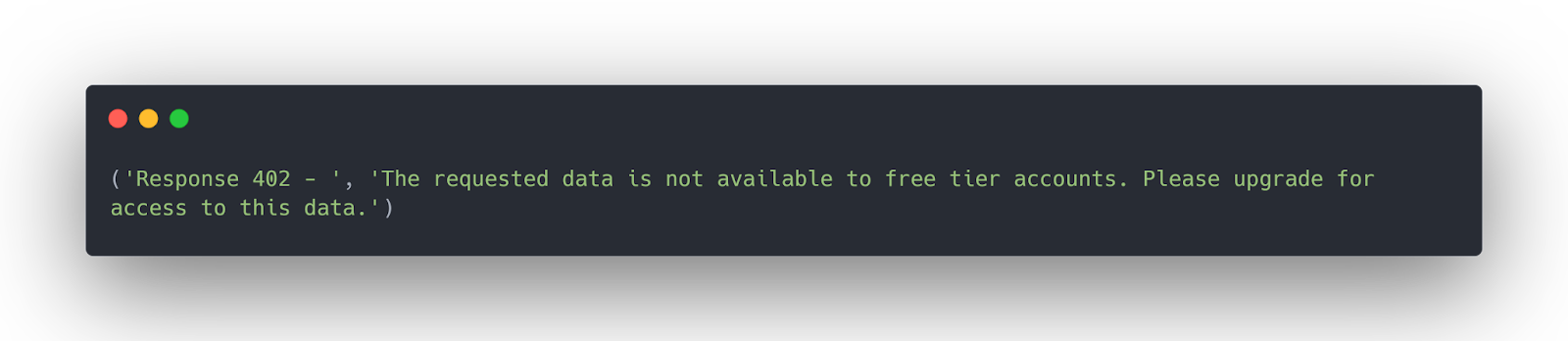
Get Stocks Balance Sheet
The balanceSheet() method, as the name suggests, returns the balance sheet of a stock. The method is not accessible with the free tier account.
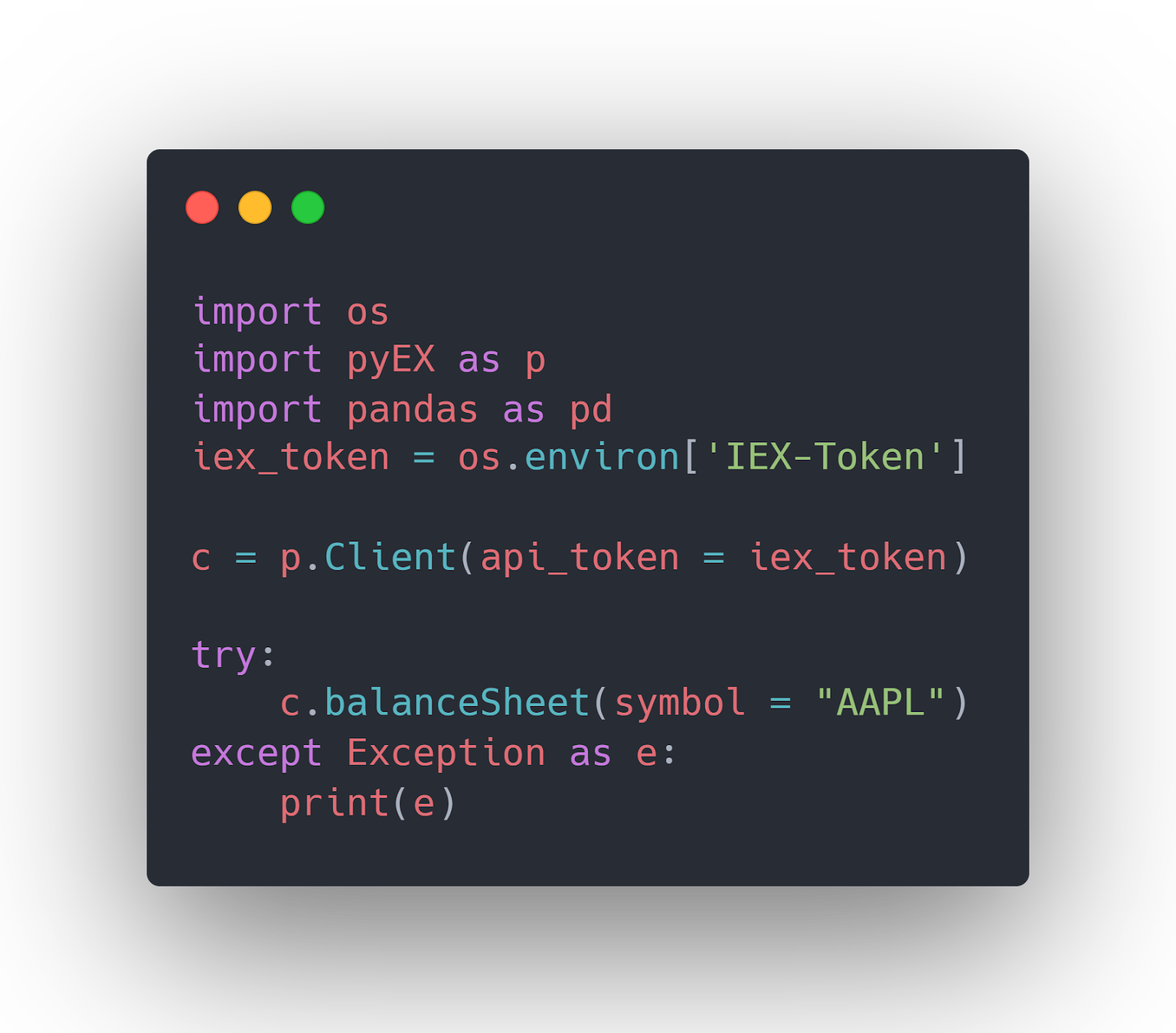
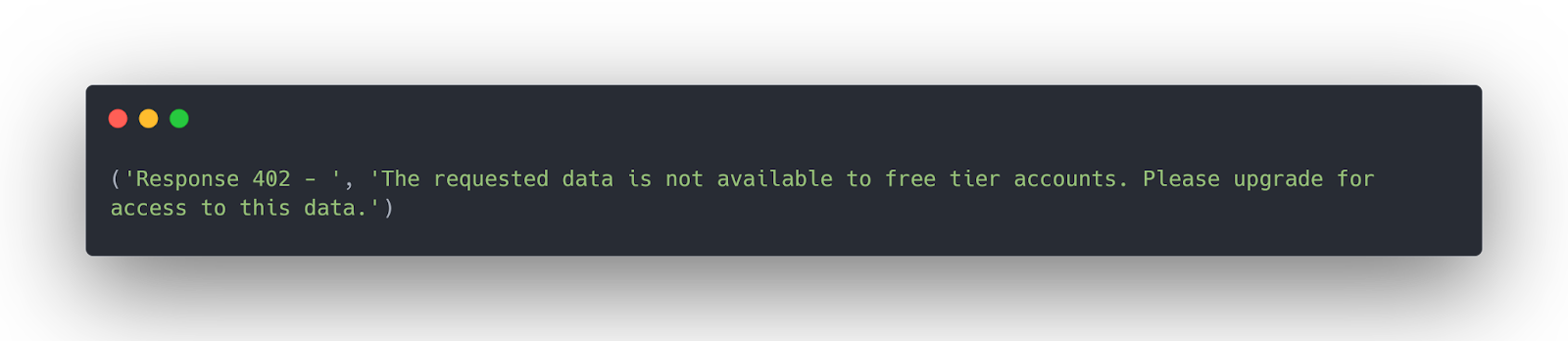
Get Stocks Cashflow
The cashFlow() method returns a company’s cashflow information. This method is also not accessible with the free tier account.
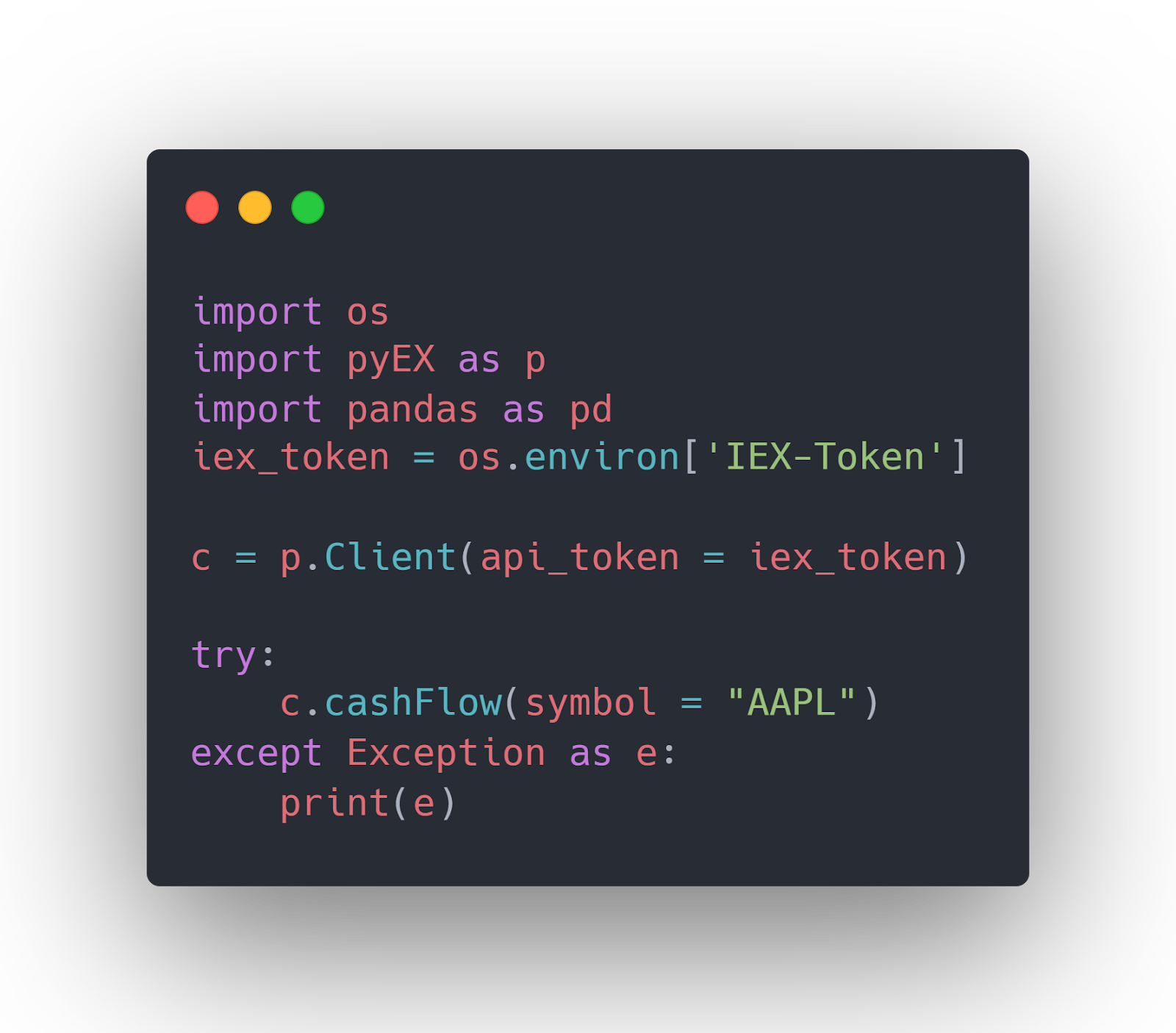
Get Stocks Dividends
You can retrieve a company’s dividends information via the dividendsDF() method. This method is only callable with the paid accounts.
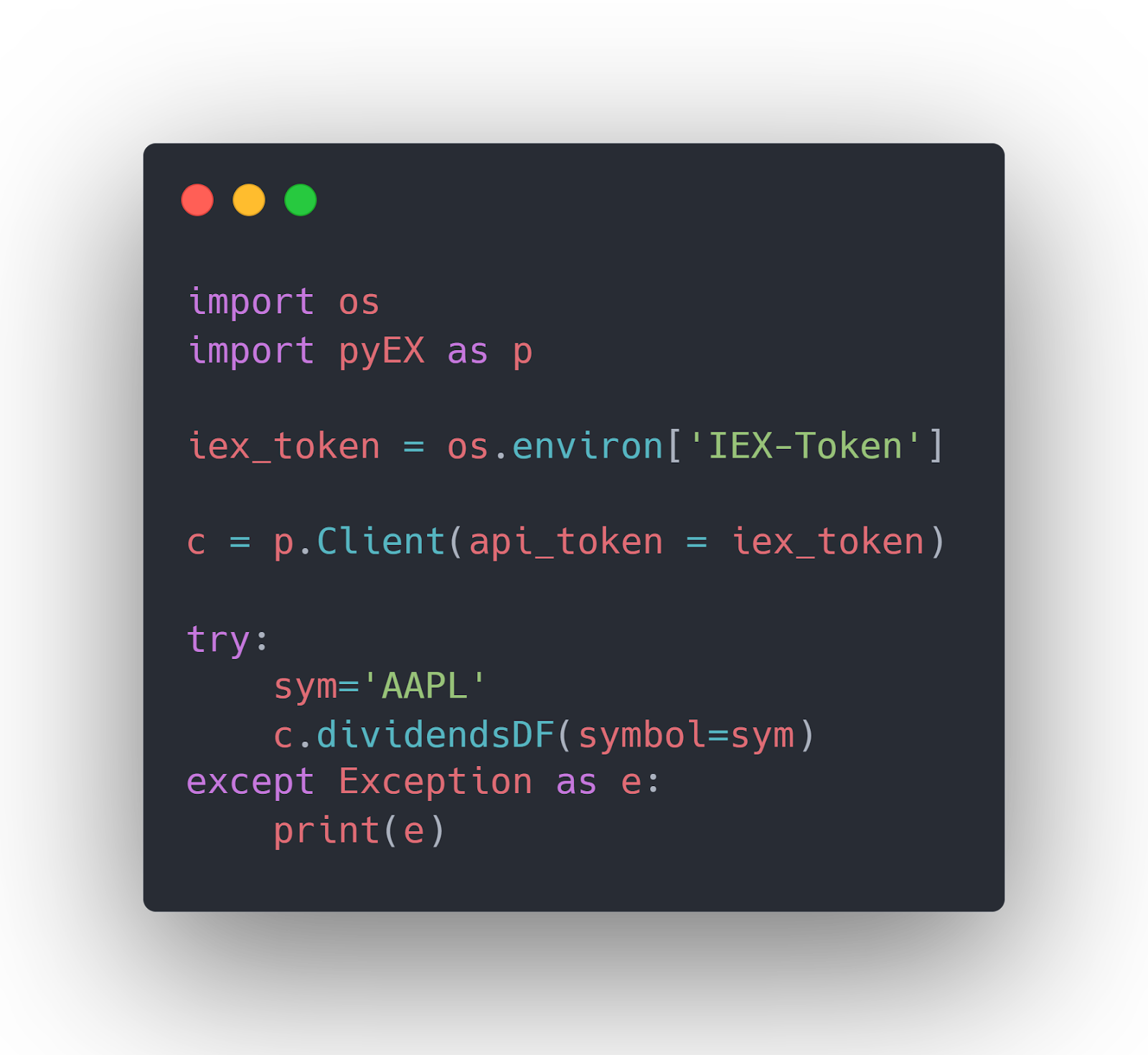
Get Stocks Quotes
The quote() method returns a company’s stock quote. The output shows the stock’s latest price, the trading volume, 52 weeks’ high and low values, percent change, etc.
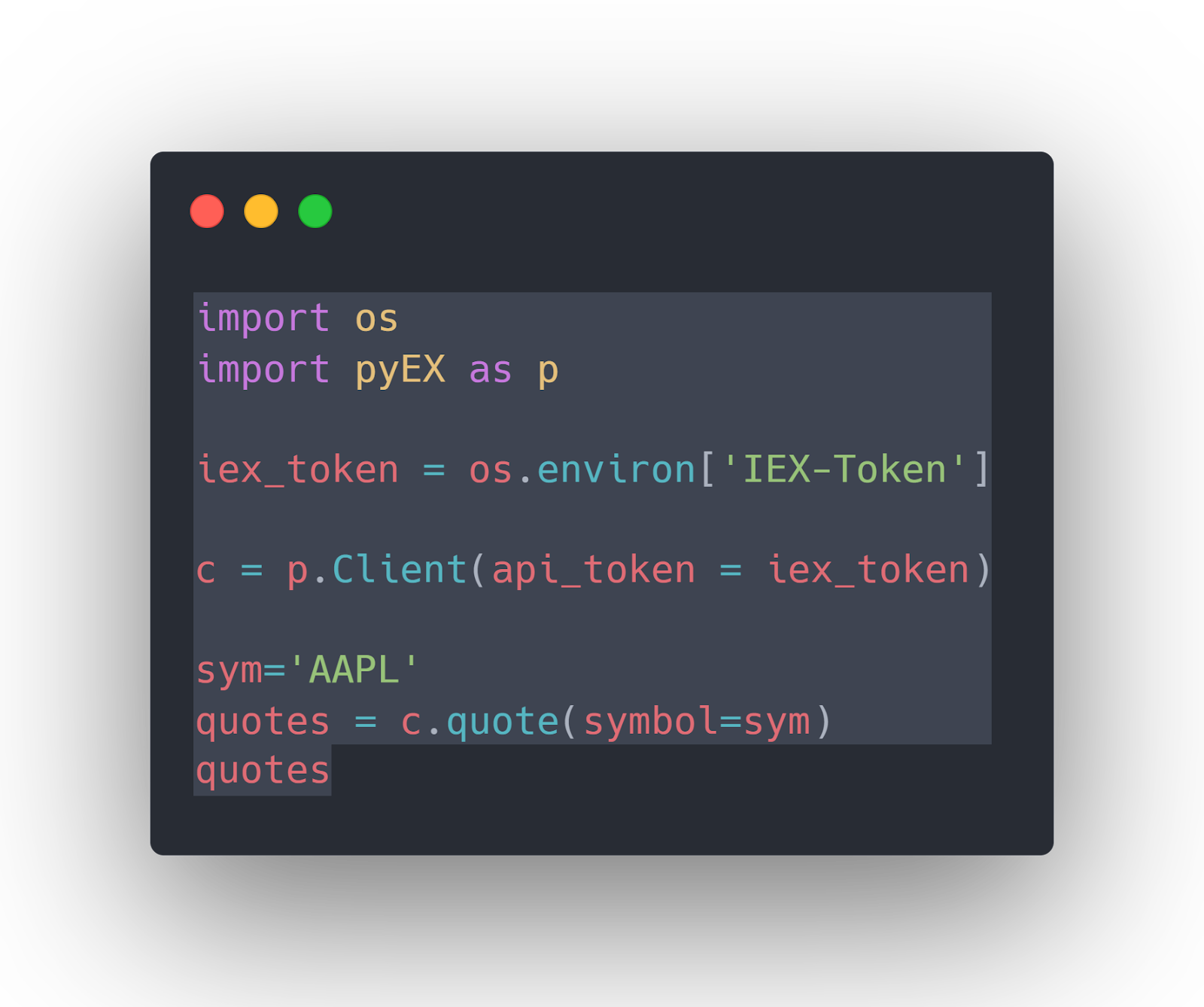
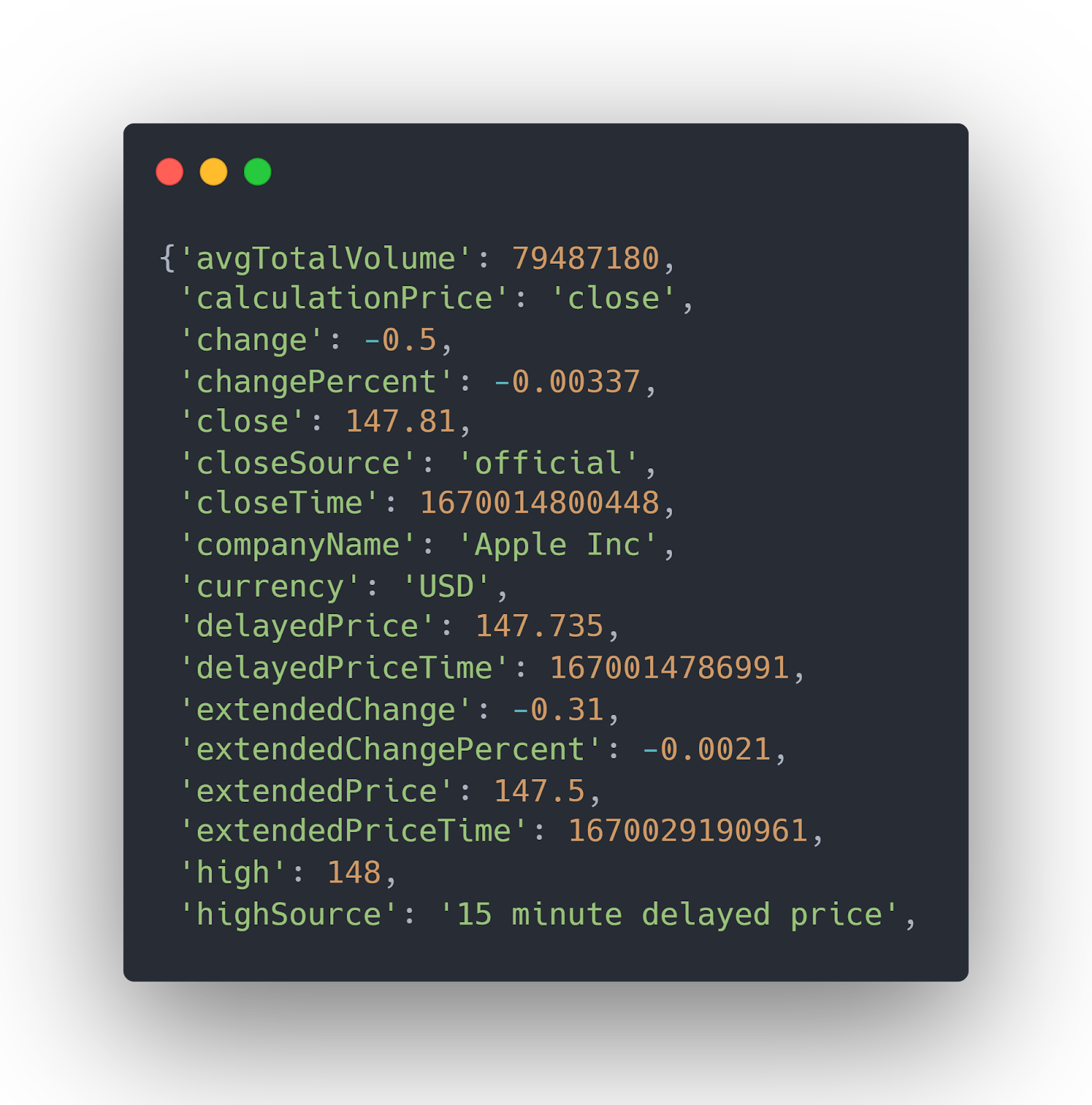
Get Stocks Technical Indicators
The technicals() method returns historical or intraday technical indicators for a stock. You need to pass the indicator id to the indicator attribute of the technicals() method. See the list of all technical indicator ids.
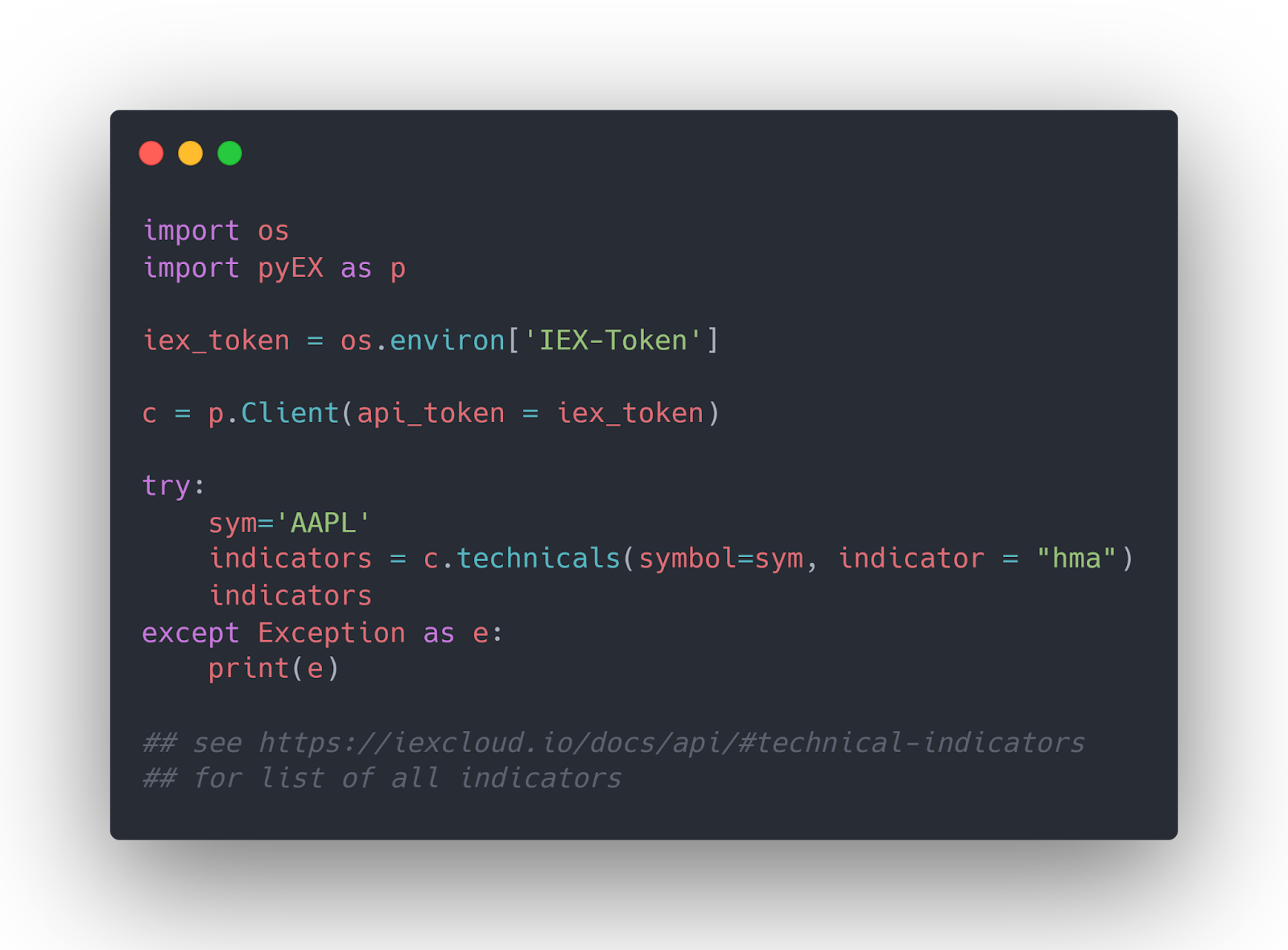
Get Stocks Stats
I could not find a function in the PyEX library that returns stock stats. You can use the stock/aapl/stats call with the requests library’s get() function. The response shows key company stats.
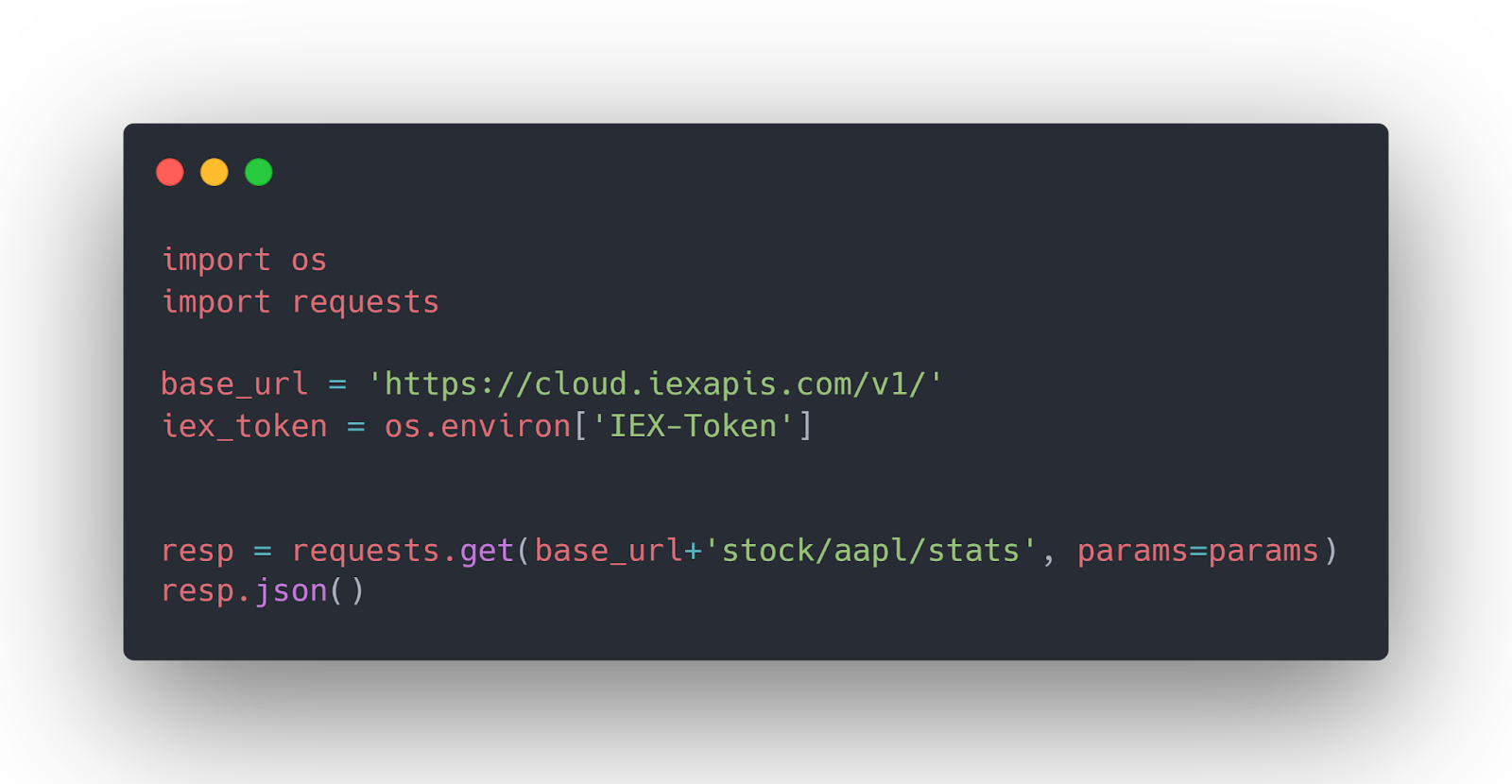
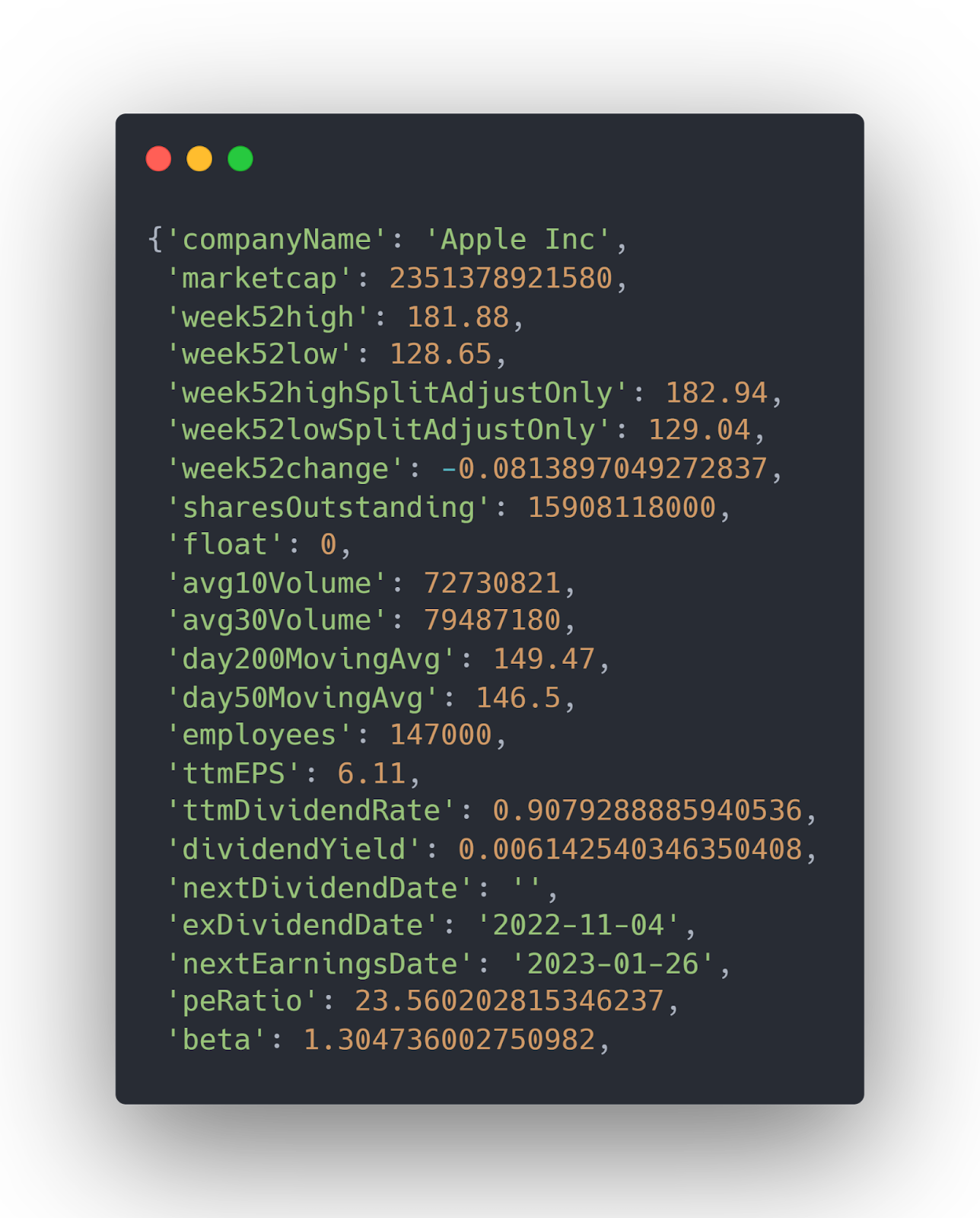
Commodities Endpoint
The commodities endpoint returns data such as oil, natural gas, and diesel prices. See the list of all commodities.
Get Oil Price
The wti() method returns crude oil west texas intermediate price in dollars per gallon.
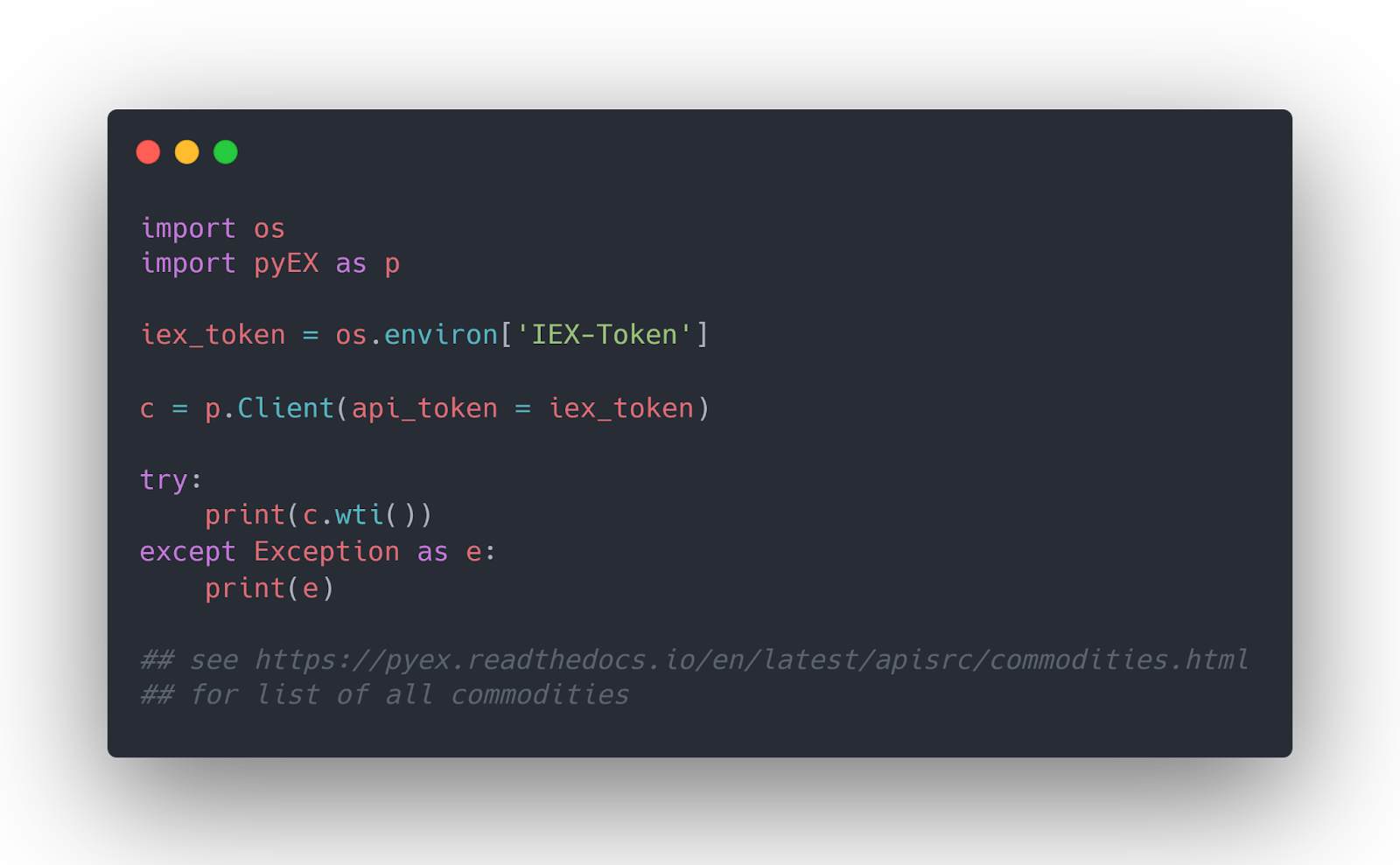
Get Natural Gas Price
The gasreg() method returns the US Regular conventional gas price in dollars per gallon.
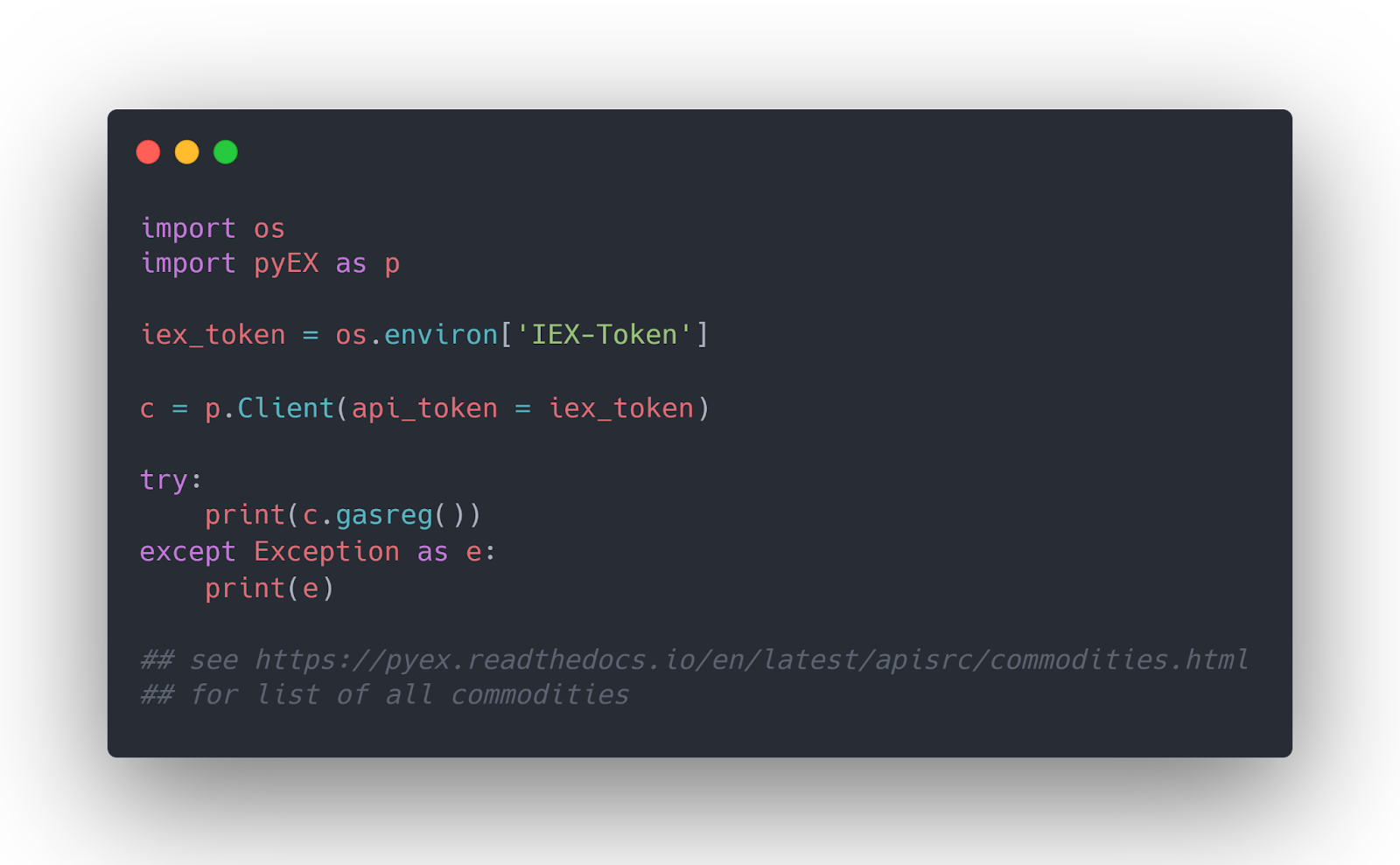
Get Diesel Price
The diesel() method retrieves the US diesel sales price in dollars per gallon.
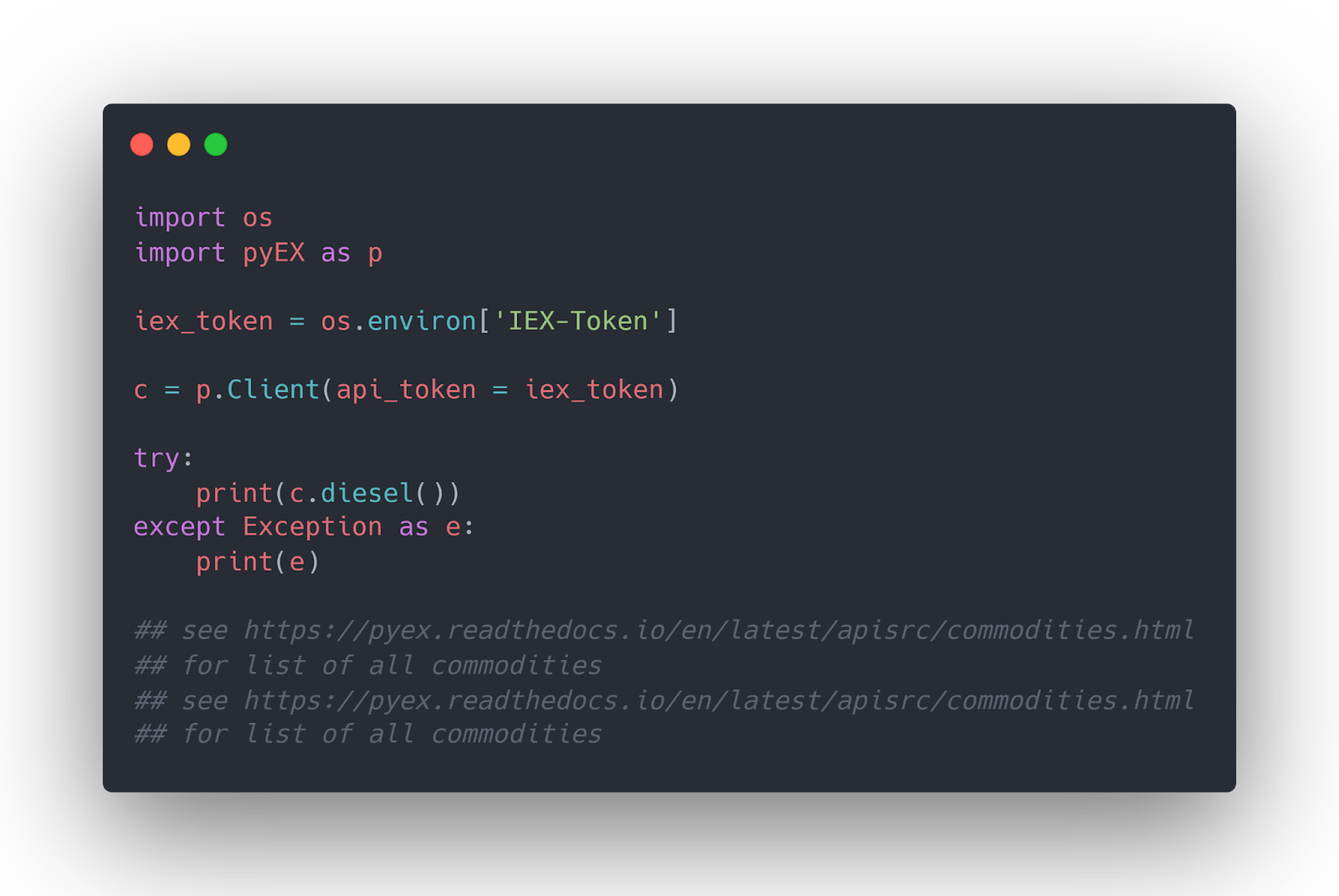
Economic Endpoint
The economic endpoint returns values for various economic indicators such as US GDP, recession probabilities, unemployment rate, etc. See the complete list.
Get Real Gross Domestic Product
The gdp() method returns US’s current gross GDP.
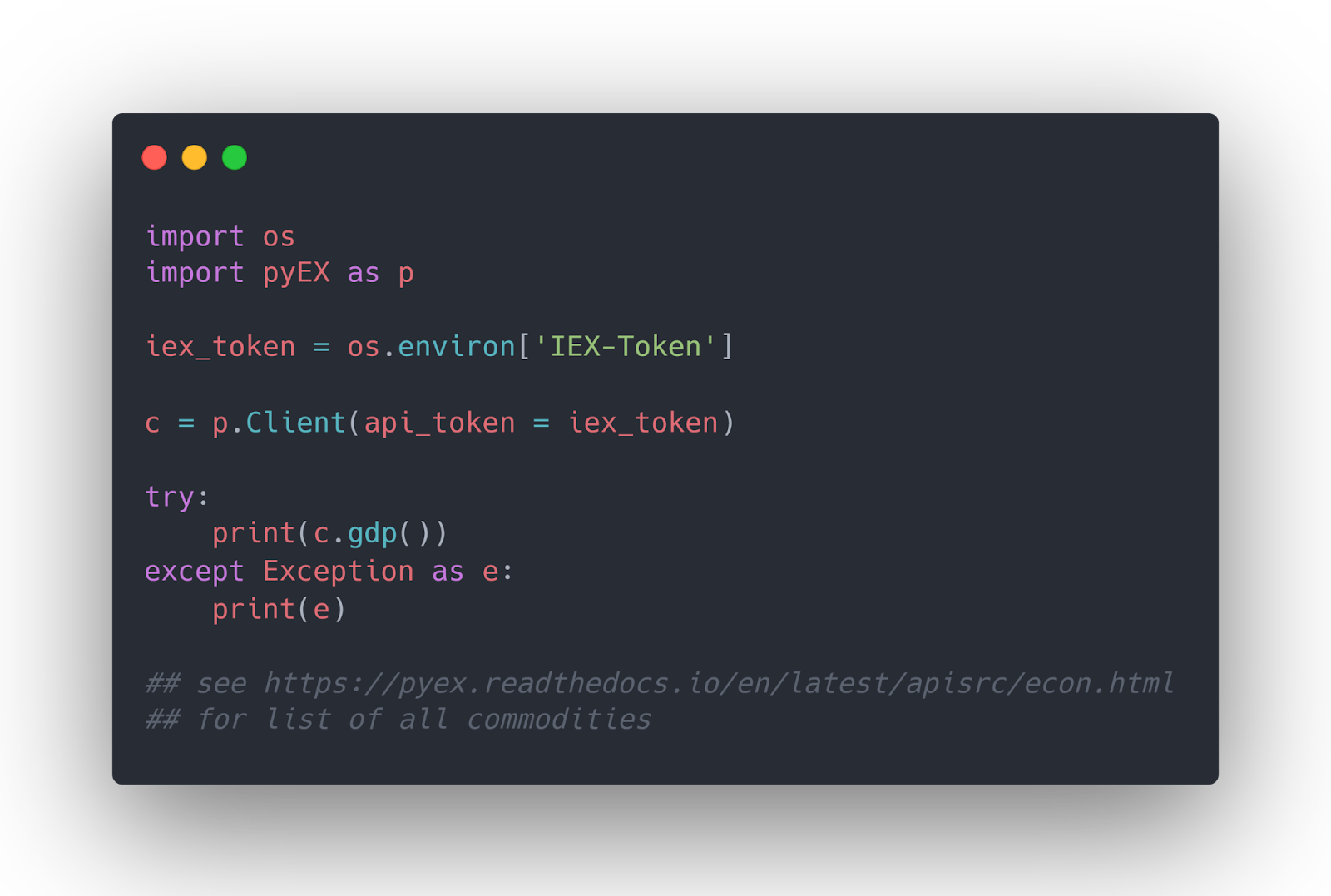
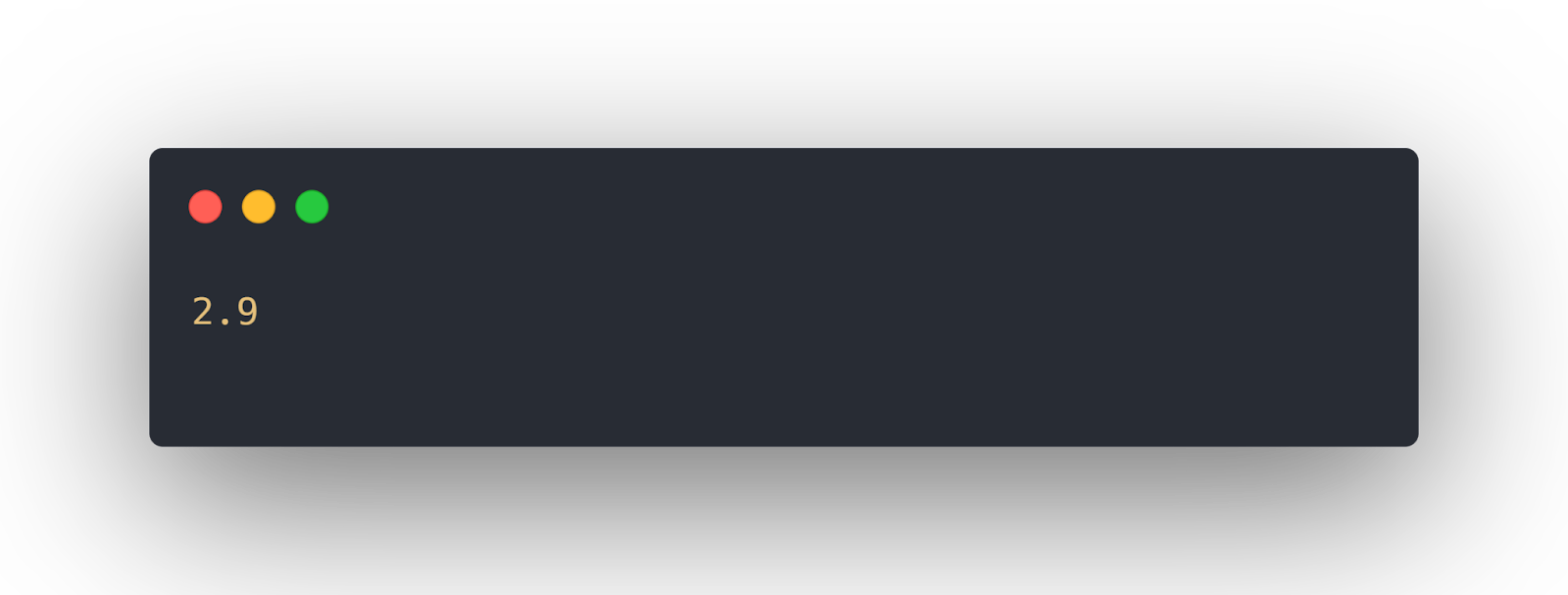
You can access time series data for economic indicators via the timeseries() method. You must pass “ECONOMIC” as the value for the id attribute, and the indicator Id to the key attribute. The indicator id for GDP is “A191RL1Q225SBEA”. See the official documentation for ids of other economic indicators.
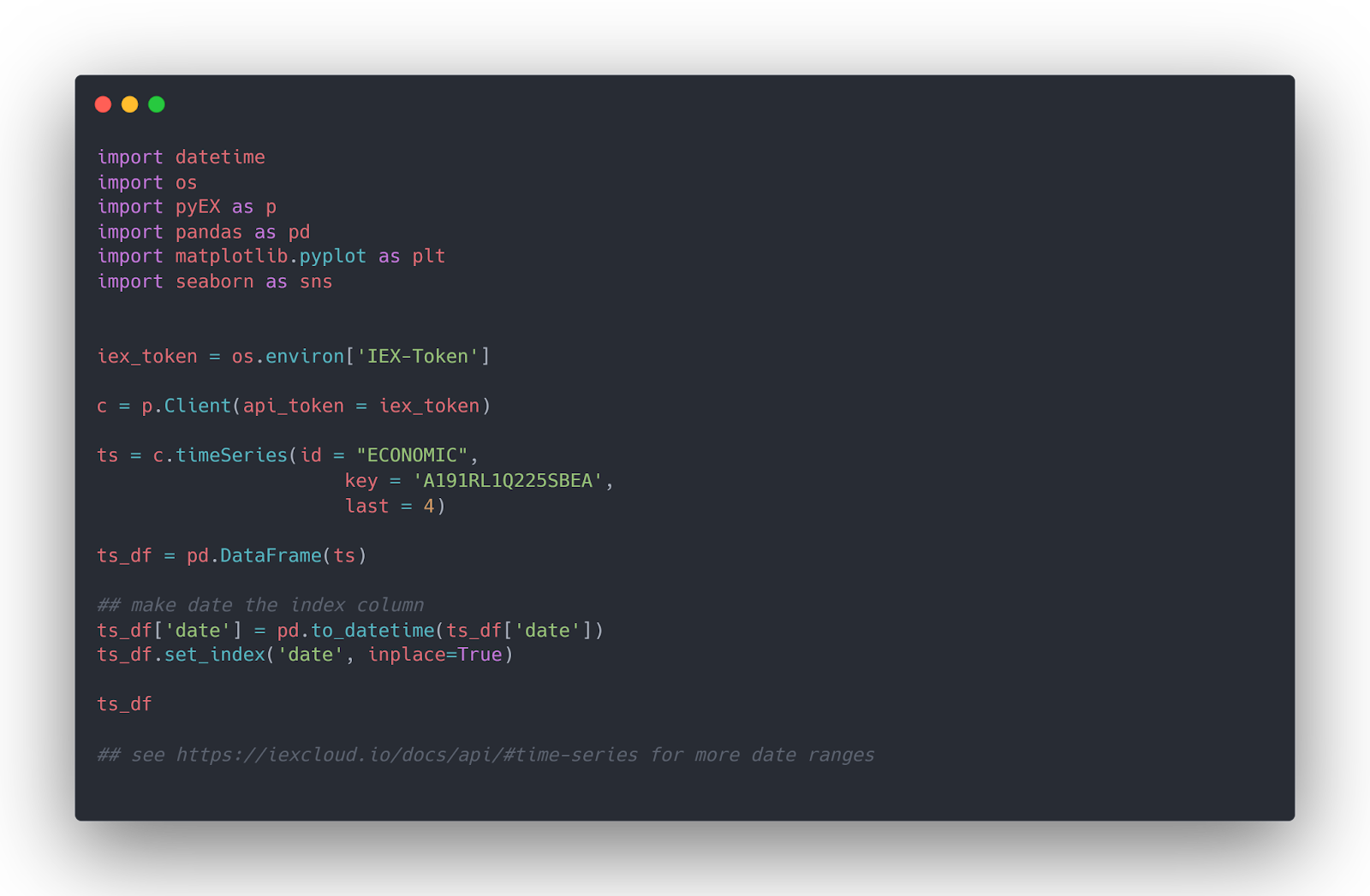
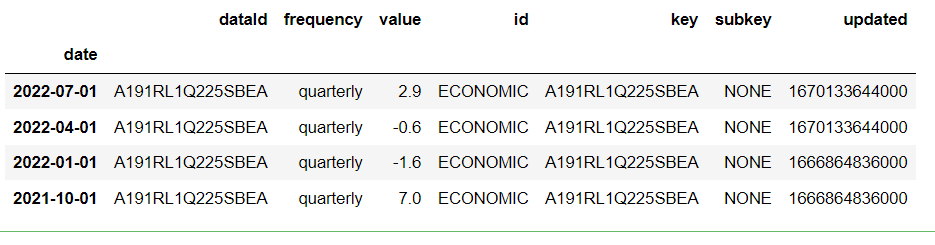
Get US Recession Probabilities
The recessionProb() method returns the current US recession probabilities.
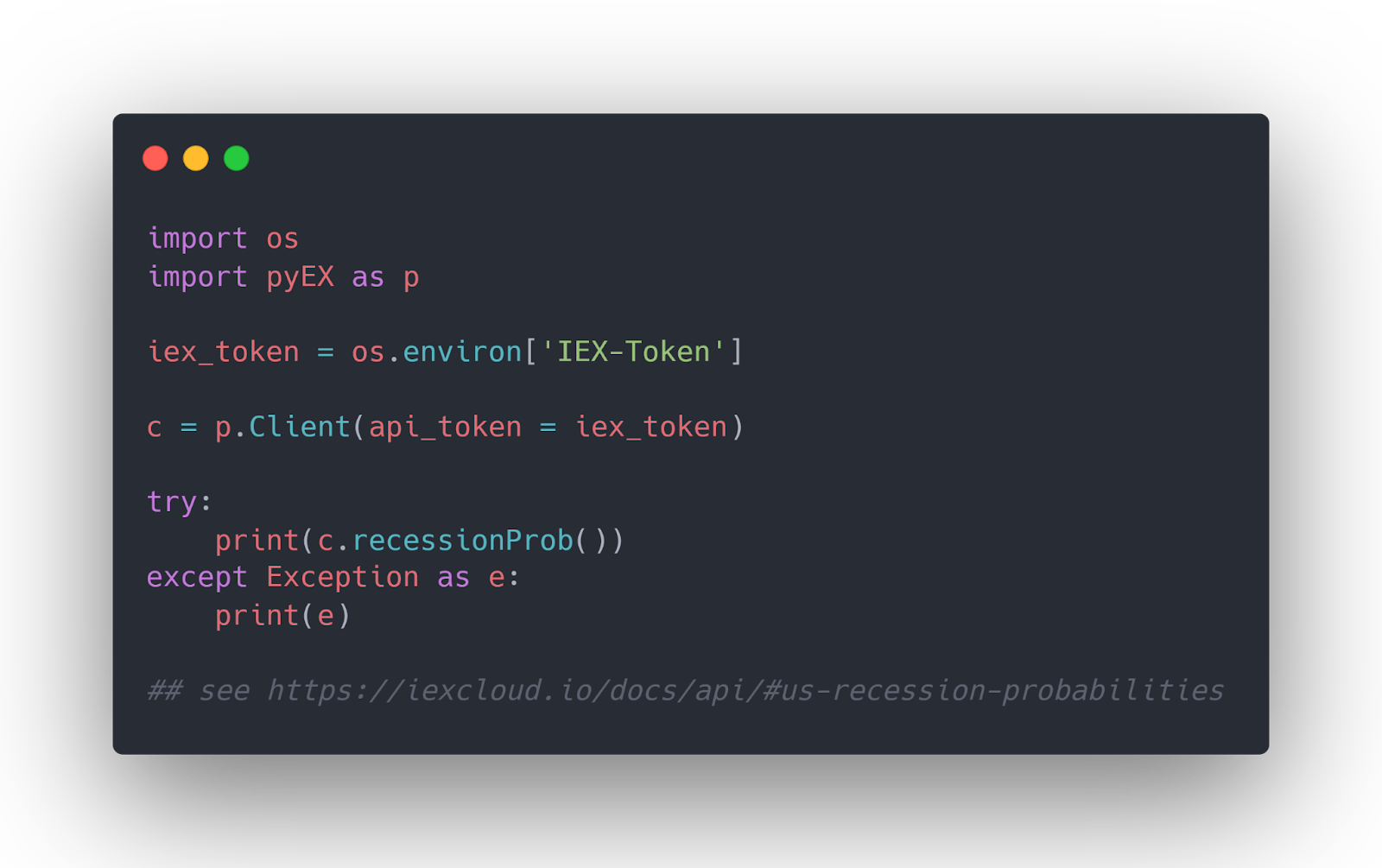
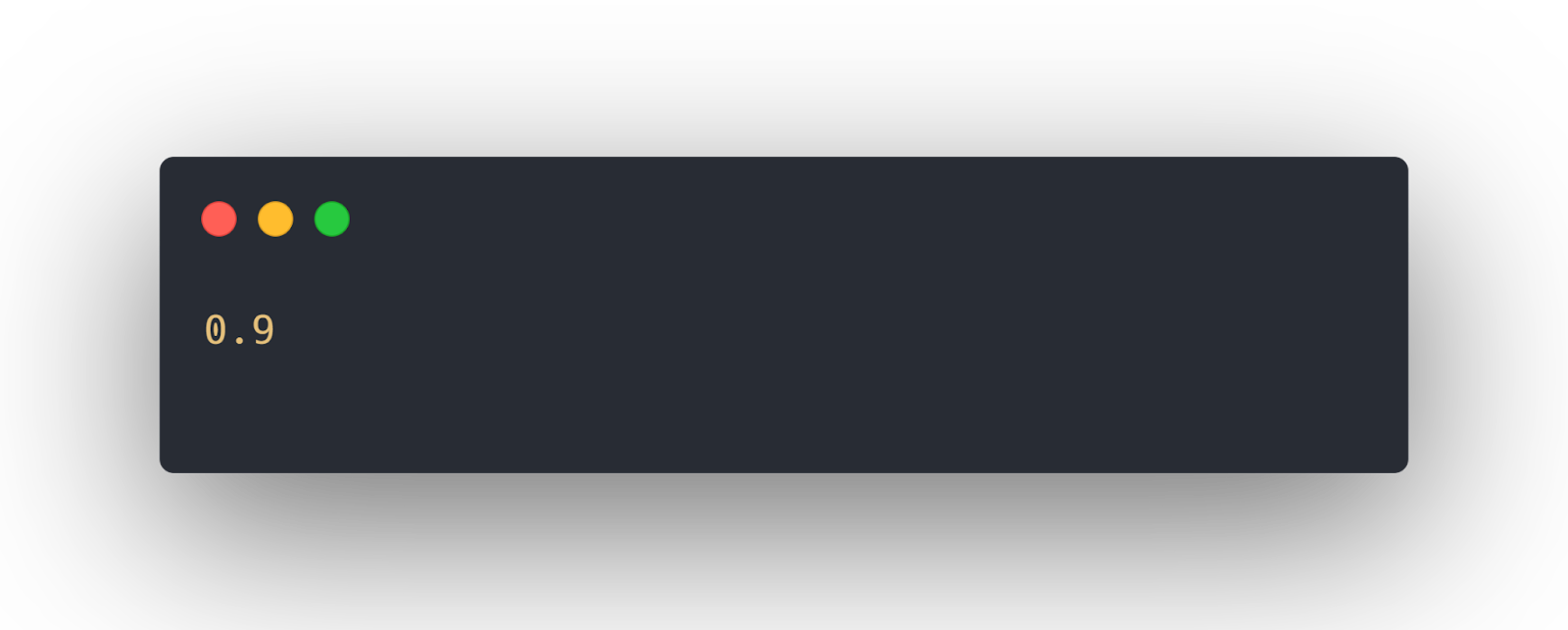
Get Unemployment Rate
The unemployment() method returns the current US unemployment rate.
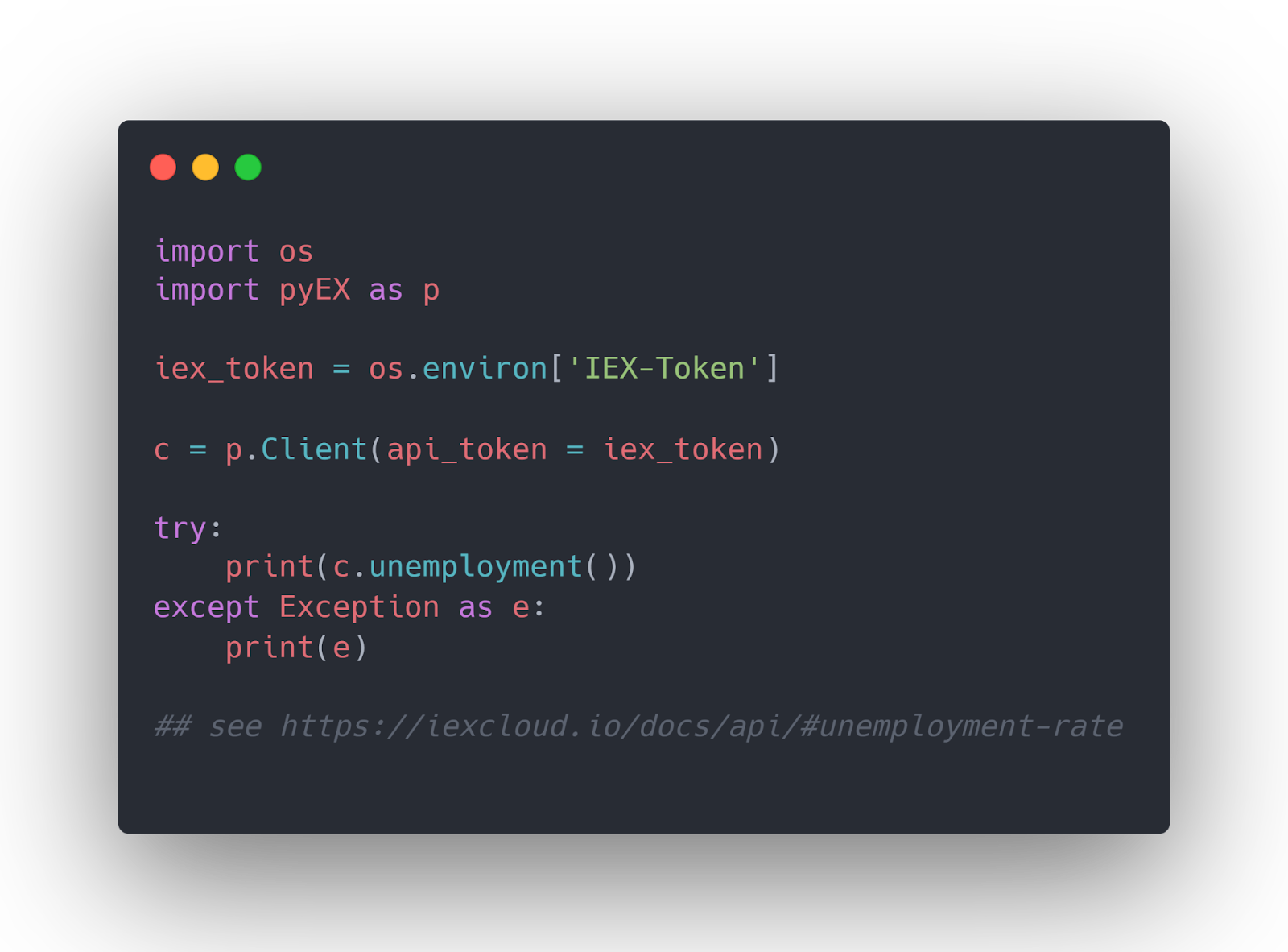
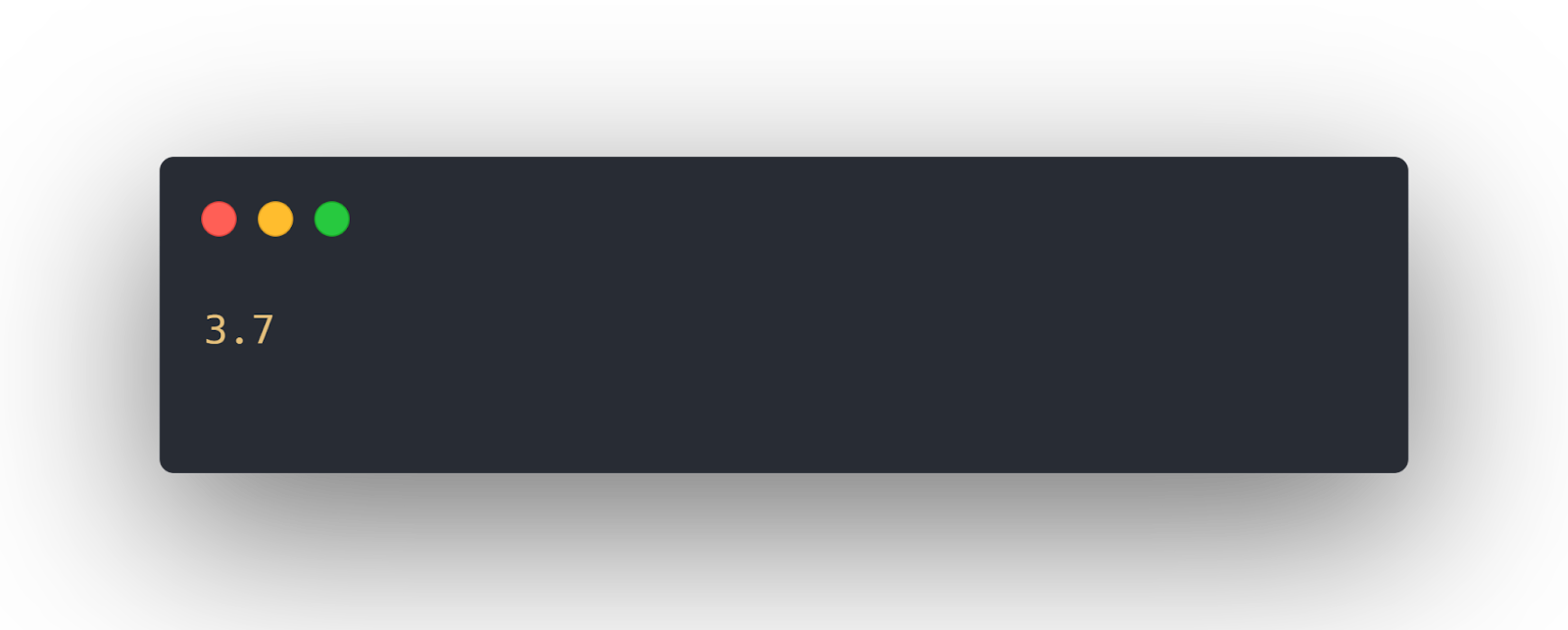
Account Endpoint
The account endpoint functions return information, e.g., current tier, payment status, credit quota usage, etc., about your IEX Cloud account.
The PyEX library doesn’t implement methods for this endpoint. You can use the requests library to access the account endpoint functions.
Get Account MetaData
The account/metadata API call returns your account’s metadata, e.g., account tier, message limit, message used, etc.
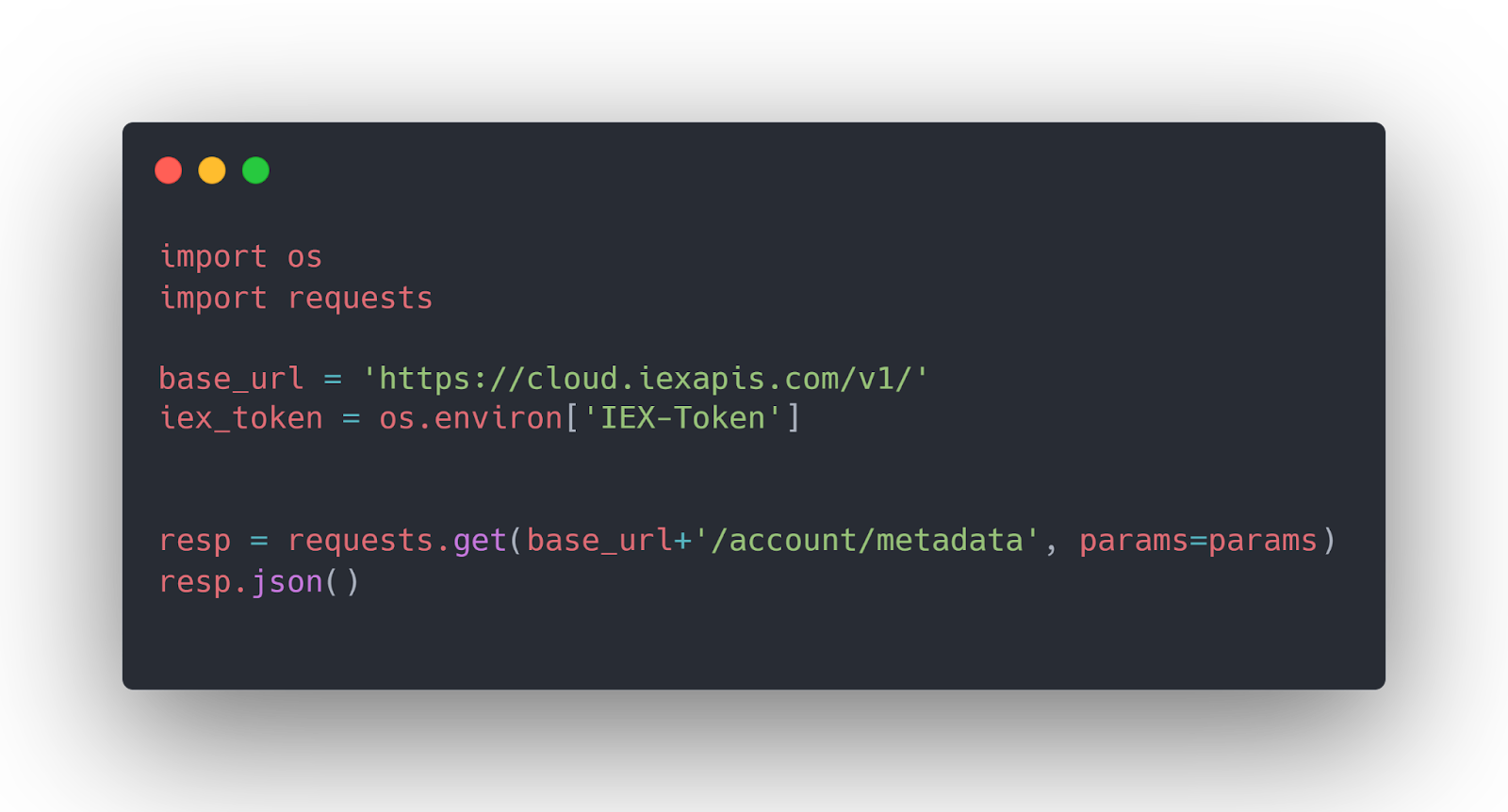
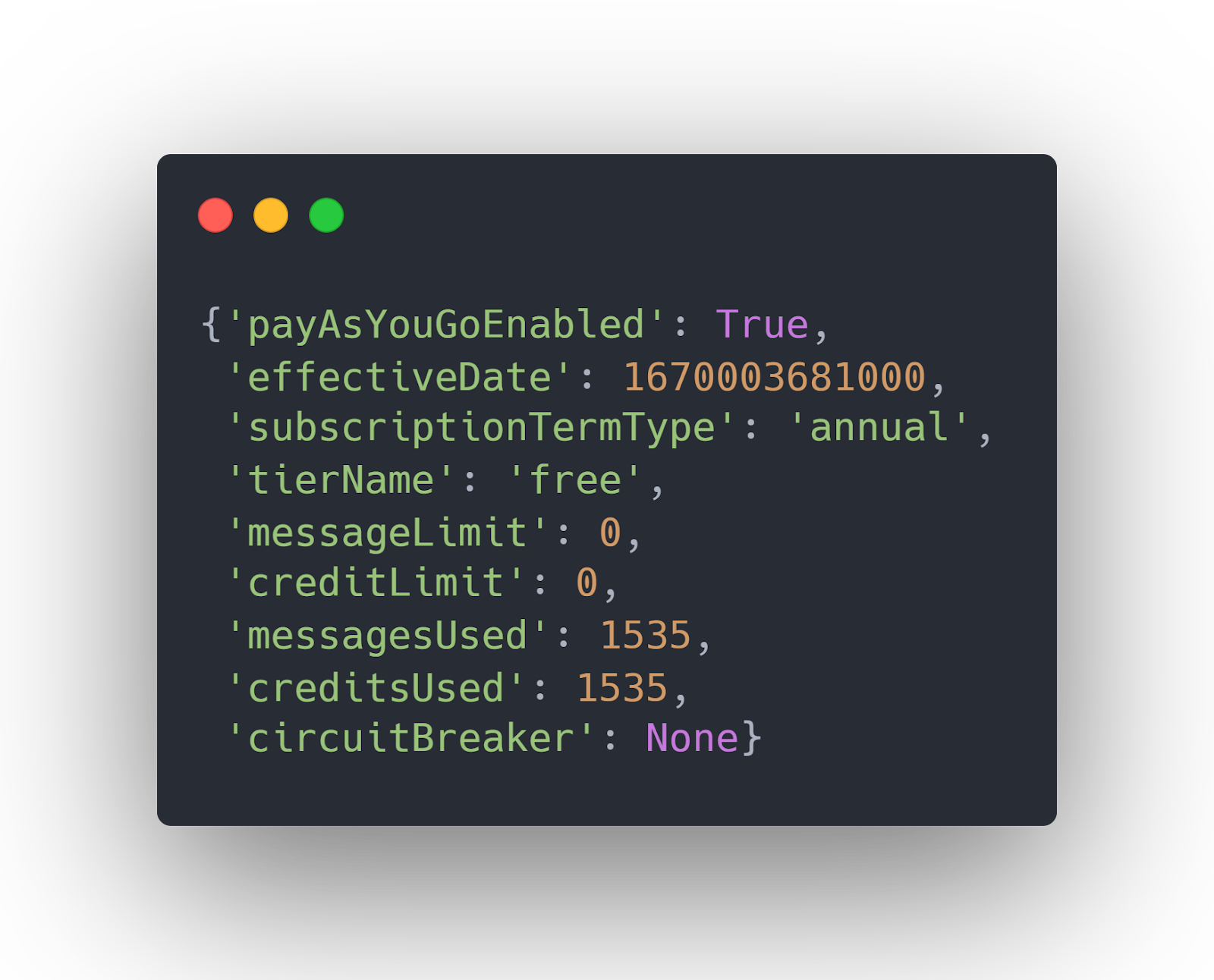
Get Account Usage
The account/usage/credits API call returns your daily and monthly account usage credit along with the information about key API calls that you made.
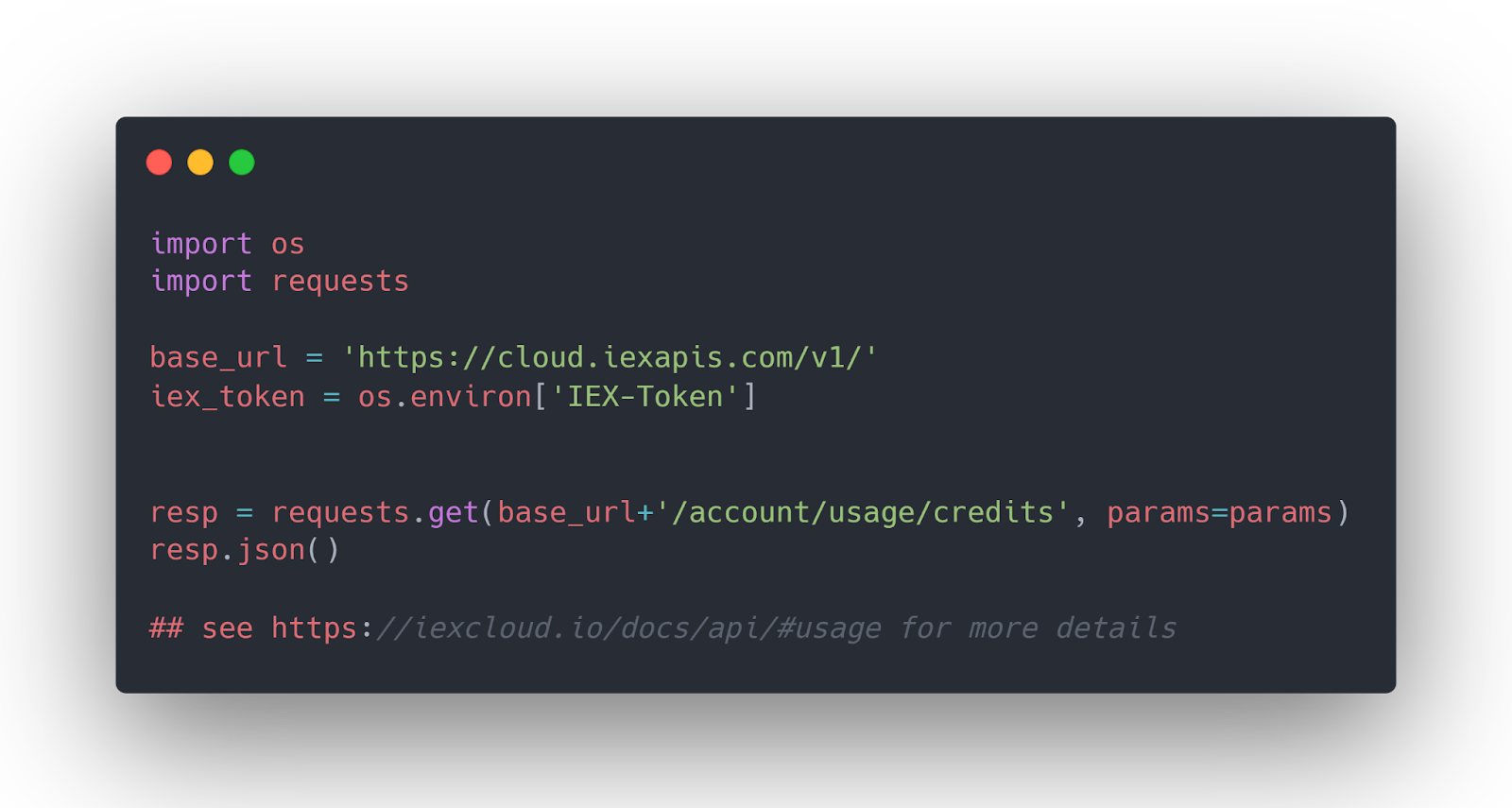
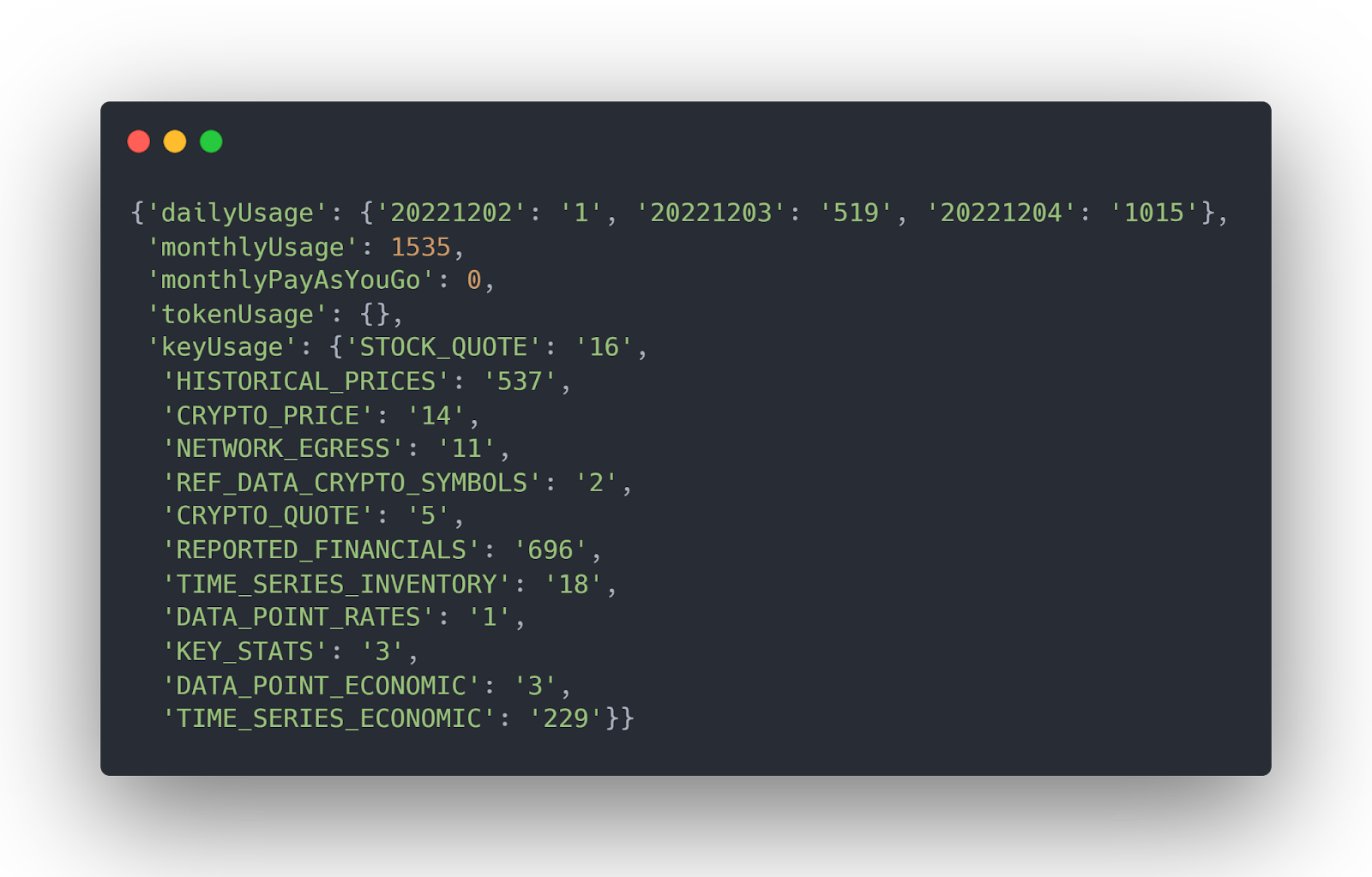
News Endpoint
The news endpoint functions return news about companies from over 3000 global news sources, including major news networks, publications, and regional media.
Get Intraday Trading News
The news() method returns a company’s intraday news. You must a company’s stock symbol to the news() method.
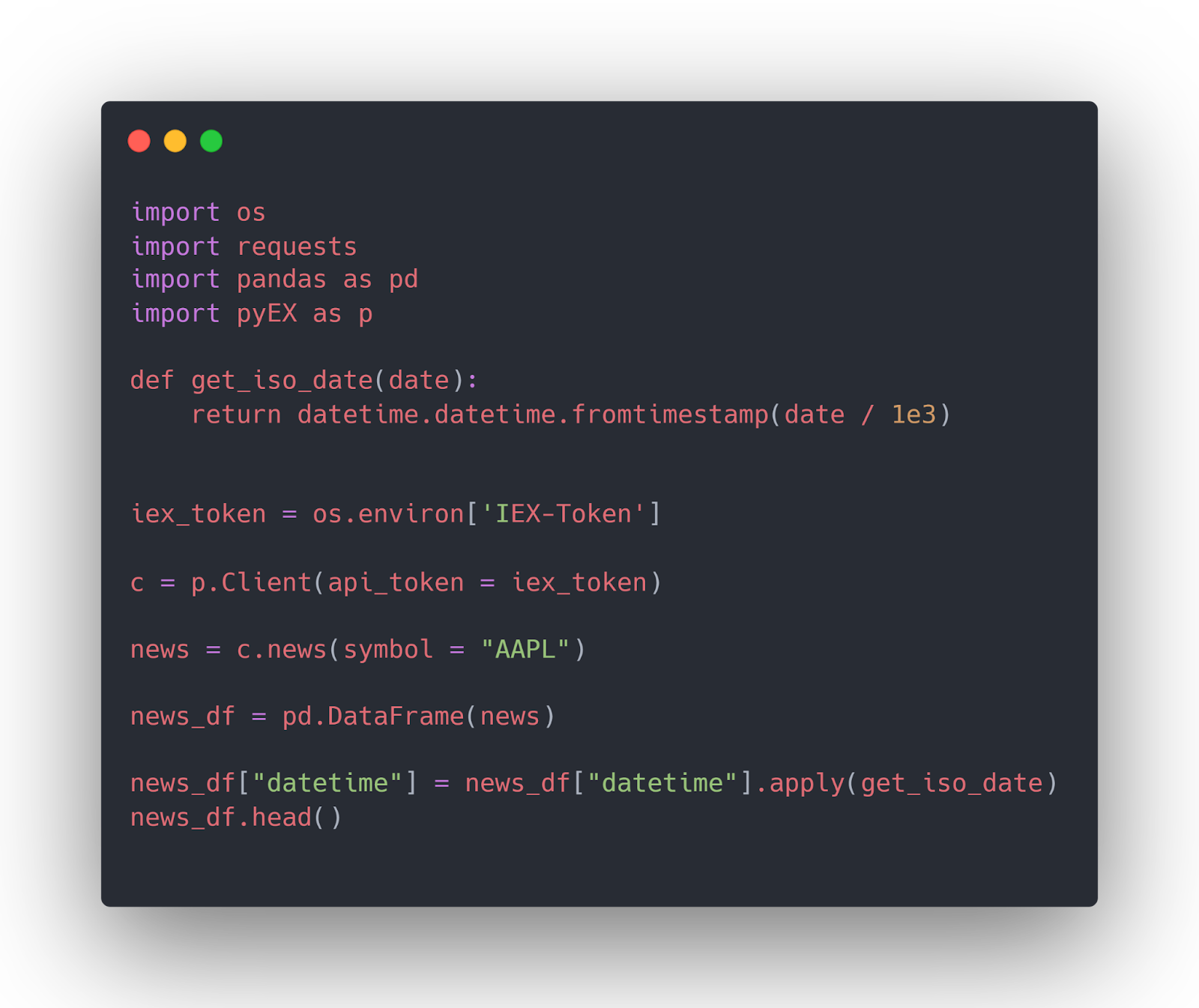
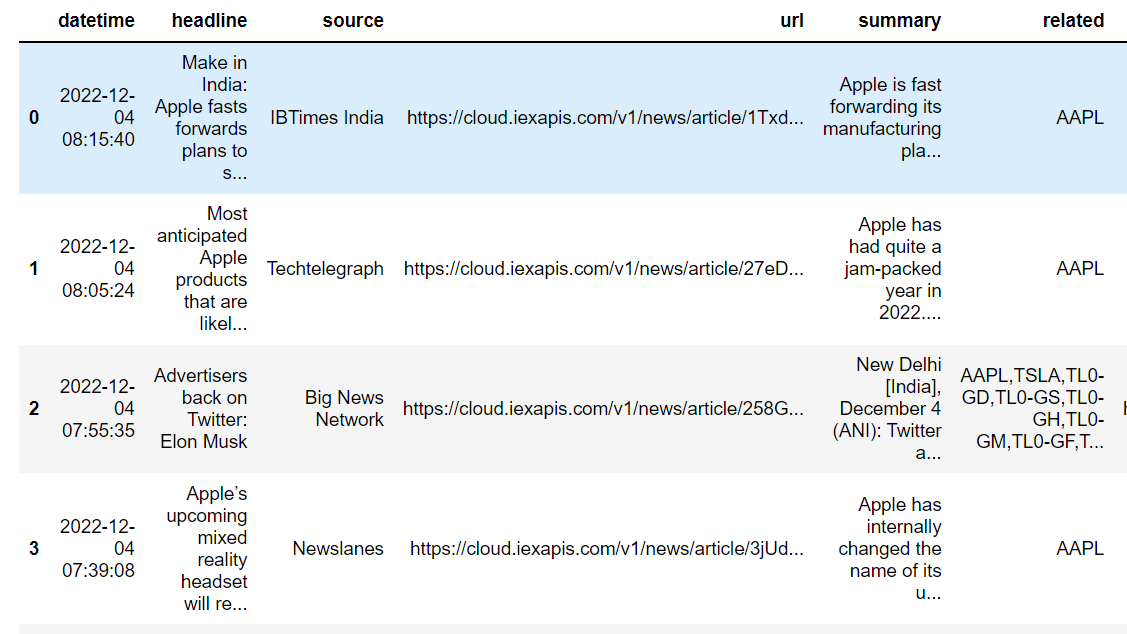
Reference Data Endpoint
The reference data endpoint functions return reference values for parameters and attributes of other endpoint functions. For instance, you can retrieve IEX Cloud’s stock symbols list that you can pass to stock endpoint functions.
Search Symbol
The searchDF() method returns up to 10 top matches for your search query. The search defaults to equity symbols listed under the stocks category. The method is not accessible with a free tier account.
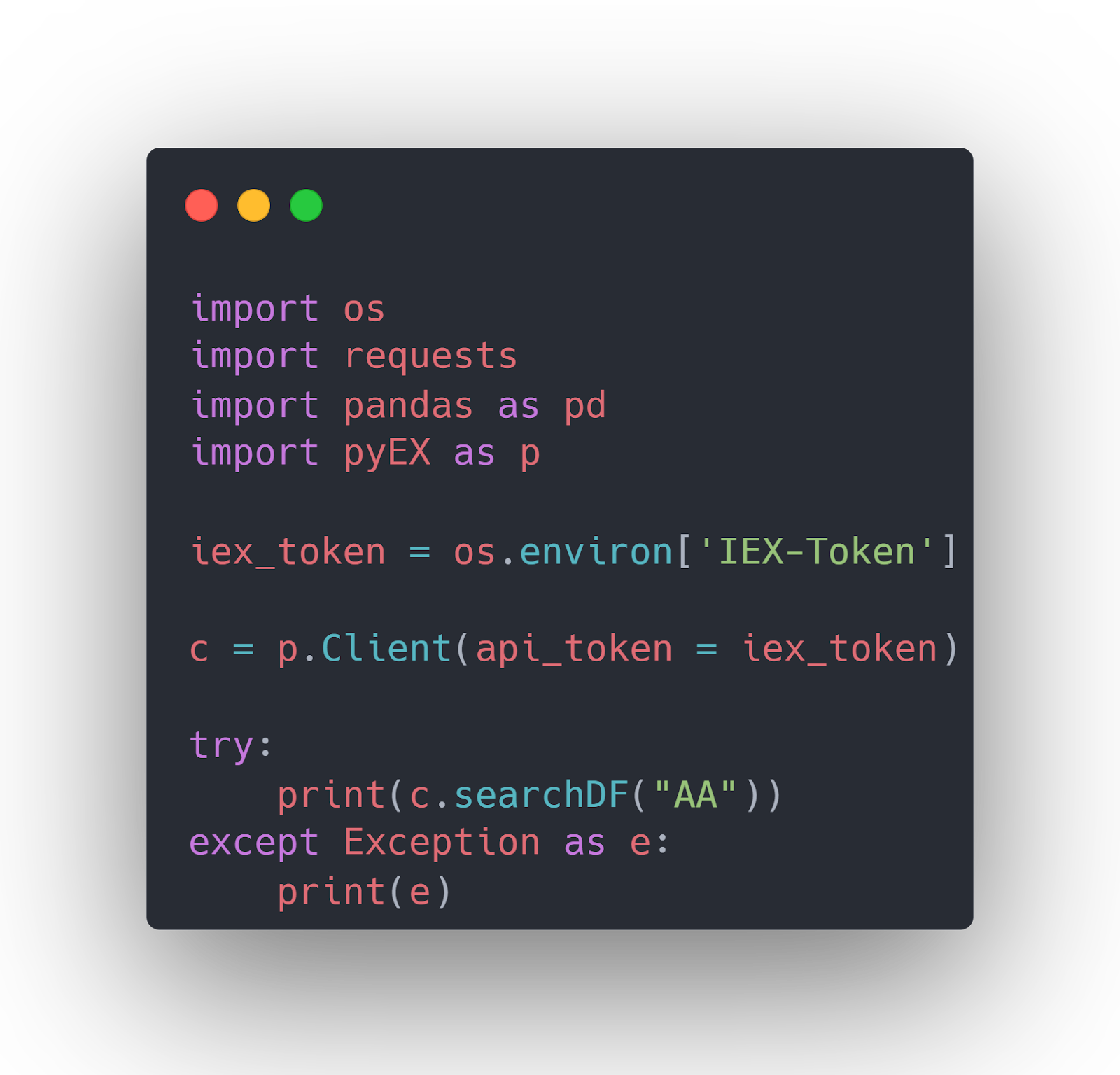
Get Stock Symbols List
The symbolsDF() method returns a list of all stock symbols that IEX cloud supports for intraday price updates.
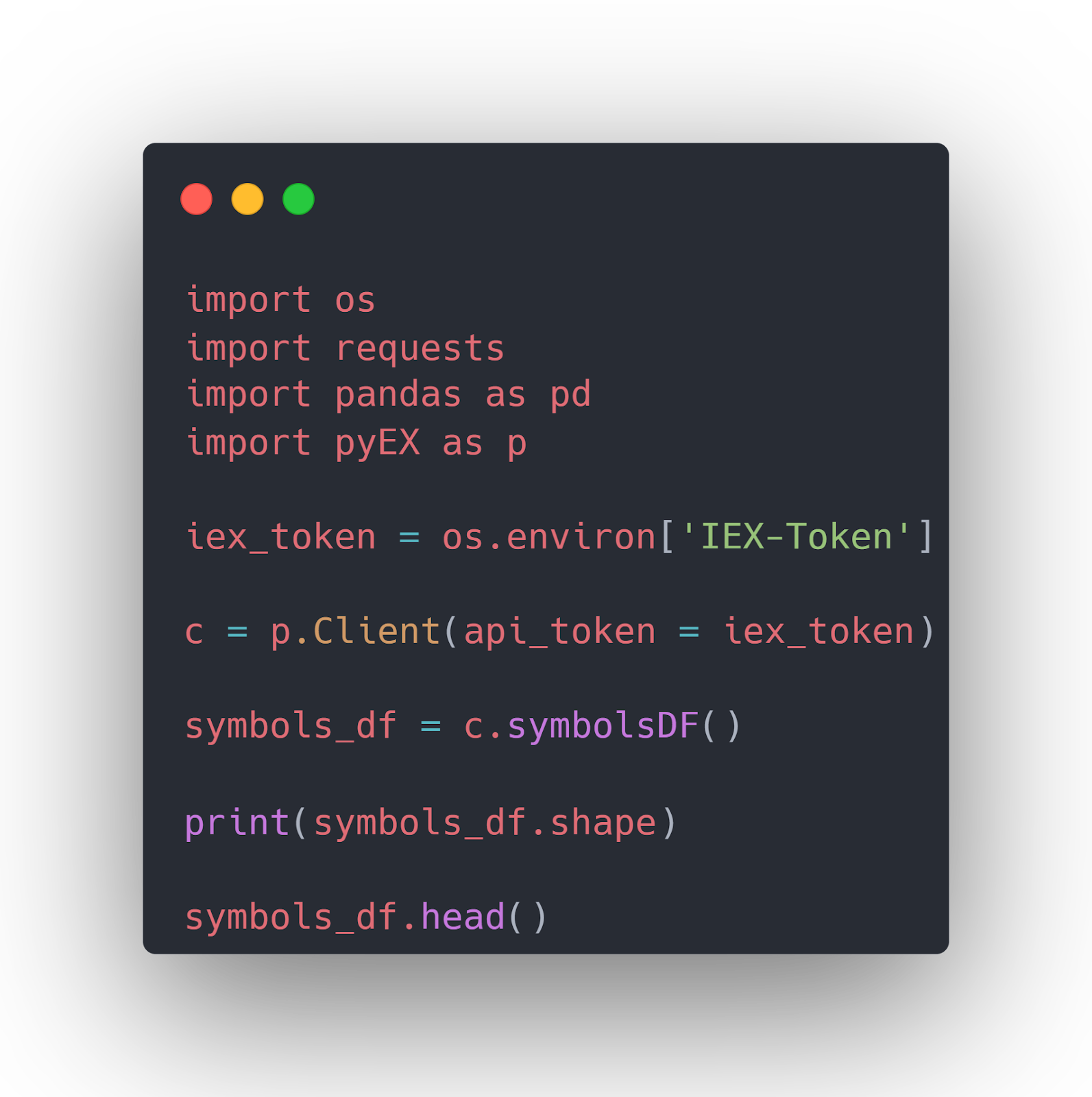
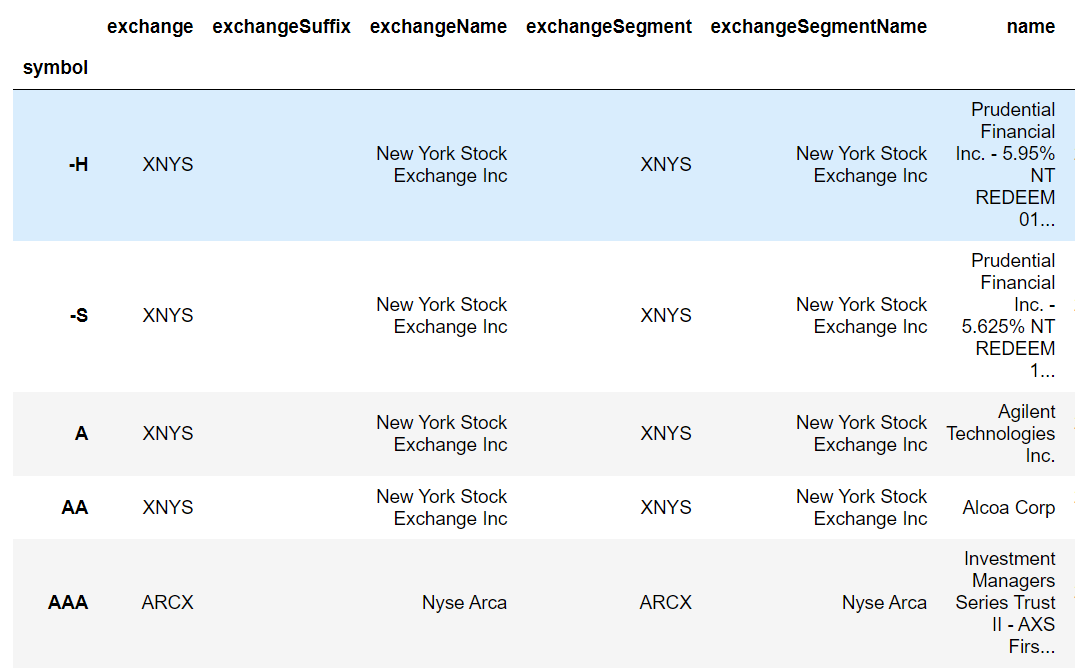
Get Cryptocurrency Symbols List
The cryptoSymbolsDF() method retrieves all cryptocurrencies that IEX Cloud supports.
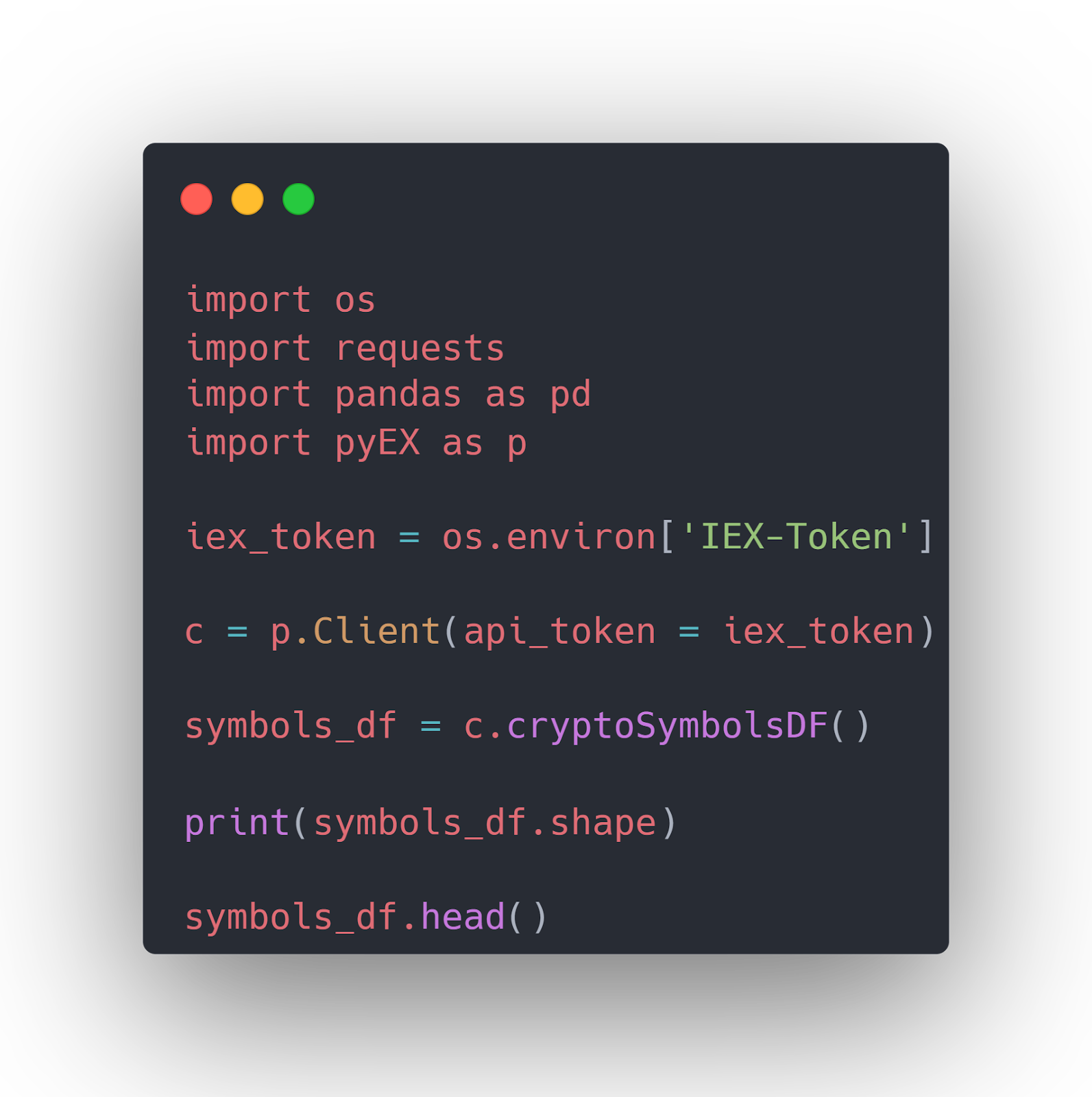
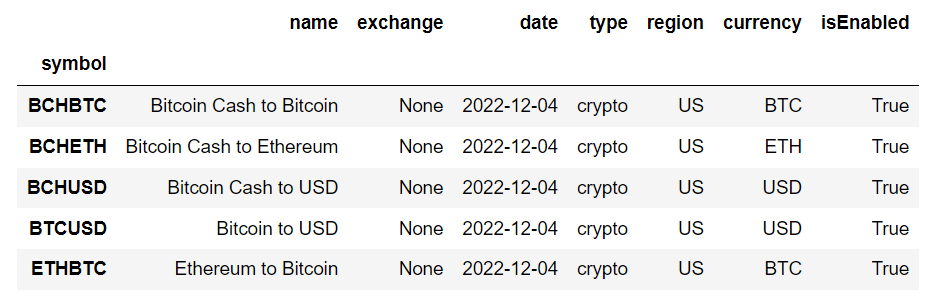
Get US Exchanges List
The exchangesDF() method returns a list of all US exchanges.
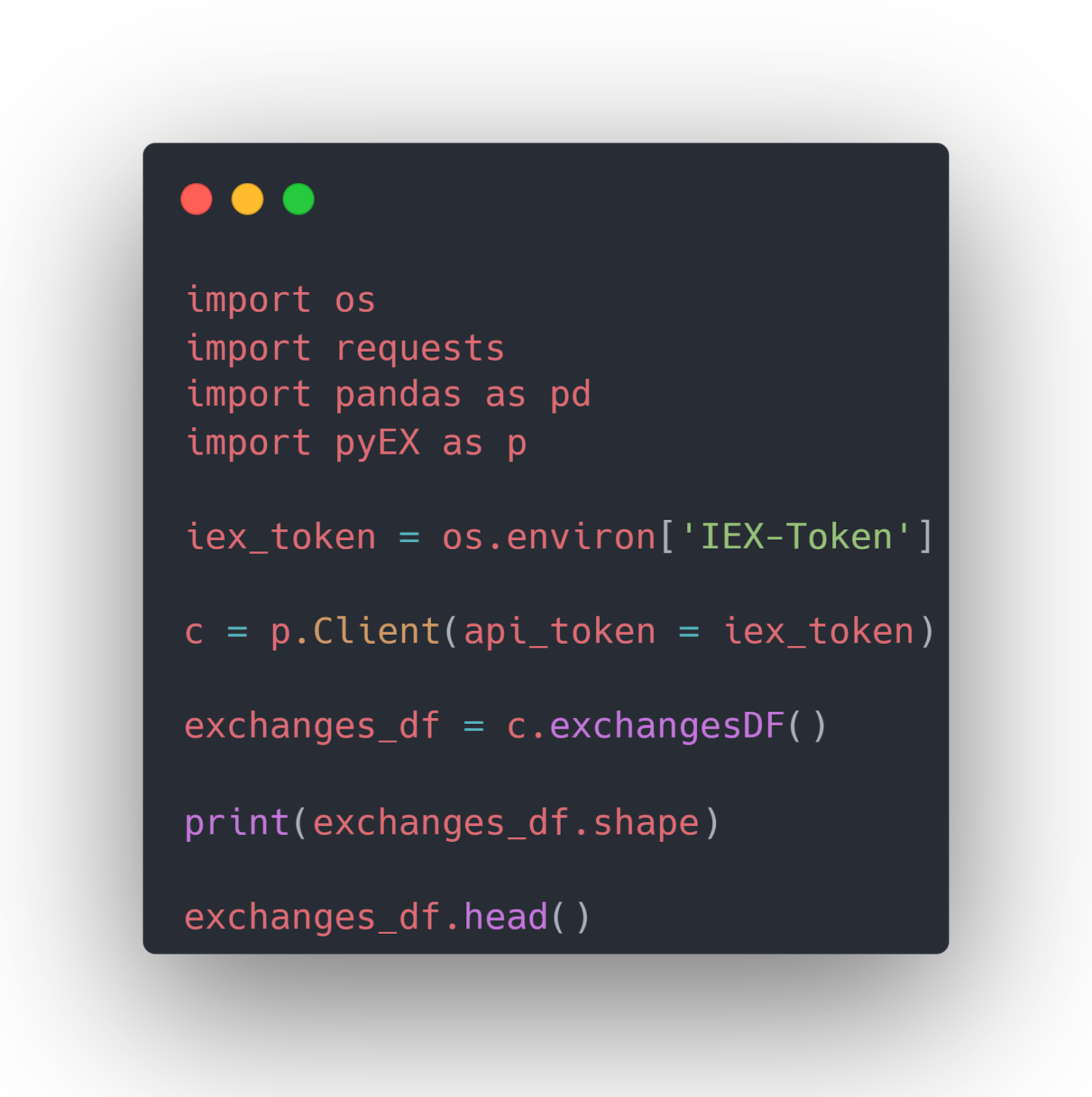
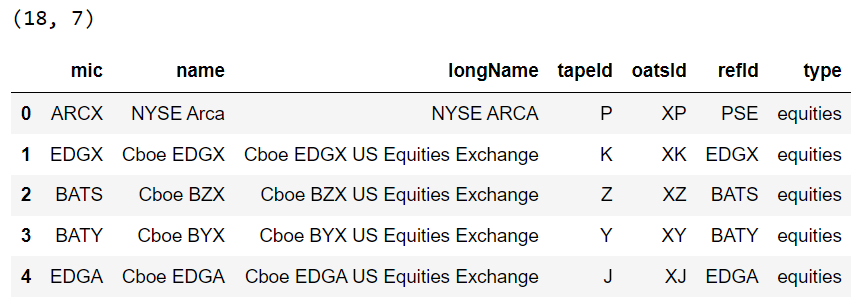
Get International Exchanges List
The internationalExchangesDF() method returns a list of all international exchanges that IEX Cloud supports.
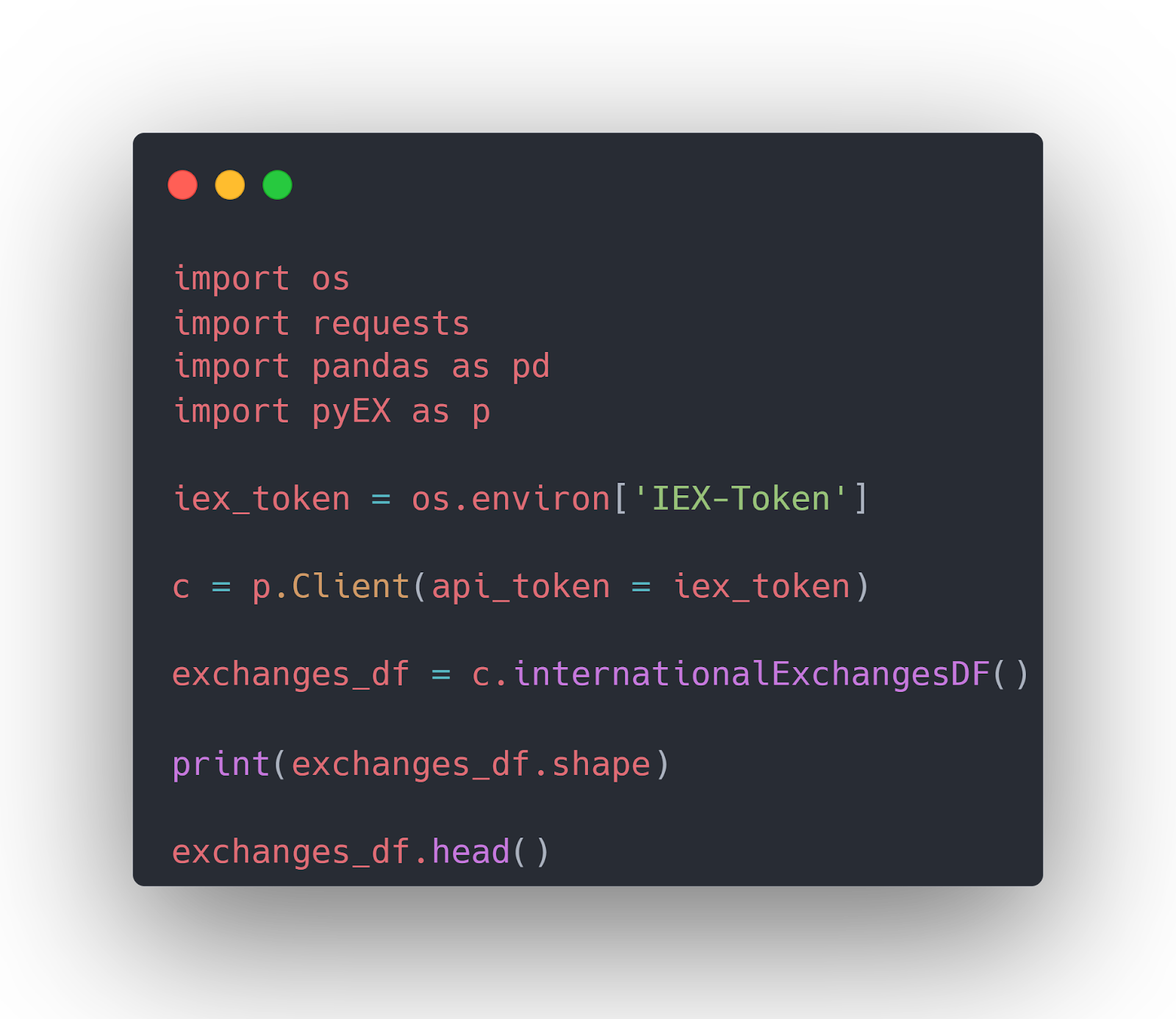
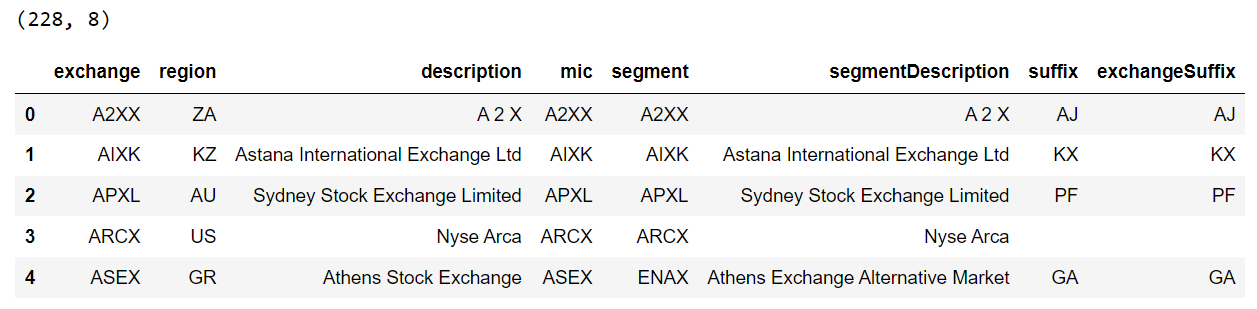
Investors Exchange Data
Investors exchange data endpoint functions return data from Investors Exchange (IEX), the parent company of IEX Cloud.
IEX Top Asking and Bid Prices
The iexTopsDF() method retrieves top asking and bid prices in near real-time for all IEX securities.
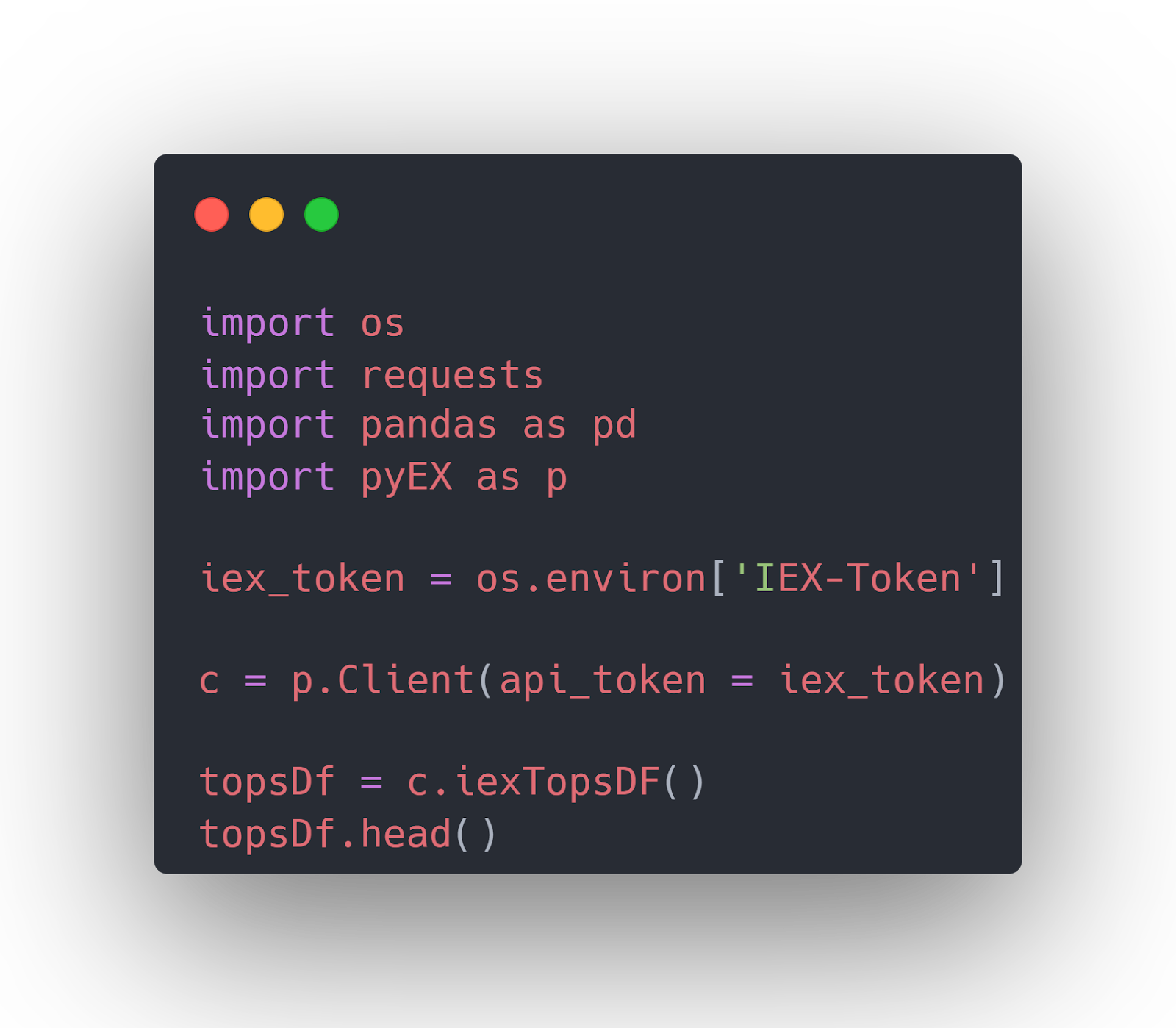
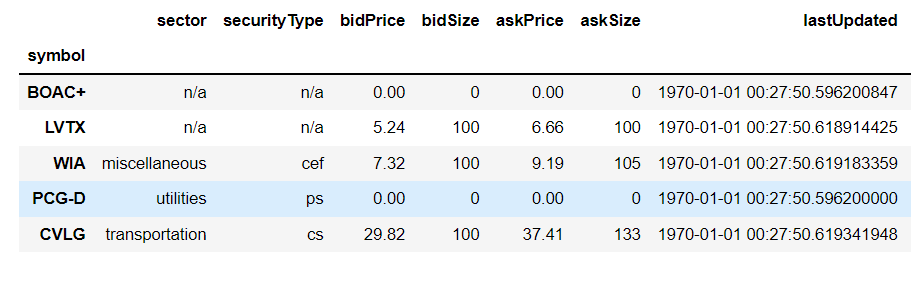
Get Last Trade Data
The iexLastDF() method returns near real-time last sale price, size, and time of trade for all symbols on IEX.
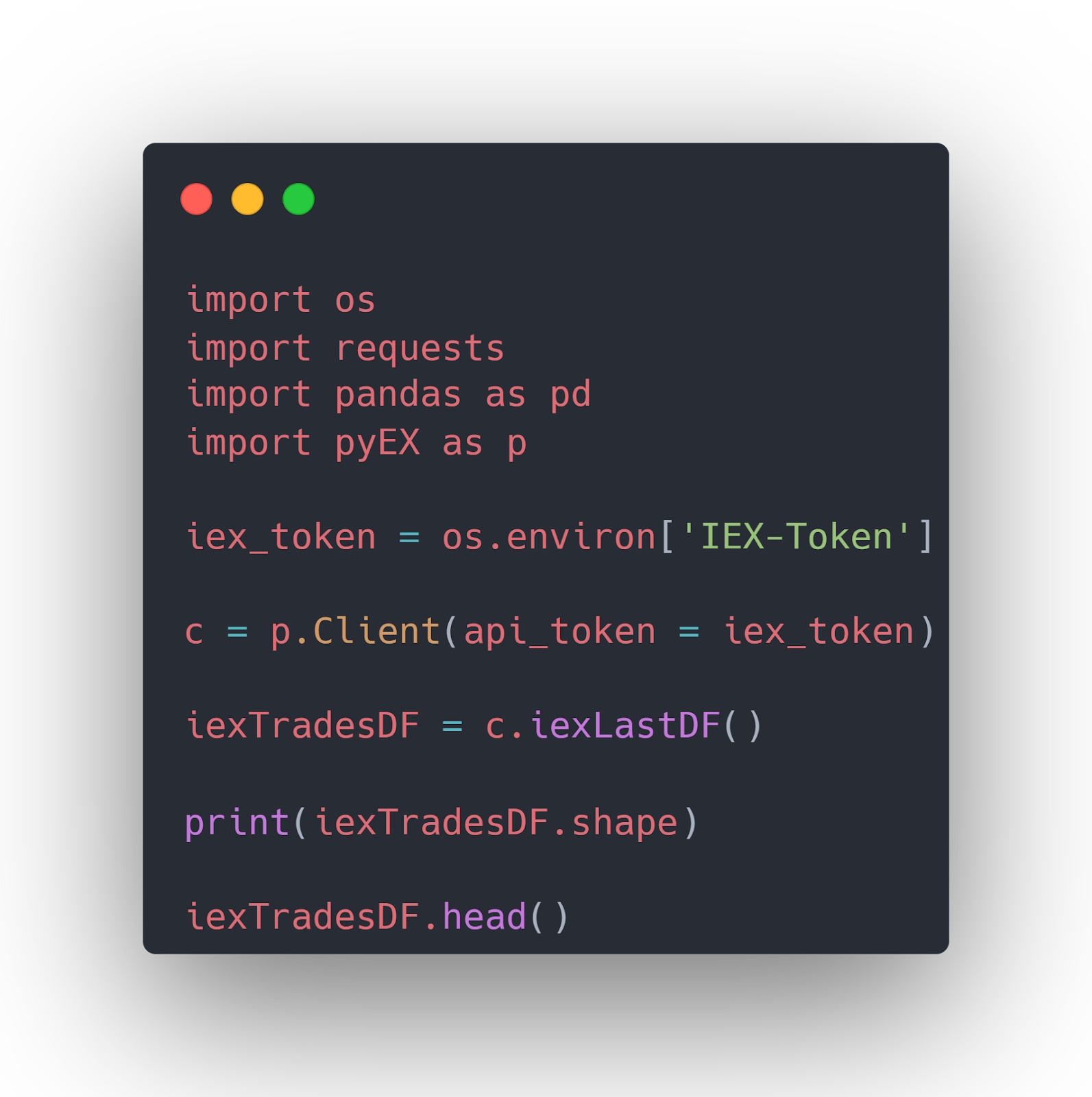
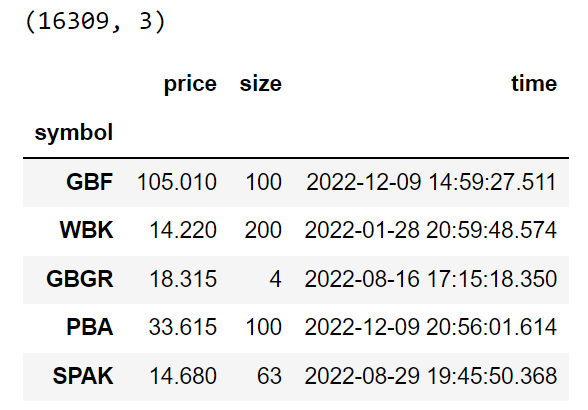
Get IEX Book Data
The iexBook() method returns recent asking and bid prices for a symbol on IEX.
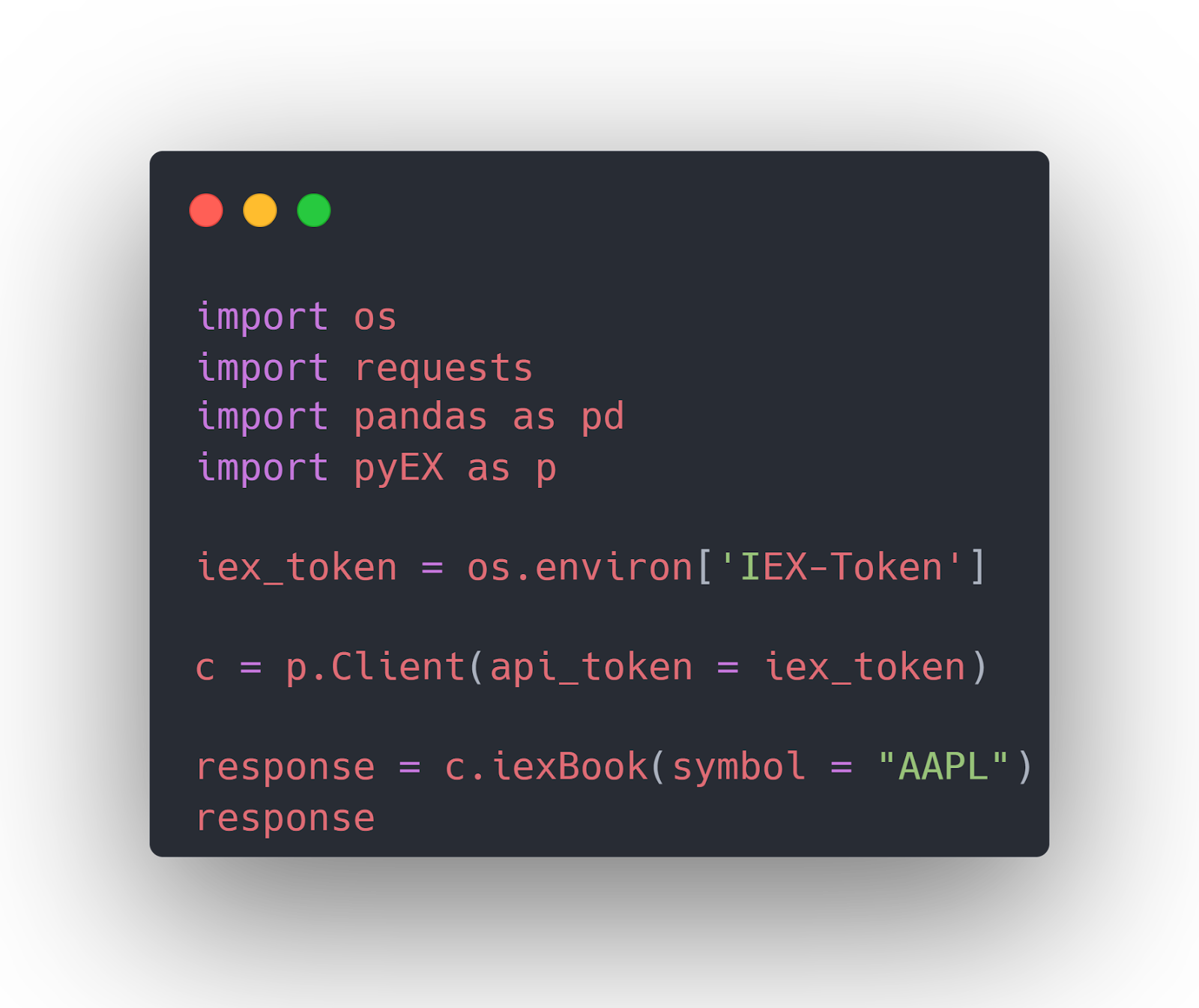
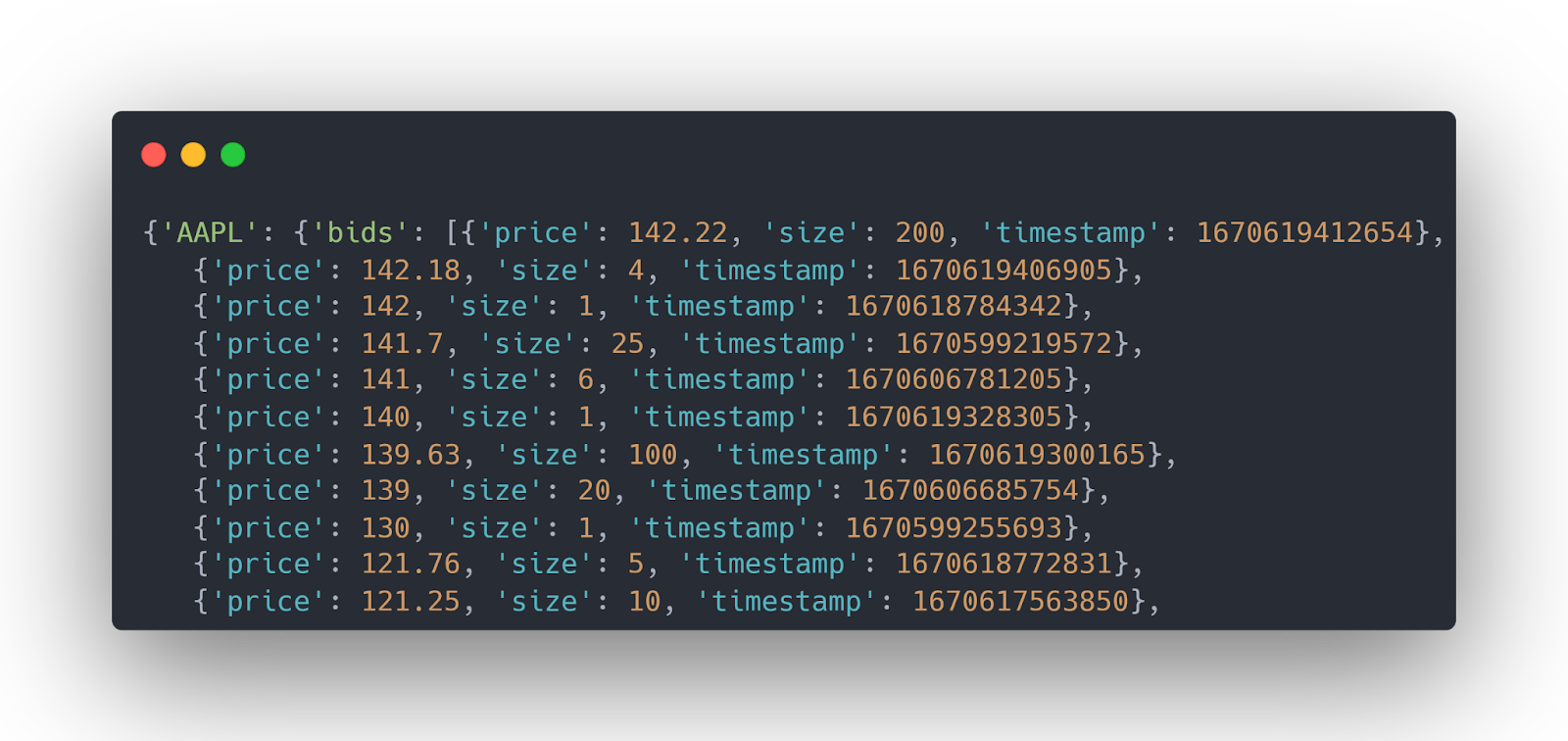
Advanced Topics
Streaming Data
The PyEX library methods that end with SSE, e.g., cryptoQuotesSSE(), return streaming data. I encountered an error (explained later in the Common Errors and Issues section) calling streaming endpoints via the PyEX library. I used the requests library instead.
The base URL to access IEX Cloud streaming functions via the requests library is:
https://cloud-sse.iexapis.com/stable/
Functions URLs for all streaming functions is available on the official documentation. Streaming endpoints are only available with paid subscriptions.
IEX Cloud supports Server-sent Events (SSE) over Websockets for streaming data. You can use the SSEClient library to iterate over the HTTP Server-sent Event streams.
The following code fetching streaming crypto quotes for Bitcoin in US dollars.
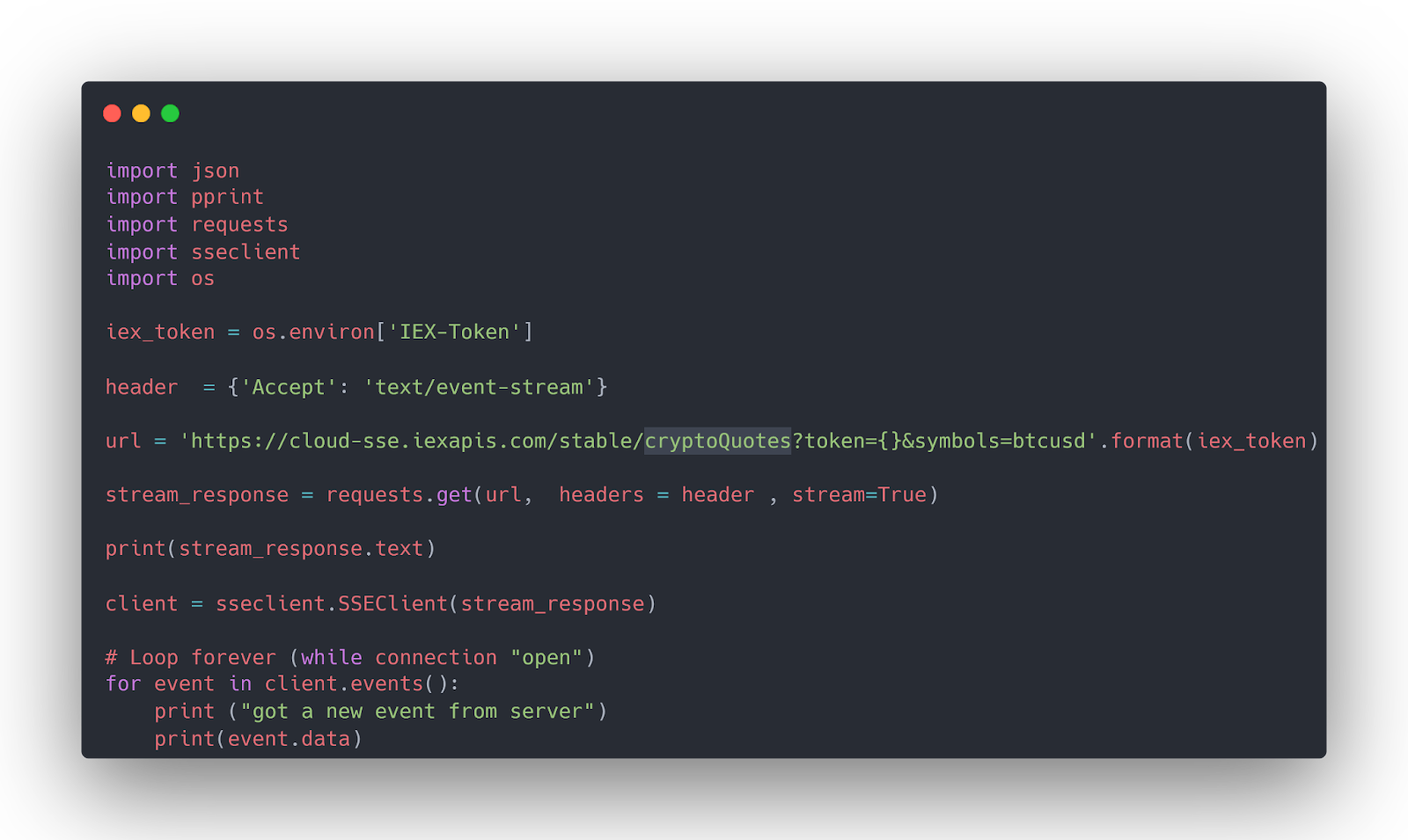
Automatic Tasks
Once you fetch data from IEX Cloud, you can customize it to fit your application needs. For instance, you can use the following script to display the last trade values for a symbol on the Investors Exchange (IEX) after every 10 seconds.
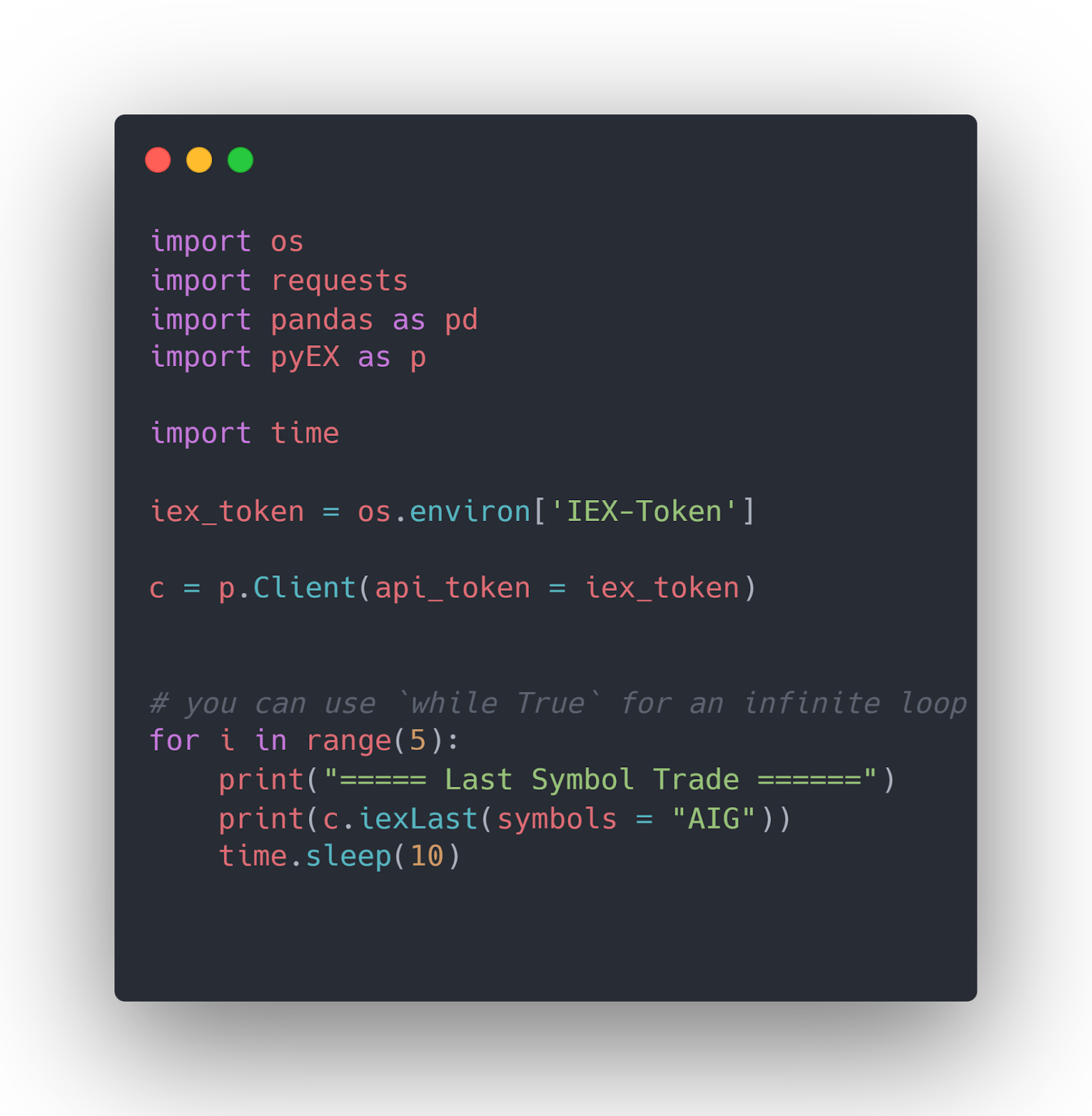
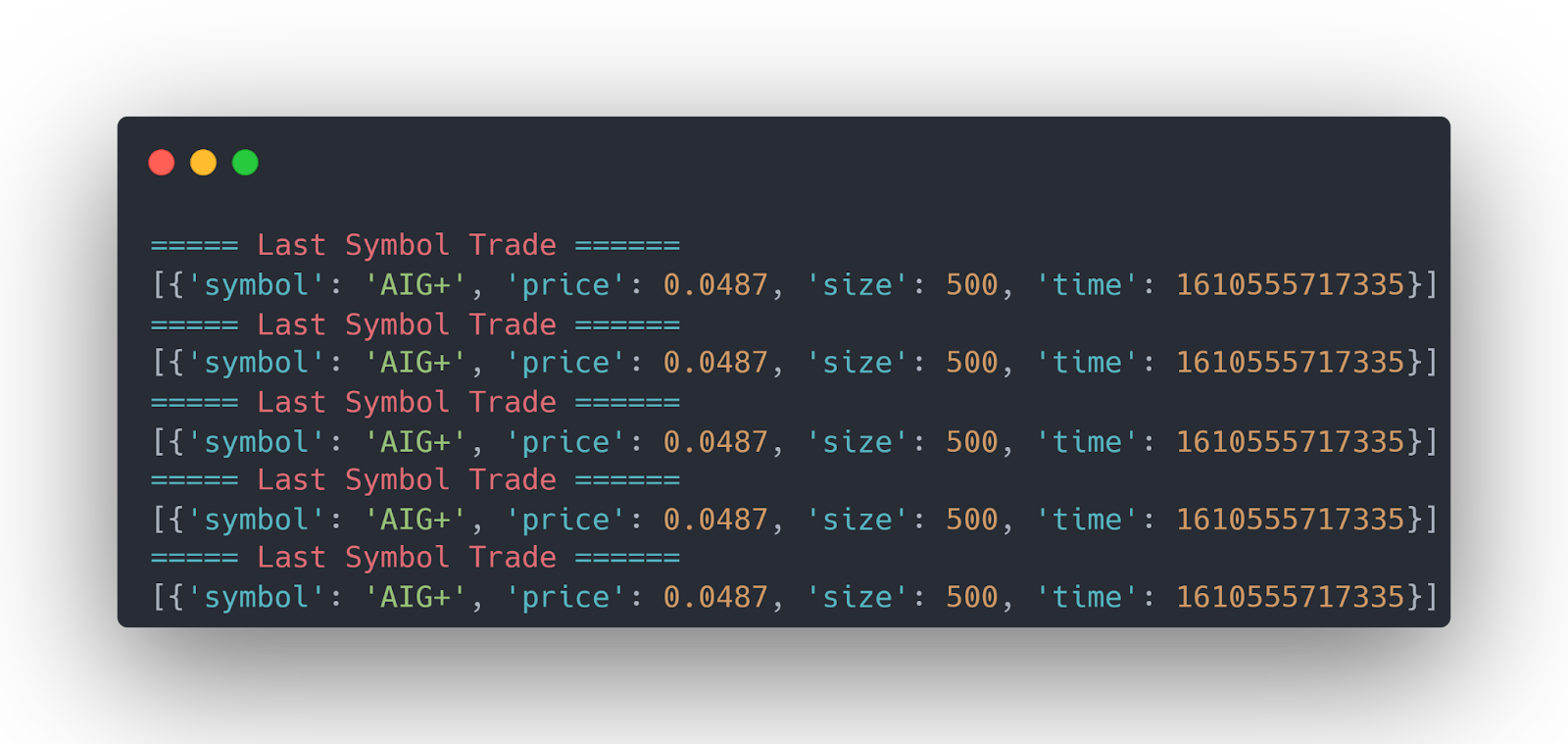
Common Errors and Issues
There are some issues and errors that I came across while accessing IEX Cloud API.
Get Daily Values for Currency Pairs
The historicalFXDF() method from the Client class of the PyEX library returns daily values for currency pairs. I got the following error while accessing this method despite providing a valid API Key.
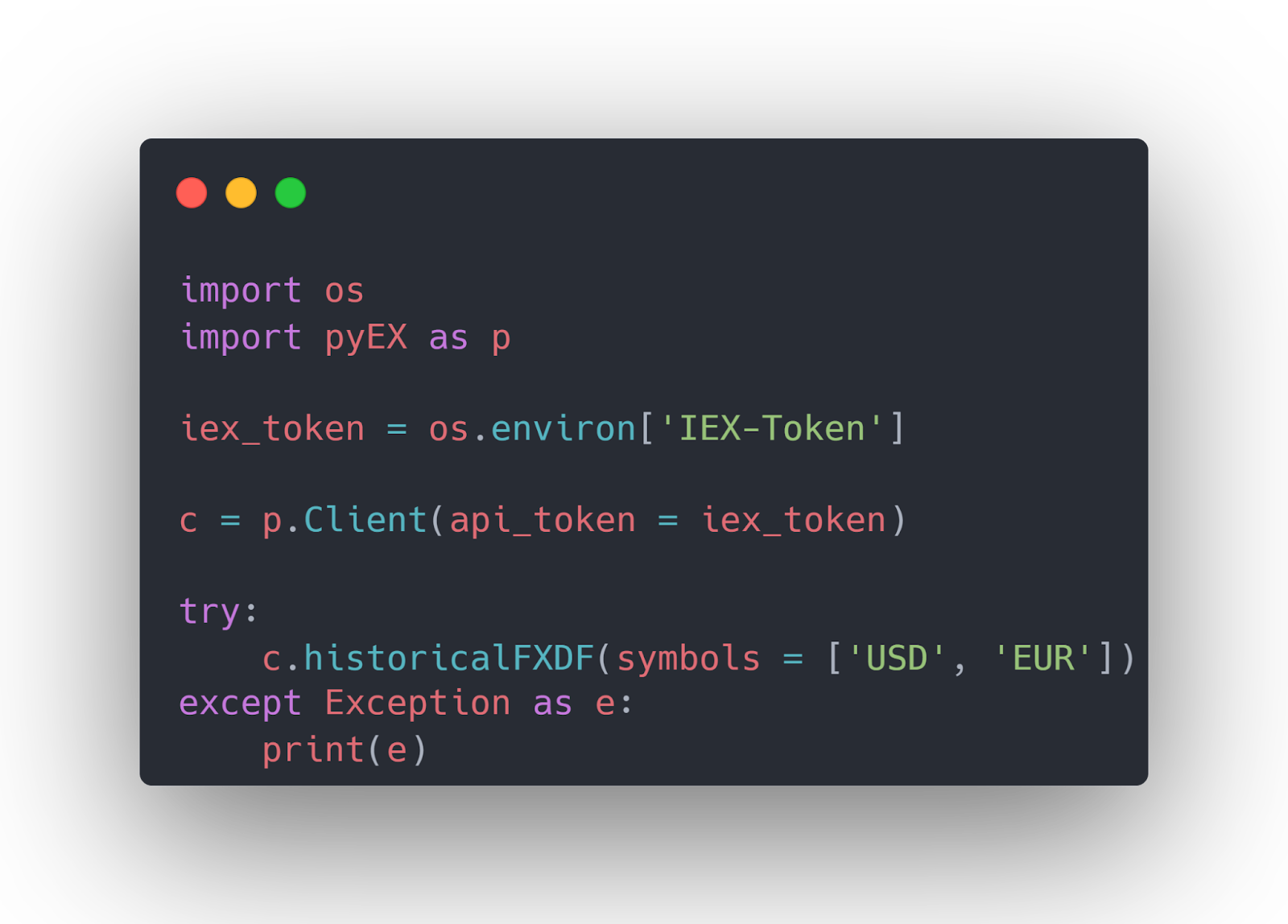
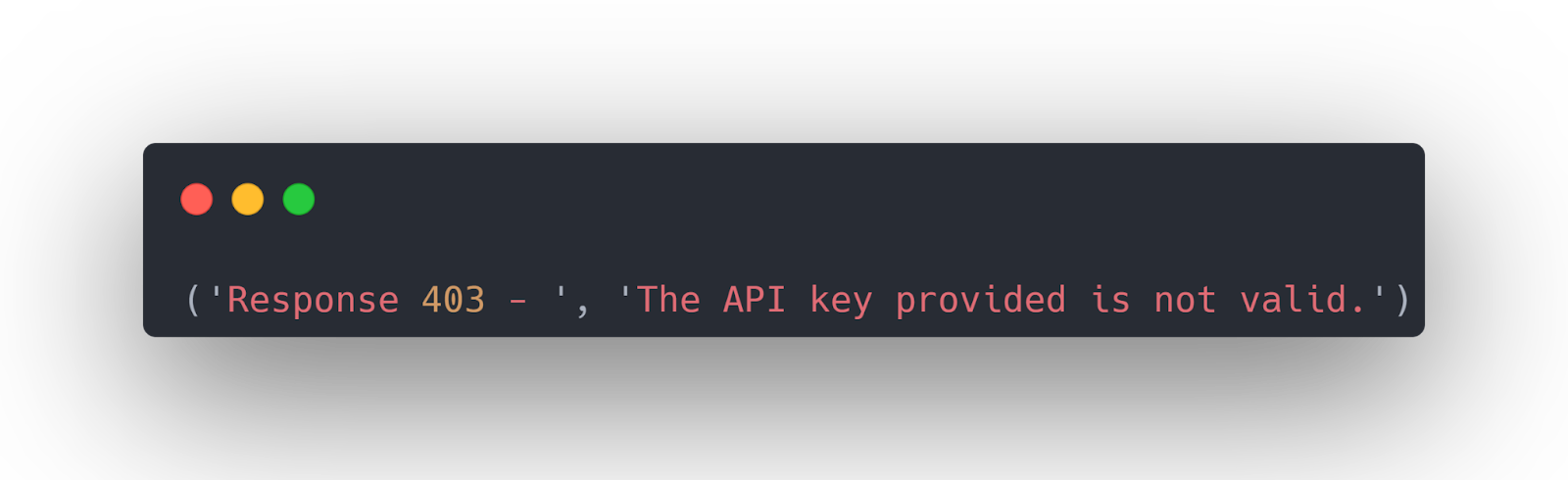
I accessed the same endpoint with the same API key using the requests library function and successfully made the call.
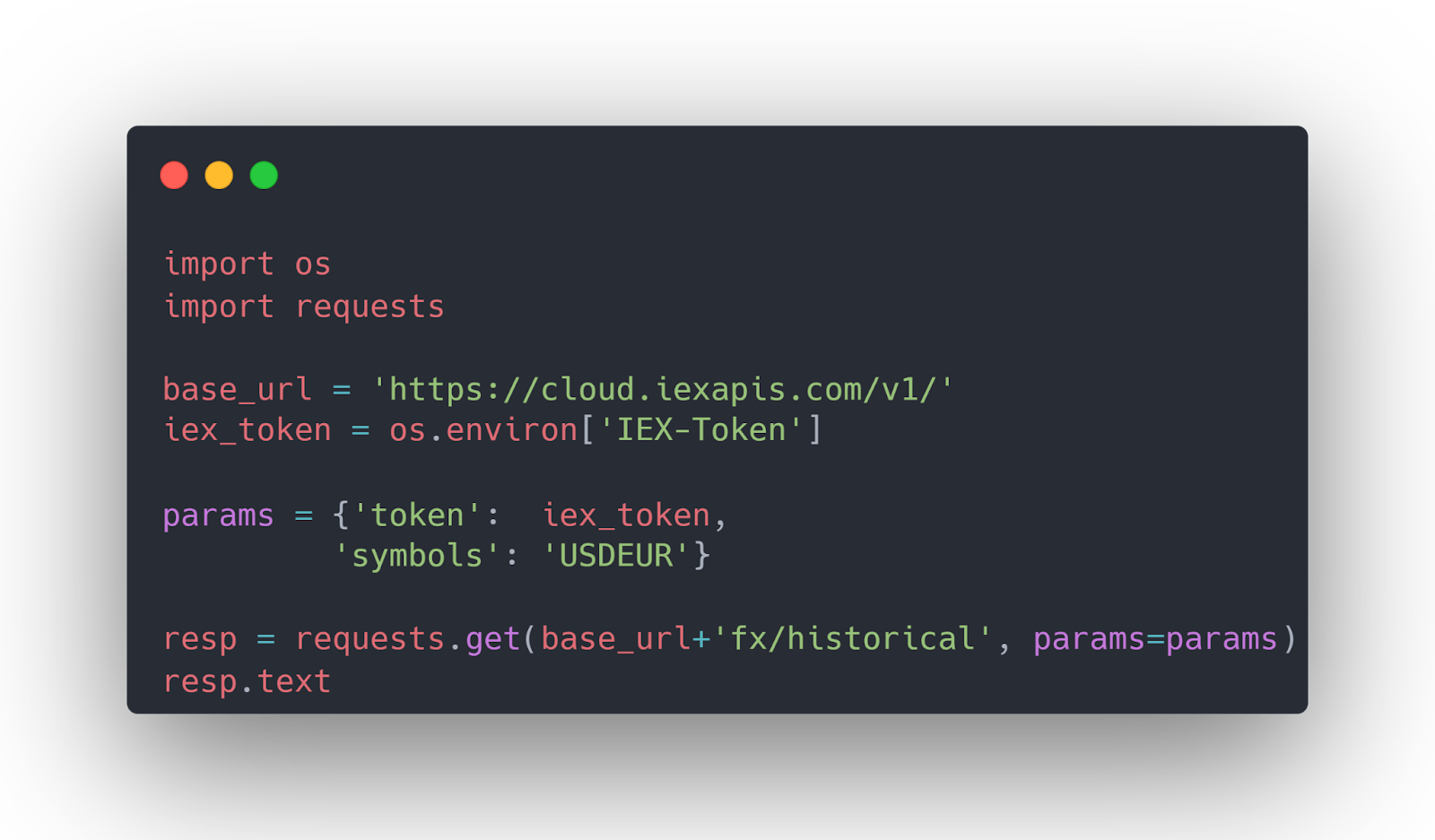
Get Stocks OHLC Values
Accessing a stock’s open, high, low, and closing values via the ohlcDF() method also returns an error.
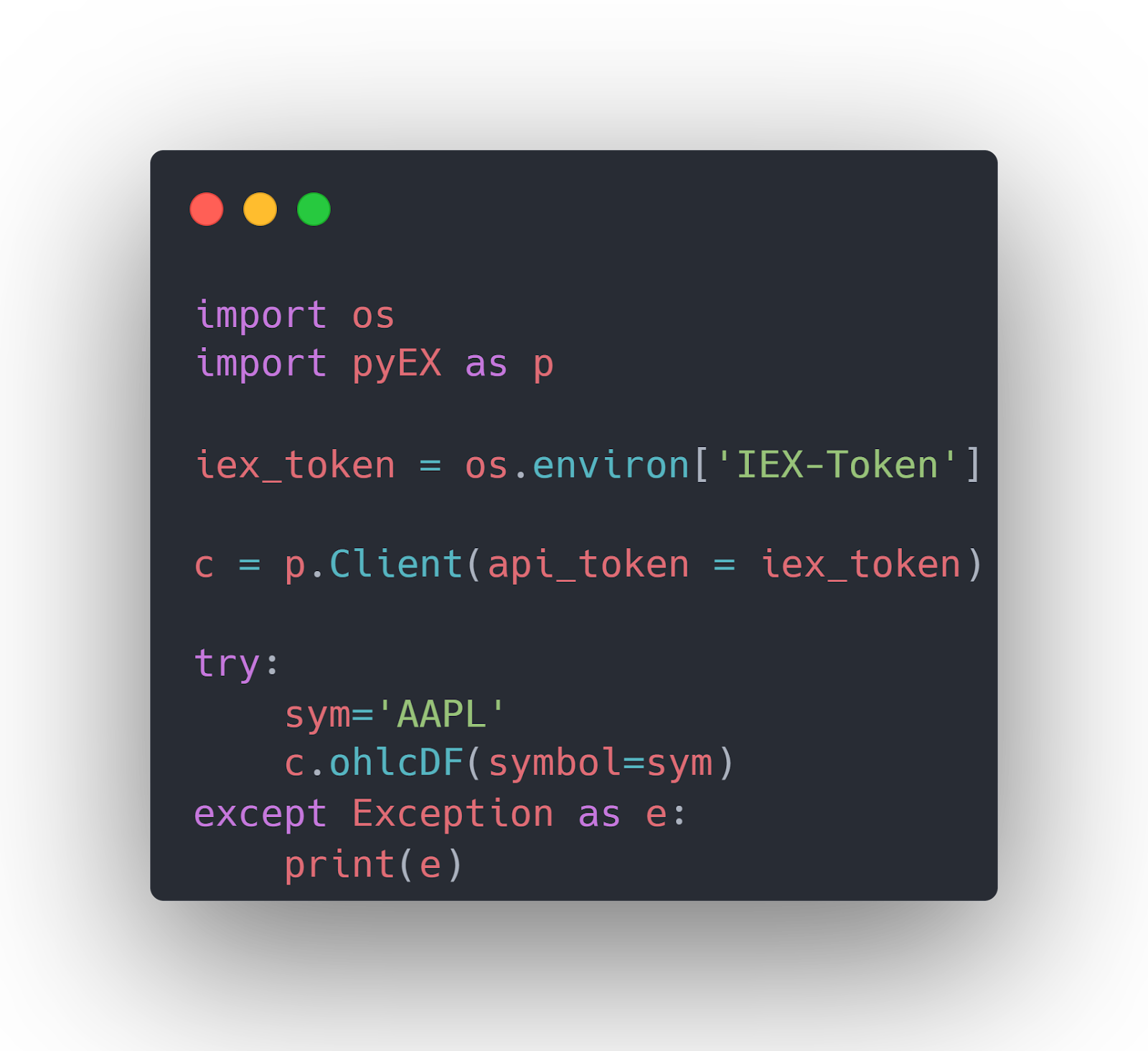
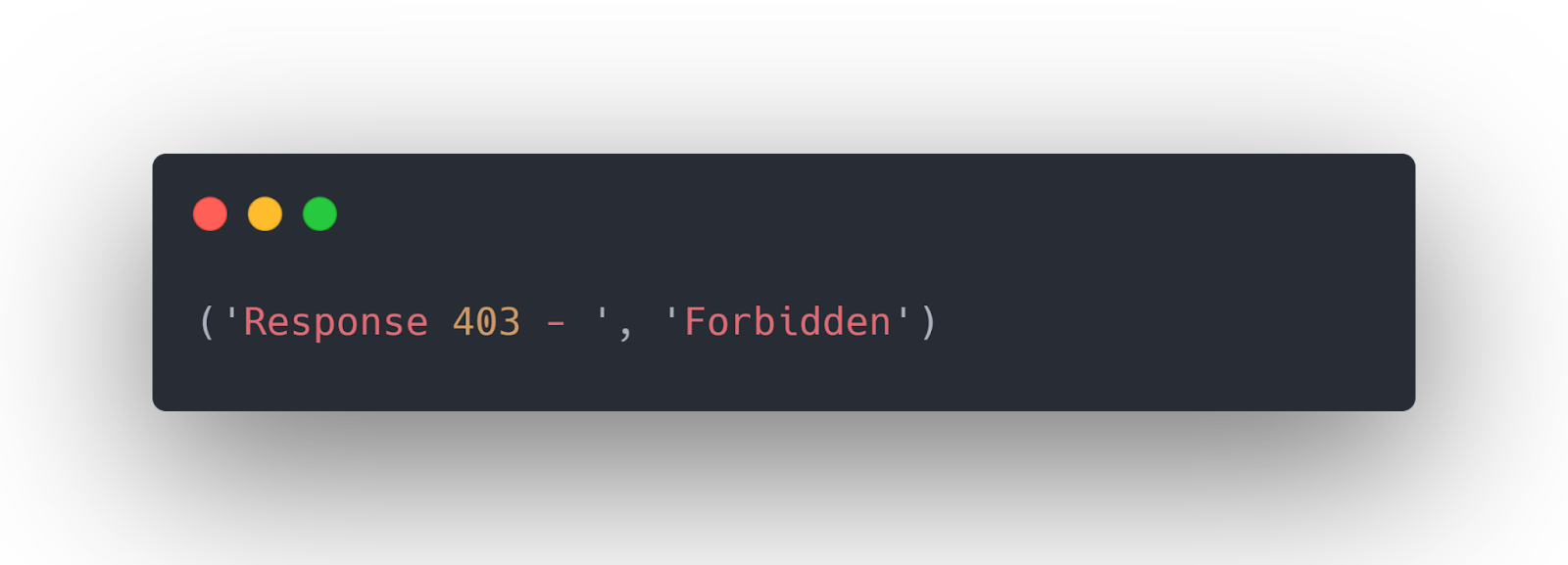
You can use the requests library to access the OHLC values for a stock.
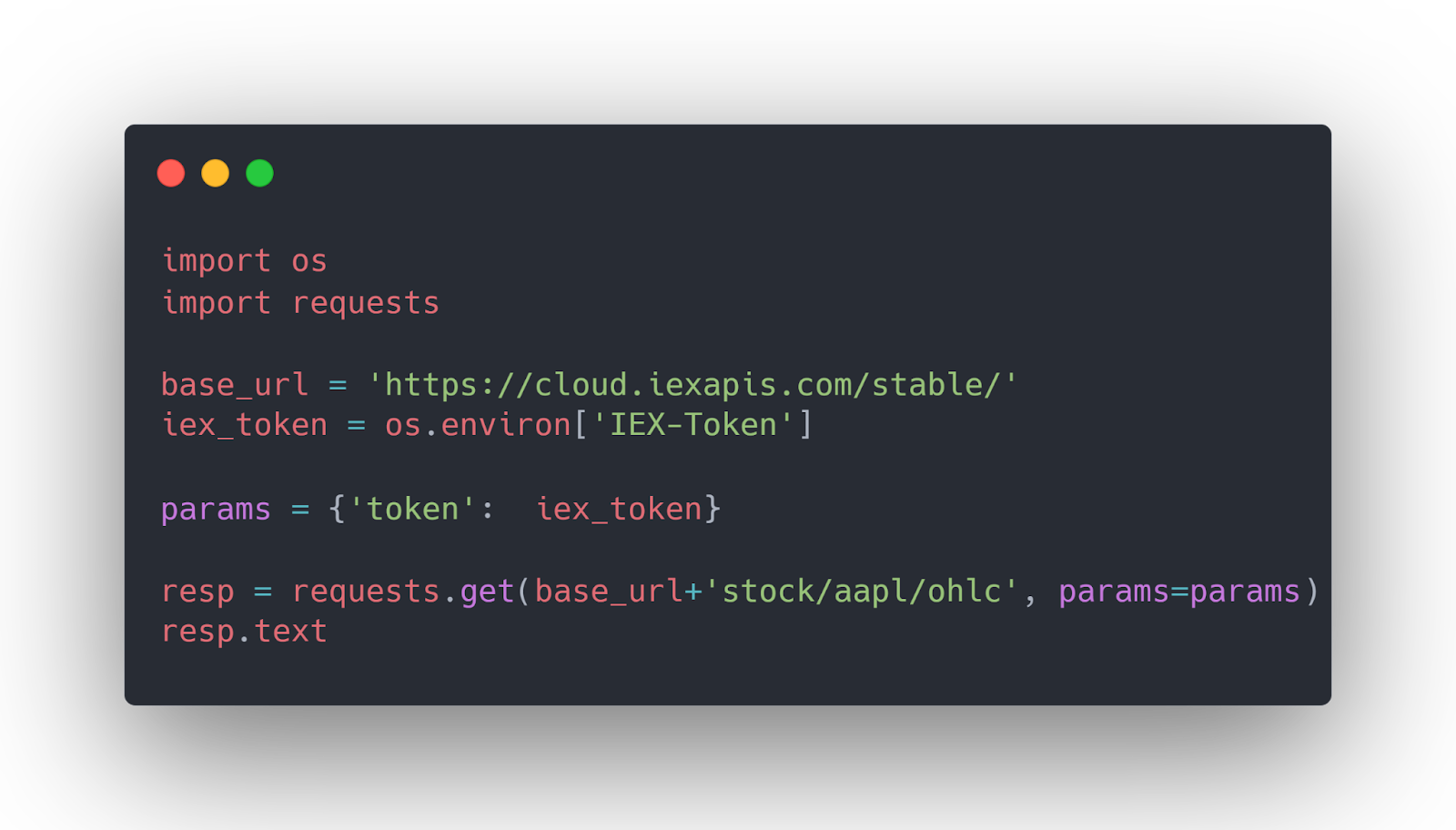
Getting Streaming Data
I encountered an exception while accessing the streaming functions using the PyEX library methods.
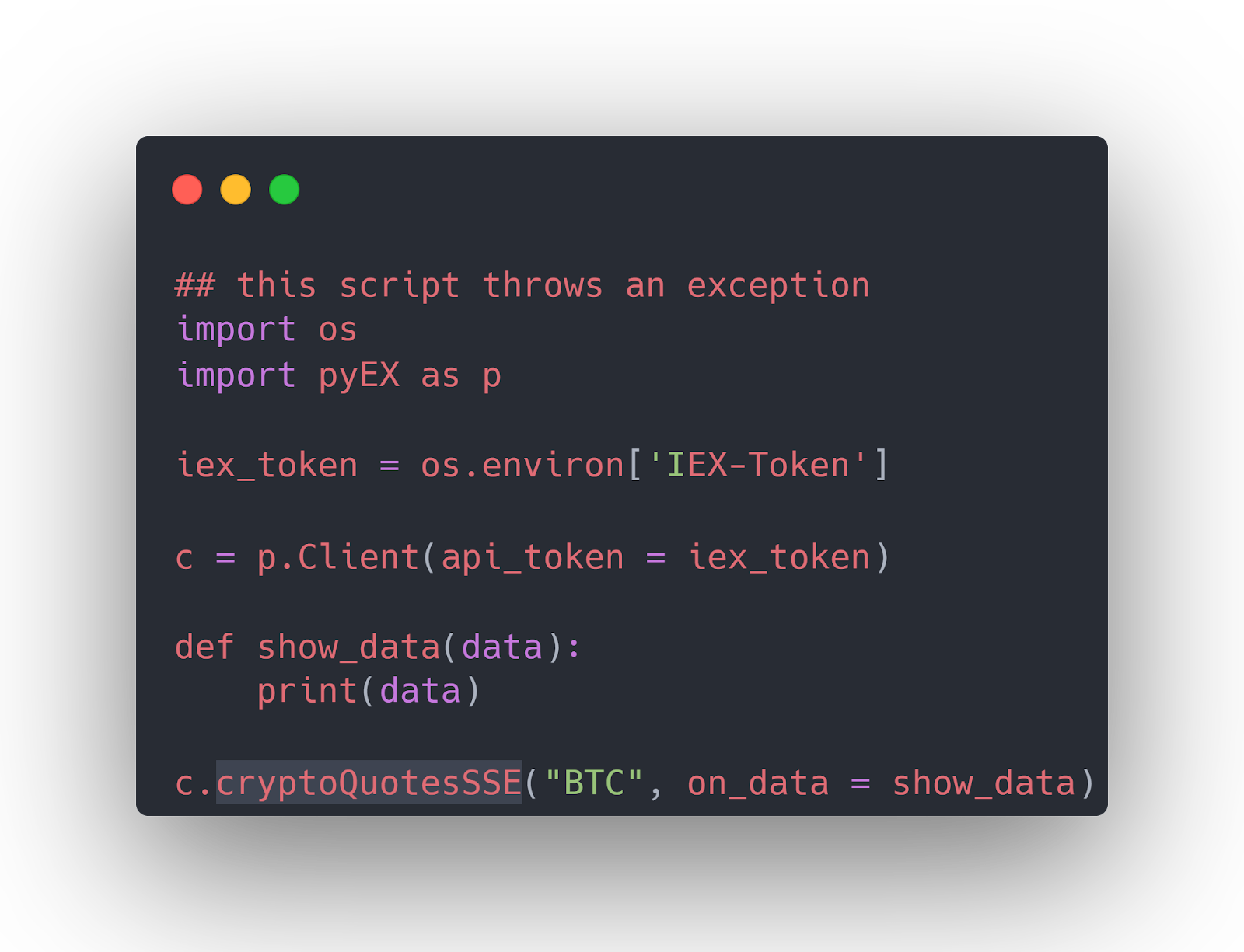
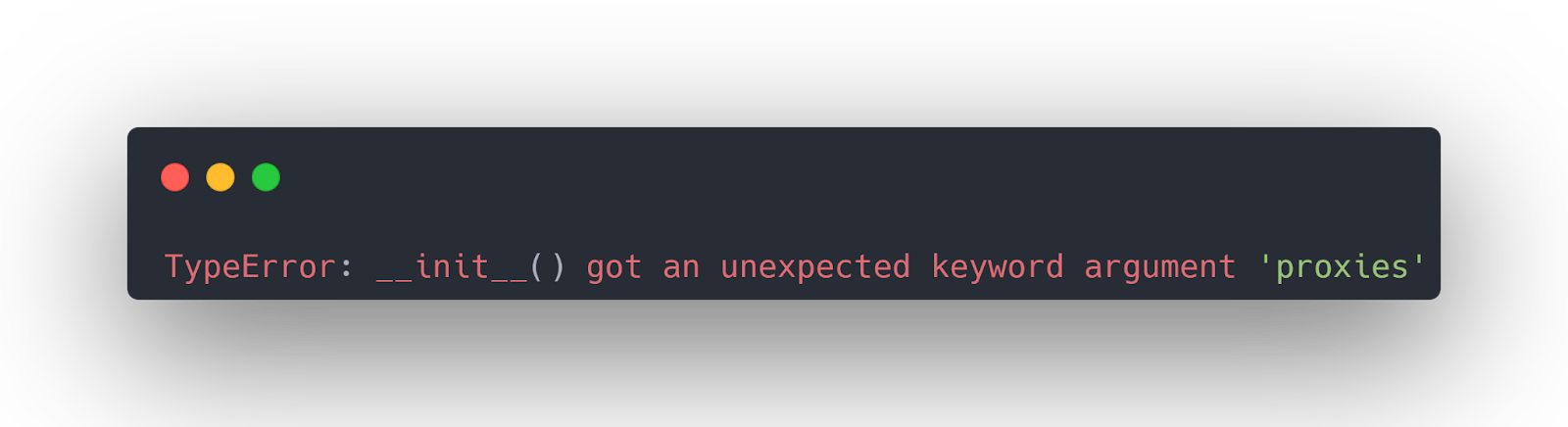
You can use the requests library to access streaming functions.
IEX Cloud Alternatives
Following are some IEX Cloud alternatives:
Frequently Asked Questions
Where Does IEX Cloud Get Its Data?
IEX Cloud real-time prices are based on trades occurring on the Investors Exchange. Consolidate Tape Association (SIP) provides 15-minute delayed stock prices from all US exchanges.
Who Owns IEX Cloud?
IEX (Investors Exchange), a US-based stock exchange, owns the IEX Cloud.
Who Are IEX Cloud Competitors?
Following are the top competitors to IEX Cloud:
1. Alpha Vantage
2. MarketBeat
3. Finnworlds
What Clients Are Available for IEX Cloud API?
I could not find an official client library for IEX Cloud API. There are some unofficial clients for various programming languages:
1. PyEX
2. IEX.js
3. IEXSharp
4. IEXtrading4j
5. GoInvest
The Bottom Line
The IEX Cloud provides near real-time financial data that guides traders to make better stock purchasing or selling decisions. The IEX Cloud API takes it further and enables traders and developers to develop customized financial software that relies on IEX Cloud data.
In this article, you saw how to fetch cryptocurrency and stock market data from the IEX Cloud API using the Python PyEX and requests library. While the PyEX library abstracts the complexities of calling the IEX Cloud REST API, it doesn’t implement all the endpoints and has some issues with the existing endpoints, as you saw in this article. In such cases, you can revert to the requests library, which can fetch data from all IEX Cloud API endpoints, although a bit more complex.